Resolving the UnknownEntityException in Hibernate
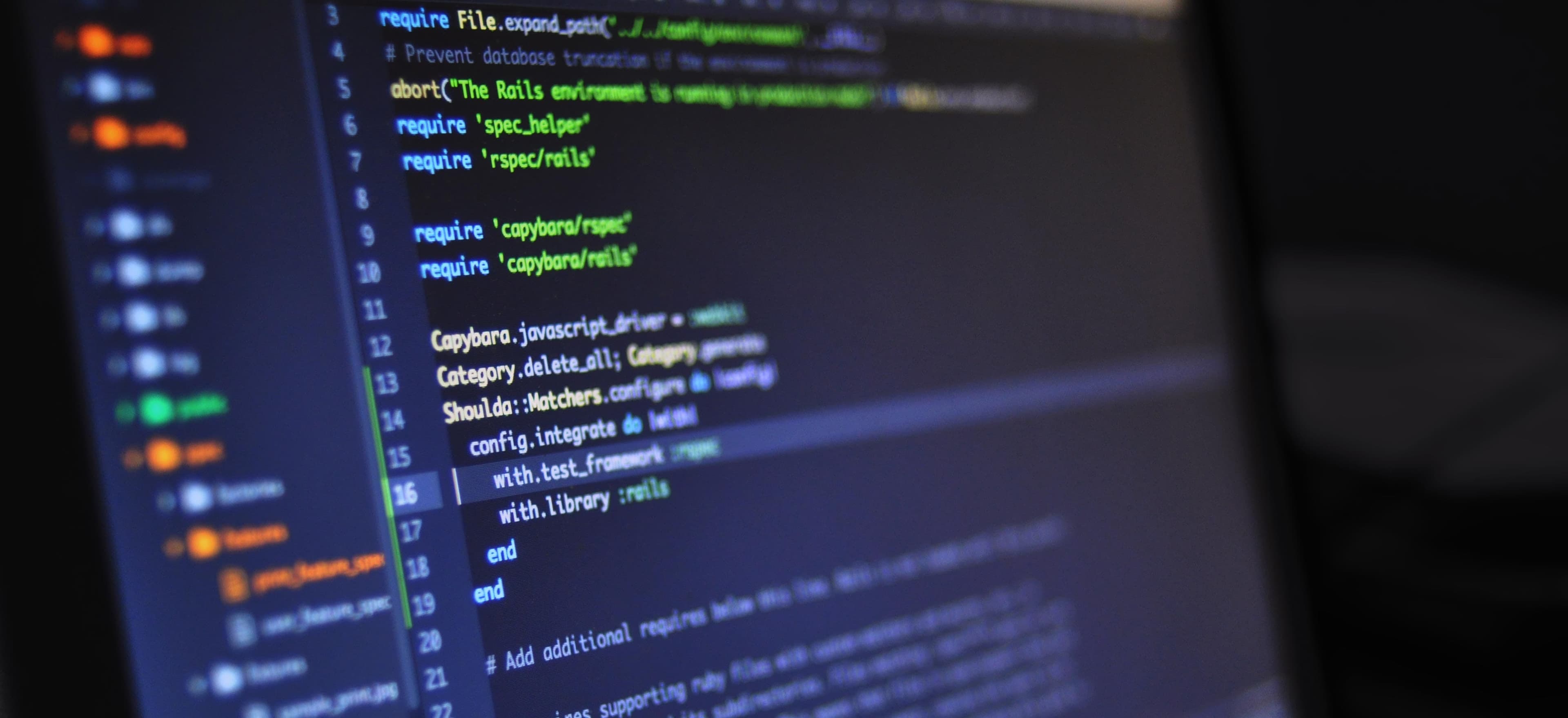
- Published on
Resolving the UnknownEntityException in Hibernate
Hibernate is a powerful ORM solution for Java applications that allows developers to interact with databases in an object-oriented manner. However, as with any framework, developers may run into challenges or exceptions. One common exception in Hibernate is the UnknownEntityException
. In this article, we will delve into what this exception is, why it occurs, and how to effectively resolve it.
Table of Contents
- Understanding UnknownEntityException
- Common Causes
- Resolving the Exception
- Best Practices
- Conclusion
Understanding UnknownEntityException
UnknownEntityException
occurs when Hibernate does not recognize the specified entity during a transactional operation, such as when performing a persist
, merge
, or remove
. This exception usually arises from issues related to the entity classes or misconfigured mappings.
Example of Exception
Here is a typical stack trace where UnknownEntityException
might appear:
org.hibernate.UnknownEntityException: No entity found for query
This message suggests that Hibernate was unable to find the entity declared in the query.
Common Causes
Identifying the root cause is essential before we can resolve the exception. Here are some typical scenarios that could trigger UnknownEntityException
:
-
Entity Not Annotated Properly: The entity class might not be annotated with
@Entity
, which is crucial for Hibernate to recognize it as an entity.@Entity public class User { // fields, getters, and setters }
-
Incorrect Mapping Configuration: The entity’s mapping could be wrongly defined in the
persistence.xml
file or in annotated references. -
Missing Entity in Session: If the entity was not saved in the session’s context before attempts to interact with it (e.g., calling a method on it).
-
Transient Entities: When trying to merge or remove entities that are transient (not yet persisted in the database).
-
Unregistered Entity Classes: The entity class must be included in the configuration or via annotated packages in Hibernate.
Resolving the Exception
Recognizing potential issues is only the first step. Below, we will discuss specific resolutions alongside actual code examples.
Ensure Proper Entity Annotation
Always confirm that your entity class has the @Entity
annotation. This is the linchpin that informs Hibernate of the class's role.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue
private Long id;
private String username;
// Getters and Setters
}
Check for Correct Mappings
Make sure that your entity is correctly mapped in your persistence.xml
or hibernate.cfg.xml
file. If using annotations, ensure that your package structure is specified for scanning.
Example of persistence.xml
<persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence
http://xmlns.jcp.org/xml/ns/persistence/persistence_2_1.xsd"
version="2.1">
<persistence-unit name="exampleUnit">
<class>com.example.User</class>
<properties>
<property name="hibernate.hbm2ddl.auto" value="update"/>
<property name="hibernate.dialect" value="org.hibernate.dialect.H2Dialect"/>
</properties>
</persistence-unit>
</persistence>
Persist Before Transaction
Before working with an entity, always ensure that it is persisted in the database. If it’s not, you may encounter UnknownEntityException
.
EntityManager em = entityManagerFactory.createEntityManager();
em.getTransaction().begin();
User user = new User();
user.setUsername("john.doe");
em.persist(user); // Ensure it's persisted
em.getTransaction().commit();
em.close();
Using the Correct EntityState
When working with the merge
method, make sure the entity is not transient. For instance, if you try to merge an unpersisted entity, Hibernate will throw an UnknownEntityException
.
User detachedUser = new User(); // Transient
detachedUser.setId(1L); // If 1L is not in DB and you call merge
em.merge(detachedUser); // This will throw the exception
Validating Your Entity Configuration
If your entities are scattered across multiple packages, ensure to scan all relevant packages to register each entity:
<context:component-scan base-package="com.example.entity"/>
Alternatively, you can specify classes encapsulating core entities explicitly.
Best Practices
To prevent running into UnknownEntityException
in the future, consider following these best practices:
-
Consistent Entity Annotations: Always annotate your entity classes properly.
-
Transactional Management: Use proper transaction management to ensure entities exist in context when needed.
-
Use Unit Tests: Regularly execute unit tests for your entity interactions to catch mapping issues early.
-
Error Logging: Implement robust error logging around entity operations to surface issues quickly.
-
Refer to the Hibernate Documentation: Stay current with the Hibernate documentation for best practices and configuration setups.
My Closing Thoughts on the Matter
In summary, UnknownEntityException
can disrupt Hibernate operations and lead to confusion for developers. However, by understanding its causes and implementing the resolutions outlined above, you can minimize the likelihood of encountering this issue in your Java applications. Always keep your entities well-documented, well-configured, and consistently tested to ensure seamless interaction with the database using Hibernate.
By embracing these strategies, your journey with Hibernate will be a little smoother, allowing you to focus on building amazing applications instead of battling exceptions. Happy coding!