Navigating Challenges in JAX-RS Hypermedia API Support
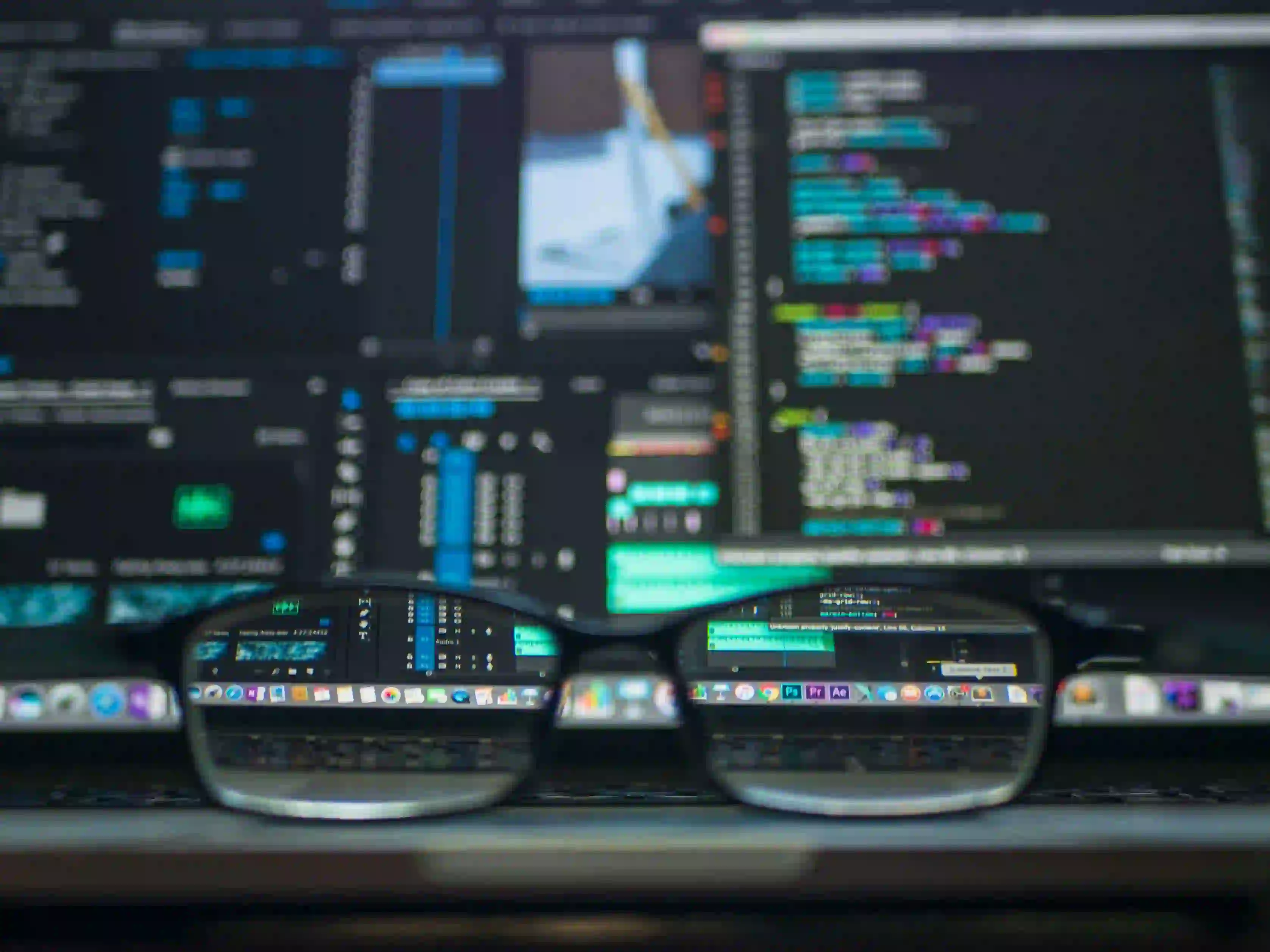
Navigating Challenges in JAX-RS Hypermedia API Support
The Opening Bytes
The evolution of RESTful APIs towards hypermedia-driven approaches has transformed how clients interact with server resources. JAX-RS (Java API for RESTful Web Services) plays an essential role in implementing these RESTful services in Java. However, challenges exist in providing comprehensive support for hypermedia-driven APIs. In this blog post, we will explore these challenges, discuss best practices, and provide exemplary code snippets to help you enhance your understanding of JAX-RS and hypermedia.
What is Hypermedia and Why It Matters
Hypermedia, as defined by the field of hypermedia application protocol (HATEOAS), allows clients to navigate through an API dynamically. In simpler terms, this means that the API serves not only data but also links to other resources. This dynamic navigation helps decouple clients from the API's internal structure and makes APIs more flexible and intuitive.
Here is a simple example of a RESTful response that includes hypermedia links:
{
"id": 1,
"name": "John Doe",
"_links": {
"self": {
"href": "/users/1"
},
"friends": {
"href": "/users/1/friends"
}
}
}
In the above example, the _links
section provides URLs for related resources. This approach empowers clients to explore further without prior knowledge of available endpoints.
The Challenges of Implementing Hypermedia in JAX-RS
While the concept seems straightforward, implementing hypermedia in JAX-RS APIs poses several challenges, including:
1. Designing Hypermedia Models
Creating a model that effectively represents hypermedia can be complex. You need to consider how to structure your resources, define relationships, and maintain readability.
2. Discoverability
Clients should easily discover links to related resources. This means a well-structured API is necessary, often maintained through conventions or documentation.
3. Versioning
As APIs evolve, maintaining backward compatibility while extending hypermedia links can become a daunting task.
4. Client-Side Handling
Clients need to be prepared to manage hypermedia responses. This can complicate client implementation, especially for those who are used to static endpoint definitions.
5. Tooling Support
Many tools and libraries do not have robust support for hypermedia, making it difficult to integrate seamlessly into existing workflows.
Implementing Hypermedia in JAX-RS
Let us walk through a practical implementation of hypermedia within a JAX-RS application while addressing the aforementioned challenges.
Step 1: Set Up Dependencies
Before diving into coding, ensure you have the necessary dependencies. Using Maven, include the following in your pom.xml
:
<dependency>
<groupId>javax.ws.rs</groupId>
<artifactId>javax.ws.rs-api</artifactId>
<version>2.1</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.35</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-jackson</artifactId>
<version>2.35</version>
</dependency>
Step 2: Create a Resource Class
Now, let's create a simple resource class that represents a User
entity and includes hypermedia links.
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.UriBuilder;
import java.net.URI;
@Path("/users")
public class UserResource {
@GET
@Path("/{userId}")
@Produces(MediaType.APPLICATION_JSON)
public Response getUser(@PathParam("userId") int userId) {
User user = new User(userId, "John Doe"); // Simulating a user retrieval
// Creating hypermedia links
URI selfLink = UriBuilder.fromResource(UserResource.class)
.path("/{userId}")
.build(userId);
URI friendsLink = UriBuilder.fromResource(UserResource.class)
.path("/{userId}/friends")
.build(userId);
user.addLink("self", selfLink.toString());
user.addLink("friends", friendsLink.toString());
return Response.ok(user).build();
}
}
Commentary on the Code
- Hypermedia Creation: The links are generated using
UriBuilder
, ensuring that they are constructed correctly based on the resource. - User Representation: The
User
class should have a method,addLink
, which allows adding links dynamically to its representation.
Step 3: User Entity with Hypermedia Support
Here's a basic User
class structure that includes a method for managing links.
import java.util.HashMap;
import java.util.Map;
public class User {
private int id;
private String name;
private Map<String, String> links = new HashMap<>();
public User(int id, String name) {
this.id = id;
this.name = name;
}
public void addLink(String rel, String href) {
this.links.put(rel, href);
}
// Getters for id, name, and links
}
Why This Implementation Matters
This design approach adheres to REST principles by promoting discoverability. Clients calling the GET /users/1
endpoint can easily find related resources, which helps them navigate the API efficiently.
Best Practices for Hypermedia Implementation
Implementing hypermedia APIs requires careful adherence to best practices. Here are a few guidelines:
-
Use Standard Media Types: Employ established media types for your hypermedia. The IANA registry provides a list of these types, such as HAL (Hypertext Application Language) and json:api.
-
Document Your Links: Ensure that your API documentation clearly outlines the structure of hypermedia responses. This is crucial for usability.
-
Client-Side Libraries: Consider using or creating client-side libraries that can easily parse and handle hypermedia responses.
-
Test Extensively: Verify how your API behaves with changes, especially concerning versioning and new hypermedia links.
Bringing It All Together
Navigating the challenges of JAX-RS hypermedia API support can seem daunting, but understanding the principles and employing best practices can significantly enhance your implementation. By adopting hypermedia, you not only enrich your API but also pave the way for a more intuitive client experience.
For further reading on RESTful services and hypermedia, check out Restful Web Services with JAX-RS and Hypermedia as the Engine of Application State.
By integrating these principles into your API development process, you will find yourself better equipped to build robust, user-friendly, and future-proof web services.