Unlocking the Mystery: Java 14's New Features Explained
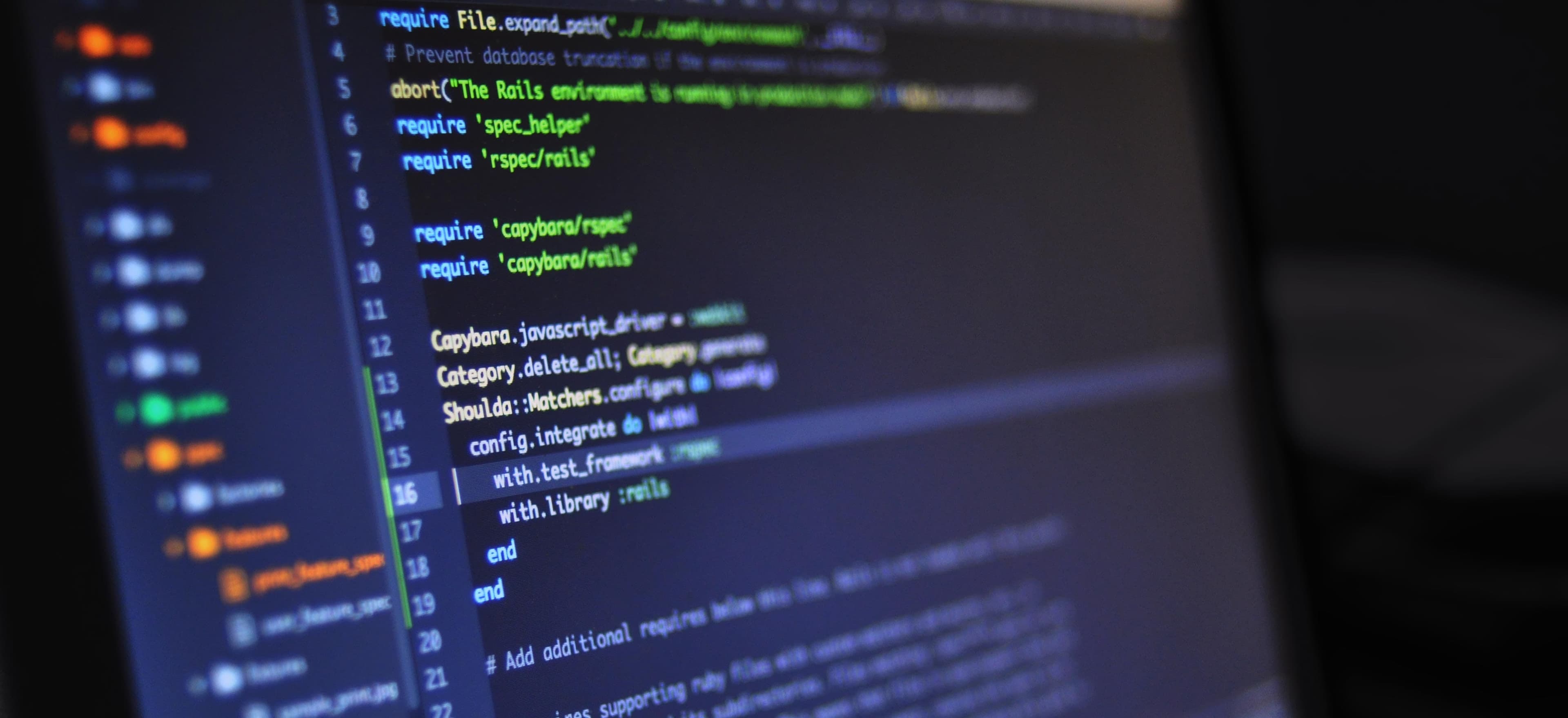
- Published on
Unlocking the Mystery: Java 14's New Features Explained
Java, one of the oldest yet most versatile programming languages, continues to evolve. With every new version, it introduces exciting features that enhance both performance and developer experience. Released in March 2020, Java 14 came packed with innovations that improved the language's usability while adding valuable tools for developers. In this blog post, we'll delve into the new features of Java 14, understanding their purpose and how they can be leveraged in your projects.
Table of Contents
Diving Into the Subject to Java 14
Java 14 is part of the six-month release cycle introduced by the Java community, allowing for more frequent updates and improvements. This version emphasizes both developer productivity and code quality. With several new features and enhancements to existing APIs, Java 14 aims to streamline the development process while boosting application performance.
Key Features of Java 14
1. Pattern Matching for instanceof
One of the most discussed features in Java 14 is the introduction of pattern matching for the instanceof
operator. Prior to this feature, developers often needed to cast objects after checking their type, leading to redundant code.
Code Example:
public class PatternMatchingExample {
public static String getLength(Object obj) {
// Traditional instanceof check
if (obj instanceof String) {
String str = (String) obj; // Explicit cast
return String.format("String length: %d", str.length());
}
return "Not a String";
}
}
With Java 14, you can simplify this to:
public class PatternMatchingExample {
public static String getLength(Object obj) {
// Pattern matching for instanceof
if (obj instanceof String str) {
return String.format("String length: %d", str.length());
}
return "Not a String";
}
}
Why? The pattern matching feature reduces boilerplate code, making it clearer and easier to read. It also minimizes the risk of ClassCastException
since the type-checking and casting happen in one streamlined step.
2. NPE Prevention in JDK
APIs
Another significant feature aimed at reducing potential pitfalls is the prevention of NullPointerExceptions (NPEs) from certain JDK APIs. Specifically, methods in the API that throw NPEs will now provide clearer information when they are invoked with null parameters.
Why? This feature promotes better code safety and debugging. Developers can now catch these potential issues earlier in the development process, leading to cleaner and more reliable code.
3. Improved Switch Expressions
Java 14 enhances the previously introduced switch expressions, making them more powerful and versatile. Now, it's possible to use a yield
statement to return values from the switch expression directly.
Code Example:
public class SwitchExample {
public static String getDayType(int day) {
switch (day) {
case 1, 2, 3, 4, 5 -> {
yield "Weekday";
}
case 6, 7 -> {
yield "Weekend";
}
default -> {
yield "Invalid day";
}
}
}
}
Why? This enhancement allows for more readable and maintainable code. The return value of a switch statement can now be handled directly without needing additional variables or complex flow control.
4. Text Blocks
Text Blocks provide a way to express multi-line string literals without the need for escape sequences. This feature reduces boilerplate and improves readability, especially when it comes to JSON, SQL, or XML.
Code Example:
public class TextBlockExample {
public static String getJsonData() {
return """
{
"name": "John Doe",
"age": 30,
"isEmployed": true
}
""";
}
}
Why? Text Blocks eliminate the need for concatenation and escape characters, enhancing code clarity and making it easier to visualize the intended output. This is especially important in web development, where developers are frequently manipulating large blocks of text.
5. New JEP Features
Java 14 includes several new Java Enhancement Proposals (JEPs) that enhance the Java language overall. Some of the most notable ones are:
- JEP 305: Pattern Matching for
instanceof
: As discussed earlier, this allows seamless type checks and casting. - JEP 343: Packaging Tool: A new tool for packaging Java applications into native executables.
- JEP 344: Abortable Mixed Collections: Controls for optimizing garbage collection, improving performance.
Why? Each of these JEPs contributes to making Java 14 more robust and efficient, continuing the tradition of Java being a relevant language in a rapidly evolving tech world.
Key Takeaways
In conclusion, Java 14 has brought significant advancements and enhancements to the language, with features designed to improve developer productivity and code quality. Emphasizing readability, maintainability, and performance, Java 14 sets a new standard for future iterations of the language. Exploring these features can lead to more efficient coding practices and better software solutions.
As you continue your journey in Java development, feel free to refer to the official Oracle documentation for in-depth information and updates. Your commitment to understanding and mastering these new features will undoubtedly enhance your programming skill set.
Embrace Java 14 and unlock the potential it brings to your projects!
This post aims to be an engaging resource for understanding Java 14's features. From pattern matching to text blocks, these advancements deliver excellence while refining the development process. Dive into the new Java world, and watch your coding efficiency skyrocket!
Checkout our other articles