Overcoming Challenges in Transforming Collections Effectively
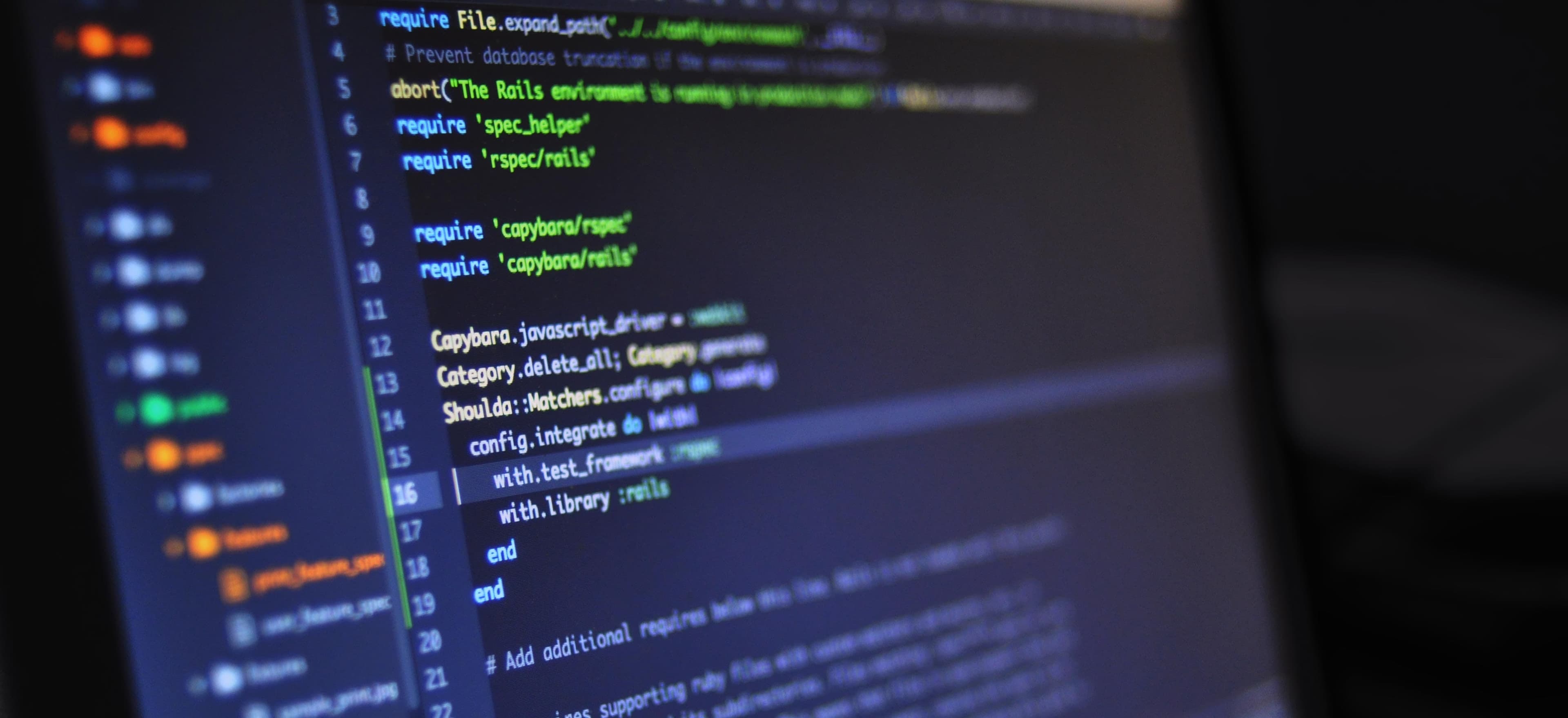
- Published on
Overcoming Challenges in Transforming Collections Effectively
The Roadmap
In the realm of data management, transforming collections is fundamental in leveraging stored data for analysis, reporting, and various applications. However, this process comes with several challenges that can hinder effectiveness. In this blog post, we will delve into practical strategies to overcome these challenges, with a focus on Java as our primary programming language.
Whether you're a novice programmer or an experienced developer, understanding how to manage collections and transform them efficiently can lead to better performance and optimized systems.
Understanding Collections in Java
Java Collections Framework (JCF) offers a unified architecture for representing and manipulating collections. It consists of interfaces (like List, Set, and Map) and classes that implement these interfaces, such as ArrayList, HashSet, and HashMap.
Why Use Collections in Java?
- Efficiency: Collections make it easier to manage multiple objects systematically.
- Easy to Manipulate: Built-in methods allow for quick data manipulation.
- Flexibility: The framework adapts to various data types and structures.
Here’s an example of creating a simple list of numbers using ArrayList
:
import java.util.ArrayList;
import java.util.List;
public class NumberList {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>();
// Adding items to the list
numbers.add(1);
numbers.add(2);
numbers.add(3);
// Printing the list
System.out.println("Numbers in the list: " + numbers);
}
}
Commentary:
In the code above, we demonstrate basic operations with an ArrayList
. Using collections in Java allows you to dynamically manage your data—adding, removing, and iterating over elements efficiently.
Common Challenges in Transforming Collections
As you work with collections, you may encounter several obstacles:
- Performance Issues: Large datasets can cause slowdowns, especially during transformation processes.
- Memory Management: Inefficient use of resources can lead to memory leaks or excessive garbage collection.
- Complexity: Understanding complex data transformations can be overwhelming and may lead to bugs.
Strategies for Overcoming Challenges
1. Optimize Data Structures
Choosing the right collection type is crucial. For instance, if you need quick lookups, consider using a HashMap
:
import java.util.HashMap;
import java.util.Map;
public class UserMap {
public static void main(String[] args) {
Map<Integer, String> users = new HashMap<>();
users.put(1, "Alice");
users.put(2, "Bob");
// Accessing a user
System.out.println("User with ID 1: " + users.get(1));
}
}
Commentary:
Using a HashMap
for storing user data allows us to retrieve values in O(1) time complexity. Understanding when to use which data structure can significantly improve performance.
2. Stream API for Transformation
Java 8 introduced the Stream API, which allows for functional-style programming with collections. This can aid in transforming data effortlessly. For example, filtering even numbers from a list can be achieved succinctly:
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class FilterEvenNumbers {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>();
for (int i = 0; i < 10; i++) {
numbers.add(i);
}
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println("Even Numbers: " + evenNumbers);
}
}
Commentary:
The Stream API simplifies operations like filtering and mapping, making transformations more readable and concise. By optimizing iterables through streams, both code readability and efficiency are enhanced.
3. Efficient Memory Management
To avoid memory issues, always consider the size of your collections. Pre-size your lists or maps when you know the expected size to minimize dynamic resizing. For example:
import java.util.ArrayList;
public class PreSizeList {
public static void main(String[] args) {
// Initializing ArrayList with an expected size
ArrayList<Integer> numbers = new ArrayList<>(100);
for (int i = 0; i < 100; i++) {
numbers.add(i);
}
System.out.println("Pre-sized List Length: " + numbers.size());
}
}
Commentary:
By initializing the ArrayList
with an expected size, you reduce the overhead of resizing during the addition of elements. This precaution can significantly enhance performance when dealing with large datasets.
Handling Nested Collections
Working with nested collections, such as a list of maps or a map of lists, adds additional complexity but can be managed with careful design and understanding.
Example of Handling Nested Collections
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class NestedCollections {
public static void main(String[] args) {
List<Map<String, String>> users = new ArrayList<>();
Map<String, String> user1 = new HashMap<>();
user1.put("id", "1");
user1.put("name", "Alice");
users.add(user1);
Map<String, String> user2 = new HashMap<>();
user2.put("id", "2");
user2.put("name", "Bob");
users.add(user2);
for (Map<String, String> user : users) {
System.out.println("User ID: " + user.get("id") + ", Name: " + user.get("name"));
}
}
}
Commentary:
In this sample, we create a list of maps to represent user data. Although it might seem cumbersome, leveraging nested collections can provide a way to handle more complex data models efficiently.
Key Takeaways
Overcoming challenges in transforming collections requires a thoughtful approach and a solid understanding of Java's capabilities. By choosing the right data structures, utilizing the Stream API, ensuring efficient memory management, and handling nested collections adeptly, you can transform data effectively and build highly efficient applications.
By implementing these strategies, you can significantly improve the performance and reliability of your Java applications. For more details on Java Collections, check out the official Java Documentation.
For further insights into optimizing your Java code and collections, consider visiting this tutorial on Java Collections.
Strong data management and transformation abilities will not only enhance your applications but also boost your career as a proficient Java developer. Happy coding!
Checkout our other articles