Is TDD Still Relevant in Modern Software Development?
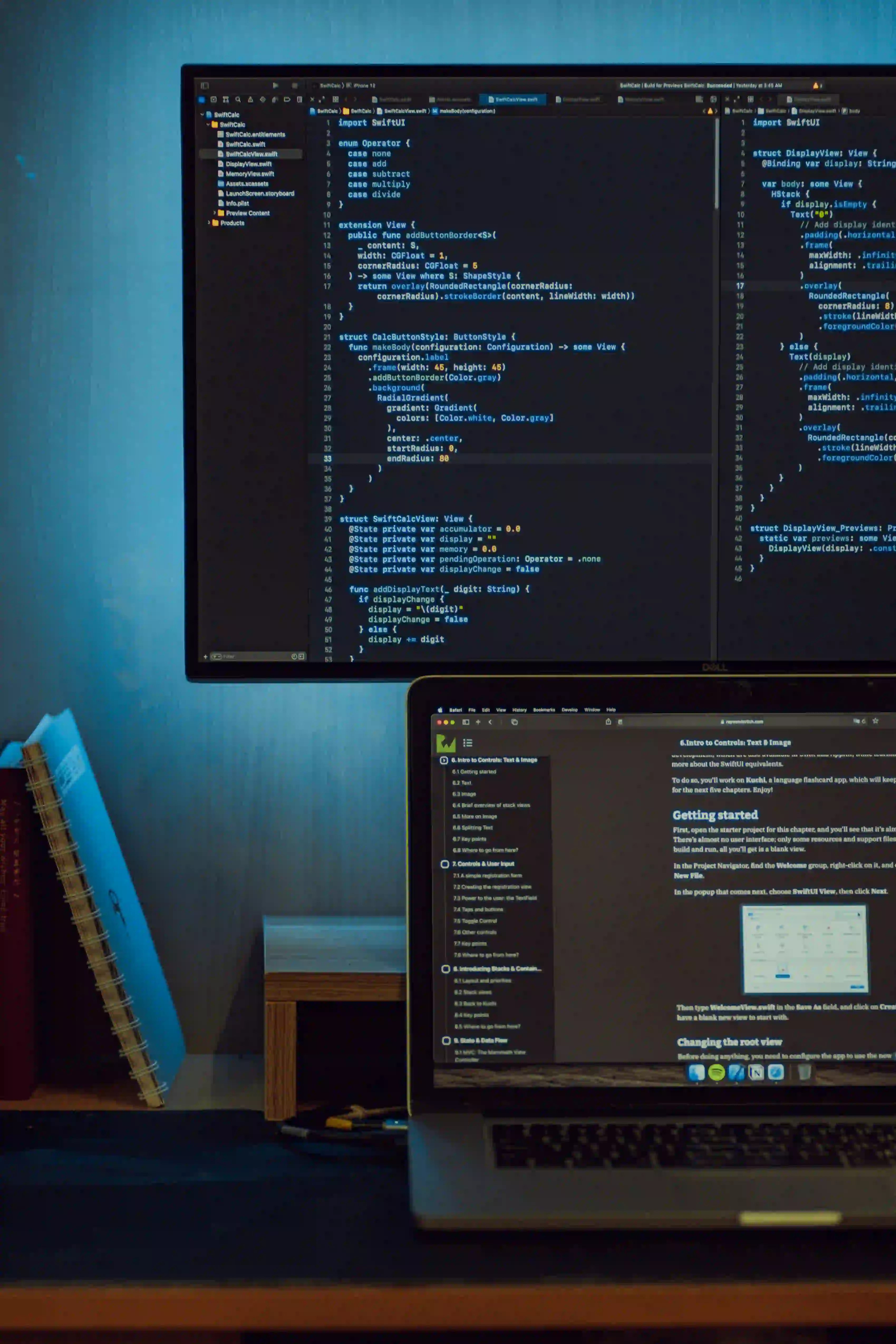
Is TDD Still Relevant in Modern Software Development?
Test-Driven Development (TDD) is a software development process rooted in the concept of writing automated tests before writing the actual code. Since its inception, TDD has generated considerable debate within the programming community. Critics argue it's time-consuming, while proponents cite its effectiveness in improving code quality and maintainability. So, is TDD still relevant in modern software development? Let's explore this question through several lenses.
What is TDD?
At its core, TDD follows a simple yet structured approach:
-
Write a Test: Begin by writing a test for the piece of functionality you’re about to implement. This test will fail initially because the functionality doesn’t exist yet.
-
Run the Test: Execute the test to ensure it fails. This is a critical step to validate that your test is indeed testing what it’s supposed to.
-
Write the Code: Implement the simplest code needed to pass the test.
-
Run the Test Again: Check if the newly implemented code passes the test.
-
Refactor: Clean up the code while ensuring it remains functional and still passes the tests.
-
Repeat: Repeat this process for the next piece of functionality.
Here’s a simple example to illustrate TDD:
// Step 1: Write a failing test
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class CalculatorTest {
@Test
void add_Numbers_ShouldReturnSum() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
// After writing the test, we realize the Calculator class does not exist yet.
// Step 3: Write the code
class Calculator {
public int add(int a, int b) {
return a + b;
}
}
// Step 5: Refactor if needed
In the example above, we start with a failing test (Step 1). We then create the Calculator
class to make the test pass (Step 3). If our initial implementation works as intended, we can refactor the code afterward (Step 5).
Advantages of TDD
While TDD might seem daunting at first, its benefits in modern software development can’t be overstated:
-
Improved Code Quality: Writing tests first ensures that you focus on the requirements from the outset. This often leads to cleaner, more maintainable code.
-
Documentation: The tests serve as a form of documentation. New developers can understand the expected behavior of the codebase through the tests provided.
-
Reduced Bugs: Since tests are written before the code, TDD emphasizes catching bugs early in the development process. Fixing issues at this stage is less costly than post-deployment.
-
Confidence in Refactoring: Continuous integration and frequent refactoring can be daunting. TDD provides safety nets through existing tests, giving developers the confidence to improve and change code.
-
Encourages Simple Design: TDD promotes a modular approach. When you focus on writing tests for small pieces of functionality, it encourages compartmentalization.
Challenges of TDD
Despite its advantages, TDD is not without its challenges:
-
Learning Curve: Beginners might find TDD to be overly complex or time-consuming. Understanding how to write good tests requires practice and depth in testing strategies.
-
Initial Slowdown: The process of writing tests before the actual code can seem slow initially. However, the speed improves as you become accustomed to the practice.
-
Maintaining Tests: With software evolution, outdated tests can become liabilities. Regularly updating them adds additional overhead that can be difficult to manage.
Is TDD Still Relevant?
As development practices evolve, one might wonder whether TDD still holds its ground. The short answer is yes. TDD remains relevant today for several reasons:
Agile Methodology
Agile development emphasizes iterative progress and adaptability. TDD fits neatly within this paradigm, allowing teams to deliver functional software in short cycles, while reducing risks through testing.
DevOps Integration
In a DevOps culture, where continuous delivery and deployment are pivotal, TDD helps streamline the integration process. Automation through TDD ensures that code changes can be continuously tested and integrated with confidence.
Unit Testing Frameworks
Modern languages and frameworks have embraced TDD, providing robust support for unit testing. Libraries such as JUnit for Java, Mocha for JavaScript, and NUnit for .NET make the TDD process smoother and more manageable.
Continuous Growth of Microservices
Microservices architecture encourages the decomposition of applications into smaller services. TDD has a beneficial role here by assuring that each microservice can function independently and correctly through rigorous testing.
Alternatives to TDD
While TDD has its merits, several alternative approaches exist:
-
Behavior-Driven Development (BDD): BDD extends TDD by introducing a more business-focused description of solutions. Frameworks like Cucumber allow for writing test cases in natural language.
-
Acceptance Test-Driven Development (ATDD): ATDD focuses more on the end-user's perspective by ensuring that requirements are agreed upon and captured in the form of acceptance criteria, which serve as tests.
-
Just-Enough Testing: Some teams prefer to do just-enough testing as opposed to a fully TDD approach. This might mean writing tests only for the most critical parts of the application.
My Closing Thoughts on the Matter
In summary, TDD is still a viable and relevant methodology in modern software development. Despite its challenges, its benefits far outweigh the drawbacks for most teams. By fostering a culture of quality and iterative improvement, TDD complements contemporary Agile and DevOps practices effectively.
For further reading on the benefits of TDD, consider exploring Martin Fowler’s article on TDD and the testing best practices by Google.
In a rapidly evolving landscape, sticking to principles like TDD can safeguard developers against the pitfalls of poor software quality. Whether you are a seasoned professional or just starting, incorporating TDD into your development practices can pay off considerably in the long run.