Common Pitfalls When Integrating Jersey with Spring
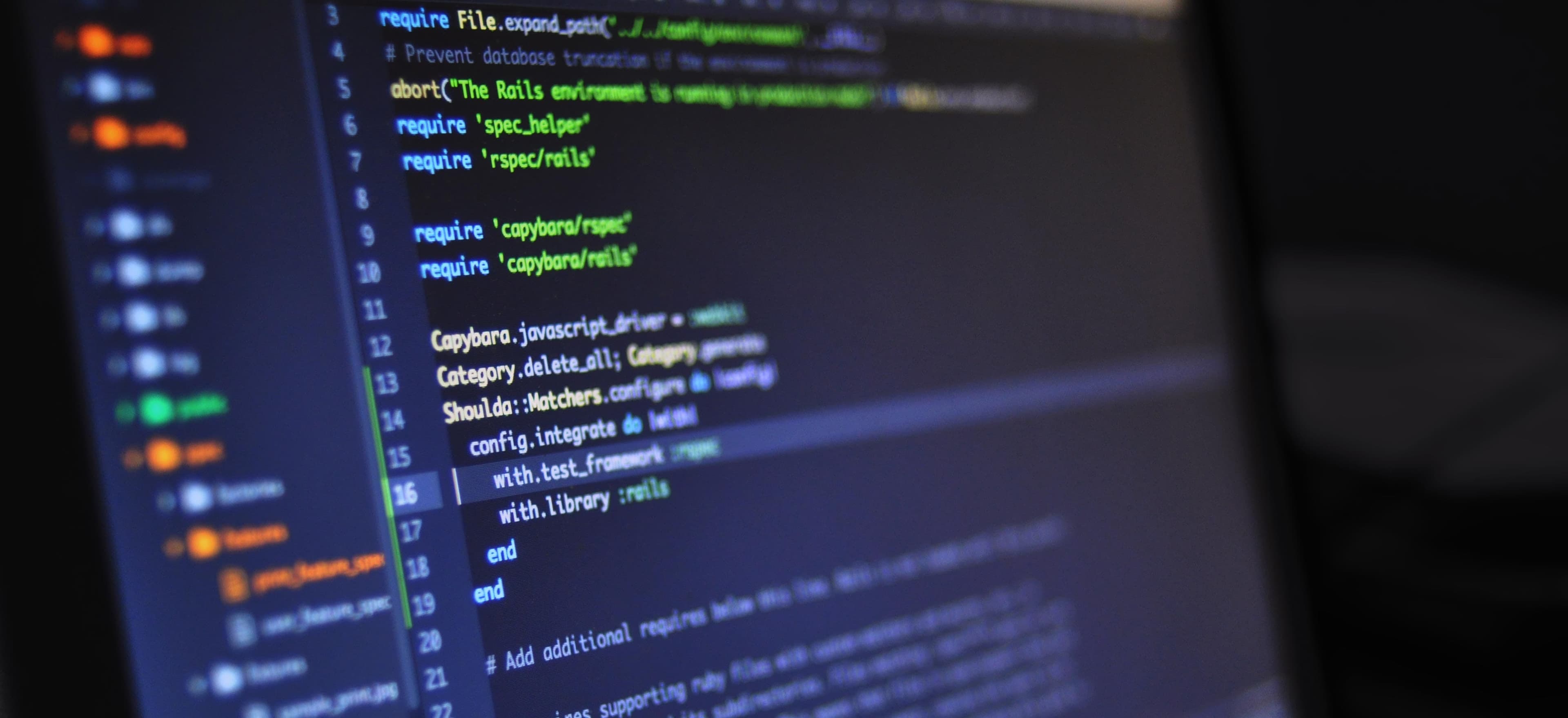
- Published on
Common Pitfalls When Integrating Jersey with Spring
When building RESTful services, Jersey and Spring often come up as the two leading frameworks. Jersey facilitates the development of RESTful web services with ease, while Spring offers robust dependency injection and management capabilities. Despite their complementary nature, integrating Jersey with Spring is not always seamless. This article will explore common pitfalls developers encounter during this integration, alongside strategies for avoiding these issues.
Understanding Jersey and Spring
Before diving into integration pitfalls, it's essential to understand why developers choose to combine these two frameworks.
Jersey
Jersey is an open-source framework that acts as a JAX-RS (Java API for RESTful Web Services) implementation. It simplifies the creation of RESTful services with its annotations and built-in functionalities. It's known for its flexible URI, content negotiation, and support for a variety of media types.
Spring
Spring is a comprehensive framework that facilitates building Java applications. It provides extensive capabilities including Dependency Injection (DI), aspect-oriented programming (AOP), and declarative transaction management, among others. Spring's IoC (Inversion of Control) container simplifies the management of application components.
Why Integrate Jersey with Spring?
Integrating Jersey with Spring allows developers to leverage Jersey's capabilities to create RESTful services while also benefiting from Spring's dependency management and configuration features. This combination enhances modularity, testability, and maintainability in application design.
Pitfall #1: Conflicting Component Scanning
One of the most common issues developers face when integrating Spring with Jersey is component scanning conflicts. Both frameworks rely on their mechanisms for scanning and managing beans (Spring’s @Component
vs. Jersey’s @Path
).
Solution
To resolve this conflict, you need to ensure that each framework’s scanning configuration does not interfere with the other. You can achieve this by defining specific base packages for component scanning in both configurations.
@Configuration
@EnableWebMvc
@ComponentScan(basePackages = "com.example.spring") // Spring components
public class AppConfig {
// Spring configuration
}
public class JerseyConfig extends ResourceConfig {
public JerseyConfig() {
packages("com.example.jersey"); // Jersey components
}
}
In this code snippet, we clearly delineate which packages each framework should scan, minimizing conflicts and ensuring both configurations work harmoniously.
Pitfall #2: Incorrect Servlet Configuration
Another common pitfall is the misconfiguration of the Jersey servlet in the web.xml file. If the servlet mapping is incorrect, requests may not be routed to the Jersey framework properly.
Solution
Ensure you have the correct configuration in your web.xml
:
<servlet>
<servlet-name>JerseyServlet</servlet-name>
<servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>javax.ws.rs.Application</param-name>
<param-value>com.example.MyApplication</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>JerseyServlet</servlet-name>
<url-pattern>/api/*</url-pattern>
</servlet-mapping>
By ensuring the url-pattern
and the application class are configured correctly, you will avoid potential errors in request mapping.
Pitfall #3: Bean Overriding Issues
When using Jersey in a Spring environment, users often encounter issues with bean overriding. If a bean is defined in both Jersey and Spring, conflicts will occur, leading to runtime errors.
Solution
To avoid bean conflicts, clearly define your beans and use qualifiers where necessary depending on your dependency management style.
@Component
@Singleton // Jersey's @Singleton, makes this bean a singleton
public class MyService {
// Service Logic
}
@Configuration
public class AppConfig {
@Bean
public MyService mySpringService() {
return new MyService(); // This ensures a Spring-managed bean
}
}
Using @Singleton
in combination with Spring’s @Bean
annotation prevents overlaps and guides the framework on which instance to use.
Pitfall #4: Exception Handling
Exception handling can become tricky when integrating Jersey with Spring. Jersey's default exception handling may not match your Spring-based configuration, leading to confusion when errors arise.
Solution
You can implement a custom exception mapper for Jersey to unify exception handling across the application. For example:
@Provider
public class MyExceptionMapper implements ExceptionMapper<MyCustomException> {
@Override
public Response toResponse(MyCustomException exception) {
return Response.status(Response.Status.BAD_REQUEST)
.entity(exception.getMessage())
.build();
}
}
This custom exception mapper allows you to centralize and standardize error responses, making it easier to handle exceptions in a way that fits both Jersey and Spring's paradigms.
Pitfall #5: Lack of Unit Testing
With the integration of multiple frameworks, developers sometimes neglect the need for unit testing across their services. If services are not tested properly, it becomes difficult to identify integration issues early.
Solution
Integrate JUnit or another testing framework to test your services thoroughly. Consider using Mockito to mock dependencies and streamline the testing process.
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = {AppConfig.class})
public class MyServiceTest {
@Autowired
private MyService myService;
@Test
public void testServiceMethod() {
// Assuming the method under test returns a value
String result = myService.performAction();
assertEquals("Expected Value", result);
}
}
By incorporating unit tests, you can swiftly identify and rectify issues in your integration setup, ensuring smoother application performance and stability.
My Closing Thoughts on the Matter
Integrating Jersey with Spring can yield powerful RESTful services, but it requires careful attention to detail. By understanding common pitfalls and implementing the solutions discussed, you can significantly enhance your integration process. Make sure to test thoroughly, handle exceptions properly, and maintain clear configurations for a successful development experience.
For further reading on Spring and Jersey, you can explore the Spring documentation and the Jersey documentation. Both resources offer comprehensive guides and examples to enrich your development endeavors.
Happy coding!
Checkout our other articles