How Swallowed Exceptions Can Crash Your Java Application
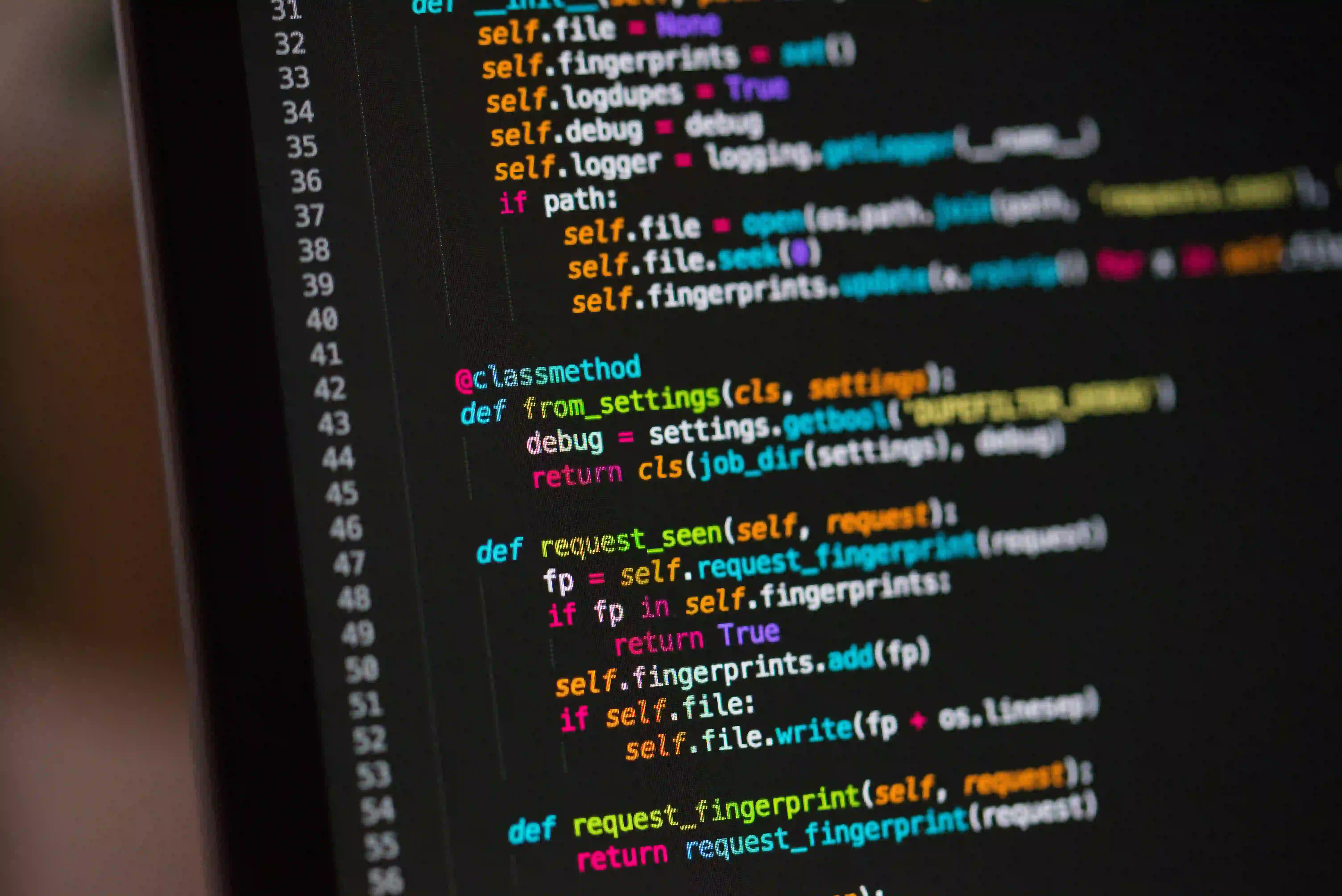
How Swallowed Exceptions Can Crash Your Java Application
In the world of Java programming, handling exceptions is a critical aspect that can determine the robustness and reliability of your applications. However, one often-overlooked pitfall is the phenomenon of "swallowed exceptions." These are exceptions that are caught but not properly handled, leading to silent failures that can eventually crash your application or cause unpredictable behavior.
In this blog post, we will delve into what swallowed exceptions are, how they can affect your application, and best practices to handle them effectively.
Understanding Exceptions in Java
Java is an object-oriented programming language that uses exceptions to signal that something unexpected has happened during the execution of a program. Exceptions are instances of the Throwable
class and can be categorized into two main types:
- Checked Exceptions: These are exceptions that applications should anticipate and handle, such as
IOException
. The compiler forces you to handle these exceptions at compile time. - Unchecked Exceptions: These are runtime exceptions that indicate programming errors, such as
NullPointerException
orArrayIndexOutOfBoundsException
. You aren't required to catch these exceptions, hence they can go unnoticed.
What Are Swallowed Exceptions?
Swallowed exceptions occur when an exception is caught without any action being taken on it. A typical scenario might look like this:
try {
// Potentially problematic code
String str = null;
int length = str.length(); // This will throw a NullPointerException
} catch (NullPointerException e) {
// Exception is caught but ignored
}
In the above code snippet, the NullPointerException
is caught, but nothing is done with that information. This means that any subsequent operations that rely on the value of length
may fail or produce incorrect results without notifying the developer about the underlying issue.
Why Swallowed Exceptions Are Dangerous
-
Silent Failures: An application might continue executing despite having encountered an exception. Consequently, bugs can go undetected, making them difficult to reproduce and fix.
-
Difficulty in Debugging: If an exception is caught and not logged, developers might spend hours or even days trying to isolate the cause of a bug, only to find out it resulted from an unhandled exception.
-
Resource Leaks: When exceptions are swallowed, resources like file handles or network connections might not be released, leading to resource exhaustion or application crashes.
-
User Experience: If exceptions result in silent failures, the end-user might experience unexpected application behaviors without any meaningful feedback from the application, leading to frustration.
Best Practices for Handling Exceptions
- Always Log Caught Exceptions
If you catch an exception, make sure to log it immediately. This provides valuable context when debugging and can help avoid silent failures.
import java.util.logging.Level;
import java.util.logging.Logger;
public class Example {
private static final Logger LOGGER = Logger.getLogger(Example.class.getName());
public void doSomething() {
try {
String str = null;
int length = str.length();
} catch (NullPointerException e) {
LOGGER.log(Level.SEVERE, "A null pointer exception occurred", e);
}
}
}
In this example, if a NullPointerException
occurs, it gets logged with a severity level of SEVERE
, along with the stack trace for further investigation.
- Rethrow Exceptions When Necessary
If you cannot handle an exception appropriately, consider rethrowing it. This allows upstream handlers to take action.
public void processFile(String filename) throws IOException {
try {
// Code that reads from a file
} catch (IOException e) {
// Log but rethrow
LOGGER.log(Level.WARNING, "Failed to process file", e);
throw e; // Rethrow the exception for further handling
}
}
In this case, the exception is neither swallowed nor completely ignored; it is logged and then rethrown for higher-level processing.
- Use Specific Exception Handling
Catch the most specific exceptions first, rather than catching broad exceptions such as Exception
or Throwable
. This helps ensure that you're handling only the exceptions you expect and can handle meaningfully.
try {
// Code that might throw different exceptions
} catch (FileNotFoundException e) {
LOGGER.log(Level.WARNING, "File not found", e);
} catch (IOException e) {
LOGGER.log(Level.SEVERE, "Input/output error occurred", e);
} catch (Exception e) {
LOGGER.log(Level.SEVERE, "An unexpected error occurred", e);
}
By doing this, you can provide more precise error handling and logging.
- Utilize Global Exception Handlers
Frameworks like Spring and Java EE provide global exception handling capabilities. This allows you to centralize exception management and ensures that no exception goes unhandled.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleAllExceptions(Exception ex) {
LOGGER.log(Level.SEVERE, "An unexpected error occurred", ex);
return new ResponseEntity<>("Internal Server Error", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
In this example, all unhandled exceptions are caught at a global level, ensuring that the application provides a consistent error response.
- Conduct Regular Code Reviews
Regular code reviews are an excellent way to ensure that exception handling is robust across your codebase. Encourage team members to question how exceptions are being managed, and assess whether all possible exceptions are being caught and properly addressed.
The Bottom Line
Swallowed exceptions may seem like a trivial issue, but they can lead to resource leaks, silent failures, and a frustrating user experience. By implementing best practices such as logging exceptions, rethrowing them when necessary, using specific exception handling, leveraging global exception handlers, and conducting regular code reviews, you can bolster the reliability of your Java applications.
To dive deeper into Java exception handling, you may refer to the Oracle Java Documentation and consider looking at the Java Best Practices for more insights.
By being vigilant with exception handling and avoiding the pitfalls of swallowed exceptions, you can create more robust, maintainable, and user-friendly applications. Happy coding!