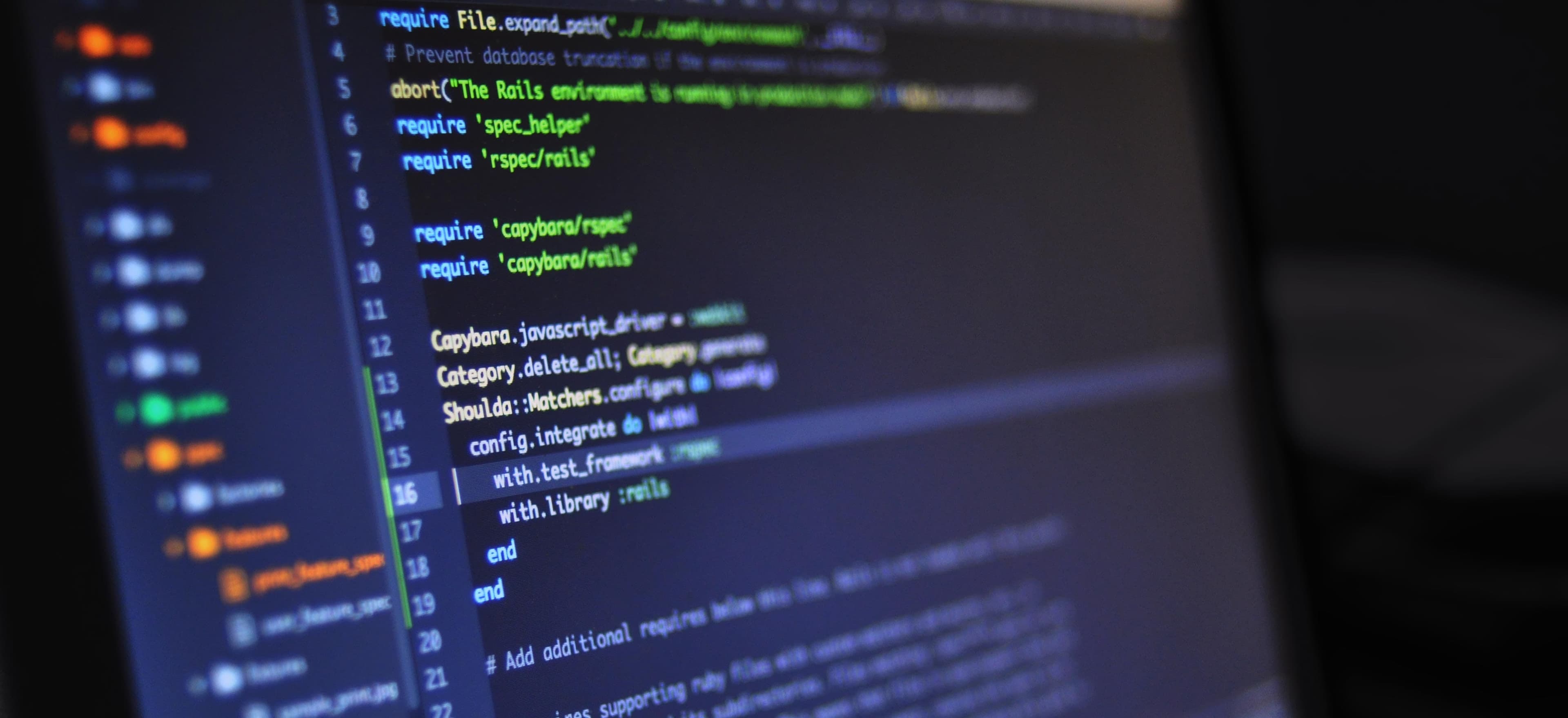
- Published on
Why Choose Servlet Over JSP for Dynamic Web Applications?
When developing dynamic web applications in Java, developers have a choice between Servlets and JavaServer Pages (JSP). While both technologies serve the purpose of generating dynamic web content, there are key differences that might make one a better choice than the other depending on the context and requirements of your project. In this blog post, we'll delve into the reasons why you might choose Servlets over JSP, providing clarity through examples and commentary.
Table of Contents
- Understanding Servlets and JSP
- Key Differences Between Servlets and JSP
- Advantages of Using Servlets
- When to Use JSP
- Final Thoughts
1. Understanding Servlets and JSP
Servlets are Java programs that run on a web server and handle requests and responses between clients (usually web browsers) and servers. They are part of the Java EE specification and are designed to extend the capabilities of servers that host applications accessed via a request-response programming model.
JSP, on the other hand, is a markup language that allows for embedding Java code in HTML pages. JSP essentially gets translated into Servlets by the server, making it easier to create dynamic content in a more visually appealing manner for developers familiar with HTML.
2. Key Differences Between Servlets and JSP
Here’s a quick comparison of the two technologies:
| Feature | Servlet | JSP | |-----------------------|-------------------------------------|---------------------------------------| | Syntax | Java-based | HTML with embedded Java | | Development focus | Logic (code) | Presentation (view) | | Ease of use | More complex | Easier for HTML developers | | Maintenance | Harder due to Java coding | Easier to manage with HTML tags | | Compilation | Compiles at runtime | Compiles once on first request | | Performance | Faster due to pre-compiled code | Slower due to translation to Servlets |
Code Example
@WebServlet("/hello")
public class HelloWorldServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h1>Hello, World!</h1>");
out.println("</body></html>");
}
}
In this example, we define a simple servlet that responds with "Hello, World!" This is a pure Java approach, allowing full control over the generated content.
3. Advantages of Using Servlets
3.1 Fine-Grained Control
Servelts provide finer control over the request-response cycle. It allows for precise handling of HTTP requests, including GET, POST, and custom methods, giving developers the flexibility to manage application flows better.
3.2 Better Performance
Since Servlets are compiled to bytecode once and executed on the server, they are typically faster than JSP pages that need to be compiled into Servlets each time they are accessed.
3.3 Enhanced Security
Servlets allow for a more controlled environment when dealing with user inputs. You can implement detailed request filtering and validation before processing, which helps in building a more secure application.
3.4 Clean Separation of Concerns
By utilizing Servlets, you can achieve a clean separation between your application’s business logic and presentation logic. This contributes to better maintainability and organizes your codebase effectively.
3.5 Scalability
For large-scale applications, Servlets can handle more complex scenarios gracefully. You can implement multiple Servlets designed for specific tasks, enabling better scalability.
Code Snippet with Detailed Logic
@WebServlet("/calculate")
public class CalculationServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int number1 = Integer.parseInt(request.getParameter("number1"));
int number2 = Integer.parseInt(request.getParameter("number2"));
int sum = number1 + number2;
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h1>Sum: " + sum + "</h1>");
out.println("</body></html>");
}
}
In the example above, the servlet calculates the sum of two numbers provided via an HTML form. This demonstrates how Servlets handle input securely and provide output efficiently.
4. When to Use JSP
While Servlets have their strengths, it's essential to consider when using JSP might be more appropriate:
-
Simpler Applications: For simple web applications, where the business logic is minimal, using JSP allows developers to focus on creating user interfaces quickly.
-
Creative Layouts: If your project involves heavy HTML development, JSP tends to be more accommodating. It allows for a quicker turn-around when working on presentations.
-
Combined Logic Needs: If you require quick data visualization along with only minor backend logic, JSP can streamline workflow by integrating both aspects in a single file.
5. Final Thoughts
Choosing between Servlets and JSP largely depends on the needs of your project. Servlets are better suited for applications that require detailed control, efficient performance, and increased security, while JSP is ideal for simpler applications focused primarily on presentation.
Ultimately, understanding the strengths and weaknesses of each technology allows developers to make informed choices that can lead to better-performing and more maintainable web applications. For more insights on Java technologies, consider visiting Oracle’s official Java page.
The power of Java exists not just in its features, but also in its flexibility to adapt to various scenarios. Whether you choose Servlets or JSP, the ultimate goal should always be to build effective, user-friendly web applications.