Mastering Java 8 Streams: Common Pitfalls to Avoid
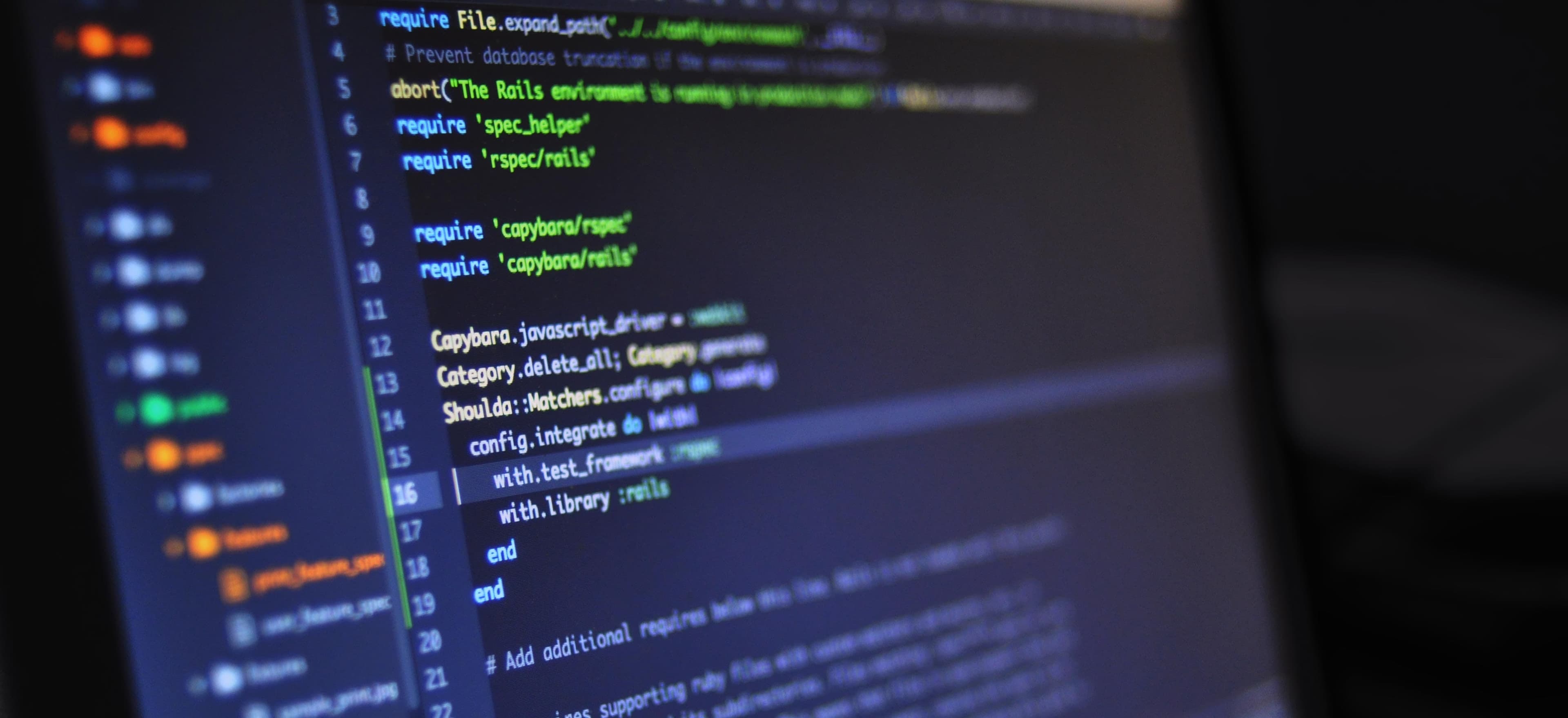
- Published on
Mastering Java 8 Streams: Common Pitfalls to Avoid
Java 8 introduced a powerful new abstraction for handling collections: Streams. It allows developers to express complex data processing queries in a declarative way, making code cleaner and easier to understand. However, with great power comes great responsibility—there are several pitfalls that developers can encounter when using Streams. In this blog post, we will explore these common pitfalls and provide practical examples to help you avoid them.
Understanding Java Streams
Before delving into the pitfalls, let’s take a moment to understand what Streams are. A Stream is a sequence of elements that supports various methods which can be processed in a functional style. This includes methods to filter, map, and reduce elements.
The key features of Streams include:
- Laziness: Operations on Streams are lazy and only executed when necessary.
- Chaining: You can chain together operations to form a pipeline.
- Parallelism: Streams can easily be run in parallel, taking advantage of multi-core architectures.
Example of a Simple Stream Operation
Here’s a simple example of using a Stream to process a list of integers:
import java.util.Arrays;
import java.util.List;
public class SimpleStreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
// Using Stream to filter out even numbers and square them
List<Integer> squaredEvens = numbers.stream()
.filter(n -> n % 2 == 0) // Filter even numbers
.map(n -> n * n) // Square even numbers
.toList(); // Collect results into a List
System.out.println(squaredEvens); // Output: [4, 16, 36]
}
}
In this example, we first filter out the even numbers from a list and then square each even number. The result is collected into a new list.
Common Pitfalls When Using Java Streams
1. Not Understanding the Difference between Intermediate and Terminal Operations
One of the most common mistakes is confusing intermediate and terminal operations. Intermediate operations like filter
, map
, and sorted
are streams that return another Stream and are lazy. Terminal operations like collect
, forEach
, and reduce
produce a result or a side-effect.
Why This Matters
If you forget to include a terminal operation, the Stream pipeline will not execute, leading to unexpected results or even silent failures.
Example:
import java.util.Arrays;
import java.util.List;
public class MissingTerminalOperation {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Intermediate operation only, no terminal operation.
Stream<Integer> stream = numbers.stream()
.filter(n -> n > 2);
// Attempting to access the stream here will not yield any results
System.out.println(stream.count()); // Will output 0 if accessed here
}
}
2. Not Handling Nulls Properly
Java Streams can throw NullPointerException
if the data being processed contains null elements. That’s why it’s essential to account for null values when working with Streams.
Why This Matters
Ignoring nulls can lead to runtime exceptions, breaking your application.
Example:
import java.util.Arrays;
import java.util.List;
public class NullHandlingInStream {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", null, "Bob", "Charlie");
// Filtering nulls before processing
List<String> nonNullNames = names.stream()
.filter(Objects::nonNull) // Filter to remove null values
.collect(Collectors.toList());
System.out.println(nonNullNames); // Output: [Alice, Bob, Charlie]
}
}
3. Overusing the forEach
Operation
It can be tempting to use forEach
to perform actions with Stream elements directly, but this often leads to less functional-style programming. More importantly, using forEach
can lead to unintended side effects.
Why This Matters
Avoiding forEach
as a terminal operation when data transformation is unnecessary preserves the functional programming principles that Streams advocate.
Example:
import java.util.Arrays;
import java.util.List;
public class OverusingForEach {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using forEach leads to side-effects and no return value
numbers.stream()
.forEach(n -> System.out.println(n * n));
// Better to collect and print outside the stream
List<Integer> squared = numbers.stream()
.map(n -> n * n)
.toList();
System.out.println(squared); // Output: [1, 4, 9, 16, 25]
}
}
4. Using filter
Inefficiently
Another pitfall is using filter
in a way that it can introduce performance issues. Using multiple filters can lead to inefficient pipelines, especially when the filters could have been combined.
Why This Matters
Combining filters where possible minimizes the number of passes over the data.
Example:
import java.util.Arrays;
import java.util.List;
public class InefficientFilters {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
// Suboptimal filtering, two passes over data
List<Integer> evenAndGreaterThanTwo = numbers.stream()
.filter(n -> n % 2 == 0)
.filter(n -> n > 2)
.toList();
// Better practice would be to combine conditions
List<Integer> combinedFilter = numbers.stream()
.filter(n -> n % 2 == 0 && n > 2) // One pass over data
.toList();
System.out.println(combinedFilter); // Output: [4, 6]
}
}
5. Ignoring Concurrency Issues with Parallel Streams
While Java makes it easy to run Streams in parallel with the parallelStream()
method, it’s important to understand that doing so can cause race conditions or unexpected behavior when mutable data is involved.
Why This Matters
Parallel streams should only be used when you're certain that the operations within the stream are stateless and side-effect free.
Example:
import java.util.Arrays;
import java.util.List;
public class ParallelStreamPitfall {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
StringBuilder buffer = new StringBuilder(); // Mutable state
// Risk of concurrent modification
names.parallelStream()
.forEach(name -> buffer.append(name.charAt(0))); // Unsafe
System.out.println(buffer.toString());
}
}
In this case, multiple threads may modify the buffer
simultaneously, leading to undefined behavior. To avoid this issue, consider using thread-safe data structures or ensure that no mutable states are shared across threads.
In Conclusion, Here is What Matters
Java 8 Streams provide a powerful way to process collections of data elegantly. However, as seen, there are several common pitfalls that developers encounter. By understanding the differences between intermediate and terminal operations, handling nulls properly, minimizing side effects, combining filters, and being cautious with parallelism, you can master Streams effectively.
Further Reading
If you're looking to dive deeper into Java Streams and best practices, consider the following resources:
- Official Java Documentation on Streams
- Functional Programming in Java
- Java 8 Streams in Action
By adhering to these foundational principles, you can write clean, efficient, and robust Java code using Streams. Get started on your journey today!
Checkout our other articles