Common Pitfalls in HATEOAS API Development with JAX-RS
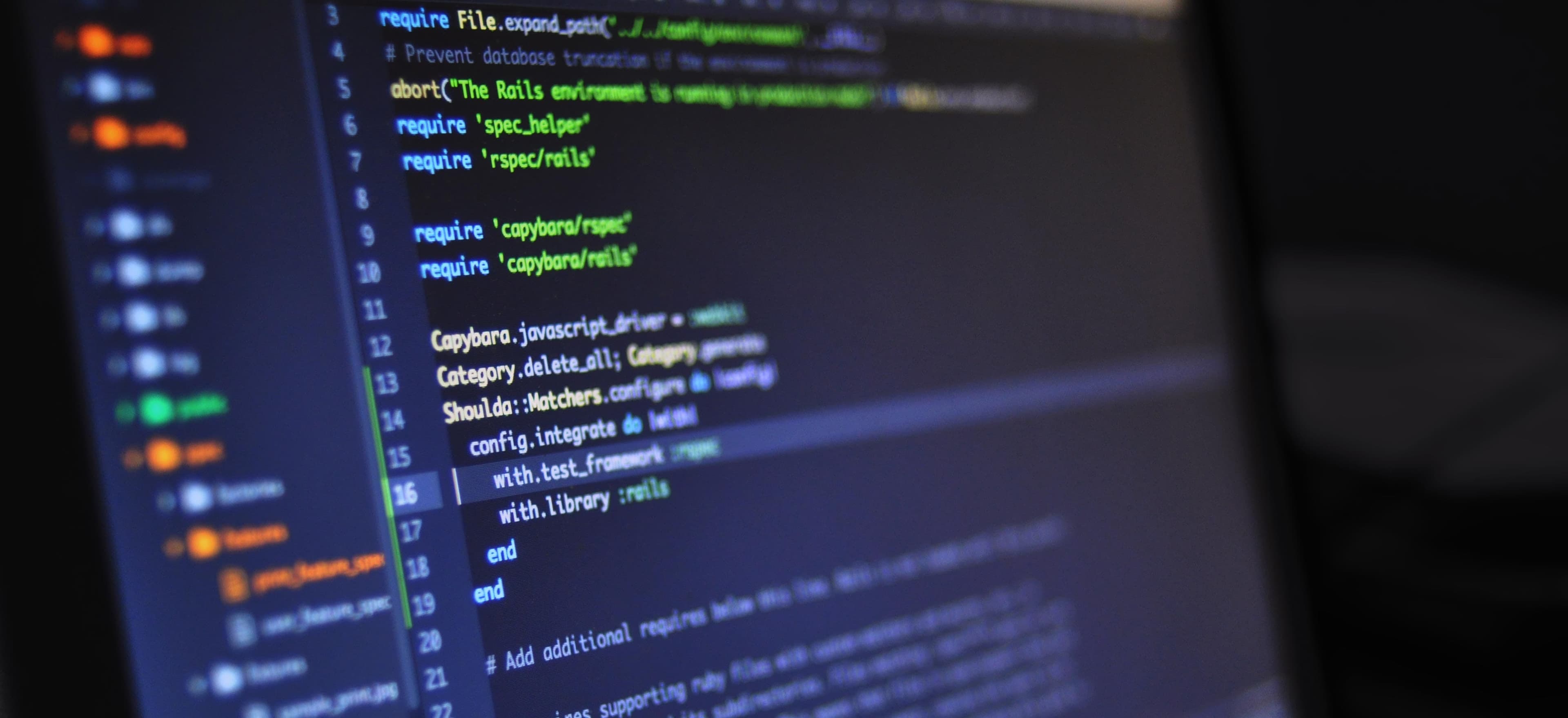
- Published on
Common Pitfalls in HATEOAS API Development with JAX-RS
The Starting Line
Hypermedia as the Engine of Application State (HATEOAS) is a crucial aspect of RESTful web services, allowing for responsive and self-descriptive APIs. It promotes discoverability and enables clients to understand how to navigate through the API landscape without prior knowledge of its structure. However, developing HATEOAS-compliant APIs can be challenging, especially when using Java technologies like JAX-RS. In this blog post, we will explore common pitfalls in HATEOAS API development with JAX-RS, along with best practices and code examples to help you avoid these traps.
Understanding HATEOAS
Before diving into pitfalls, it's important to clarify what HATEOAS is. According to the REST architecture, a client interacts with the server entirely through hypermedia. This means that clients can navigate through resources using links provided by the server responses. When done right, HATEOAS improves flexibility and decouples clients from server-side changes, enabling a more resilient architecture.
Common Pitfalls in HATEOAS API Development
1. Not Returning Links in Responses
One of the most overlooked aspects of HATEOAS is the inclusion of hypermedia links in the API responses. It is crucial that each representation returned by your API includes URLs to relevant actions.
Example:
Here’s a simple example of how to include links in a JAX-RS resource:
@GET
@Path("/users/{id}")
@Produces(MediaType.APPLICATION_JSON)
public Response getUser(@PathParam("id") Long id) {
User user = userService.findById(id);
if (user == null) {
return Response.status(Response.Status.NOT_FOUND).build();
}
// Create links for related resources
Link profileLink = Link.fromUriBuilder(
uriInfo.getAbsolutePathBuilder().path("profile").build())
.rel("profile").build();
Link ordersLink = Link.fromUriBuilder(
uriInfo.getAbsolutePathBuilder().path("orders").build())
.rel("orders").build();
// Build the response
return Response.ok(
new UserRepresentation(user, Arrays.asList(profileLink, ordersLink)))
.build();
}
Why This Matters
Failing to return links prevents clients from understanding how to navigate the API effectively. They may know how to access one resource, but how do they find others? Each object should come with its state change links.
2. Not Standardizing Link Relations
When designing your API, it's essential to standardize the link relations. Using inconsistent or non-descriptive relation names (e.g., "next," "prev," "related") can create confusion.
Example:
Link nextLink = Link.fromUriBuilder(
uriInfo.getAbsolutePathBuilder().queryParam("page", nextPage).build())
.rel("next").build();
// A better approach would involve using a clearly defined namespace.
Link userLink = Link.fromUri("https://api.example.com/users/" + userId)
.rel("user").build();
Why This Matters
Using descriptive relation names allows clients to understand the actions available to them. It also allows for automated documentation tools to generate user-friendly API documentation. Consider using established standards, like IANA Link Relations, for consistency.
3. Ignoring Media Types
Often, developers overlook the importance of media types in API responses. When you return a resource, the media type should not only indicate the data format but also convey the specific representations supported.
Example:
@Produces({"application/vnd.api+json", "application/json"})
@GET
@Path("/products/{productId}")
public Response getProduct(@PathParam("productId") Long productId) {
Product product = productService.findById(productId);
if (product == null) {
return Response.status(Response.Status.NOT_FOUND).build();
}
Link selfLink = Link.fromUriBuilder(
uriInfo.getAbsolutePathBuilder()).rel("self").build();
return Response.ok(new ProductRepresentation(product, selfLink))
.type("application/vnd.api+json").build();
}
Why This Matters
Specifying the media type enables clients to handle responses correctly and adapt to different representations as necessary. A well-defined media type can also help in achieving API versioning while maintaining backward compatibility.
4. Hardcoding URLs
Hardcoding URLs in your API can lead to significant maintenance challenges. If a base URL changes or the structure of the endpoint undergoes modifications, it may break all clients.
Example:
Instead of hardcoding:
Link selfLink = Link.fromUri("http://example.com/api/products/" + productId).rel("self").build();
Adopt URI building strategies:
Link selfLink = Link.fromUriBuilder(uriInfo.getAbsolutePath()).rel("self").build();
Why This Matters
Dynamic generation of URLs based on the request context safeguards your API against unintended breakages. It allows your API to evolve without needing each client to update their hardcoded links.
5. Neglecting Client Discovery
Although your API might be compliant with HATEOAS, if clients cannot easily discover it, it’s of no use. This aspect often requires keeping a balance between self-documenting API responses and creating comprehensive documentation along with an API specification.
Why This Matters
When clients can discover available endpoints easily, it improves their experience. Clear documentation also ensures that new developers can quickly understand how to integrate with your API, reducing the learning curve.
Final Considerations
Incorporating HATEOAS into your JAX-RS API development is a significant step toward creating flexible, discoverable, and user-friendly APIs. By avoiding the common pitfalls outlined in this blog post, you can construct robust APIs that meet the standards of modern application architecture. For further reading on RESTful web services and HATEOAS, check out resources on RESTful API Design and Best Practices for HATEOAS.
Additional Resources
- JAX-RS Documentation
- Understanding HATEOAS
By keeping these principles in mind and crafting an excellent HATEOAS implementation, you can ensure that your APIs are not just functional but also user-friendly and adaptable to the evolving requirements of clients and services. Happy coding!