Overcoming Common Hurdles in Software Development Teams
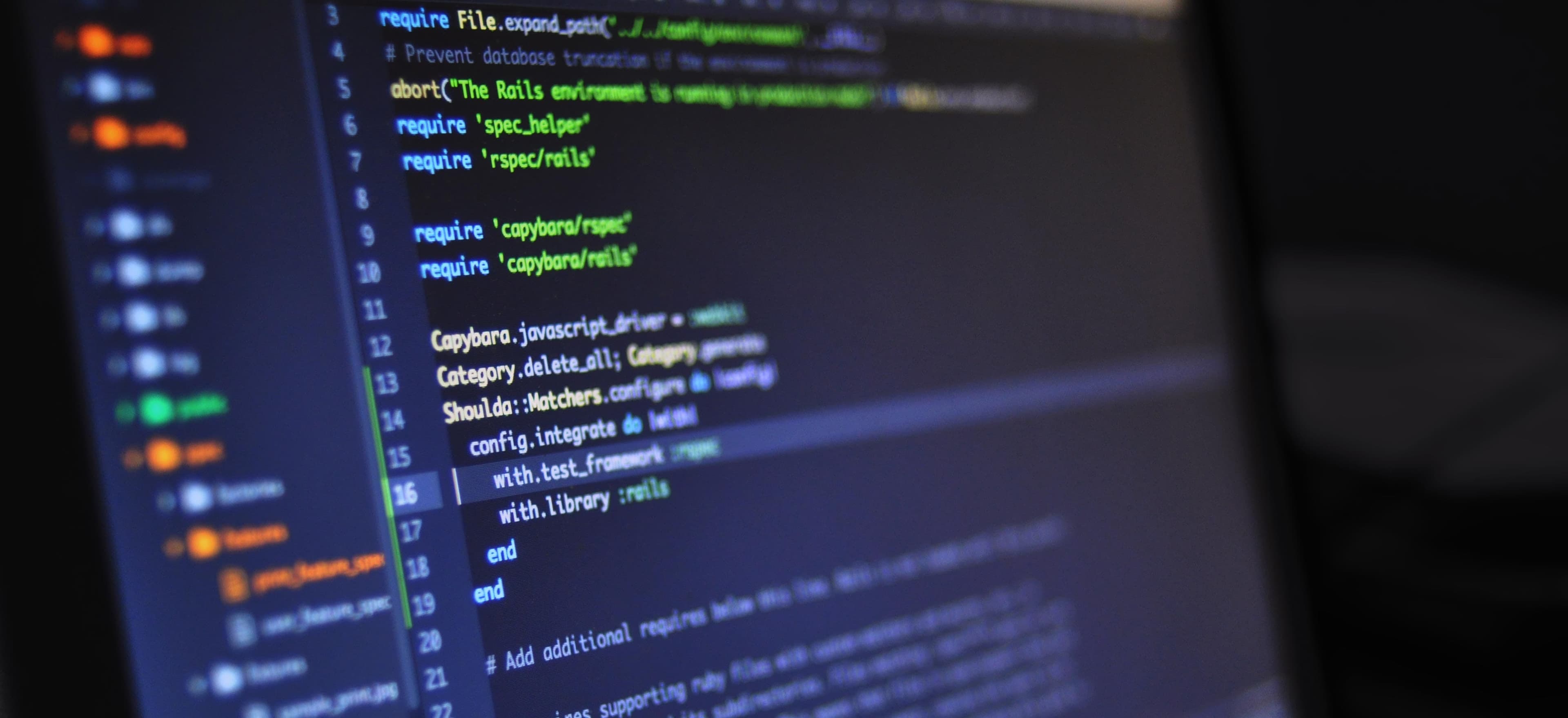
- Published on
Overcoming Common Hurdles in Software Development Teams
In the ever-evolving landscape of software development, teams often face a myriad of challenges. Whether it's adjusting to new technologies, managing communication gaps, or handling deadlines, these hurdles can significantly impact productivity and team morale. In this blog, we'll explore some common obstacles encountered in software development teams and offer practical strategies for overcoming them.
Understanding Common Hurdles
Before delving into solutions, it's crucial to identify the common hurdles that teams typically face. These include:
- Communication Barriers
- Lack of Collaboration
- Unclear Requirements
- Scope Creep
- Technical Debt
Let’s dissect each of these challenges and outline actionable steps to address them.
1. Communication Barriers
Effective communication is the backbone of any thriving software development team. However, silos often develop, especially in remote environments.
Strategies to Improve Communication:
-
Regular Stand-up Meetings: Hold short, daily team huddles to discuss progress and roadblocks. This keeps everyone in the loop and fosters a team-oriented environment.
-
Use Collaborative Tools: Embrace communication platforms like Slack or Microsoft Teams for real-time interactions and quick updates.
Example:
public class TeamChat {
public static void main(String[] args) {
SlackBot slack = new SlackBot();
slack.postMessage("Good morning everyone! Let's tackle today's challenges together.");
}
}
In this code snippet, we're simulating a Slack notification to get the team energized for the day. Constant communication can help break down barriers.
2. Lack of Collaboration
Collaboration is essential for innovation in software development. When team members work in isolation, it can hinder creativity and reduce the quality of output.
Strategies to Foster Collaboration:
-
Pair Programming: pairing developers together can spark innovative solutions and facilitate skill-sharing.
-
Code Reviews: Conduct regular code reviews. This encourages feedback, helps catch errors early, and fosters a sense of shared ownership.
Example:
public class CodeReview {
public void reviewCode(String code) {
// Call reviewer for feedback
Reviewer reviewer = new Reviewer();
reviewer.giveFeedback(code);
}
}
This simple class represents a code review process, emphasizing the importance of peer feedback in breaking down silos and fostering collaboration.
3. Unclear Requirements
One of the most significant pitfalls in software development is the lack of clear requirements, leading to wasted time and resources.
Strategies for Clarity:
-
Engagement with Stakeholders: Involve stakeholders early and often to understand their needs. Regular feedback loops can ensure everyone is aligned.
-
User Stories and Acceptance Criteria: Use methodologies like Agile or Scrum to create user stories and define acceptance criteria. This clarifies expectations and deliverables.
Example:
public class UserStory {
private String story;
private String acceptanceCriteria;
public UserStory(String story, String acceptanceCriteria) {
this.story = story;
this.acceptanceCriteria = acceptanceCriteria;
}
public void display() {
System.out.println("User Story: " + story + " | Acceptance Criteria: " + acceptanceCriteria);
}
}
Using user stories helps to encapsulate the requirements in a clear and understandable format, helping to avoid confusion.
4. Scope Creep
Scope creep refers to the gradual expansion of project boundaries, often leading to delays and budget overruns.
Strategies to Manage Scope:
-
Set Clear Boundaries: At the project's outset, clearly define what's included and what's not.
-
Change Control Process: Implement a formal process for handling requests for changes. This includes assessing the impact on timelines and resources.
Example:
public class ScopeManager {
private boolean isChangeRequested = false;
public void requestChange() {
isChangeRequested = true;
}
public void assessImpact() {
if (isChangeRequested) {
System.out.println("Assessing the impact of the requested change...");
// Additional processing logic
}
}
}
This example shows how to manage change requests actively. Proactive management of scope can prevent delays and dissatisfaction.
5. Technical Debt
Technical debt refers to the implied cost of future refactoring caused by the expedient but not optimal decisions made during the project lifecycle. Ignoring technical debt can lead to higher long-term maintenance costs.
Strategies to Manage Technical Debt:
-
Regular Refactoring: Make it part of the team’s routine to revisit and improve existing code. This can reduce long-term maintenance overhead.
-
Quality Metrics: Use coding standards and metrics to help assess technical debt and prioritize debts that need addressing.
Example:
public class TechnicalDebt {
public void refactorCode(String code) {
// Refactor logic goes here
System.out.println("Refactoring code for better maintainability...");
}
}
In this example, we highlight the significance of scheduled refactoring sessions to manage technical debt effectively.
The Bottom Line
Overcoming the hurdles faced in software development requires proactive measures and a culture of collaboration. By fostering communication, clarifying requirements, setting boundaries for scope, and managing technical debt, teams can significantly enhance their productivity and morale.
Always remember that every challenge comes with an opportunity for growth. Embrace these hurdles as chances to learn and improve as a cohesive unit—thus, propelling your software development team toward success.
If you want to dive deeper into improving team dynamics in software development, consider checking out Agile Approaches for Software Development or Effective Communication Strategies in Teams.
By implementing the discussed strategies, not only can teams overcome operational hurdles, but they can also excel and pave the way for innovation in their projects. Happy coding!
Checkout our other articles