Solving Race Conditions: Optimistic vs Pessimistic Locking
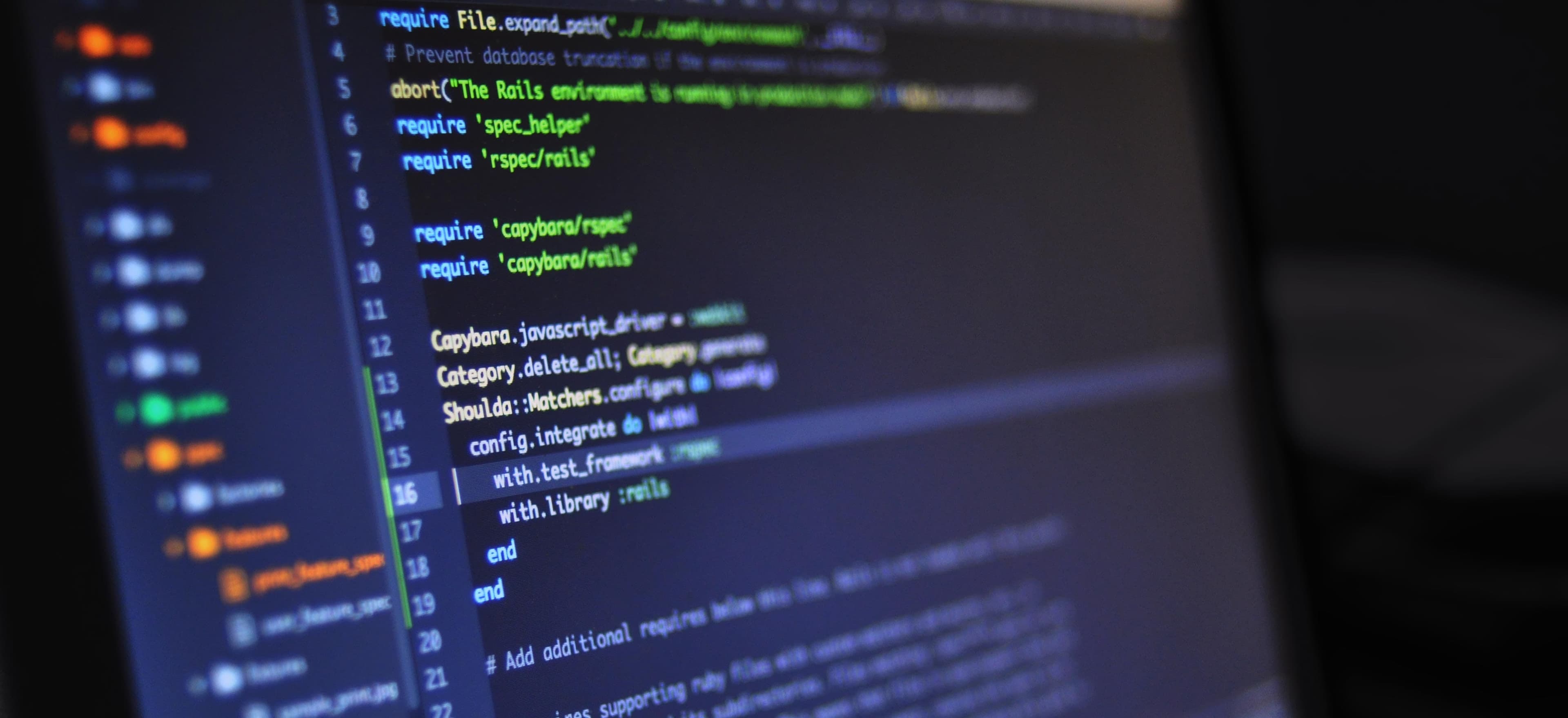
- Published on
Solving Race Conditions: Optimistic vs Pessimistic Locking in Java
Race conditions can be a nightmare for developers, leading to unpredictable behavior in applications. In a multi-threaded environment, where multiple threads try to access shared resources, the integrity of the data can quickly deteriorate without proper synchronization mechanisms. In this blog post, we will delve into two popular strategies for handling race conditions in Java applications: optimistic locking and pessimistic locking.
What Are Race Conditions?
A race condition occurs when the behavior of software depends on the relative timing of events, such as the order in which threads execute. This can lead to poor data integrity and unpredictable results if not managed correctly. For example, if two threads simultaneously attempt to update a user's account balance, it can result in one thread overwriting the other's changes, leading to incorrect balance values.
To avoid these problems, developers can choose between optimistic and pessimistic locking approaches, each with its pros and cons.
Pessimistic Locking
Pessimistic locking is a strategy that assumes conflicts will occur. It effectively locks resources, preventing other threads from modifying data until the lock is released. This locking mechanism is straightforward and easy to understand.
Key Features of Pessimistic Locking
- Immediate Locking: As soon as a thread needs to access a resource, it locks that resource.
- Blocking: Other threads attempting to access the locked resource are suspended until the lock is released.
- Prevention: This approach prevents concurrent updates, ensuring data integrity.
Example of Pessimistic Locking in Java
Let's consider a simple banking application where two threads try to update an account's balance.
public class BankAccount {
private double balance;
public BankAccount(double initialBalance) {
this.balance = initialBalance;
}
public synchronized void deposit(double amount) {
balance += amount;
}
public synchronized void withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
} else {
throw new IllegalArgumentException("Insufficient funds");
}
}
public synchronized double getBalance() {
return balance;
}
}
Explanation
- Synchronized Methods: The methods
deposit
,withdraw
, andgetBalance
are synchronized. This means that only one thread can execute any of these methods on a givenBankAccount
instance at a time. - Thread Safety: This approach ensures that no two threads can update the
balance
simultaneously, which inherently maintains data integrity.
Cons of Pessimistic Locking
- Performance: It can lead to bottlenecks, particularly in systems with high contention.
- Thread Blocking: Threads can be blocked, leading to inefficient resource utilization.
Optimistic Locking
In contrast, optimistic locking operates on the assumption that conflicts will be rare. Rather than locking the resource immediately, it allows multiple threads to access the resource simultaneously. However, before committing changes, the algorithm checks if another thread modified the resource.
Key Features of Optimistic Locking
- No Immediate Locking: Resources are accessed without locks.
- Validation Before Commit: Changes are validated before being written back to the resource.
- Conflict Resolution: In case of changes by other threads, the transaction can be retried or aborted.
Example of Optimistic Locking in Java
Here’s an implementation using versioning to demonstrate optimistic locking:
public class Account {
private double balance;
private int version;
public Account(double initialBalance) {
this.balance = initialBalance;
this.version = 0;
}
public double getBalance() {
return balance;
}
public synchronized boolean updateBalance(double newBalance, int expectedVersion) {
if (expectedVersion == this.version) {
this.balance = newBalance;
this.version++;
return true; // Update successful
}
return false; // Update failed due to version mismatch
}
}
Explanation
- Versioning: Each
Account
object keeps track of its version. When trying to update the balance, the thread must provide the current version. - Atomic Update: The method
updateBalance
checks if the expected version matches the actual version before making the update, ensuring thread safety without permanent locking.
Cons of Optimistic Locking
- Retry Logic: If a conflict occurs, the failed transaction must be retried, which can add complexity.
- Complex Error Handling: You'll have to manage failures gracefully and decide how to handle retries.
When to Use Each Strategy
Choosing between optimistic and pessimistic locking largely depends on your application's needs:
-
Pessimistic Locking is better suited for situations where:
- Conflicts are expected to occur frequently.
- The cost of handling conflicts is high.
- You need guaranteed data integrity immediately.
-
Optimistic Locking works best when:
- Conflicts are rare.
- You want to maximize throughput and minimize resource contention.
- Transaction latencies are low.
To Wrap Things Up
Both optimistic and pessimistic locking have their place in Java application development. Understanding the specifics of each approach allows you to make an informed decision based on the unique requirements and constraints of your application. When facing race conditions, the key is to evaluate your use case critically and choose the locking strategy that provides the needed balance between performance, complexity, and data integrity.
For further reading, you might find the following articles useful:
By mastering race conditions and locking strategies, you can build robust and efficient multi-threaded applications in Java. Happy coding!
Checkout our other articles