Mastering Microservices: Top Interview Challenges Explained
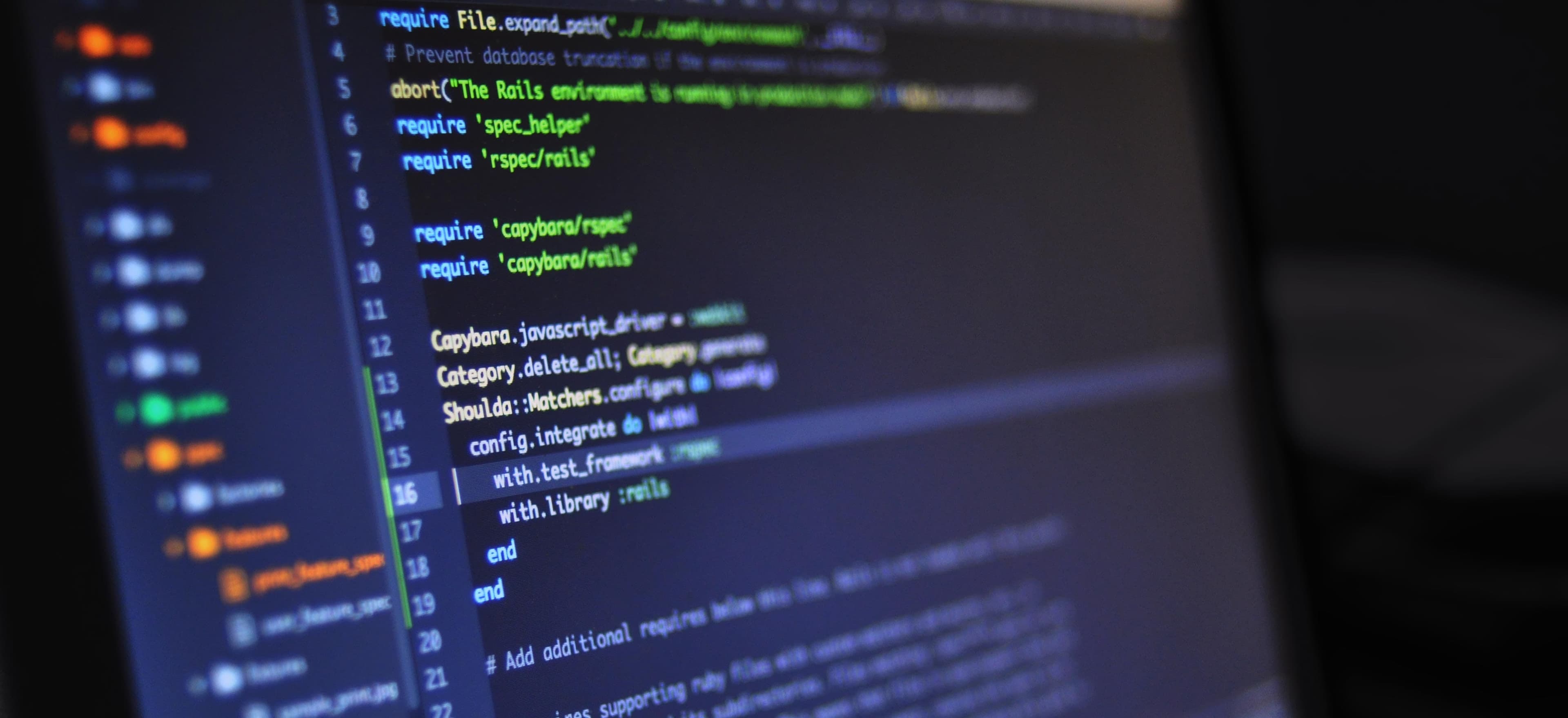
- Published on
Mastering Microservices: Top Interview Challenges Explained
Microservices architecture has revolutionized the way applications are developed and deployed. This shift offers scalability, flexibility, and faster deployments. However, transitioning from monolithic architectures to microservices comes with its own set of challenges. In this post, we will explore some of the most common challenges encountered in microservices interviews and provide insights into how to tackle them.
What Are Microservices?
Microservices are a software architectural style that structures an application as a collection of small, autonomous services. Each service is designed to handle specific business capabilities and can be developed, deployed, and scaled independently. This design allows development teams to be more agile and focuses on continuous delivery.
Understanding the Challenges
Facing interviews focusing on microservices can be daunting due to the plethora of challenges candidates can encounter. Below, we will explore some of the top challenges and how to effectively navigate them during interviews.
1. Service Decomposition
Challenge: Deciding how to decompose a monolithic application into microservices can be unclear and complex.
Solution: Discuss the concept of bounded context from Domain-Driven Design (DDD). Bounded contexts help determine service boundaries based on business capabilities.
// Example of a service that manages customer information
public class CustomerService {
private Repository<Customer> customerRepository;
public CustomerService(Repository<Customer> repository) {
this.customerRepository = repository;
}
public Customer getCustomerById(Long id) {
return customerRepository.findById(id);
}
public void createCustomer(Customer customer) {
customerRepository.save(customer);
}
}
Why: This code snippet signifies the independence of the CustomerService
. It can be modified or scaled without affecting other services.
2. Data Management
Challenge: In a microservice architecture, each service manages its data. This leads to complexities in data consistency and transactions.
Solution: Promote the use of eventual consistency and distributed transactions using patterns like Saga or CQRS (Command Query Responsibility Segregation).
// Example of an Event Publisher for a transaction
public class OrderService {
private final EventPublisher eventPublisher;
public OrderService(EventPublisher eventPublisher) {
this.eventPublisher = eventPublisher;
}
public void placeOrder(Order order) {
// Place the order logic here
eventPublisher.publish(new OrderPlacedEvent(order.getId()));
}
}
Why: The OrderService
publishes an event after successfully placing an order, illustrating an eventual consistency model. This allows other services to react and update their state accordingly, promoting loose coupling.
3. Inter-Service Communication
Challenge: Microservices often need to communicate with each other, and selecting an appropriate communication method is crucial.
Solution: Consider synchronous communication with REST APIs for real-time requirements, or use asynchronous messaging with message brokers like Kafka or RabbitMQ for scalability.
// Example of a REST client to communicate with another service
@RestController
public class InventoryClient {
private final RestTemplate restTemplate;
public InventoryClient(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
@GetMapping("/inventory/{productId}")
public ProductInventory checkInventory(@PathVariable String productId) {
return restTemplate.getForObject("http://inventory-service/inventory/" + productId, ProductInventory.class);
}
}
Why: By using a REST client, you can see how the InventoryClient
accesses the inventory service, highlighting the synchronous communication aspect.
4. Monitoring and Logging
Challenge: With multiple services running independently, maintaining observability can be hard.
Solution: Implement distributed tracing, centralized logging, and monitoring solutions such as ELK Stack (Elasticsearch, Logstash, Kibana) or Prometheus with Grafana.
Why: Having a centralized logging system allows for tracing requests across services, which is critical for identifying and debugging issues in a microservices environment.
5. Security Challenges
Challenge: Securing microservices exposes new vulnerabilities, especially in communication.
Solution: Use OAuth2 and JWT for authentication and authorization between services. Implement API gateways to route incoming requests and enforce security policies.
// JWT Token Generation Example
public String generateToken(User user) {
Map<String, Object> claims = new HashMap<>();
claims.put("username", user.getUsername());
return Jwts.builder()
.setClaims(claims)
.setIssuedAt(new Date(System.currentTimeMillis()))
.setExpiration(new Date(System.currentTimeMillis() + 1000 * 60 * 60 * 10)) // 10 hours
.signWith(SignatureAlgorithm.HS256, secretKey)
.compact();
}
Why: This JWT token generation script demonstrates how to create a secure token for user authentication, showcasing an important approach in securing microservices.
6. Deployment Strategies
Challenge: Implementing consistent and reliable deployment processes can be tricky in microservices.
Solution: Discuss various strategies such as Blue-Green deployments, Canary releases, or rolling updates to mitigate risks when deploying changes.
Additional Considerations
1. Versioning
When services evolve, managing versions is essential to avoid breaking changes. Communicate the importance of semantic versioning and backward compatibility.
2. Testing Strategies
Emphasize the necessity of automated unit tests, integration tests, and end-to-end tests tailored to microservices.
3. Service Discovery
In a microservices architecture, services must discover each other dynamically. Using tools like Consul or Spring Cloud Netflix Eureka can simplify this process.
4. Resilience and Fault Tolerance
Network failures can occur, hence resilience is critical. Discuss patterns such as Circuit Breaker with libraries like Hystrix or Resilience4j.
A Final Look
Mastering microservices requires a profound understanding of diverse challenges that can arise in a dynamic environment. By preparing for these challenges—ranging from service decomposition to security practices—you will be equipped to discuss your microservices expertise confidently during interviews.
As the industry shifts towards microservices, equipped with these insights, you can not only tackle interview challenges but also contribute significantly when implementing microservices in real-world applications.
For further reading on microservices architecture, check out Microservices Patterns and Building Microservices.
Now that you're familiar with the common microservices interview challenges, it's your turn to contribute your knowledge and experiences. Happy coding!