Top Mistakes Developers Make When Saving Data on Android
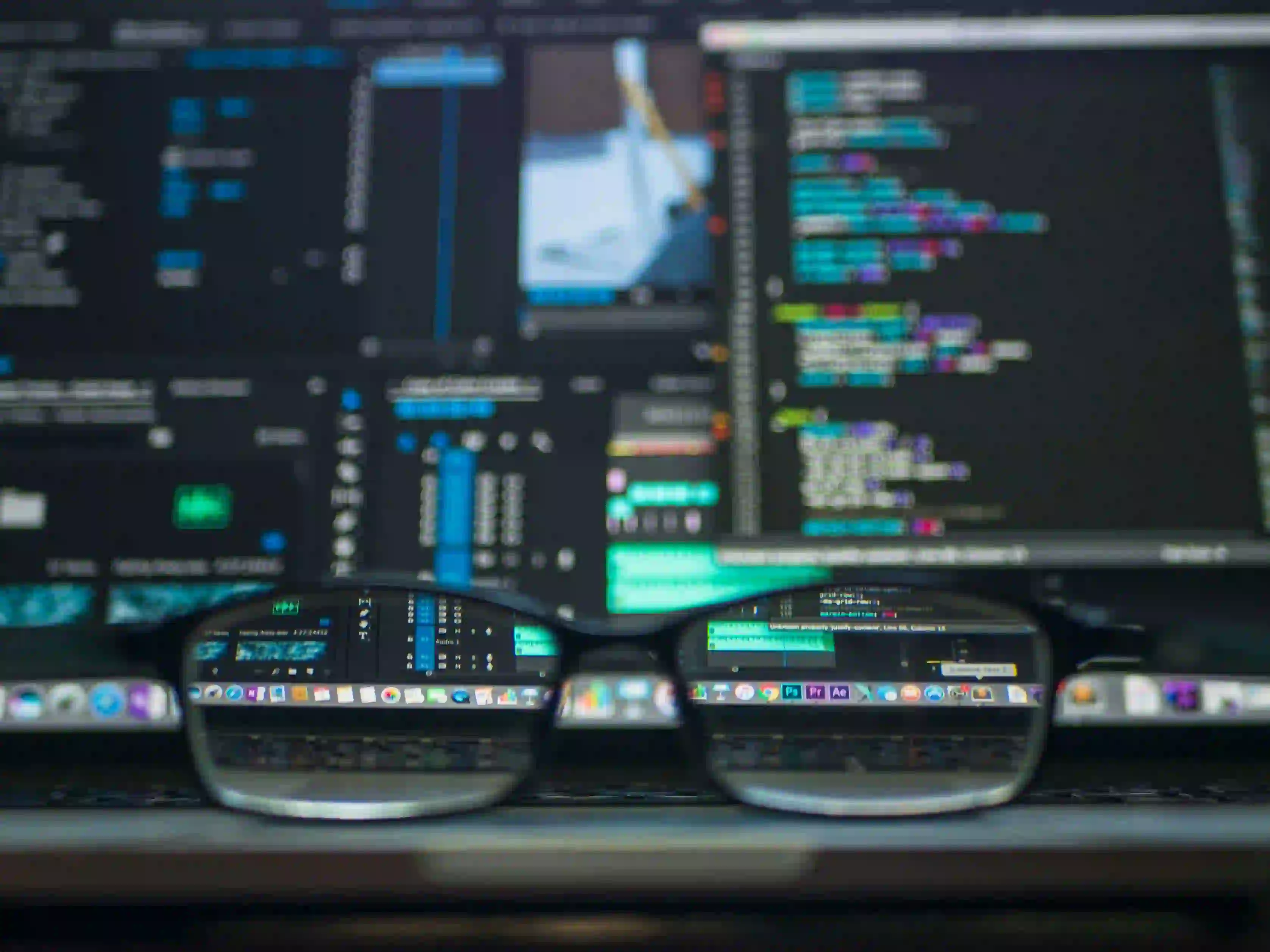
Top Mistakes Developers Make When Saving Data on Android
Android development offers a multitude of options for persisting data. However, with great power comes great responsibility! Many developers, particularly those who are new to the platform, often stumble upon pitfalls that can lead to inefficient applications or even data loss.
In this blog post, we will discuss the top mistakes developers make when saving data on Android, and provide you with best practices to ensure your applications are robust and data-efficient.
1. Not Choosing the Right Data Storage Option
One of the primary mistakes developers make is not selecting the appropriate data storage mechanism. Android provides several options, including:
- SharedPreferences: For simple key-value pairs.
- SQLite Database: For structured and relational data.
- Room Persistence Library: An abstraction layer over SQLite that provides an easier way to handle databases.
- File Storage: For saving files in internal or external storage.
Why It Matters
Choosing the right storage option can significantly impact the performance and scalability of your app. For instance, using SharedPreferences for large datasets can lead to performance issues. Conversely, opting for SQLite for small configurations could be overkill.
Example
// Using SharedPreferences to save user settings
SharedPreferences sharedPreferences = getSharedPreferences("AppSettings", Context.MODE_PRIVATE);
SharedPreferences.Editor editor = sharedPreferences.edit();
editor.putBoolean("NotificationsEnabled", true);
editor.apply(); // Use apply() for non-blocking, async save.
In this example, SharedPreferences is ideal for quick access settings like notification preferences. For complex models, consider using Room instead.
2. Ignoring Data Security
Many developers overlook security aspects when saving sensitive data. Whether it’s user credentials, personal data, or API keys, not encrypting this data can lead to vulnerabilities.
Why It Matters
Data breaches can have legal, financial, and reputational consequences. This makes it crucial to securely handle and store sensitive information.
Example
// Example of encrypting data before saving in SharedPreferences
String originalData = "API_KEY";
String encryptedData = encryptData(originalData);
editor.putString("ApiKey", encryptedData);
editor.apply();
Using libraries like Android Keystore for secure data storage is highly recommended.
3. Failing to Handle Configuration Changes
Configuration changes, such as screen rotations, can lead to data loss if not handled correctly. Developers often neglect to save and restore the state, resulting in a poor user experience.
Why It Matters
Users expect their navigational state to be maintained through configuration changes. Losing data in such cases diminishes the perceived quality of your app.
Example
@Override
public void onSaveInstanceState(Bundle outState) {
super.onSaveInstanceState(outState);
outState.putString("username", username);
}
@Override
public void onRestoreInstanceState(Bundle savedInstanceState) {
super.onRestoreInstanceState(savedInstanceState);
username = savedInstanceState.getString("username");
}
By overriding onSaveInstanceState
, you can ensure that important data is preserved during configuration changes.
4. Not Using Asynchronous Operations
Saving data on the main thread is a common mistake among developers, especially those unfamiliar with the Android lifecycle. This can result in blocked user interfaces and a suboptimal user experience.
Why It Matters
Long blocking operations can cause ANRs (Application Not Responding) errors, ultimately leading to app crashes and frustrations for users.
Example
// Utilize AsyncTask (deprecating, but for demonstration)
new AsyncTask<Void, Void, Void>() {
@Override
protected Void doInBackground(Void... voids) {
// Perform data saving in background
saveDataInBackground();
return null;
}
}.execute();
Alternatively, consider using Kotlin Coroutines or the WorkManager for better handling of background tasks.
5. Inadequate Error Handling
Many developers neglect error handling when interacting with databases or file systems. Ignoring potential exceptions can cause the app to crash or behave unexpectedly.
Why It Matters
User trust is critical. A robust app should handle errors gracefully, ensuring users have a consistent experience.
Example
try {
// Example database operation
databaseHelper.insertData(user);
} catch (SQLiteException e) {
Log.e("SaveData", "Database error", e);
// Notify user of the error
Toast.makeText(context, "Failed to save data.", Toast.LENGTH_SHORT).show();
}
This approach captures exceptions and informs the user without crashing the app.
6. Not Testing Data Persistence
Developers often test app functionality but may overlook testing data persistence. This can led to undetected bugs that surface only after deployment.
Why It Matters
Thorough testing ensures your app behaves correctly in various scenarios, reducing the likelihood of negative user experiences.
Best Practices
- Write unit tests for your data storage logic.
- Use UI tests to ensure that data is retained across configuration changes.
- Consider edge cases, such as low storage conditions.
7. Neglecting Data Cleanup
Failing to clean up old or unused data can cause bloat in your app, leading to performance issues and inefficient storage use.
Why It Matters
Regular maintenance of app data not only keeps the application performing smoothly but also saves precious storage on the user's device.
Example
// Example of deleting expired data
public void cleanupExpiredData() {
SQLiteDatabase db = this.getWritableDatabase();
String sql = "DELETE FROM UserData WHERE timestamp < ?";
db.execSQL(sql, new String[]{String.valueOf(System.currentTimeMillis() - EXPIRATION_TIME)});
}
Implement periodic cleanup routines, especially for apps that accumulate data over time.
Final Thoughts
Saving data in an Android application is a fundamental task, yet it comes with its challenges. Developers must consciously avoid common pitfalls to ensure robust, efficient, and secure applications.
By understanding and applying best practices such as selecting the right data storage option, implementing proper error handling, and utilizing asynchronous operations, you can enhance the quality of your Android applications.
For further information, the official Android Dev Documentation provides additional insights and guidance on best practices in data storage.
Remember, the goal is not just to save data but to do it effectively and responsibly. Happy coding!