Overcoming Performance Bottlenecks in JPA Implementations
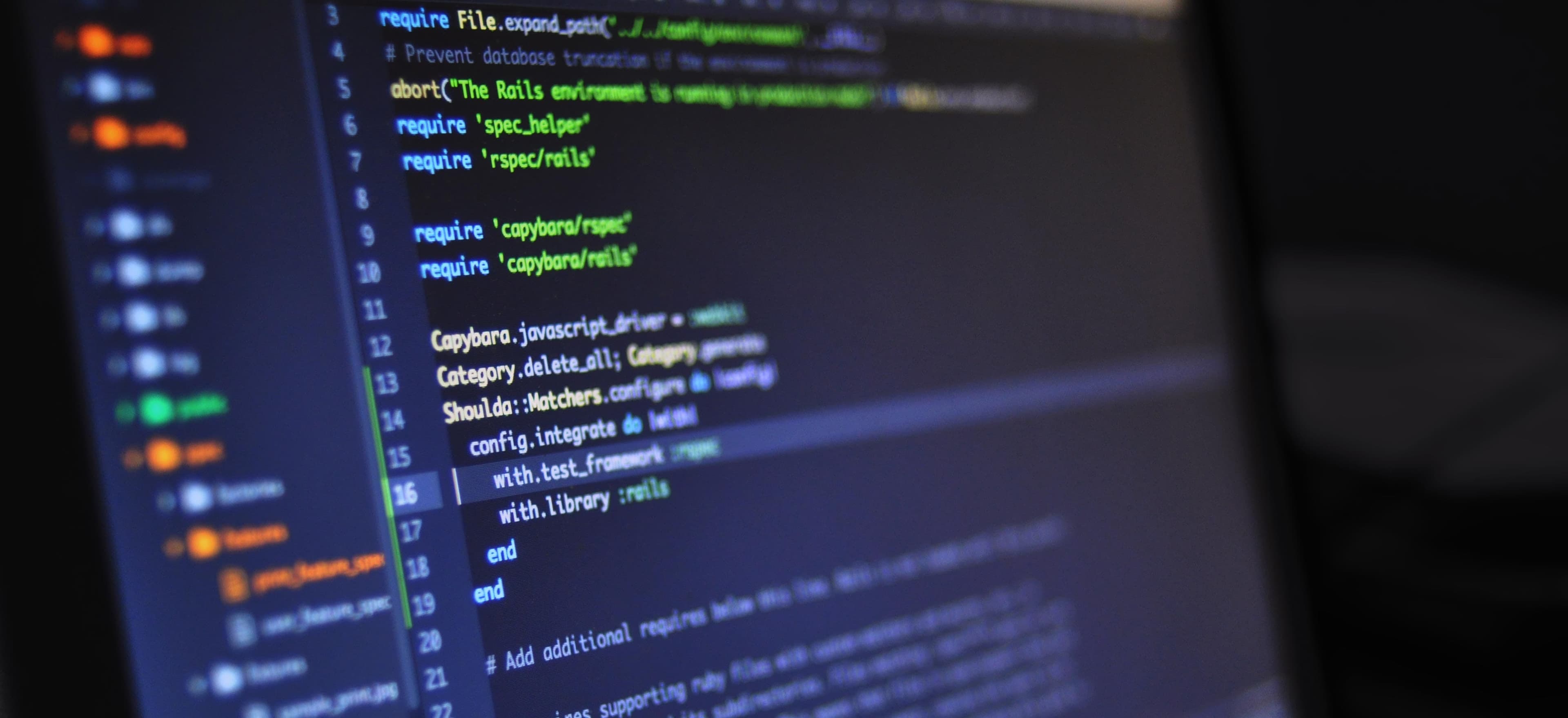
- Published on
Overcoming Performance Bottlenecks in JPA Implementations
The Java Persistence API (JPA) is a powerful framework for managing relational data in Java applications. While JPA simplifies database interactions, it can sometimes lead to performance issues, especially in complex applications. Understanding and overcoming these performance bottlenecks is crucial for developing efficient, scalable systems. In this post, we will explore common performance pitfalls in JPA implementations and offer practical strategies to mitigate them.
Understanding JPA and Its Common Pitfalls
JPA provides a specification for object-relational mapping that allows developers to interact with databases using Java objects. While it abstracts much of the complexity of SQL, developers may encounter performance bottlenecks due to several factors:
- Inefficient Queries: JPA generates SQL under the hood. Poorly structured queries can slow down application performance.
- N+1 Select Problem: This occurs when JPA fetches one record and then queries the database additional times for related records.
- Improper Use of Fetch Types: Utilizing the wrong fetch strategy can result in either too many database calls (if using LAZY) or loading too much data (if using EAGER).
- Transaction Management Issues: Inefficient transaction boundaries may lead to excessive locking and resource contention.
Let's delve into strategies for overcoming these bottlenecks.
1. Optimize Your Queries
Annotations and JPQL
Ensure that your JPA queries are efficient. Begin by reviewing the methods in your repositories. Implement the @Query
annotation to optimize your JPQL (Java Persistence Query Language) queries.
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.status = ?1")
List<User> findByStatus(String status);
}
Why this matters: Direct JPQL queries can be more optimized compared to method queries, allowing you to define exactly what data you need.
Use Projections
If your application deals with large entities, consider using projections. Projections allow you to retrieve only the necessary fields rather than the entire object.
public interface UserProjection {
String getName();
String getEmail();
}
public interface UserRepository extends JpaRepository<User, Long> {
List<UserProjection> findAllBy();
}
Why this matters: Reducing data transfer minimizes memory overhead and speeds up response times, especially beneficial in large-scale applications.
2. Avoid the N+1 Select Problem
Batch Fetching
The N+1 selects problem can be addressed by using batch fetching strategies. By configuring JPA to load related data in batches, you can significantly reduce the number of database round-trips.
@Entity
public class Order {
// ...
@OneToMany(fetch = FetchType.LAZY)
@BatchSize(size = 10)
private List<Item> items;
}
Why this matters: Instead of executing multiple queries, setting a batch size consolidates them into fewer database calls, improving overall performance.
3. Fetch Strategies with Care
EAGER vs. LAZY Fetching
Choosing the correct fetch type is critical for performance. EAGER loading fetches all related entities immediately, whereas LAZY loads them only when accessed.
@Entity
public class User {
@OneToMany(fetch = FetchType.LAZY)
private List<Post> posts; // Lazy loading to avoid loading posts when not needed
}
Why this matters: Overusing EAGER fetching can lead to excessive memory usage and longer loading times. Conversely, improper lazy loading can lead to the N+1 select problem.
4. Optimize Your Transactions
Define Transaction Boundaries
Set clear transaction boundaries to optimize performance. Utilize the @Transactional
annotation effectively.
@Service
public class UserService {
@Transactional
public void updateUser(User user) {
userRepository.save(user);
}
}
Why this matters: Defining boundaries reduces the locking overhead, allowing for smoother operations and less contention.
Propagation and Isolation Levels
Understand the implications of different transaction propagation and isolation levels, and adjust them according to your needs. Lower isolation levels can reduce contention.
@Transactional(propagation = Propagation.REQUIRES_NEW)
public void saveOrUpdate(User user) {
// This method will run in a new transaction
}
Why this matters: Fine-tuning these settings allows for optimizing performance based on the specific requirements of your operations.
5. Cache Strategies
Second-Level Cache
Implementing a second-level cache can significantly reduce database hits. JPA providers like Hibernate offer built-in caching capabilities.
<property name="hibernate.cache.use_second_level_cache" value="true" />
<property name="hibernate.cache.region.factory_class" value="org.hibernate.cache.ehcache.EhCacheRegionFactory" />
Why this matters: Caching frequently accessed data can lead to substantial performance improvements by serving data from memory instead of hitting the database.
Query Cache
Utilize the query cache to cache the results of frequently executed queries.
@Cacheable
public List<User> findByStatus(String status) {
return entityManager.createQuery("SELECT u FROM User u WHERE u.status = :status")
.setParameter("status", status)
.getResultList();
}
Why this matters: Query caching allows repetitive data requests to be served faster by avoiding direct database access.
Final Thoughts
By leveraging the strategies outlined above, Java developers can effectively overcome performance bottlenecks in JPA implementations. Understanding query optimization, fetching strategies, transaction management, and caching mechanisms are essential in developing efficient applications.
For more information on JPA performance tuning, consider reading the following resources:
- Hibernate Performance Tuning
- Java EE 8 Tutorial
By applying these techniques judiciously, you will ensure that your JPA implementation performs optimally, scales effectively, and provides a seamless experience for users. Happy coding!