Managing Concurrency: AtomicInteger in Round Robin Scheduling
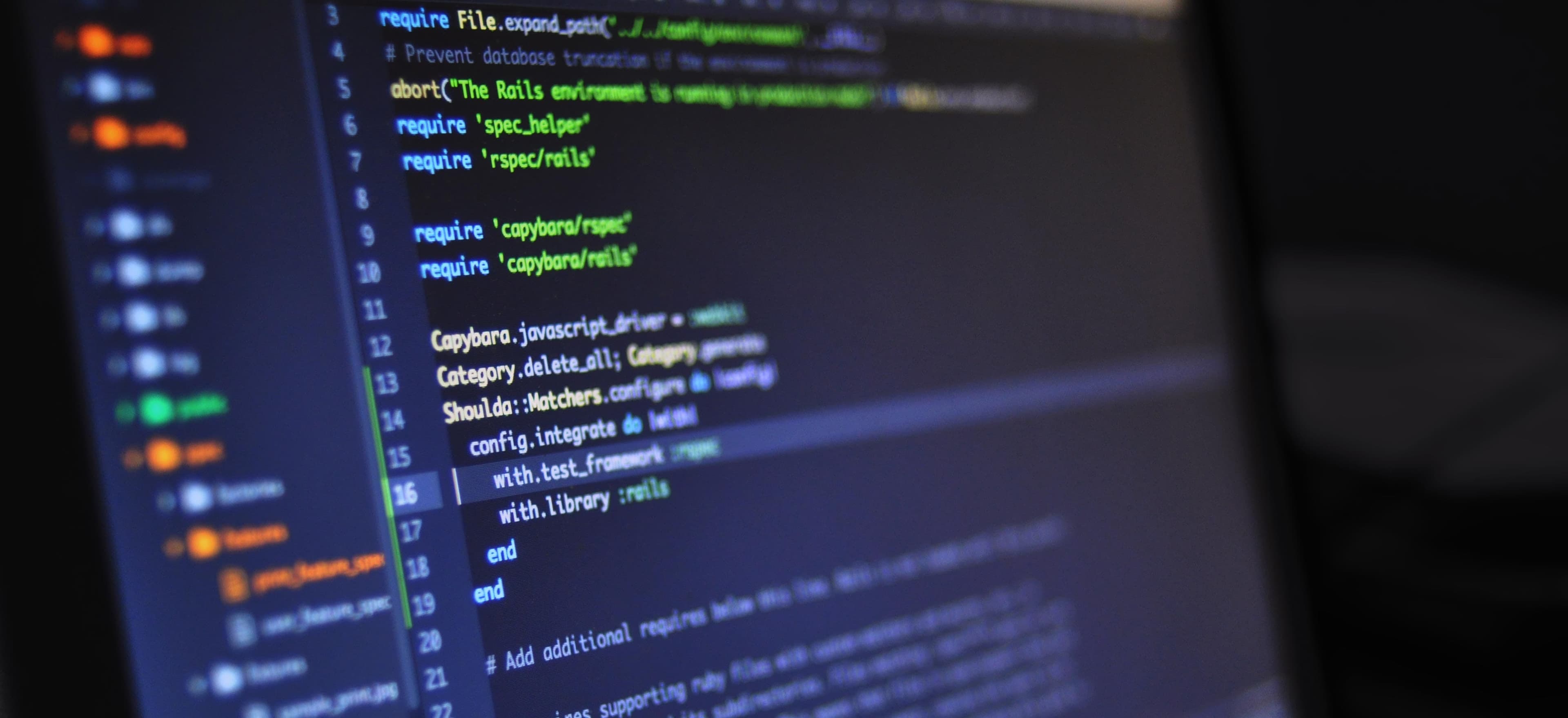
- Published on
Managing Concurrency: AtomicInteger in Round Robin Scheduling
Concurrency in programming is a challenging yet intriguing topic. It involves handling multiple threads simultaneously while ensuring that shared data remains consistent. In this post, we will explore the concept of round-robin scheduling and how AtomicInteger
helps us manage concurrency in a thread-safe manner.
What is Round Robin Scheduling?
Round Robin is one of the simplest and most widely used CPU scheduling algorithms. It assigns a fixed time unit per process and cycles through the processes. Each process is given a small time slice (or quantum) to execute. If it does not finish within that time, it is preempted and moved to the end of the queue.
Why Use Round Robin Scheduling?
- Fairness: Every process gets an equal opportunity to execute.
- Simplicity: The algorithm is straightforward to implement.
- Predictability: Time sharing improves response time in multi-user environments.
However, managing this scheduling method efficiently in a multi-threaded environment can be challenging.
The Need for Concurrency Control
In concurrent programming, multiple threads can attempt to read and write to shared variables simultaneously. Without proper synchronization, this can lead to inconsistent or corrupted data. This is where the AtomicInteger
class in Java comes into play.
What is an AtomicInteger?
AtomicInteger
is part of the java.util.concurrent.atomic
package. It provides a way to create an integer variable that can be safely updated by multiple threads. The key advantage is that it uses low-level atomic operations to perform updates, making it significantly faster than using synchronized blocks.
Benefits of Using AtomicInteger
- Thread Safety: Operations are atomic, preventing data inconsistency.
- Performance: Reduces overhead from synchronization.
- Simplicity: Requires less boilerplate code compared to synchronized methods.
Implementing Round Robin Scheduling with AtomicInteger
In this section, we will implement a simple round-robin scheduler using AtomicInteger
. We will have multiple threads representing different tasks that need to be scheduled.
Basic Structure
We will define three main components:
- Task: Represents the work to be performed by each thread.
- Scheduler: Manages the task execution in a round-robin fashion.
- Main Application: Initializes tasks and starts the scheduler.
Code Implementation
Here's how we can implement this:
import java.util.concurrent.atomic.AtomicInteger;
public class RoundRobinScheduler {
private final AtomicInteger currentTaskIndex = new AtomicInteger(0);
private final Task[] tasks;
public RoundRobinScheduler(Task[] tasks) {
this.tasks = tasks;
}
public void schedule() {
while (true) {
int index = currentTaskIndex.getAndUpdate(i -> (i + 1) % tasks.length);
Task task = tasks[index];
task.execute();
}
}
public static void main(String[] args) {
Task[] tasks = {
new Task("Task 1"),
new Task("Task 2"),
new Task("Task 3")
};
RoundRobinScheduler scheduler = new RoundRobinScheduler(tasks);
new Thread(scheduler::schedule).start();
}
}
class Task {
private final String name;
public Task(String name) {
this.name = name;
}
public void execute() {
System.out.println(name + " is executing.");
try {
Thread.sleep(500); // Simulate task execution time
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Explanation of the Code
-
AtomicInteger Usage: The
currentTaskIndex
is initialized as anAtomicInteger
. This design allows for concurrent updates, ensuring each thread gets a fair shot at executing tasks. -
Task Execution: The
schedule
method increments the index atomically and retrieves the current task. By wrapping the index update ingetAndUpdate
, we ensure that any thread accessing this will get the updated index. -
Thread Execution: We create a new thread that runs the scheduler indefinitely. Each task simulates work by sleeping for 500 milliseconds.
Why AtomicInteger?
Using AtomicInteger
in our scheduler allows us to safely manage the index across multiple threads without the need for explicit synchronization. This results in more efficient execution, as threads do not have to wait on object locks.
Potential Improvements
While the above example is a decent starting point for a round-robin scheduling system, it can still be improved:
- Dynamic Task Addition: Tasks could be added dynamically to the scheduler as they arrive.
- Handling Task Completion: Implement logic to complete tasks rather than endlessly looping.
- Enhanced Scheduling: Consider incorporating priorities or varying time slices.
Closing the Chapter
Managing concurrency can be complex, especially with algorithms like round-robin scheduling. Leveraging Java's AtomicInteger
allows for efficient updates without the overhead of synchronization locks. This ensures that multiple threads can interact with shared data safely and effectively.
For further reading on Java concurrency, consider exploring the Java Concurrency in Practice book, which provides insights into safely building concurrent applications.
By understanding the significance of atomic variables, you can elevate your multi-threaded applications. Start implementing these concepts and experience the elegance of thread-safe Java programming firsthand!