Common Pitfalls in Java String to String Array Conversion
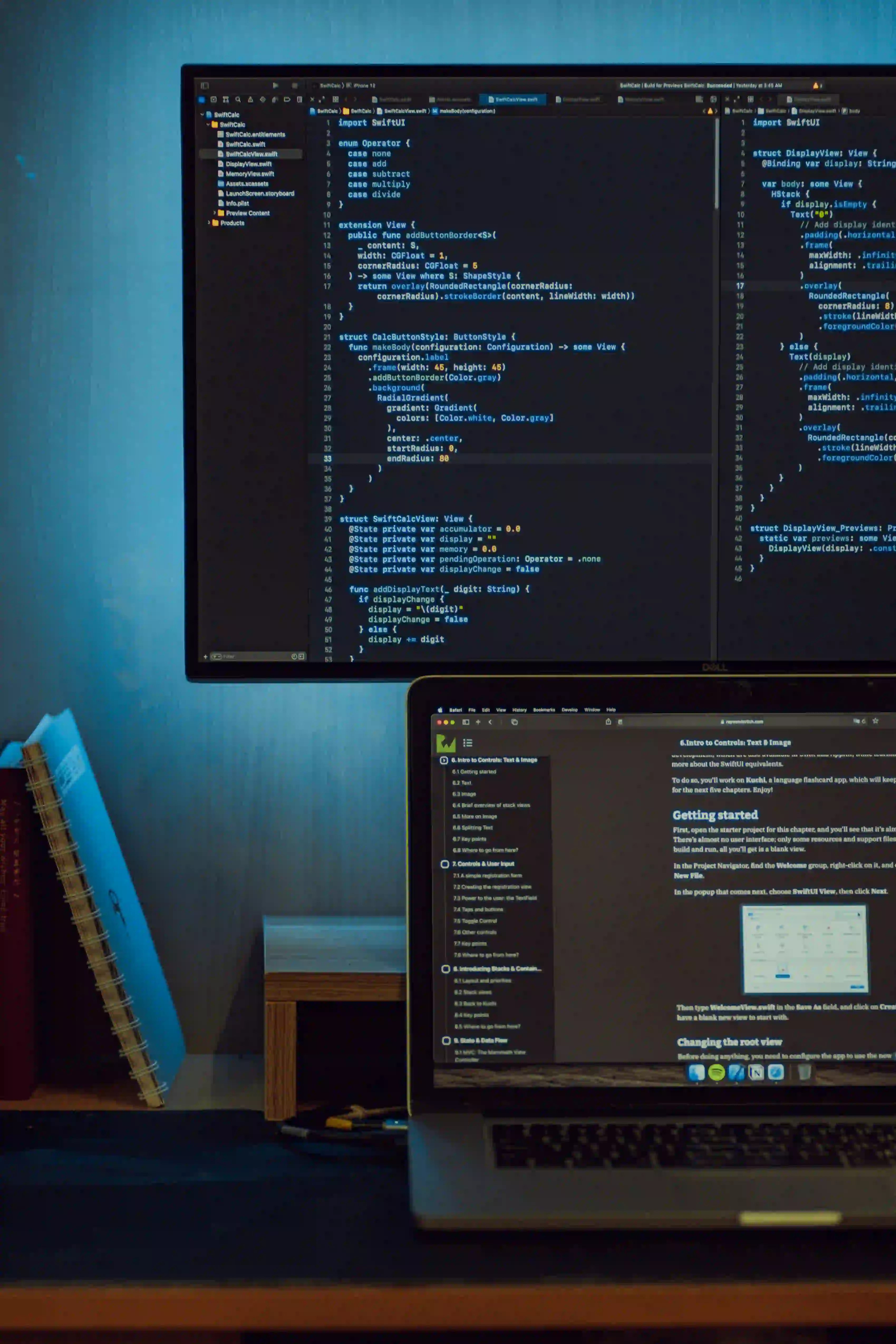
Common Pitfalls in Java String to String Array Conversion
Java is a powerful programming language that excels in versatility and performance. One of its common tasks is converting Strings into String arrays. However, as simple as this operation may seem, there are several pitfalls that developers often overlook. In this blog post, we will explore these pitfalls, provide clear examples to illustrate them, and suggest best practices to ensure smooth string-to-array conversions.
Understanding the Basics
Before diving into the pitfalls, let’s clarify the conversion process. A String to String array conversion usually involves splitting a String into multiple substrings based on a specific delimiter. For example, if we have a sentence, we may want to split it into words:
String sentence = "Java is a powerful programming language";
String[] words = sentence.split(" ");
In the snippet above, we split the sentence
into words using a space as the delimiter. This is readable, yet simple. However, various nuances can lead to unexpected results. Let’s explore these pitfalls one by one.
1. Using the Wrong Delimiter
The Pitfall
One of the most common mistakes is using the wrong delimiter or assuming that a certain character will always separate items.
Example
Consider the following code:
String data = "apple,banana,,cherry,,";
String[] fruits = data.split(",");
When we run this, the resulting array will include empty strings for the missing values:
// Result: ["apple", "banana", "", "cherry", "", ""]
The Fix
If your actual intention is to skip empty entries, you can use the -1
limit parameter in split
to omit trailing empty strings:
String[] fruits = data.split(",", -1);
// Result: ["apple", "banana", "", "cherry"]
Check out the Java String documentation for further information on the split
method.
2. Ignoring Case Sensitivity
The Pitfall
When splitting strings, developers sometimes forget that the delimiter can also be case-sensitive.
Example
Take a look at this:
String fruits = "Apple,banana,Cherry";
String[] result = fruits.split(",");
If the task is to separate fruits with case in mind, then only looking at "Apple" might cause issues if you expect case-insensitivity.
The Fix
To handle case-insensitivity, ensure that you apply the same case to your inputs:
String fruits = "apple,banana,cherry";
String[] result = fruits.toLowerCase().split(",");
// Result: ["apple", "banana", "cherry"]
3. Misestimating Array Length
The Pitfall
Another frequent mistake is presuming a fixed length for the resulting array. This can lead to potential ArrayIndexOutOfBoundsException
.
Example
Consider the following code snippet:
String input = "first,second,third";
String[] parts = input.split(",");
System.out.println(parts[3]); // Throws ArrayIndexOutOfBoundsException
The Fix
Always utilize dynamic structures such as Lists for better flexibility:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
String input = "first,second,third";
List<String> partsList = new ArrayList<>(Arrays.asList(input.split(",")));
System.out.println(partsList); // Result: [first, second, third]
4. Forgetting About Regular Expressions
The Pitfall
The split
function uses regular expressions, and people sometimes overlook this fact. Special characters can create unexpected behavior.
Example
If special characters act as delimiters:
String data = "one.two;three:four";
String regex = "[.;:]"; // Splits data at any '.', ';', or ':'
String[] elements = data.split(regex);
The Fix
To correctly handle special characters, escape them in your regular expression:
String data = "one.two;three:four";
String[] elements = data.split("[.\\;:]");
// Result: ["one", "two", "three", "four"]
5. Not Accounting for Leading/Trailing Delimiters
The Pitfall
Developers often forget to account for potential leading or trailing delimiters in the input string.
Example
String str = ",apple,banana,";
String[] items = str.split(",");
Not considering this can lead to the presence of unnecessary empty strings.
The Fix
One way to handle this is to trim the string before splitting:
String str = ",apple,banana,";
String[] items = str.trim().split(",");
// Result: ["apple", "banana"]
My Closing Thoughts on the Matter
Converting Strings to String arrays in Java might seem straightforward, but it comes with its own sharing of pitfalls. By understanding common mistakes such as using incorrect delimiters, ignoring case sensitivity, misestimating array lengths, misunderstanding how regular expressions operate in split
, and neglecting leading/trailing delimiters, you can significantly enhance the reliability of your string manipulations.
Remember, when working with strings, always test edge cases and unusual inputs. Effective string handling is an essential skill that will help you prevent common errors before they become a problem in production code.
To learn more about working with strings in Java, explore Java String Methods.
Final Thoughts
Arming yourself with this knowledge can make you a more proficient Java developer. Continually practice string manipulation scenarios and stay updated with the latest Java enhancements. Happy coding!