Eliminating Double Negatives for Clearer Code Clarity
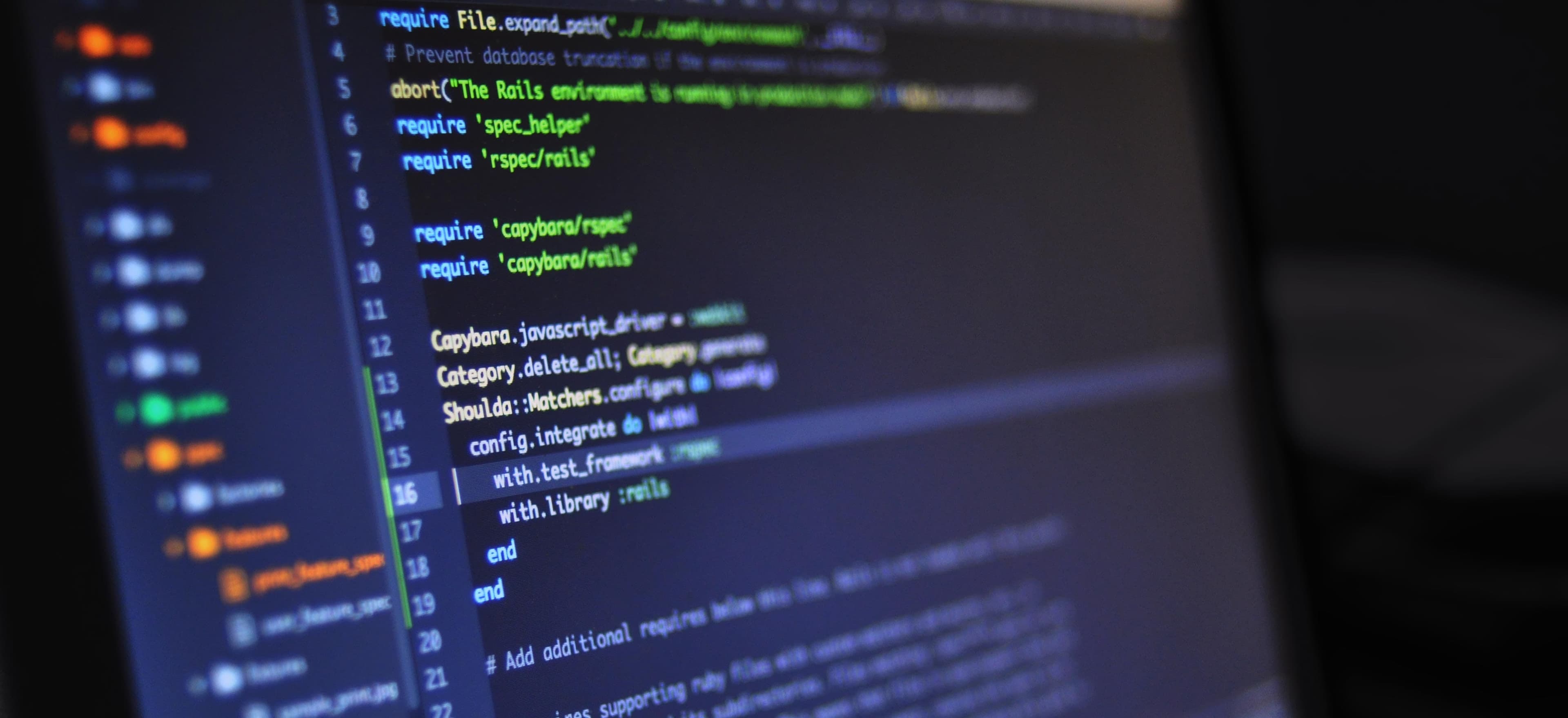
- Published on
Eliminating Double Negatives for Clearer Code Clarity
When programming, clarity is paramount. No one wants to decipher complex logic buried under layers of negations and convoluted expressions. In this blog post, we will delve into the concept of double negatives in Java, explore why they complicate code readability, and provide simple techniques to eliminate them. By the end of this article, you’ll have a clearer understanding of how to write more understandable, maintainable code.
What Are Double Negatives?
In language, a double negative occurs when two forms of negation are used in the same sentence, often leading to confusion. For example, saying "I don't want no ice cream" may lead to an interpretation that contradicts the intended meaning.
In programming, a double negative often surfaces in conditional statements. Consider the following Java example:
if (!isNotValid) {
// processing logic
}
Here, !isNotValid
introduces ambiguity. The reader must first interpret the meaning of isNotValid
and then apply the negation to reach a conclusion about what this condition actually checks.
Why Avoid Double Negatives?
-
Readability: Code is read more often than it is written. The more straightforward your expressions, the easier they are to understand.
-
Maintenance: Code that conveys your intent clearly results in fewer bugs and less confusion during maintenance.
-
Team Collaboration: In a team setting, clear code improves communication. Future developers (or even you, returning to your code after some time) won't need to grapple with dense logic.
-
Efficiency: Cleaner code often leads to more straightforward solutions, which can help with performance and reduce unnecessary complexity.
How to Identify and Remove Double Negatives
Step 1: Simplify Conditional Logic
Start by examining the conditions in your if
statements. Instead of checking for negated states, consider the logical positives. This requires a mental shift, but once you get the hang of it, it becomes second nature.
Consider the original example:
if (!isNotValid) {
// Would execute if isNotValid is false.
}
You can clarify it like so:
if (isValid) {
// processing logic
}
Why the Change? By focusing on isValid
, we eliminate negation and make the condition obvious.
Step 2: Utilize Boolean Flags Effectively
When working with flags, it's easy to accidentally introduce double negatives. If you find yourself doing this, consider revising your naming conventions or restructuring your logic.
Instead of:
boolean isUnderage = age < 18;
if (!isUnderage) {
// They can enter the club.
}
Refactor to:
boolean isOfAge = age >= 18;
if (isOfAge) {
// They can enter the club.
}
Why This Matters? The switch makes the intent crystal clear—the reader knows immediately what condition must be true for the logic that follows.
Step 3: Use Ternary Operators for Simplified Expressions
Sometimes, a double negative can emerge from overly complex expressions. Ternary operators can help deconstruct these complicated conditions into more manageable parts.
For instance, if you have:
String status = isNotValid ? "Invalid" : "Valid";
if (status.equals("Valid")) {
// proceed with logic
}
Change it to eliminate negation:
String status = isValid ? "Valid" : "Invalid";
if (status.equals("Valid")) {
// proceed with logic
}
Why Ternary? Here, we directly check for the valid status without the need for negation, maintaining code clarity.
Step 4: Refactor Current Codes
As an exercise, review your existing codebase for instances of double negatives. This may seem like an arduous task, but the results are well worth the effort. Refactoring will help not just with negations but also improve the overall readability and structure of your code.
Example for Reflection
Consider the following example that contains multiple layers of negation:
if (!(userIsNotLoggedIn || isAccountLocked)) {
// Allow access
}
Refactor it like this:
if (userIsLoggedIn && !isAccountLocked) {
// Allow access
}
Here, we clarify intent. The logic is easier to follow, and the checks are separated into clean, understandable conditions.
To Wrap Things Up
Writing clear, maintainable code is essential for both personal development and effective team collaboration. By eliminating double negatives, you improve your code's readability and clarity, creating a more effective programming environment for yourself and others.
As a best practice, always strive to write conditions and constructs that directly reflect the logic you wish to implement. This not only aids in understanding but also helps prevent bugs caused by misinterpretation.
You can find more resources on clean coding practices in Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin.
Remember, clarity is the hallmark of great code. So take that extra minute to structure your logic clearly, and you’ll save considerable time (and headaches) down the line.
Happy coding!