Enhancing Java: Boosting Composition and Delegation Support
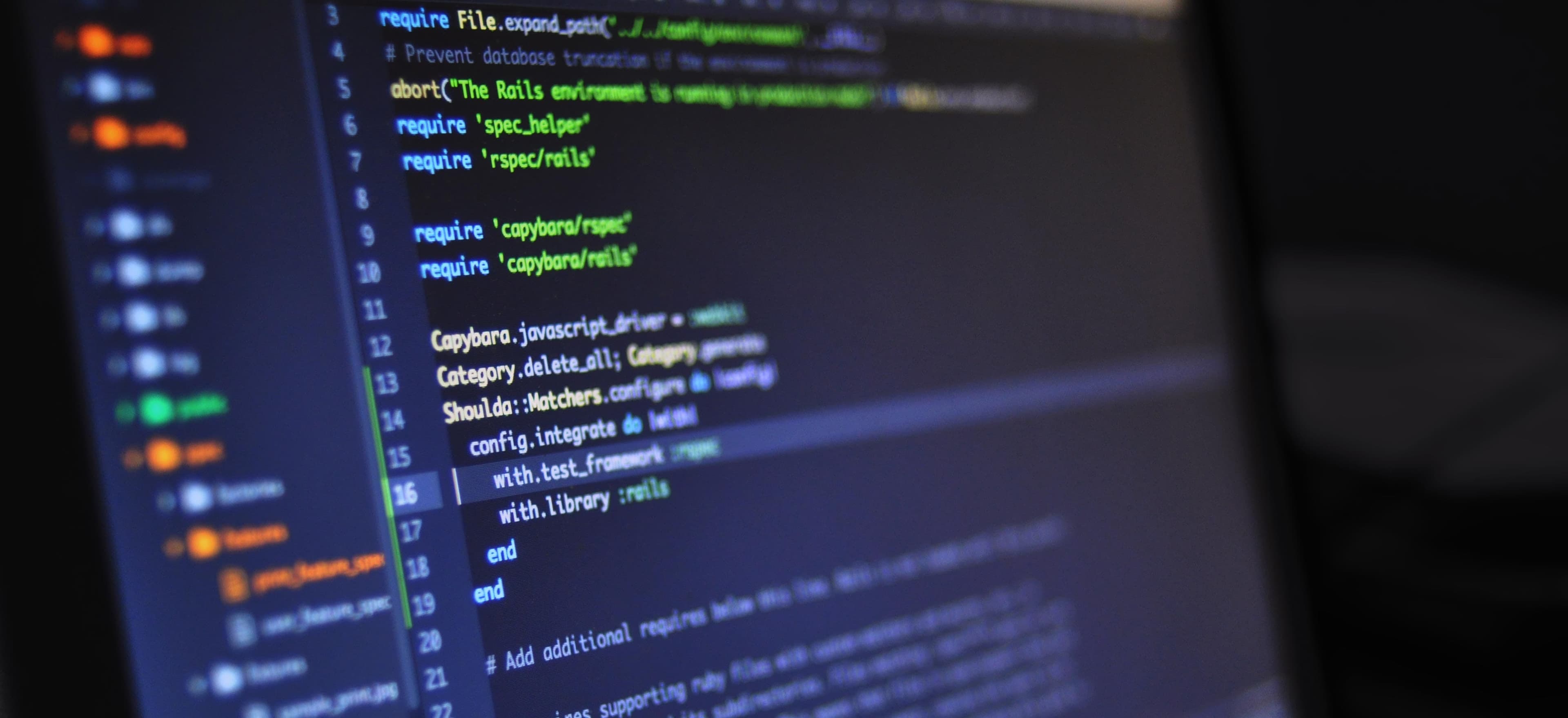
- Published on
Enhancing Java: Boosting Composition and Delegation Support
Java has long been a dominant player in the realm of software development. From its extensive libraries to its robust architecture, it provides developers with tools that enhance productivity and maintainability. However, even the best tools can be improved. In this blog post, we will explore ways to enhance Java by boosting support for composition and delegation, two design principles that can lead to cleaner, more manageable code.
What are Composition and Delegation?
Before diving into enhancements, let's define what we mean by composition and delegation:
Composition
Composition is a design principle where a class is composed of one or more objects from other classes, allowing for functionality reuse and the creation of complex types. It emphasizes a "has-a" relationship, wherein one class contains instances of another class.
Example:
class Engine {
void start() {
System.out.println("Engine starting...");
}
}
class Car {
private Engine engine;
public Car() {
this.engine = new Engine(); // Composition
}
public void start() {
this.engine.start();
System.out.println("Car is now running.");
}
}
In this example, the Car
class contains an instance of Engine
. This encapsulation allows the Car
class to leverage the Engine
functionality without inheriting from it.
Delegation
Delegation is a technique where an object hands off responsibility for a particular task to another object. This helps maintain separation of concerns and promotes loose coupling.
Example:
class Printer {
void print(String message) {
System.out.println(message);
}
}
class Document {
private Printer printer;
public Document() {
this.printer = new Printer(); // Delegation
}
public void printDocument(String content) {
printer.print(content); // Delegates the print functionality
}
}
Here, the Document
class delegates its printing responsibility to a Printer
class. This ensures that the Document
can focus on its core functionality while leveraging another class for specific tasks.
Why Composition and Delegation Matter
Composition and delegation are crucial for several reasons:
- Flexibility: They allow changes to be made to individual components without affecting the entire system.
- Reusability: Code can be reused across different parts of the application or different applications altogether.
- Simpler Testing: Each component can be tested in isolation, leading to better test coverage and reliability.
- Separation of Concerns: They help to organize code better, making it more maintainable and understandable.
Enhancing Composition and Delegation Support in Java
1. Use Interfaces for Composition
Java interfaces allow you to define methods that must be implemented by a class. Leveraging interfaces in composition can enhance functionality and foster a design that is both flexible and easily extendable.
Example of Interface in Composition:
interface Vehicle {
void drive();
}
class Engine implements Vehicle {
@Override
public void drive() {
System.out.println("Engine is now driving.");
}
}
class Car implements Vehicle {
private Vehicle vehicle;
public Car(Vehicle vehicle) {
this.vehicle = vehicle; // Composition with interface
}
@Override
public void drive() {
vehicle.drive();
System.out.println("Car is now running.");
}
}
In this code, Car
can work with any Vehicle
. The flexibility provided by the Vehicle
interface allows for different types of vehicles to be swapped in and out.
2. Builder Pattern for Flexible Composition
The Builder pattern is another design pattern that aids in effective composition, particularly when dealing with complex objects. It allows for constructing an object step by step, leading to more manageable code.
Example of Builder Pattern:
class Car {
private String color;
private String make;
private Car(CarBuilder builder) {
this.color = builder.color;
this.make = builder.make;
}
public static class CarBuilder {
private String color;
private String make;
public CarBuilder setColor(String color) {
this.color = color;
return this;
}
public CarBuilder setMake(String make) {
this.make = make;
return this;
}
public Car build() {
return new Car(this);
}
}
}
Here, rather than using a constructor with many parameters, we empower users to compose Car
objects step-by-step. This enhances readability and maintainability.
3. Use Decorator Pattern for Dynamic Behavior
The Decorator pattern is ideal for adding functionality to classes dynamically. It allows for composition of behavior and enhances flexibility, especially in complex systems.
Decorator Pattern Example:
interface Coffee {
double cost();
}
class SimpleCoffee implements Coffee {
@Override
public double cost() {
return 5.00;
}
}
abstract class CoffeeDecorator implements Coffee {
protected Coffee decoratedCoffee;
public CoffeeDecorator(Coffee coffee) {
this.decoratedCoffee = coffee;
}
@Override
public double cost() {
return decoratedCoffee.cost();
}
}
class MilkDecorator extends CoffeeDecorator {
public MilkDecorator(Coffee coffee) {
super(coffee);
}
@Override
public double cost() {
return super.cost() + 1.50; // Adds the cost of milk
}
}
With this example, we can dynamically add new features to the Coffee
, such as adding milk, by wrapping the original class. This makes the code extensible while adhering to the open/closed principle.
4. Functional Interfaces and Lambda Expressions
With Java 8 and later versions, functional interfaces and lambda expressions provide a modern approach to delegation. They help streamline the delegation of tasks in a more concise manner.
Example of Functional Interface:
@FunctionalInterface
interface Executor {
void execute();
}
class Task {
private Executor executor;
public Task(Executor executor) {
this.executor = executor; // Delegate task execution
}
public void run() {
System.out.println("Starting task...");
executor.execute(); // Delegation using lambda
}
}
// Usage
public class Main {
public static void main(String[] args) {
Task task = new Task(() -> System.out.println("Task executed!"));
task.run();
}
}
This approach allows us to utilize the power of lambdas, resulting in cleaner and more expressive code while executing tasks.
5. Frameworks and Libraries
Numerous frameworks facilitate the implementation of composition and delegation patterns in Java applications. Frameworks like Spring leverage dependency injection, allowing for clearer delegation of responsibilities.
@Component
class UserService {
@Autowired
private UserRepository userRepository;
public User findUserById(Long id) {
return userRepository.findById(id);
}
}
Here, the UserService
class is composed of a UserRepository
. The Spring framework takes care of injecting the repository, demonstrating how composition can enhance modular design.
Final Thoughts
Enhancing Java through improved support for composition and delegation can lead to cleaner, more manageable, and more flexible code. By leveraging interfaces, design patterns, functional programming, and robust frameworks, developers can build applications that not only meet today’s needs but also adapt to future challenges.
For more in-depth articles on design patterns and best practices in Java, you might explore resources like Refactoring Guru and Baeldung for practical applications and insights.
By consciously applying these design principles, we can create software that stands the test of time and resonates with maintainability and clarity. Happy coding!
Checkout our other articles