Transforming Java EE Monoliths: 5 Key Challenges
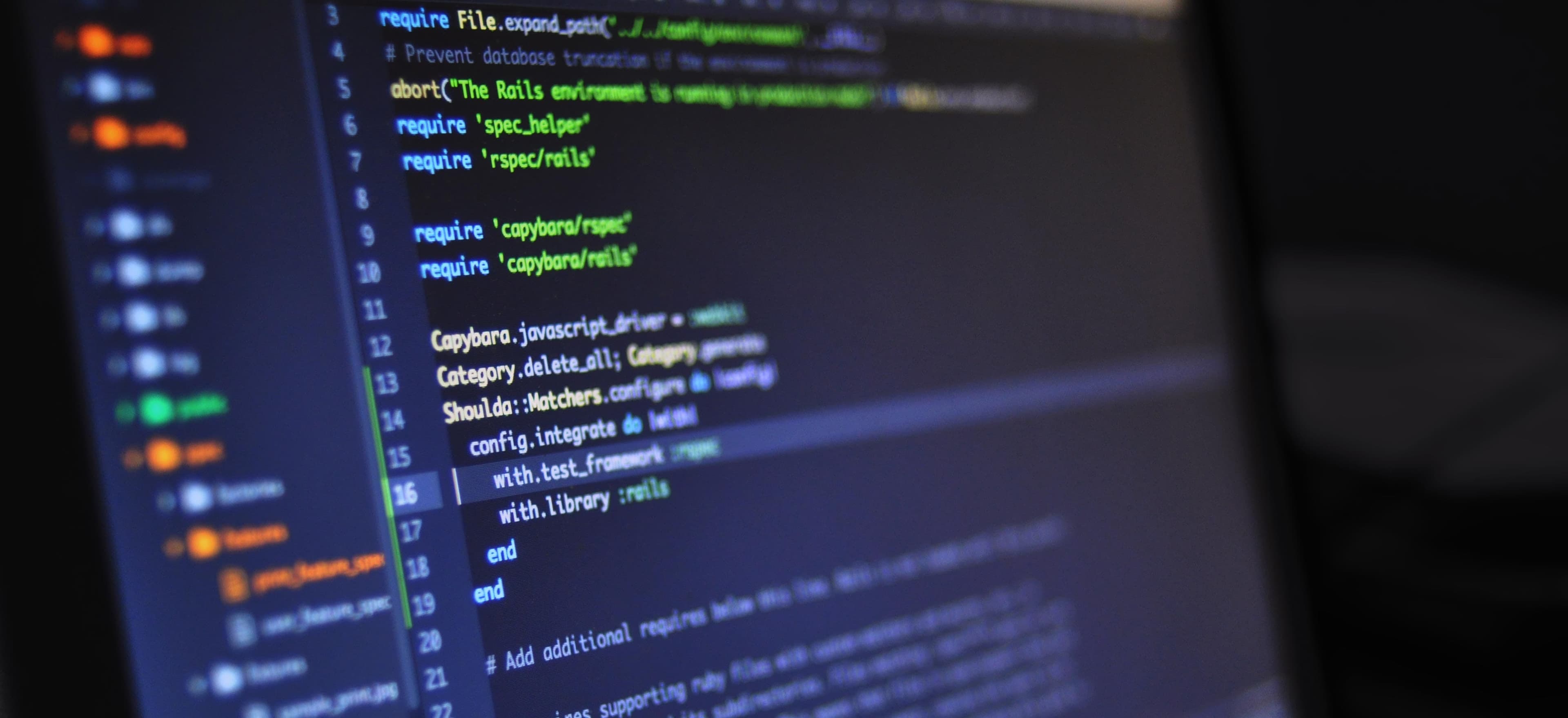
- Published on
Transforming Java EE Monoliths: 5 Key Challenges
Java EE, now known as Jakarta EE, has long been a stalwart of enterprise applications. However, as businesses evolve, so do their needs. Many organizations recognize that their monolithic Java EE applications are becoming bottlenecks, hindering agility, scalability, and speed. Migrating these monoliths to a more modular or cloud-native architecture can unleash significant potential but also comes with its own set of challenges.
In this blog, we'll explore five key challenges faced during the transformation of Java EE monoliths and provide strategies to navigate these hurdles successfully.
1. Understanding the Monolith
The Challenge
Before you can effectively transform a monolithic application, you need to fully understand its architecture and dependencies. In many cases, applications have developed over several years, leading to complexities that may not be clear even to the original developers.
The Solution
Conduct a Comprehensive Analysis
Start by mapping out the entire application architecture. Identify the different modules, relationships, and dependencies using tools like Structure101 or SonarQube.
// Example of a basic module structure
public class OrderService {
public void createOrder(OrderDTO order) {
// Business logic to create an order
}
}
// Dependency structure
public class OrderController {
private OrderService orderService;
public OrderController(OrderService service) {
this.orderService = service;
}
}
Why? This enables you to recognize tightly coupled components that may require decoupling during the migration process.
2. Decoupling Components
The Challenge
One of the primary hurdles in breaking down a monolithic application is the tightly coupled nature of its components. Breaking these dependencies can often feel like defusing a bomb – one wrong move, and everything could collapse.
The Solution
Implement Strangler Fig Pattern
The Strangler Fig Pattern allows you to gradually replace parts of a monolith with new microservices. This can be achieved through a reverse proxy that redirects incoming requests to either the old or new application, depending on the necessity.
Here’s an example of how you might begin to refactor an Order Service into a microservice:
@RestController
@RequestMapping("/orders")
public class OrderController {
@GetMapping("/{id}")
public OrderDTO getOrder(@PathVariable String id) {
// Fetch order via a new microservice
return orderService.fetchOrderById(id);
}
}
Why? This gradual replacement minimizes risks and allows for real-time performance monitoring, making it easier to pinpoint issues as they arise.
3. Data Management
The Challenge
Monolithic applications typically rely on a single database for their data storage needs. When migrating to microservices, there often arises a need to separate data into various databases according to the service it pertains to, which raises consistency and transaction management challenges.
The Solution
Focus on Database per Service and Eventual Consistency
Consider leveraging the concept of "Database per Service." Each microservice can manage its database, tailored specifically for its needs. Use event-driven architectures (such as Apache Kafka) for data synchronization across services.
// Example of publishing an event
public void createOrder(OrderDTO order) {
// Logic to create order
eventPublisher.publish(new OrderCreatedEvent(order));
}
Why? This approach not only enhances service isolation but also improves scalability. While managing consistency can be tricky, using tools like Spring Cloud Data Flow can help manage distributed data streams effectively.
4. Cultural Shift
The Challenge
Transforming monolithic applications is not just a technical challenge; it's also a cultural one. Teams accustomed to a waterfall approach might struggle with the agile methodologies necessary for microservices development.
The Solution
Embrace DevOps Practices
Introduce DevOps practices to encourage a culture of collaboration between development and operations teams. This includes adopting continuous integration and continuous deployment (CI/CD) pipelines.
Example of a Basic Jenkins Pipeline for Java EE Application
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building..'
sh 'mvn clean package'
}
}
stage('Deploy') {
steps {
echo 'Deploying....'
deployToYourServer() // hypothetical deployment command
}
}
}
}
Why? By streamlining operations, teams can deliver updates more frequently and improve the overall efficiency of the development lifecycle.
5. Performance Monitoring and Testing
The Challenge
As you break down a monolith into microservices, maintaining performance and managing testing complexity can become an uphill task. Issues that were previously managed at an application level now need individual attention.
The Solution
Invest in Application Performance Monitoring (APM)
Utilizing APM tools such as New Relic or Prometheus can help identify bottlenecks and performance constraints in your microservices. Load testing tools such as JMeter and Gatling can be integrated into your CI/CD pipeline to ensure that each service remains responsive.
Why? Proactive performance management allows you to react promptly to issues and maintain a high user experience.
Lessons Learned
Transforming Java EE monoliths into microservices can be daunting, and it's easy to overlook key challenges in the process. By investing time in understanding your existing architecture, employing the Strangler Fig Pattern, restructuring data management practices, fostering cultural changes within teams, and implementing robust performance monitoring tools, organizations can navigate the obstacle course that comes with transformation.
The benefits of this transition—agility, scalability, and maintainability—are immense, and overcoming these challenges is the first step towards unlocking them.
For those looking to dive deeper into the transformation journey, don't hesitate to explore resources like The Twelve-Factor App methodology for additional insights into building scalable and maintainable applications.
Happy coding!
Checkout our other articles