Overcoming Serialization Issues in Apache Camel and Redis
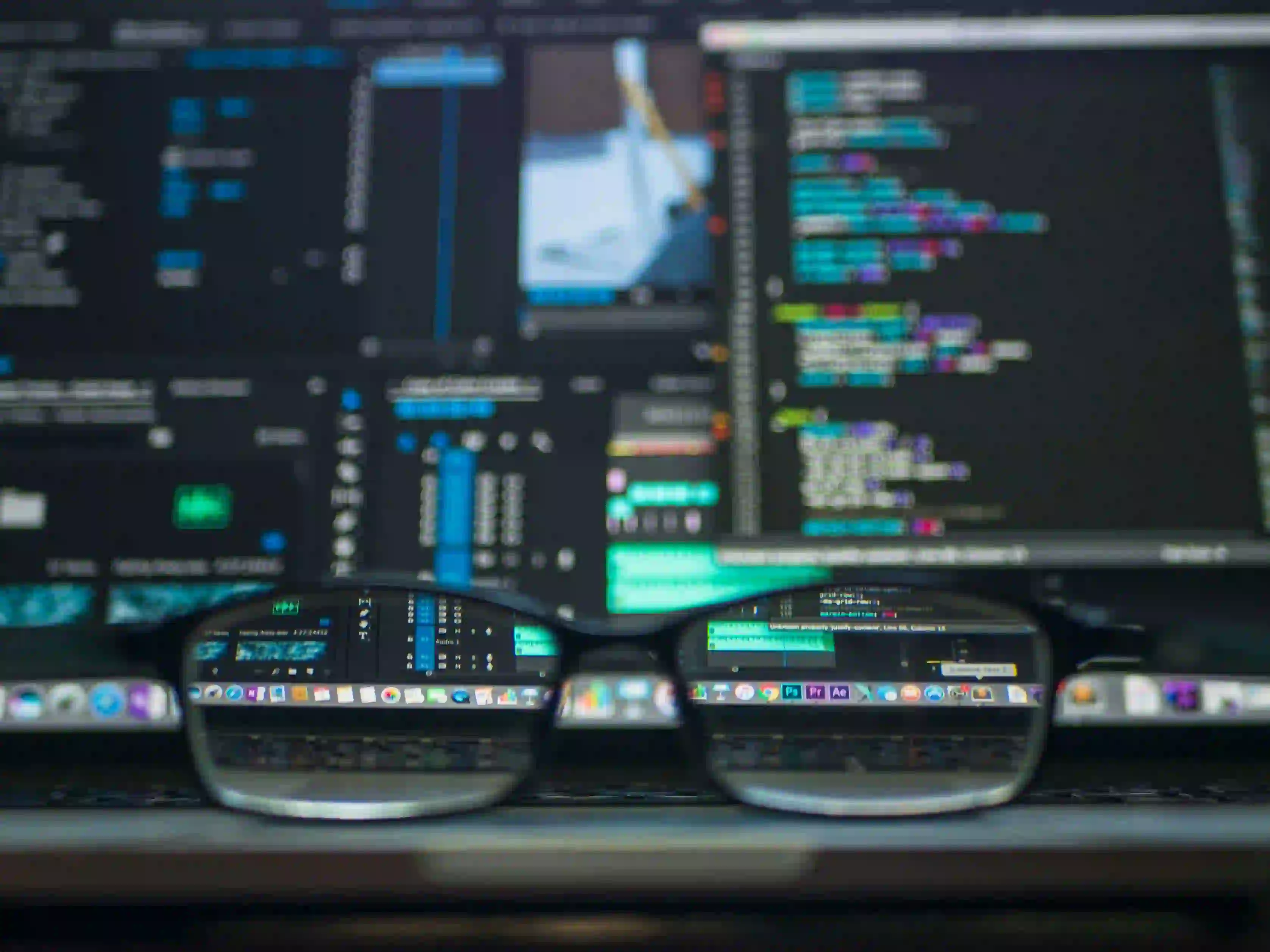
Overcoming Serialization Issues in Apache Camel and Redis
When working with data in distributed systems, serialization becomes one of the critical challenges developers face. It's especially true when integrating robust frameworks like Apache Camel with data stores such as Redis. In this blog post, we’ll explore how to overcome serialization issues in Apache Camel and Redis, providing clear explanations, examples, and insights into best practices.
Understanding Serialization
Serialization is the process of converting an object into a byte stream so that it can be easily transmitted or stored. Deserialization is the reverse process, where the byte stream is converted back into an object. Serialization is essential for sending objects over a network or storing them in databases.
Why Serialization Matters
In distributed systems, where different components may be running in separate environments, maintaining a consistent object format is crucial. For instance, Apache Camel enables route management and message processing, while Redis serves as a fast in-memory data store. When Camel routes messages containing Java objects to and from Redis, ensuring that these objects can be effectively serialized and deserialized is vital.
Common Serialization Issues
Here are some common serialization issues developers may encounter when using Apache Camel and Redis:
- Incompatibility of Object Structures: If the object structure changes after it has been serialized, deserialization may fail.
- Unsupported Data Types: Objects containing data types that aren't supported for serialization can lead to runtime exceptions.
- High Overhead: Inefficient serialization methods can introduce significant performance costs.
- Loss of Type Information: Serializing polymorphic objects may lead to loss of subclass information upon deserialization.
Solutions to Serialization Issues
1. Use Compatible Data Formats
When dealing with serialization in Apache Camel and Redis, always opt for a well-supported data format like JSON or XML. Camel provides built-in support for various data formats through its data format component.
Example - Serializing to JSON
import org.apache.camel.builder.RouteBuilder;
import org.apache.camel.model.dataformat.JsonLibrary;
public class MyRouteBuilder extends RouteBuilder {
@Override
public void configure() {
// Define a route that consumes messages from a queue and Marshall to JSON
from("direct:start")
.marshal().json(JsonLibrary.Jackson) // Using Jackson to convert to JSON
.to("redis://localhost:6379");
}
}
In this example, we use the Jackson library to marshal our Java objects to JSON. This format is widely accepted, easy to work with, and preserves the structure of your data.
2. Enable Logging for Debugging
Enable logging at various levels in your Camel application to capture serialization and deserialization errors. This can help you quickly identify issues as they arise.
import org.apache.camel.LoggingLevel;
from("direct:start")
.log(LoggingLevel.INFO, "Sending message: ${body}")
.marshal().json(JsonLibrary.Jackson)
.log(LoggingLevel.INFO, "Serialized message: ${body}")
.to("redis://localhost:6379");
This logging will provide outputs of the messages before and after serialization, helping you to see any changes.
3. Implement Custom Serialization Logic
When using complex object types or collections, you may need to implement custom serialization logic. By doing this, you gain control over how your objects are processed.
Example - Custom Serializer
import com.fasterxml.jackson.databind.ObjectMapper;
public class CustomSerializer {
private static final ObjectMapper objectMapper = new ObjectMapper();
public static String serialize(Object obj) throws Exception {
return objectMapper.writeValueAsString(obj);
}
public static <T> T deserialize(String jsonString, Class<T> clazz) throws Exception {
return objectMapper.readValue(jsonString, clazz);
}
}
Here, we use Jackson's ObjectMapper to convert objects to and from JSON strings. Custom serialization allows you to handle specific types and structures more flexibly.
4. Utilize Protobuf for Performance
In cases where high performance is required, consider using Protocol Buffers (Protobuf) as they provide a more efficient serialization format. Apache Camel supports Protobuf through its data format component.
Example - Using Protobuf
First, define your message:
syntax = "proto3";
message MyMessage {
string text = 1;
int32 number = 2;
}
Then serialize within your Camel route:
from("direct:start")
.marshal().protobuf(MyMessage.class) // Using Protobuf
.to("redis://localhost:6379");
Using Protobuf can significantly improve performance by minimizing the size of data transmitted over the network.
5. Work with Camel Redis Component
The Apache Camel Redis component has several features that can help with serialization. By configuring the Redis component to use appropriate serialization, you can ensure compatibility.
Example - Setting Up Redis Component
import org.apache.camel.component.redis.RedisComponent;
RedisComponent redisComponent = new RedisComponent();
redisComponent.setCamelContext(camelContext);
camelContext.addComponent("redis", redisComponent);
By tweaking the Redis component to fit the needs of your application, serialization issues can be minimized.
Testing and Validation
After implementing your serialization strategy, it’s essential to validate that everything works smoothly. Write unit and integration tests that:
- Verify both serialization and deserialization.
- Check for compatibility among different versions of your classes.
- Ensure that sending and retrieving data from Redis via Camel works without errors.
Useful Resources
In Conclusion, Here is What Matters
Serialization can indeed introduce complexities when integrating Apache Camel with Redis, but with the right strategies and tools, these challenges can be effectively managed. By using compatible data formats, enabling thorough logging, implementing custom serialization, leveraging Protobuf, and properly configuring the Redis component, you can overcome these hurdles.
As you work on your integration projects, keep these techniques in mind to ensure smooth data handling. By doing so, you’ll not only optimize performance but also improve the robustness of your applications. Happy coding!