Streamlining Solutions: Tackling Design Problems with Factory Method
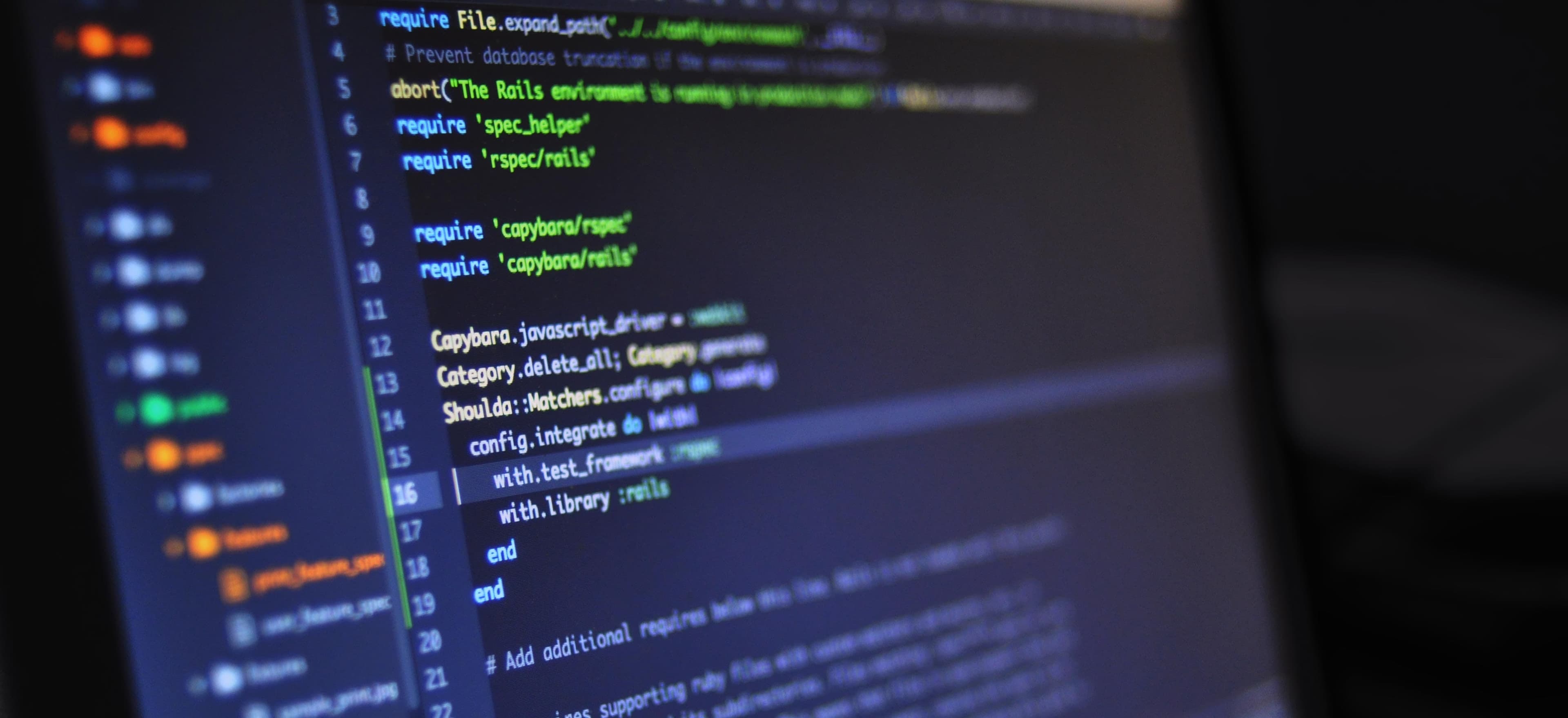
- Published on
Streamlining Solutions: Tackling Design Problems with Factory Method
In the current landscape of software development, design patterns play a crucial role in promoting code reusability and scalability. Among these patterns, the Factory Method stands out as a robust solution for addressing object creation problems. This article delves into the Factory Method, its advantages, implementation, and practical applications. Furthermore, we’ll compare it to alternative patterns to help you understand when to use it.
What is a Factory Method?
The Factory Method is a creational design pattern that defines an interface for creating an object but allows subclasses to alter the type of objects that will be created. This pattern promotes loose coupling by eliminating the need for client code to know the class of the object it creates.
Key Components
- Product: Defines the interface of objects created by the factory method.
- ConcreteProduct: Implements the Product interface.
- Creator: Declares the factory method.
- ConcreteCreator: Implements the factory method to return an instance of a ConcreteProduct.
Why Use the Factory Method?
- Separation of Concerns: It separates the instantiation logic from the business logic of your application.
- Code Scalability: New product types can be introduced without modifying existing code.
- Dependency Injection: It allows for easier injection of dependencies into classes.
Implementation of Factory Method in Java
Let’s illustrate the Factory Method with a simple example in Java.
Example: Animal Factory
We want to create a series of animal objects. Here’s how the Factory Method pattern can help:
// Product
interface Animal {
void speak();
}
// ConcreteProduct
class Dog implements Animal {
public void speak() {
System.out.println("Woof! Woof!");
}
}
class Cat implements Animal {
public void speak() {
System.out.println("Meow!");
}
}
// Creator
abstract class AnimalFactory {
public abstract Animal createAnimal();
}
// ConcreteCreator
class DogFactory extends AnimalFactory {
public Animal createAnimal() {
return new Dog();
}
}
class CatFactory extends AnimalFactory {
public Animal createAnimal() {
return new Cat();
}
}
// Client code
public class Client {
public static void main(String[] args) {
AnimalFactory dogFactory = new DogFactory();
Animal dog = dogFactory.createAnimal();
dog.speak(); // Outputs: Woof! Woof!
AnimalFactory catFactory = new CatFactory();
Animal cat = catFactory.createAnimal();
cat.speak(); // Outputs: Meow!
}
}
Breakdown of the Code
-
Product Interface: The
Animal
interface defines the functionality that all concrete products must implement. -
Concrete Products: The classes
Dog
andCat
provide specific implementations of theAnimal
interface. -
Creator: The abstract class
AnimalFactory
declares the factory methodcreateAnimal()
, which will be implemented by its subclasses. -
Concrete Creators: The
DogFactory
andCatFactory
implement thecreateAnimal()
method, returning instances ofDog
andCat
, respectively. -
Client Code: The client creates a specific factory and uses it to generate the corresponding animal objects without worrying about their concrete classes.
Advantages of Using Factory Method
- Extensibility: Adding new animals (e.g.,
Horse
,Bird
) requires creating new factory classes without altering existing code. - Decoupling: The client code interacts with the
Animal
interface, ensuring changes to concrete products have minimal impact on the client.
Relevant Links
When Not to Use the Factory Method
While the Factory Method is beneficial, certain situations warrant considering alternative patterns:
-
Simple Applications: If the application does not have varying types of objects that need to be created, the added complexity may not be justified.
-
Overhead of Additional Classes: If introducing many factories leads to cumbersome code organization, simpler solutions might be preferred.
-
Frequent Changes: If you anticipate frequent changes in the product family, the Abstract Factory Pattern may be more appropriate.
Comparison with Other Creational Patterns
Singleton Pattern
The Singleton Pattern restricts a class to a single instance and provides a global point of access. In contrast, the Factory Method allows multiple related objects to be instantiated. Thus, the implementation will depend on the specific requirement of your application.
Abstract Factory
The Abstract Factory provides an interface for creating families of related or dependent objects. If your system requires the creation of multiple products that are designed to work together, the Abstract Factory is a viable alternative. However, if your focus is primarily on a single product type with potential variations, the Factory Method may be more suitable.
A Final Look
The Factory Method Design Pattern provides a flexible solution to manage object creation, allowing for easy extension, maintainability, and adherence to the Open/Closed Principle. Understanding when and where to deploy this pattern can greatly enhance your system's architecture, especially in larger, more complex applications.
Incorporating the Factory Method into your design toolkit can lead to cleaner, more manageable code. Knowing that you can add new products without disrupting existing code opens up a realm of possibilities for future enhancements. Adopt this pattern to streamline your object creation processes, ultimately enhancing your software's robustness and resilience.
For further reading on design patterns, check out Head First Design Patterns by Eric Freeman, which offers great insights into various design approaches. Happy coding!