Mastering Timeout Configurations in Spring Cloud Zuul
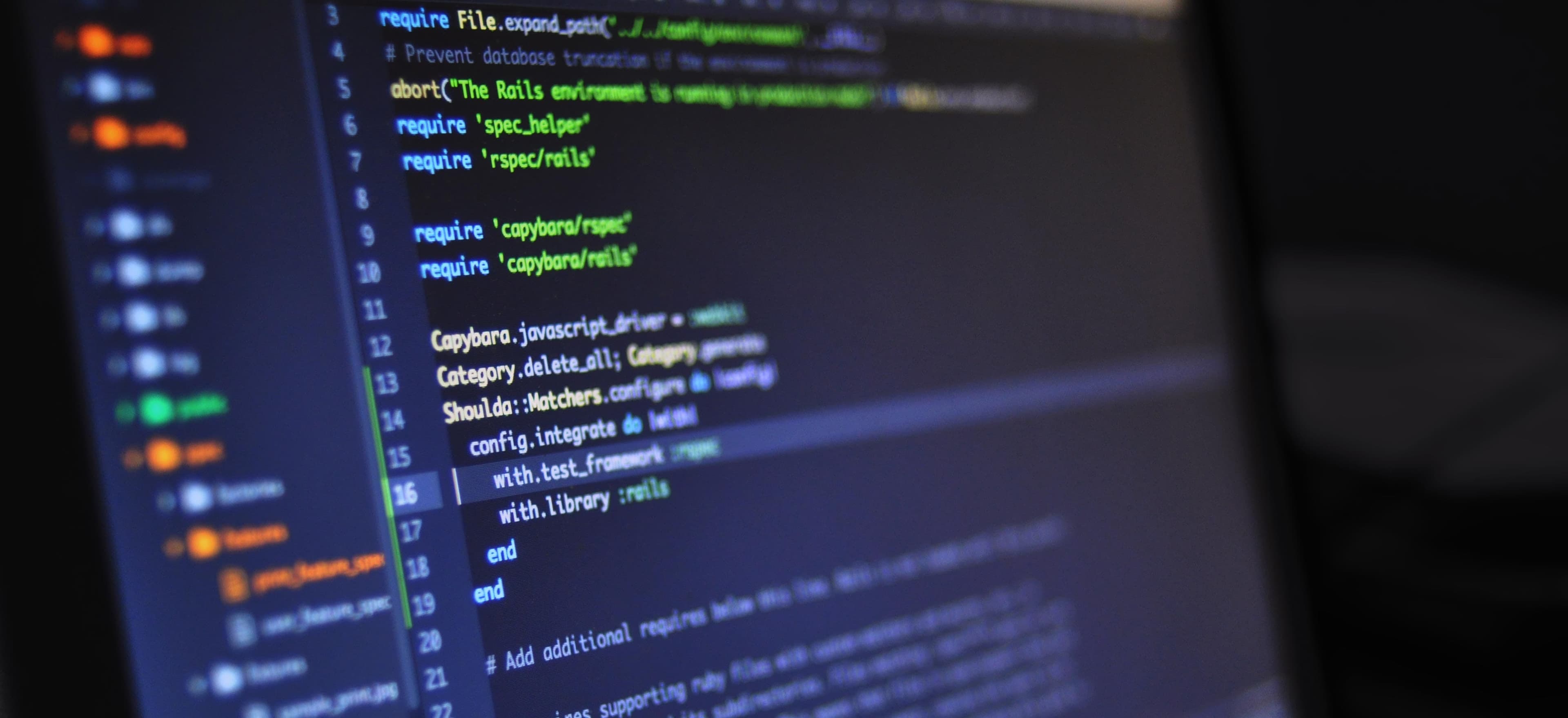
- Published on
Mastering Timeout Configurations in Spring Cloud Zuul
Spring Cloud Zuul acts as an edge service that provides dynamic routing, monitoring, resilience, and security for microservices. While Zuul simplifies routing, it is essential to keep in mind that effective timeout configurations are crucial for ensuring a reliable and performant application.
In this blog post, we will explore how to configure timeouts in Spring Cloud Zuul and the implications of these settings. We will also discuss some best practices to keep your application resilient and user-friendly.
Understanding Timeouts in Zuul
Timeouts are essential in distributed systems. They help prevent cascading failures and promise a good user experience by avoiding long waits for responses that won't arrive. In Zuul, timeouts can be configured for both the client and the server.
Here's a basic categorization:
- Connection Timeout: The time to wait when establishing a connection.
- Socket Timeout: The time to wait for data after the connection is established.
Why Are Timeouts Important?
- Resource Management: Improper timeout settings can lead to exhaustion of system resources.
- User Experience: Longer hanging times generate user frustration.
- Service Resilience: Using the right timeouts helps in maintaining application stability.
Configuring Timeout Settings
In a Spring Boot application integrated with Zuul, timeout configurations can be set in the application.yml
(or application.properties
) file. Below are some typical configurations you can implement:
Example Configuration
zuul:
routes:
# Route definition here
exampleService:
path: /example/**
url: http://localhost:8081
# Timeout settings
serviceId: exampleService
connect-timeout-millis: 5000 # 5 seconds
socket-timeout-millis: 10000 # 10 seconds
Explanation
- path: Maps the internal route to the service URL.
- url: The actual URL to which it routes the request.
- connect-timeout-millis: Limits the time allowed for the connection to be established.
- socket-timeout-millis: Limits the time allowed for data to be read after establishing the connection.
Connecting to Micronaut with Zuul
If you're running a Micronaut service behind Zuul, you can configure timeouts similarly. Here’s how you might handle it:
zuul:
routes:
micronautService:
path: /micronaut/**
url: http://localhost:8082
serviceId: micronautService
connect-timeout-millis: 3000 # 3 seconds
socket-timeout-millis: 6000 # 6 seconds
Handling Timeouts Programmatically
Sometimes, you may require programmatic control over the timeout configurations in Zuul, especially if various conditions dictate specific behaviors. This can be achieved by setting properties within your Java code.
Java Configuration
import org.springframework.cloud.netflix.zuul.filters.post.SendErrorFilter;
import org.springframework.cloud.netflix.zuul.filters.route.RibbonRoutingFilter;
import org.springframework.cloud.netflix.zuul.filters.route.SimpleRouteLocator;
@Configuration
public class ZuulConfig {
@Bean
public RibbonRoutingFilter ribbonRoutingFilter() {
RibbonRoutingFilter filter = new RibbonRoutingFilter();
filter.setConnectTimeout(5000); // 5 seconds
filter.setReadTimeout(10000); // 10 seconds
return filter;
}
}
Explanation
This snippet provides a programmatic way to define the timeout settings, making your Zuul configuration more dynamic and adaptable.
Best Practices for Timeout Management
-
Understand Service Dependencies: Analyze the typical response times and set reasonable timeouts accordingly. Use tools such as Spring Cloud Sleuth for distributed tracing.
-
Gradual Backoffs: Implement exponential backoff strategies for retries in case of timeouts. This can gracefully handle services that may be temporarily unavailable.
-
Monitoring: Use monitoring tools with alerts for errors and timeouts to quickly identify issues. Integrate with Spring Boot Actuator for health-checking endpoints and metrics.
-
Fallback Strategies: Implement a fallback strategy for user requests that may fail due to timeouts. You can return cached responses or default messages.
-
Load Testing: Regularly perform load testing on your services to better understand their behavior under stress, helping you identify optimal timeout settings.
In Conclusion, Here is What Matters
Mastering timeout configurations in Spring Cloud Zuul plays a crucial role in microservices architecture. An appropriate setup can bolster user experience, optimize resource management, and enhance service resilience.
Start by defining your timeout values based on the specifics of your application and its dependencies. Continuous monitoring and iterative improvements will ensure you maintain a healthy system.
If you need to further dive into Spring Cloud and Zuul, we recommend exploring the official Spring Cloud documentation. Happy coding!
By following this guide, you can analyze and configure timeout settings in Zuul effectively, paving the way for a performant and resilient microservices architecture.