Streamlining External Application Integration Challenges
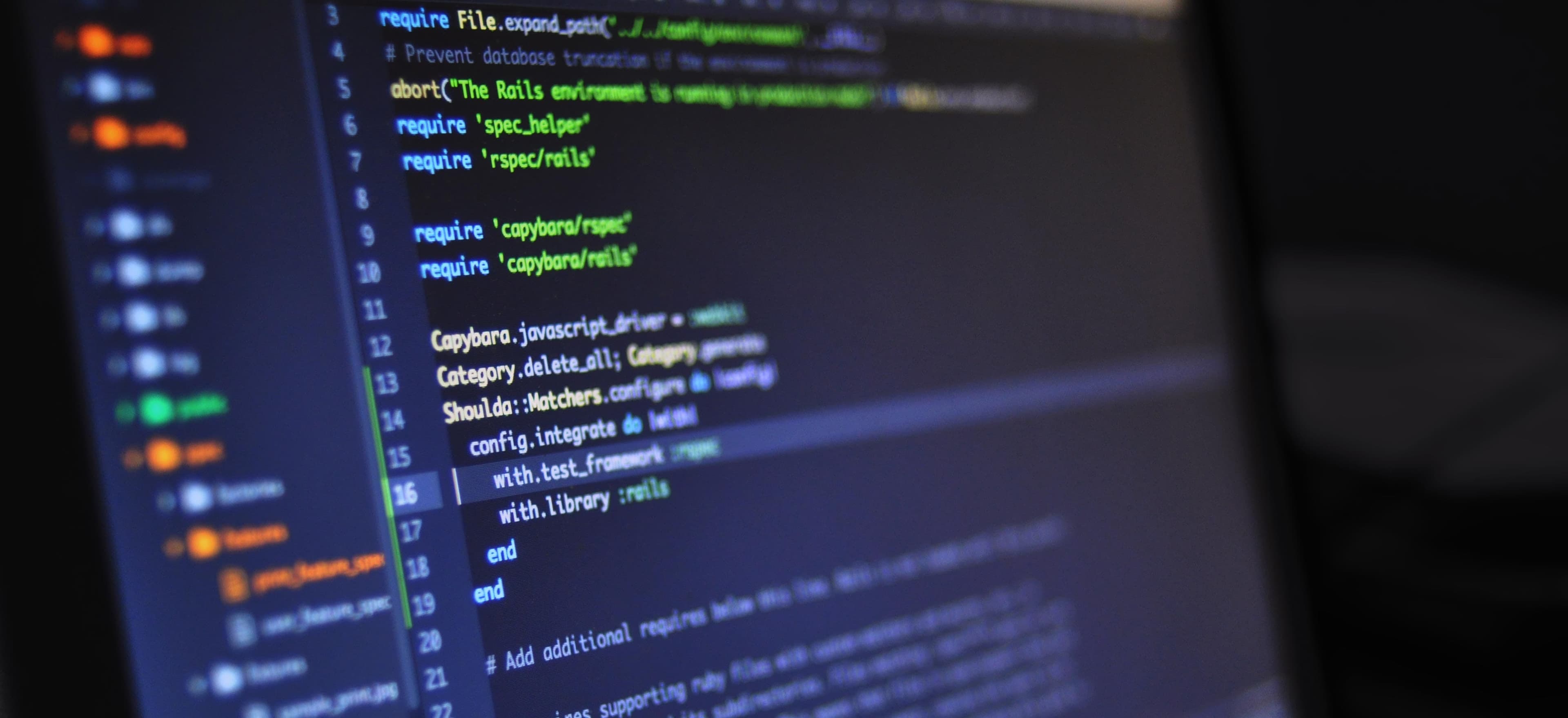
- Published on
Streamlining External Application Integration Challenges
Integrating external applications into your software systems can often be a daunting task. As we move toward a world that increasingly values connectivity, understanding how to effectively integrate disparate services becomes crucial. In this blog post, we will explore the common challenges faced during application integration, best practices for overcoming them, and how Java can be instrumental in streamlining these integrations.
Understanding Application Integration
Application integration refers to the process of enabling independent software applications to connect, share data, and interact with one another. As businesses evolve, they often employ various software solutions tailored for different tasks. This led to the need for these systems to interact seamlessly.
Key Challenges in Application Integration
-
Data Format Incompatibility
One of the most common challenges is the difference in data formats among various applications. JSON, XML, and CSV are common formats that may not be compatible across platforms. -
Network Latency
External applications often reside on different servers, leading to potential delays. Considerations must be made for stable connectivity. -
Security Concerns
When integrating external services, sensitive data is at risk. Securing data during transmission and ensuring compliance with regulations is paramount. -
Version Control Issues
Different applications may evolve at different paces, creating potential compatibility issues. -
Complexity in API Management
Each application might use a different API, complicating the interaction process.
Strategies for Successful Integration
To successfully integrate external applications, consider the following strategies:
1. Use Standard Protocols and Formats
By using universally accepted protocols like REST and SOAP, and data formats like JSON or XML, compatibility issues can be significantly reduced.
2. Implement a Middleware
Middleware acts as a translator between different applications. It can handle data transformation, routing, and orchestration, simplifying the integration process.
3. Focus on Security
Utilizing protocols such as HTTPS, and implementing OAuth for delegated access can help secure your data. Regular audits are essential for compliance.
4. Leverage API Gateways
API gateways streamline the management of APIs, allowing for easier version control, monitoring, and security enforcement.
5. Monitor and Optimize Performance
Continuous monitoring of integration processes can help identify bottlenecks and optimize performance. Utilizing tools for rate limiting and request logging can be beneficial.
How Java Facilitates Integration
Java offers a robust platform with libraries and frameworks designed explicitly for application integration. Here we will examine key tools and techniques in Java that can ease external application integration.
Java Libraries for Integration
- Apache Camel: A powerful open-source integration framework that allows you to define routing and mediation rules in a variety of domain-specific languages, including Java and Spring XML.
- Spring Integration: A part of the Spring Framework, which provides support for building messaging-based architectures.
Sample Code: Using Apache Camel for Integration
Here’s an example of how to set up a simple integration route with Apache Camel.
import org.apache.camel.CamelContext;
import org.apache.camel.impl.DefaultCamelContext;
import org.apache.camel.builder.RouteBuilder;
public class CamelIntegration {
public static void main(String[] args) {
CamelContext context = new DefaultCamelContext();
try {
context.addRoutes(new RouteBuilder() {
@Override
public void configure() {
from("direct:start")
.log("Received: ${body}")
.to("http://example.com/api/data");
}
});
context.start();
Thread.sleep(5000);
context.stop();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why this code is effective:
This code snippet creates a simple Camel route that listens for incoming messages on the direct:start
endpoint, logs the incoming message, and sends it to an external API. This approach highlights the ease of configuration and flexibility Apache Camel offers for managing external integrations.
Handling API Calls with RestTemplate
Java’s RestTemplate class from the Spring framework simplifies communication with HTTP servers. Here’s a quick snippet:
import org.springframework.web.client.RestTemplate;
public class ApiClient {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
String url = "http://example.com/api/data";
String response = restTemplate.getForObject(url, String.class);
System.out.println("Response from external API: " + response);
}
}
Why this code is effective:
The RestTemplate
provides a simple way to call external REST APIs and handle responses. This method reduces boilerplate code and enhances readability, letting developers focus on business logic.
Best Practices for Java Integration
-
Maintain Loose Coupling: Design your services to be loosely coupled to enhance reusability and flexibility.
-
Version Management: Keep your APIs and integrations versioned to avoid breaking changes.
-
Testing: Implement thorough testing, including unit tests, integration tests, and performance tests, to ensure that integrations work as expected before deploying.
-
Error Handling: Implement robust error handling mechanisms. Utilize retries and circuit breakers to manage failures gracefully.
-
Documentation: Maintain clear documentation for APIs and integrations. This aids both development and future troubleshooting.
The Closing Argument
Streamlining external application integration can significantly enhance your software's capabilities and your organization’s operational efficiency. By addressing common challenges and following best practices with powerful Java frameworks and libraries, you can create a reliable and scalable integration layer.
To dive deeper into application integration and its nuances, check out resources like Apache Camel Documentation and Spring Integration Tutorials.
Emphasizing robust security measures, performance monitoring, and optimum use of tools can turn your integration challenges into a strategic advantage. Let integration not be a barrier but a bridge to greater capabilities in your business operations.