Mastering Java Streams: Overcoming SummaryStatistics Pitfalls
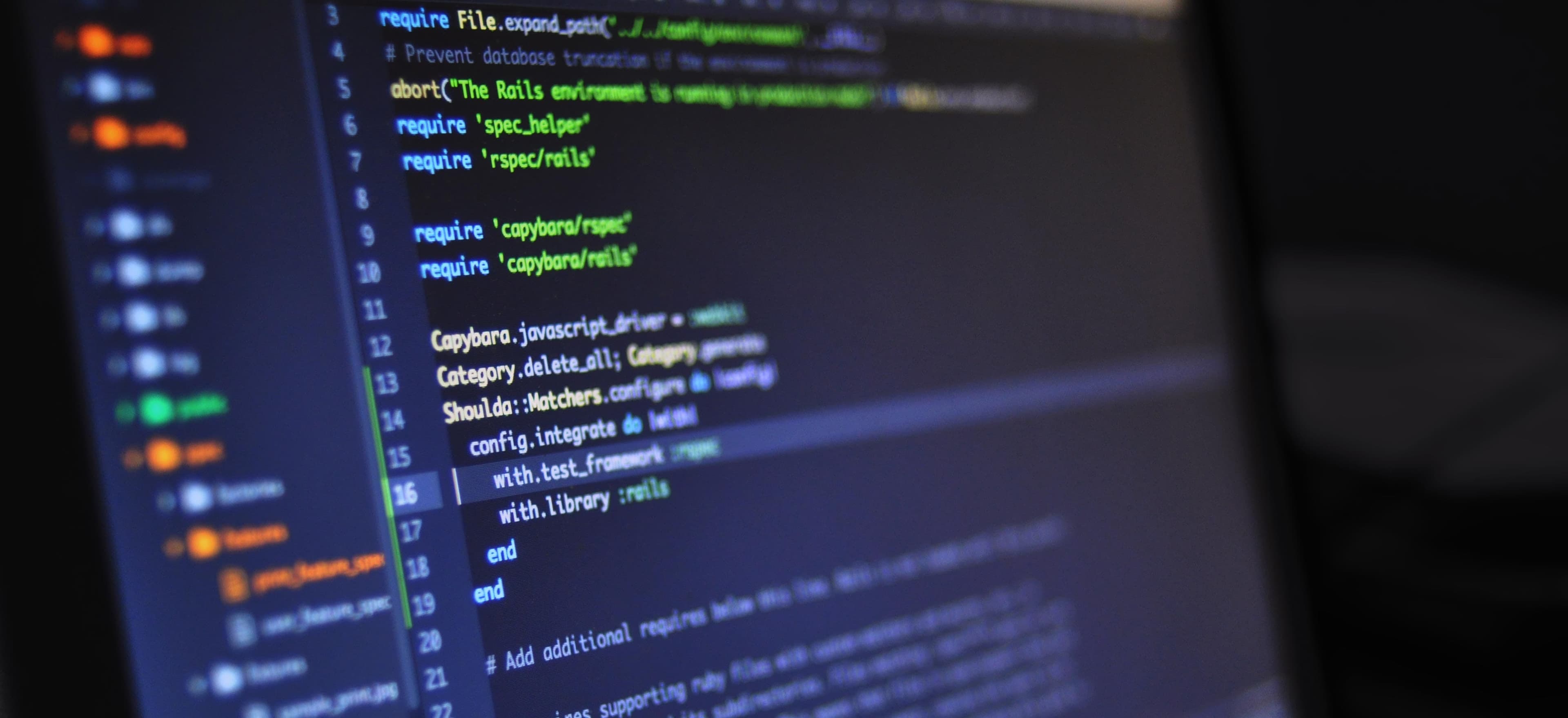
- Published on
Mastering Java Streams: Overcoming SummaryStatistics Pitfalls
Java Streams, introduced in Java 8, revolutionized the way we handle and manipulate collections of data. They allow developers to process sequences of elements in a functional style, enabling operations like filtering, mapping, and reducing. Among the powerful utilities available to us are the SummaryStatistics
class and the various stream functionalities, which can provide us with quick insights into our data. However, while these tools can be immensely helpful, they also come with pitfalls that developers must navigate.
In this blog post, we will dive deep into the SummaryStatistics
class, explore its functionalities, and discuss common pitfalls users face. We'll also provide actionable solutions and best practices to master its usage.
Understanding the Basics of SummaryStatistics
Before we tackle the pitfalls, let’s first familiarize ourselves with the SummaryStatistics
class. This class is part of the java.util
package and is designed to collect statistics such as count, sum, min, average, and max while processing a stream of data.
Here’s a quick example of how SummaryStatistics
works:
import java.util.IntSummaryStatistics;
import java.util.stream.IntStream;
public class SummaryStatisticsExample {
public static void main(String[] args) {
IntSummaryStatistics stats = IntStream.rangeClosed(1, 10)
.summaryStatistics();
System.out.println("Count: " + stats.getCount());
System.out.println("Sum: " + stats.getSum());
System.out.println("Min: " + stats.getMin());
System.out.println("Max: " + stats.getMax());
System.out.println("Average: " + stats.getAverage());
}
}
Explanation
In the example above:
- We create an
IntStream
of integers from 1 to 10. - We call the
summaryStatistics()
method to get anIntSummaryStatistics
object. - We then print various statistics collected from the stream.
This is a great first step, but let's discuss common pitfalls developers encounter when utilizing SummaryStatistics
and how to overcome them.
Common Pitfalls with SummaryStatistics
Pitfall 1: Incomplete Data
One major pitfall occurs when the data being analyzed is incomplete or filtered out during stream processing. This can lead to misleading statistics.
Solution
To mitigate this, always check the count before performing operations on the summary statistics. For example:
import java.util.IntSummaryStatistics;
import java.util.stream.IntStream;
public class ImprovedSummaryStatistics {
public static void main(String[] args) {
IntSummaryStatistics stats = IntStream.rangeClosed(1, 20)
.filter(n -> n % 2 == 0) // Filtering even numbers
.summaryStatistics();
if (stats.getCount() > 0) {
System.out.println("Average: " + stats.getAverage());
} else {
System.out.println("No data available.");
}
}
}
Pitfall 2: Overuse in Multiple Operations
Many developers mistakenly use SummaryStatistics
in multiple terminal operations, causing performance overhead since it calculates statistics multiple times.
Solution
It is efficient to collect statistics in one go and store them in a variable. Here is an improved approach:
import java.util.IntSummaryStatistics;
import java.util.stream.IntStream;
public class EfficientSummaryStatistics {
public static void main(String[] args) {
IntSummaryStatistics stats = IntStream.rangeClosed(1, 1000)
.filter(n -> n % 3 == 0)
.summaryStatistics();
System.out.println("Count: " + stats.getCount());
System.out.println("Sum: " + stats.getSum());
System.out.println("Average: " + stats.getAverage());
System.out.println("Max: " + stats.getMax());
}
}
Pitfall 3: Ignoring Precision
In some cases, the average calculated can be frail due to integer calculations. This can lead to incorrect results if you're not careful with data types.
Solution
Use double
streams when you anticipate fractions for averaging. Here’s an example:
import java.util.DoubleSummaryStatistics;
import java.util.stream.DoubleStream;
public class PrecisionInStatistics {
public static void main(String[] args) {
DoubleSummaryStatistics stats = DoubleStream.of(1.5, 2.5, 3.0, 4.0)
.summaryStatistics();
System.out.println("Average: " + stats.getAverage()); // Should provide precise average.
}
}
Best Practices for Using SummaryStatistics
1. Stream Only Once
Always use the stream only once to avoid redundant calculations. Store the SummaryStatistics
result if you need to access the data multiple times.
2. Stream Composition
If you have complex stream processing, consider using method references or lambda expressions to improve code clarity and maintainability.
3. Test for Edge Cases
Always account for edge cases. The count should be checked before performing operations to prevent NoSuchElementException
.
4. Use Additional Libraries When Necessary
For more complex statistical requirements, consider libraries such as Apache Commons Math or JFreeChart, which offer extensive statistical capabilities beyond what’s available in the Java standard library.
Final Considerations
Mastering Java Streams and SummaryStatistics
can greatly enhance your data processing capabilities. By recognizing common pitfalls and adhering to best practices, you can avoid falling into traps that can lead to inefficient code or incorrect data interpretations.
Working with data is a powerful skill in today’s development landscape. By equipping yourself with the right knowledge and tools, you can streamline your programming workflow and improve the quality of your applications. For further reading, you can explore the Java documentation on Streams or get insights from the Java Tutorials.
Feel empowered to tackle your data processing needs using these guidelines, and happy coding!