Mastering Recursive Techniques to Reverse a String
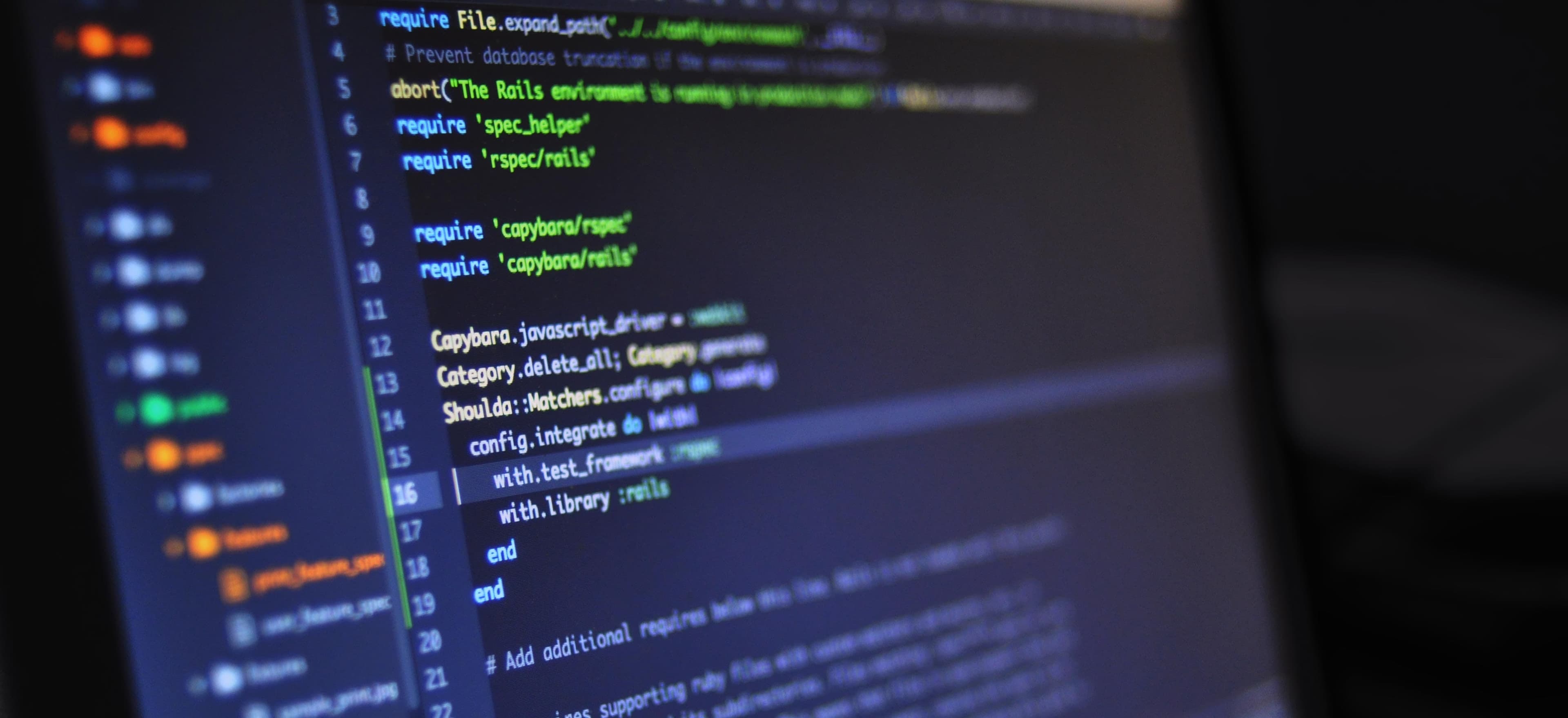
- Published on
Mastering Recursive Techniques to Reverse a String in Java
Reversing a string is a common exercise for programmers and is often used as an introduction to recursion. In this blog post, we'll explore how to reverse a string using recursive techniques in Java. We'll discuss the concept of recursion, walk through the code, and analyze its effectiveness.
Understanding Recursion
Recursion is a programming technique where a method calls itself to solve a problem. It is particularly useful in scenarios where a problem can be broken down into smaller sub-problems. This approach can yield elegant and simple solutions for problems that may seem complex at first.
Before diving into the code, let's establish a basic understanding of how a string reversal works. Consider the string "hello". The reversed string should be "olleh". Looking at it recursively, the last character can be removed, and the process is repeated until the string is empty.
Why Choose Recursion for String Reversal?
- Simplicity: The recursive solution often involves less code and can be easier to understand.
- Alignment with Mathematical Principles: Recursion mirrors mathematical definitions, making it an intuitive approach for those familiar with math.
- Breaking Down Problems: Recursion allows complex problems to be simplified into smaller, more manageable pieces.
With these advantages in mind, let's take a look at how we can implement a recursive function to reverse a string in Java.
The Recursive Solution
Code Implementation
public class StringReversal {
public static String reverseString(String str) {
// Base case: if the string is empty or has one character, return it
if (str.isEmpty()) {
return str;
}
// Recursive case: take the last character and append it to the reversed substring
return str.charAt(str.length() - 1) + reverseString(str.substring(0, str.length() - 1));
}
public static void main(String[] args) {
String original = "hello";
String reversed = reverseString(original);
System.out.println("Original String: " + original);
System.out.println("Reversed String: " + reversed);
}
}
Explanation of the Code
-
Base Case: The method first checks if the string is empty. If it is, the function returns the string itself. This is crucial to prevent infinite recursion.
-
Recursive Case: The method appends the last character of the current string (
str.charAt(str.length() - 1)
) to the result of the recursive call, which processes the substring without the last character (str.substring(0, str.length() - 1)
). -
Output: In the
main
method, we test thereverseString
function with the string "hello". The output confirms that the string has been reversed correctly.
The Power of Recursion
The beauty of recursion lies not just in its ability to simplify code but also in its ability to solve complex problems with elegant solutions. When employing recursion, it's essential to consider the efficiency and stack depth used during execution. Java has a default stack size limit, and extensive recursion can lead to StackOverflowError
.
For practical string reversal tasks, recursion is convenient, but for longer strings, iterative solutions generally perform better in terms of both time complexity and memory usage.
Exploring Alternatives: Iteration vs. Recursion
While recursion is an elegant solution, iterative approaches can be more efficient. Let's explore both methods side by side.
Iterative Solution
public class StringReversal {
public static String reverseStringIteratively(String str) {
StringBuilder reversed = new StringBuilder();
for (int i = str.length() - 1; i >= 0; i--) {
reversed.append(str.charAt(i));
}
return reversed.toString();
}
public static void main(String[] args) {
String original = "hello";
String reversedIterative = reverseStringIteratively(original);
System.out.println("Original String: " + original);
System.out.println("Reversed String (Iterative): " + reversedIterative);
}
}
Summary of Iterative Solution
-
StringBuilder: Instead of concatenating strings (which can be inefficient), we use a
StringBuilder
, which is mutable and helps to improve performance. -
Loop: A for-loop runs backward through the original string, allowing characters to be appended in reverse order.
Performance Comparison
- Time Complexity: Both methods have a time complexity of O(n) where n is the length of the string.
- Space Complexity: Recursive solutions generally have higher space complexity due to call stack usage, whereas iterative solutions use only O(1) additional space, making them more memory efficient for larger strings.
Wrapping Up
Reversing a string with recursive techniques provides a unique perspective on problem-solving in programming. While the recursive approach is elegant and straightforward, understanding the potential performance implications is crucial when dealing with larger data sets.
Further Reading
- Recursion in Java - A comprehensive guide on recursion in Java.
- String Manipulation in Java - Explore various methods for string handling in Java.
By mastering these techniques, you not only become adept at string manipulation but also enhance your overall programming skills in Java. Happy coding!