Mastering Optimistic Locking: Avoiding Data Conflicts
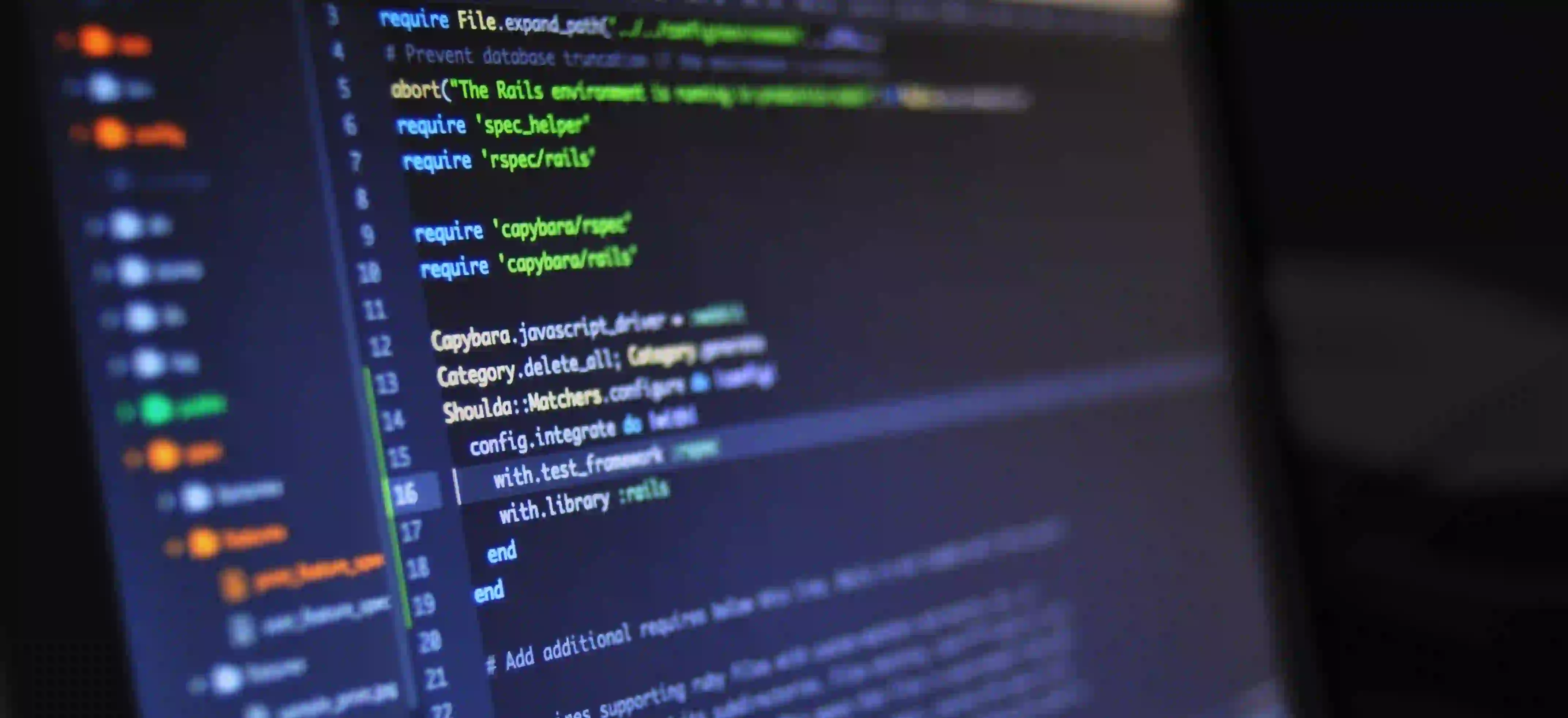
Mastering Optimistic Locking: Avoiding Data Conflicts in Java Applications
In the world of software development, especially in Java applications, data integrity and concurrency control are paramount. One effective way to handle this is through optimistic locking. Unlike pessimistic locking that prevents conflicts by locking data, optimistic locking assumes that multiple transactions can complete without interfering with each other. When data is updated, optimistic locking checks if a conflict has occurred, providing a robust way to handle concurrency.
This blog post delves into optimistic locking, its mechanisms, when to use it, and how to implement it in Java applications. We'll explore code examples that illustrate the 'why' behind each piece of code, ensuring that you thoroughly understand this essential concept.
Understanding Optimistic Locking
Before diving into implementation details, it's vital to understand the essence of optimistic locking.
- Optimistic Locking: This approach is based on the principle that most transactions do not conflict. Instead of locking data immediately, it checks at the time of the update whether another transaction has modified the data.
- Conflict Detection: If a conflict is detected, the transaction would be rolled back, allowing the user to retry the operation.
When to Use Optimistic Locking
Optimistic locking is ideal for scenarios where:
- High read activity: Read operations greatly outnumber write operations.
- Minimal contention: The likelihood of transactions conflicting is low.
- Performance: You want to minimize wait times typically associated with pessimistic locking.
Conversely, pessimistic locking would be a better fit when:
- High write activity: You expect many transactions to attempt to modify the same data simultaneously.
- Critical data integrity: You can't afford any conflicts.
Setting Up Optimistic Locking in Java
To demonstrate optimistic locking, we will use Java Persistence API (JPA) along with Hibernate. This combination is popular for managing database interactions in Java applications.
Step 1: Maven Dependencies
In your pom.xml
, include the necessary dependencies:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.30.Final</version>
</dependency>
<dependency>
<groupId>javax.persistence</groupId>
<artifactId>javax.persistence-api</artifactId>
<version>2.2</version>
</dependency>
Step 2: Define Your Entity
First, we need to define an entity class representing our database table. We will add a version field that Hibernate will use for optimistic locking.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Version;
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Double price;
@Version
private Long version; // Version field for optimistic locking
// Getters and Setters
}
Why Use a Version Field?
The @Version
annotation tells Hibernate to use this field to track changes. Each time the entity is updated, the version is incremented. This ensures that when two transactions try to update the same record, Hibernate can detect any conflicts based on version mismatches.
Step 3: Implementing the Logic
Next, let’s create a service that manages updates to our Product
entity. Here, we will demonstrate how optimistic locking works.
import javax.persistence.EntityManager;
import javax.persistence.EntityTransaction;
public class ProductService {
private EntityManager entityManager;
public ProductService(EntityManager entityManager) {
this.entityManager = entityManager;
}
public void updateProduct(Long productId, String newName, Double newPrice) {
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
try {
Product product = entityManager.find(Product.class, productId);
product.setName(newName);
product.setPrice(newPrice);
entityManager.merge(product); // This call saves changes
transaction.commit(); // Commit the transaction
} catch (OptimisticLockException e) {
transaction.rollback();
System.out.println("Update failed due to a concurrent modification. Please try again.");
} catch (Exception e) {
transaction.rollback();
e.printStackTrace();
}
}
}
Why Handle OptimisticLockException?
Here, we use a try-catch block to manage OptimisticLockException
. If another transaction has updated the record after it was loaded but before it was saved, Hibernate throws this exception. Rolling back the transaction avoids any incomplete data being saved, ensuring data integrity.
Step 4: Testing Your Implementation
To test your optimistic locking implementation, run two separate threads or instances of the application attempting to update the same product at the same time. You’ll see that the second transaction will fail gracefully with an exception.
Example Test Code
Here’s a simple test harness:
public class OptimisticLockingTest {
public static void main(String[] args) {
EntityManagerFactory emf = Persistence.createEntityManagerFactory("YourPersistenceUnit");
EntityManager em = emf.createEntityManager();
ProductService productService = new ProductService(em);
// First Thread - Simulating User A
new Thread(() -> {
productService.updateProduct(1L, "New Product Name A", 99.99);
}).start();
// Second Thread - Simulating User B
new Thread(() -> {
productService.updateProduct(1L, "New Product Name B", 89.99);
}).start();
em.close();
emf.close();
}
}
Why Use Threads for Testing?
This concurrent test simulates real-world scenarios where multiple users might try to perform updates on the same record. You’ll notice that one update at a time will succeed, while the other must handle the conflict appropriately.
To Wrap Things Up
Optimistic locking is an effective strategy for managing concurrency in Java applications, allowing for high throughput in read-heavy environments while maintaining data integrity. By using the @Version
annotation in JPA and handling OptimisticLockException
, you can implement this pattern seamlessly within your applications.
To expand your knowledge further on this topic, you can check out the official Hibernate documentation which provides deeper insights into its features and other locking strategies.
By mastering optimistic locking, you can build scalable, reliable Java applications capable of gracefully handling concurrent data operations. Happy coding!