Common Pitfalls in Google Cloud Deploy for Java Projects
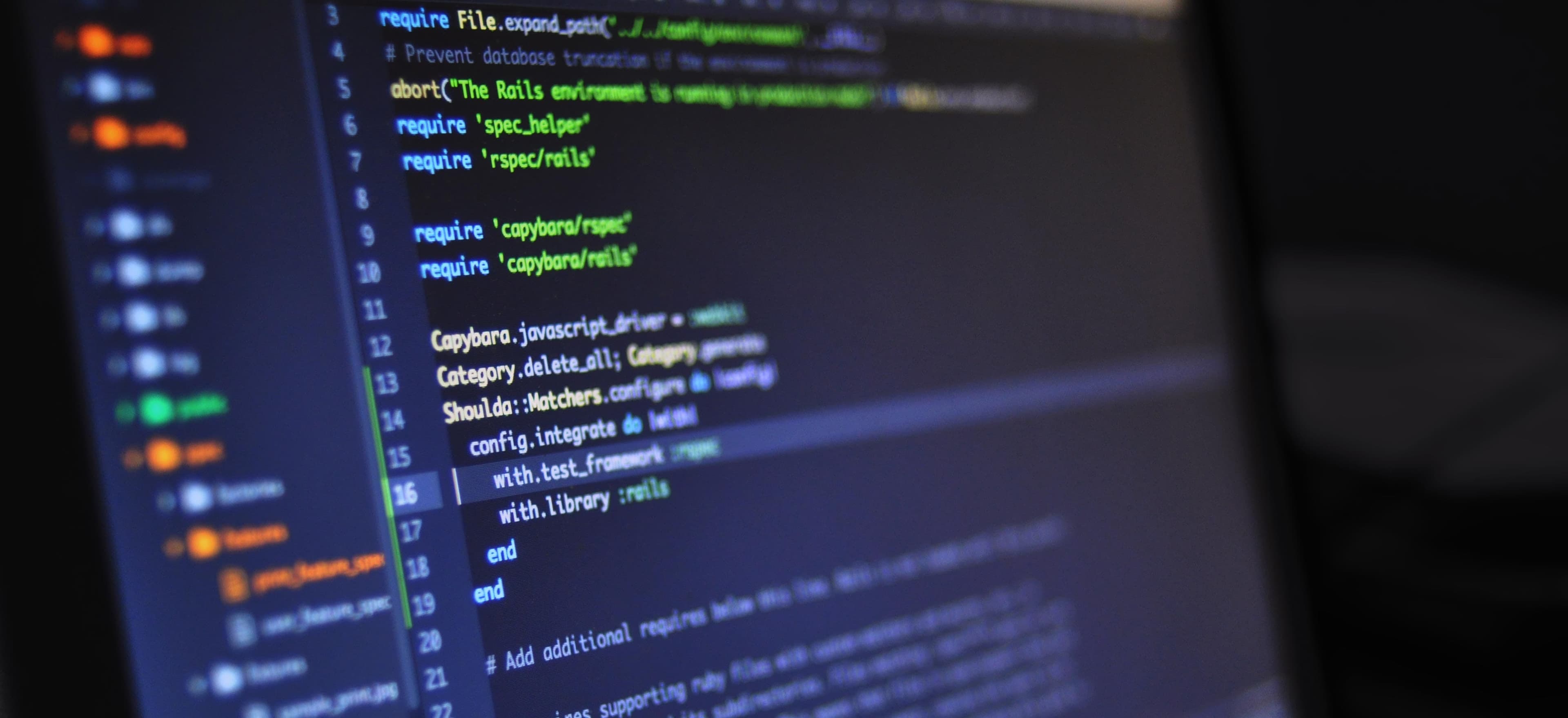
- Published on
Common Pitfalls in Google Cloud Deploy for Java Projects
Deploying Java applications to Google Cloud can be an exciting yet challenging experience. With its robust services and excellent scalability, the Google Cloud Platform (GCP) is a popular choice for developers. However, there are several common pitfalls that developers may encounter during deployment. In this blog post, we’ll explore these issues and offer solutions to help you navigate the deployment process more efficiently.
Understanding Google Cloud Deploy
Google Cloud Deploy is a fully managed continuous delivery service that helps coordinate and automate the delivery of applications on Google Kubernetes Engine (GKE) and other Google Cloud services. It integrates seamlessly with your CI/CD pipeline, providing tools for managing production deployments.
Why Choose Google Cloud for Java Projects?
Java, being a versatile and widely-used programming language, has numerous frameworks that can simplify development. When integrating Java applications with GCP, you can benefit from:
- Scalability: GCP’s infrastructure can scale with your application demands.
- Integrated Tools: GCP offers a suite of tools to facilitate development, monitoring, and logging.
- Global Reach: Deploy your application in multiple regions to optimize performance.
Pitfall 1: Misconfigured IAM Permissions
The Issue
Google Cloud IAM (Identity and Access Management) controls who can access resources in your cloud environment. Many developers overlook configuring the right permissions.
The Solution
Ensure your service account provides the necessary roles to access GCP resources securely.
Example: Grant permissions to allow the compute engine to manage Cloud Storage files.
gcloud projects add-iam-policy-binding your-project-id \
--member serviceAccount:your-service-account@your-project-id.iam.gserviceaccount.com \
--role roles/storage.admin
Why: Using the correct permissions inhibits unnecessary exposures and ensures that only authorized users or services can operate on your resources.
Resource
For a detailed understanding of roles and permissions, check the official IAM documentation.
Pitfall 2: Ignoring Environment Configuration
The Issue
A common mistake that developers make is hardcoding environment variables within the application. This complicates the deployment process and can lead to inconsistent behavior between different environments (development, testing, production).
The Solution
Use GCP's Secret Manager or configuration files to manage environment variables.
Example: Retrieve a secret stored in Secret Manager within your Java application.
import com.google.cloud.secretmanager.v1.*;
public class SecretManagerExample {
public static String accessSecretVersion(String projectId, String secretId, String versionId) {
SecretManagerServiceClient client = SecretManagerServiceClient.create();
SecretVersionName secretVersionName = SecretVersionName.of(projectId, secretId, versionId);
AccessSecretVersionResponse response = client.accessSecretVersion(secretVersionName);
return response.getPayload().getData().toStringUtf8();
}
}
Why: This approach ensures that your secrets remain secure, and it also makes it easy to alter configurations without modifying the application code.
Resource
Explore more about Google Cloud Secret Manager for handling sensitive data effectively.
Pitfall 3: Overlooking Logging and Monitoring
The Issue
Failing to implement logging and monitoring can lead to difficulties in debugging and maintaining your application. Developers often underestimate the importance of these features until an issue arises.
The Solution
Implement GCP's Stackdriver for logging and monitoring right from the start.
Example: Configure Cloud Logging for your Java application.
import com.google.cloud.logging.*;
public class LoggingExample {
public static void main(String[] args) {
Logging logging = LoggingOptions.getDefaultInstance().getService();
LogEntry logEntry = LogEntry.newBuilder(Payload.StringPayload.of("Hello, world!"))
.setSeverity(Severity.INFO)
.setLogName("my-log")
.build();
logging.write(Collections.singleton(logEntry));
}
}
Why: Logging helps capture crucial information that can assist developers in resolving bugs faster. Monitoring allows you to observe application performance and resource usage in real time.
Resource
For in-depth guidance, read the Logging documentation to learn how to set up and use Cloud Logging effectively.
Pitfall 4: Deployment Configuration Missteps
The Issue
Miscalculating resource requirements (CPU, memory, etc.) during configuration can lead to overprovisioning or underprovisioning services. This miscalculation increases costs or causes application performance issues.
The Solution
Use GCP's built-in tools to analyze your application's resource needs and set quotas accordingly.
Example: Utilize Kubernetes resource requests and limits in a deployment YAML file.
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-java-app
spec:
replicas: 3
template:
spec:
containers:
- name: java-app
image: gcr.io/my-project/my-java-app:latest
resources:
requests:
memory: "512Mi"
cpu: "500m"
limits:
memory: "1Gi"
cpu: "1"
Why: Setting requests and limits ensures that your application has the minimum necessary resources to run while preventing it from consuming more resources than required.
Resource
Check out the Kubernetes resource management documentation to delve deeper into resource allocation.
Key Takeaways
Deploying Java applications on Google Cloud can be a powerful way to leverage cloud capabilities. Avoiding the common pitfalls mentioned above will streamline your deployment process and help you create sustainable, scalable, and maintainable applications.
By understanding these challenges and incorporating strategies to address them, you can enhance productivity and ensure a robust deployment environment. Remember, the key to a successful deployment lies in careful planning and continual refinement.
Further Reading
Want to dive deeper into Google Cloud and Java? Check out these resources:
- Google Cloud Java Client Libraries
- Google Kubernetes Engine Introduction
Feel free to share your thoughts on deploying Java applications in the comments below! What pitfalls have you encountered, and how did you resolve them?
Checkout our other articles