Overcoming Common Fork/Join Challenges in Java 7
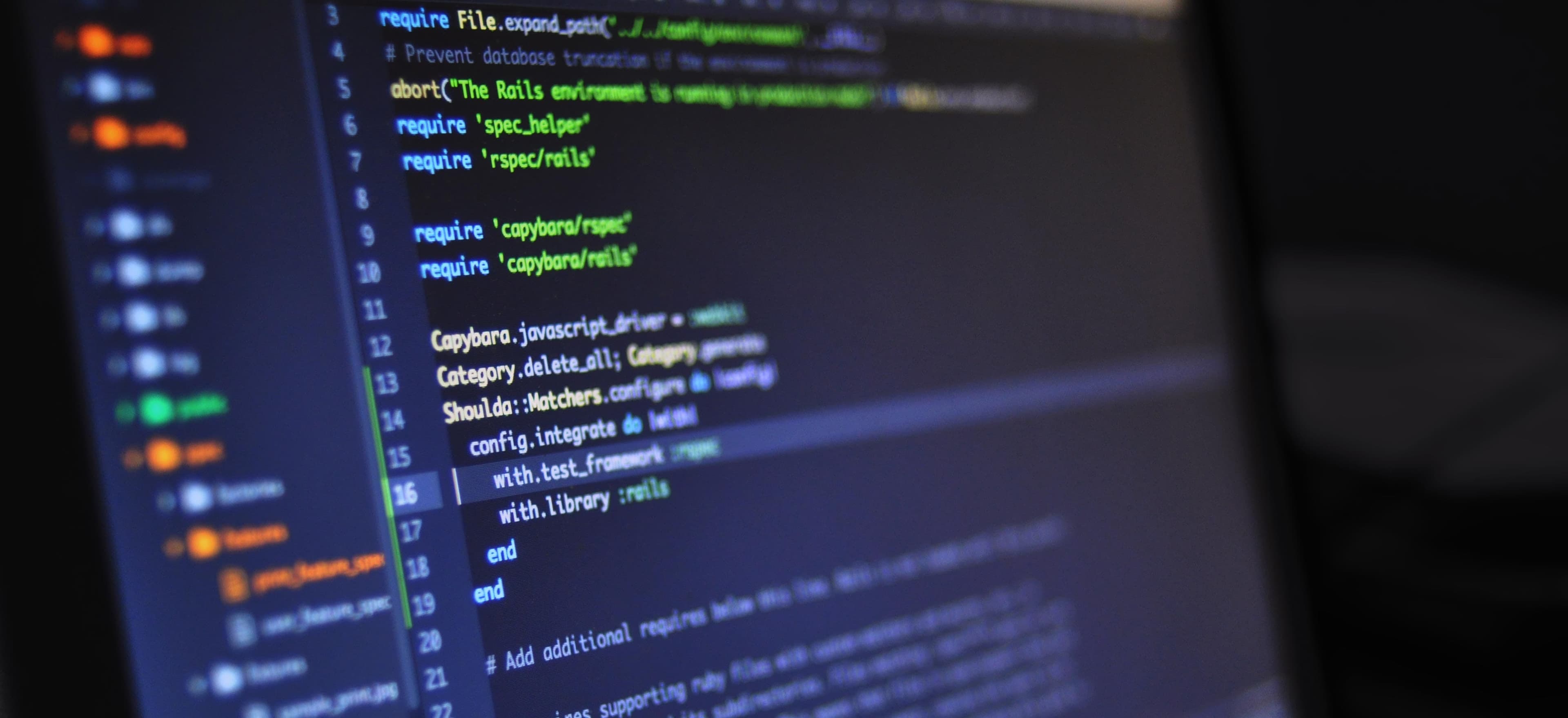
- Published on
Overcoming Common Fork/Join Challenges in Java 7
Java 7 introduced the Fork/Join framework, a powerful library designed for parallel programming, particularly when dealing with tasks that can be broken down into smaller subtasks. It enables developers to write applications that take advantage of multi-core processors. However, working with this framework comes with its own set of challenges.
In this blog post, we will dive into the common challenges you might face when using the Fork/Join framework in Java 7 and provide strategies to overcome them. So, let’s get started.
Understanding the Fork/Join Framework
Before we tackle the challenges, it's crucial to understand how the Fork/Join framework works. The framework is primarily based on two concepts: forking (splitting tasks into smaller tasks) and joining (combining the results of those tasks).
The core components are:
- ForkJoinPool: This pool manages a set of worker threads that execute the tasks.
- RecursiveTask: A task that returns a result.
- RecursiveAction: A task that does not return a result.
Here’s a basic example using a RecursiveTask
to compute the sum of an array of integers:
import java.util.concurrent.RecursiveTask;
import java.util.concurrent.ForkJoinPool;
public class SumTask extends RecursiveTask<Integer> {
private final int[] numbers;
private final int start;
private final int end;
public SumTask(int[] numbers, int start, int end) {
this.numbers = numbers;
this.start = start;
this.end = end;
}
@Override
protected Integer compute() {
if (end - start <= 10) { // Base case for small tasks
int sum = 0;
for (int i = start; i < end; i++) {
sum += numbers[i];
}
return sum;
} else {
int mid = (start + end) / 2;
SumTask task1 = new SumTask(numbers, start, mid);
SumTask task2 = new SumTask(numbers, mid, end);
task1.fork(); // Fork the first task
int result2 = task2.compute(); // Compute the second task
int result1 = task1.join(); // Join the first task result
return result1 + result2; // Combine results
}
}
public static void main(String[] args) {
int[] numbers = new int[100]; // Example array
for (int i = 0; i < numbers.length; i++) {
numbers[i] = i + 1; // Populate array with numbers 1-100
}
ForkJoinPool pool = new ForkJoinPool();
SumTask sumTask = new SumTask(numbers, 0, numbers.length);
int result = pool.invoke(sumTask); // Starts the task
System.out.println("Total Sum: " + result);
}
}
Commentary on the Code
In this example, the SumTask
class extends RecursiveTask<Integer>
. The compute
method decides whether the task should be executed directly or be split into subtasks. If the range is small (defined by our threshold of 10), it calculates the sum directly. Otherwise, it splits the range into two halves, forks the first half, computes the second half, and joins the results.
Common Challenges
1. Task Granularity
Challenge: Setting the threshold too high or too low can significantly affect performance. High granularity leads to excessive overhead because threads aren't utilized efficiently, while low granularity results in too many small tasks, leading to thread contention.
Solution: Experiment with different thresholds. Measure performance, and aim for a balance where the overhead of managing tasks does not outweigh the benefits of parallelization. A good rule of thumb is to keep the granularity size between 100 and 1000 iterations per task, depending on the complexity.
2. Managing State
Challenge: The Fork/Join framework is designed to handle stateless tasks, but sometimes developers need to maintain some state across tasks, which can lead to complex synchronization issues.
Solution: Avoid sharing mutable state across tasks. Use immutable objects where possible or consider using thread-safe collections provided by Java. If state must be shared, implement fine-grained locking or use atomic variables, which are designed for concurrent modifications.
3. Load Balancing
Challenge: If tasks are unevenly divided among threads, some threads may finish early, while others may still be processing. This results in poor resource utilization.
Solution: Implement better load balancing by dynamically adjusting the task division based on runtime conditions. You can also use a work-stealing approach, where idle threads can "steal" tasks from busier threads. This is already a feature in the Fork/Join framework, but understanding and utilizing it effectively can improve performance.
4. Understanding the Work Stealing Algorithm
Challenge: The work-stealing algorithm can be unintuitive. If tasks do not take roughly the same amount of time, some threads may become bottlenecks.
Solution: Design your tasks to perform similar amounts of work. Profiling your application can help identify where load imbalances may occur. Consider using fixed chunk sizes for tasks; this method allows threads to process a similar number of operations despite differences in execution time.
5. Debugging Parallel Code
Challenge: Debugging parallel code can be complex due to non-deterministic behavior, race conditions, and difficult-to-reproduce bugs.
Solution: Utilize logging and diagnostic tools to gain insights into the execution of your tasks. Tools like Java VisualVM can help observe thread activities and performance metrics. Additionally, testing with smaller data sets or controlled environments can help isolate issues efficiently.
Final Considerations
The Fork/Join framework in Java 7 significantly simplifies parallel programming. However, understanding its complexities and the common challenges can help you take full advantage of its capabilities. By addressing task granularity, managing state appropriately, ensuring load balancing, understanding work stealing, and effectively debugging, you can create high-performance applications that leverage multi-core processors effectively.
For more information about the Fork/Join framework, refer to the official Java documentation. Additionally, you can find various optimization techniques in Oracle's Java Concurrency tutorial.
Thanks for reading! I hope this article helps you navigate the intricacies of the Fork/Join framework. Happy coding!
Checkout our other articles