Common Java Extensions You Didn't Know Existed
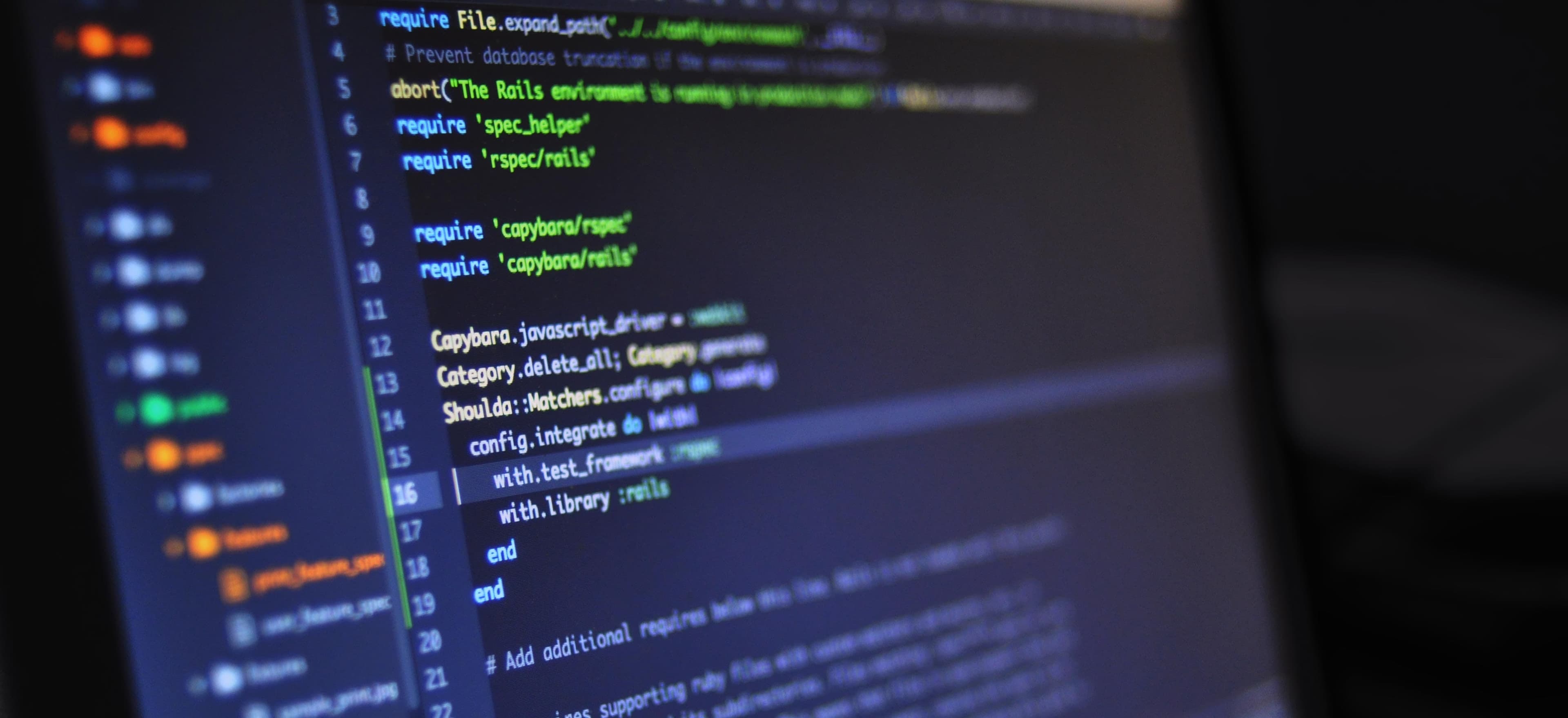
- Published on
Common Java Extensions You Didn't Know Existed
Java is one of the most widely used programming languages in the world, renowned for its platform independence, versatility, and robust ecosystem. While many developers are familiar with the core features of Java, there are numerous extensions and libraries that can significantly enhance development productivity, simplify complex tasks, and add powerful functionalities. This blog post will explore some of these common Java extensions that you may not know existed, along with practical examples to illustrate their usage.
1. Lombok
What is Lombok?
Lombok is a Java library that helps to reduce boilerplate code by automatically generating getters, setters, constructors, toString()
, hashCode()
, and equals()
methods. This library is highly valued for improving code readability and reducing the clutter that often comes with Java classes.
Why Use Lombok?
Developers often spend a significant amount of time writing boilerplate code. These repetitive tasks can detract from the more critical aspects of software development, such as implementing business logic and features. Lombok alleviates this burden.
Example: Using Lombok Annotations
Here is a simple example of how to use Lombok in a Java class.
import lombok.Data;
@Data
public class User {
private String name;
private int age;
}
In the example above, the @Data
annotation includes several other Lombok annotations (like @Getter
, @Setter
, @ToString
, and @EqualsAndHashCode
), so you don't need to write those methods manually.
Why This Matters?
Less boilerplate means cleaner code, making it easier to maintain and understand. You can learn more about Lombok here.
2. Apache Commons
What is Apache Commons?
Apache Commons is a collection of reusable Java components that provide utilities for tasks ranging from file handling to data manipulation and string operations. The library is modular, consisting of various subprojects that you can include as needed.
Why Use Apache Commons?
Leveraging established libraries can speed up development by allowing you to focus on implementing core functionality rather than reinventing the wheel.
Example: Using Commons Lang for String Manipulation
For instance, Apache Commons Lang provides a class called StringUtils
that has numerous static methods for string manipulation.
import org.apache.commons.lang3.StringUtils;
public class StringExample {
public static void main(String[] args) {
String str = " Hello, World! ";
// Trim whitespace
String trimmed = StringUtils.trim(str);
System.out.println(trimmed); // Outputs: "Hello, World!"
}
}
Why This Matters?
Using StringUtils
reduces errors and redundancy while improving code clarity. You can find more about Apache Commons libraries here.
3. JUnit
What is JUnit?
JUnit is a widely-used framework for unit testing in Java. It allows developers to write and run repeatable tests, fostering better code quality and maintainability.
Why Use JUnit?
Test-driven development (TDD) improves the reliability of your code and allows for rapid iteration and refactoring.
Example: Writing a Simple Test Case with JUnit
Here’s how you can write a simple unit test for a method in a class.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void addTest() {
Calculator calculator = new Calculator();
int result = calculator.add(10, 20);
assertEquals(30, result);
}
}
Why This Matters?
Automated testing ensures that your code works as intended whenever changes are made. The ability to catch regressions early can save time and costs in the long run.
4. Gson
What is Gson?
Gson is a Java library from Google that allows for the conversion of Java objects into JSON and vice versa. It is particularly useful for handling data formats in web applications or APIs.
Why Use Gson?
With APIs becoming a standard means of communication between applications, the ability to easily convert objects to and from JSON is crucial.
Example: Using Gson for JSON Serialization and Deserialization
Here's how to use Gson to convert a Java object into JSON.
import com.google.gson.Gson;
public class GsonExample {
public static void main(String[] args) {
Gson gson = new Gson();
User user = new User("Alice", 30);
// Serialize user to JSON
String json = gson.toJson(user);
System.out.println(json); // Outputs: {"name":"Alice","age":30}
// Deserialize JSON back to User object
User userFromJson = gson.fromJson(json, User.class);
System.out.println(userFromJson.getName()); // Outputs: Alice
}
}
Why This Matters?
Managing data in JSON format simplifies the integration of different systems. Having a library like Gson can greatly streamline the process.
5. Mockito
What is Mockito?
Mockito is a testing framework for Java that focuses on creating mock objects. This is particularly useful for isolating components during testing, allowing developers to verify interactions with those mocks.
Why Use Mockito?
When dealing with expensive operations (like API calls or database transactions), mocking helps ensure your tests remain fast and reliable.
Example: Using Mockito to Mock a Service
Here’s a brief example of how Mockito can be used in unit tests.
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
public void testGetUser() {
UserService userService = mock(UserService.class);
User user = new User("Bob", 25);
when(userService.getUser(1)).thenReturn(user);
assertEquals("Bob", userService.getUser(1).getName());
}
}
Why This Matters?
Mockito allows you to thoroughly test the interaction between components without relying on their other complex dependencies.
Wrapping Up
While the Java programming language is robust on its own, leveraging extensions and libraries can enhance your development experience and improve your code quality. From reducing boilerplate with Lombok to managing JSON data with Gson, each of these tools provides unique advantages that can empower developers.
Knowing about these extensions not only helps streamline development but can also enhance your overall productivity. As you incorporate these tools into your workflow, you may find yourself writing cleaner, more efficient code at an impressive speed.
Additional Resources
- Lombok Documentation
- Apache Commons Documentation
- JUnit 5 User Guide
- Gson GitHub Repository
- Mockito Official Documentation
In the ever-evolving landscape of technology, staying updated with these tools can help you excel in Java development. Don't hesitate; delve deeper into these libraries and discover how they can benefit you! Happy coding!