Understanding Lucene Components: Overcoming Common Pitfalls
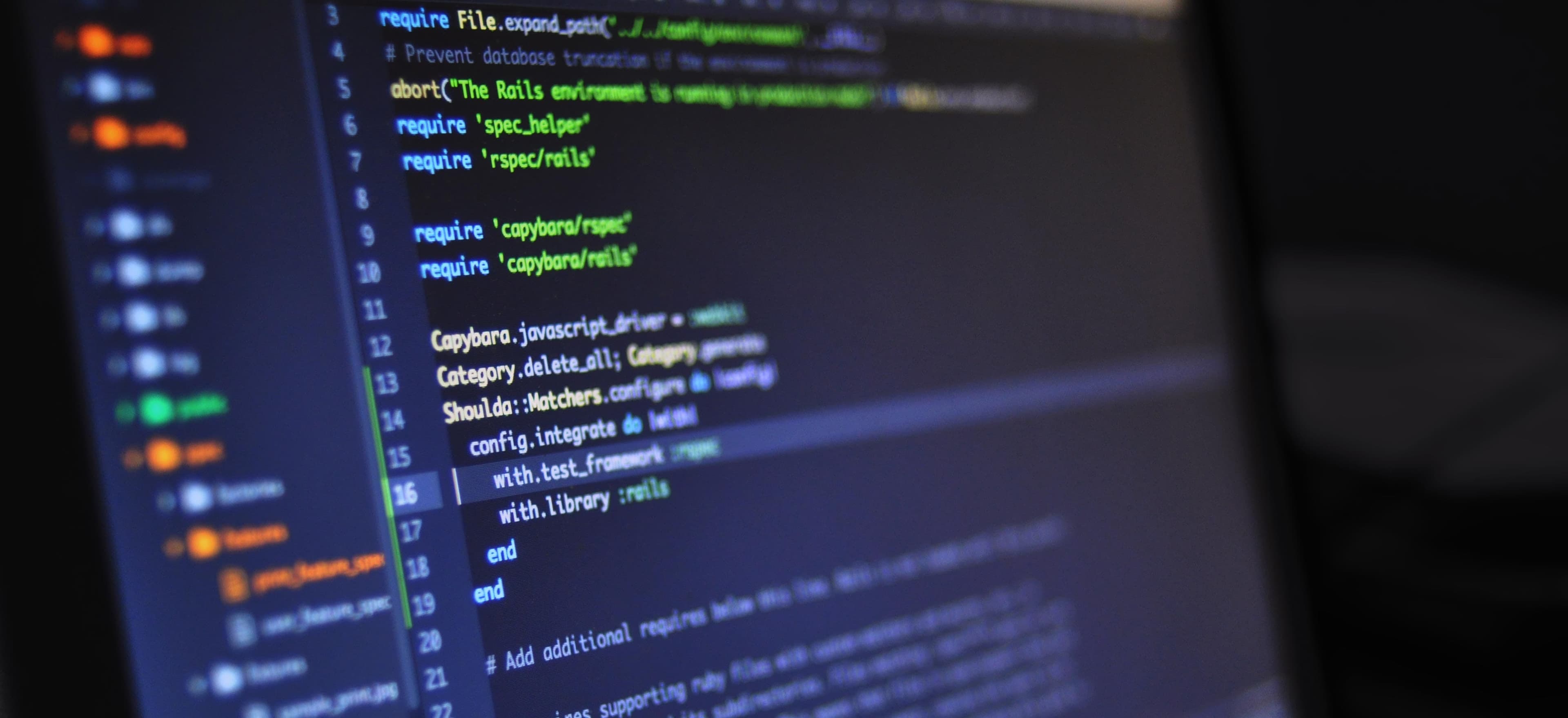
- Published on
Understanding Lucene Components: Overcoming Common Pitfalls
Apache Lucene is a powerful library made for full-text searching and text indexing. It is widely used in applications requiring complex search capabilities, like Elasticsearch and Apache Solr. Despite its robust features, many developers encounter common pitfalls while implementing Lucene. This blog post aims to demystify Lucene components and guide you in overcoming these challenges.
What is Apache Lucene?
Apache Lucene is a highly efficient search engine library written in Java. It offers indexing and searching capabilities for various types of documents, making it a go-to choice for developers who require fast and accurate text retrieval.
Core Components of Lucene
Lucene is built upon several essential components:
- Indexing
- Searching
- Document and Field Representation
- Analyzers
- Query parsers
Each of these components plays a crucial role in building a functional and efficient search engine.
1. Indexing
Indexing is the process of converting data into a searchable format. Lucene creates an inverted index for efficient retrieval of information. Below is a basic example of creating an index.
import org.apache.lucene.document.Document;
import org.apache.lucene.document.Field;
import org.apache.lucene.document.StringField;
import org.apache.lucene.index.IndexWriter;
import org.apache.lucene.index.IndexWriterConfig;
import org.apache.lucene.store.Directory;
import org.apache.lucene.store.RAMDirectory;
import org.apache.lucene.analysis.standard.StandardAnalyzer;
public class IndexCreator {
public static void main(String[] args) {
// Create an in-memory index
try (Directory index = new RAMDirectory()) {
StandardAnalyzer analyzer = new StandardAnalyzer();
IndexWriterConfig config = new IndexWriterConfig(analyzer);
IndexWriter writer = new IndexWriter(index, config);
addDoc(writer, "Lucene in Action", "1939");
addDoc(writer, "Lucene for Dummies", "2005");
addDoc(writer, "Managing Gigabytes", "1992");
writer.close();
} catch (Exception e) {
e.printStackTrace();
}
}
private static void addDoc(IndexWriter writer, String title, String value) throws IOException {
Document doc = new Document();
doc.add(new StringField("title", title, Field.Store.YES));
doc.add(new StringField("year", value, Field.Store.YES));
writer.addDocument(doc);
}
}
In this example, we create an in-memory index and add documents to it. Each document is composed of fields (like title and year) represented by StringField
. The Field.Store.YES
argument ensures that the field values are stored and retrievable during searches.
Common Pitfall in Indexing
A common mistake during indexing is neglecting to optimize the indexing process. Failing to use batching can lead to performance issues. Always consider using bulk indexing, especially for large datasets.
2. Searching
Once indexed, the data can be searched efficiently. Lucene provides a powerful search operation utilizing IndexSearcher
and Query
.
import org.apache.lucene.analysis.standard.StandardAnalyzer;
import org.apache.lucene.queryparser.classic.QueryParser;
import org.apache.lucene.search.IndexSearcher;
import org.apache.lucene.search.Query;
import org.apache.lucene.search.TopDocs;
public class Searcher {
private IndexSearcher searcher;
public Searcher(IndexSearcher searcher) {
this.searcher = searcher;
}
public void search(String queryString) throws Exception {
QueryParser parser = new QueryParser("title", new StandardAnalyzer());
Query query = parser.parse(queryString);
TopDocs results = searcher.search(query, 10);
// Process results...
}
}
In this snippet, QueryParser
dynamically constructs queries based on user input, making it easier to search through indexed documents.
Common Pitfall in Searching
A frequent mistake is failing to handle special characters in queries. Users may inadvertently include characters that have special meanings in Lucene's query language, causing the search to fail. Always sanitize and escape user input before parsing.
3. Document and Field Representation
In Lucene, documents are collections of fields. Each field can be of different data types. This flexibility allows for rich, nuanced searches.
import org.apache.lucene.document.TextField;
import org.apache.lucene.document.FieldType;
import org.apache.lucene.document.Field;
FieldType type = new FieldType();
type.setIndexOptions(IndexOptions.DOCS_AND_FREQS_AND_POSITIONS);
type.setStored(true);
Field field = new Field("content", "Text here", type);
Common Pitfall with Document Representation
Confusion can arise if developers aren't careful about field types. For instance, using TextField
for fields that should not be tokenized or analyzed can lead to unexpected search results. Choose appropriate types such as StringField
for exact matches or TextField
for full-text search.
4. Analyzers
Analyzers process text during both indexing and searching. This includes tokenization, lowercasing, and stemming. For example, using StandardAnalyzer
helps convert input text into tokens suitable for searching.
import org.apache.lucene.analysis.standard.StandardAnalyzer;
import org.apache.lucene.analysis.TokenStream;
import org.apache.lucene.analysis.tokenattributes.CharTermAttribute;
public void analyzeText(String text) throws IOException {
StandardAnalyzer analyzer = new StandardAnalyzer();
TokenStream tokenStream = analyzer.tokenStream("field", text);
CharTermAttribute attr = tokenStream.addAttribute(CharTermAttribute.class);
tokenStream.reset(); // Resets the stream to the beginning
while (tokenStream.incrementToken()) {
System.out.println(attr.toString());
}
tokenStream.close();
}
Common Pitfall with Analyzers
One of the common errors involves inconsistency in analyzers during indexing and searching. Always use the same analyzer for both processes to ensure the tokenization of terms matches.
5. Query Parsers
Query parsers allow the conversion of string queries into Lucene queries. They support a wide array of features, including wildcards, fuzzy searches, and range queries.
QueryParser parser = new QueryParser("content", new StandardAnalyzer());
Query query = parser.parse("title:Lucene AND year:[2000 TO 2022]");
// Execute your query against the searcher...
Common Pitfall with Query Parsers
Developers often misconfigure the fields while creating queries. Always double-check the field names used in the query against your index schema to avoid FieldNotFoundException
.
Closing Remarks
Navigating the complexities of Apache Lucene can be daunting. However, understanding its core components and avoiding common pitfalls will significantly enhance your development process. Lucene is a powerful tool for creating efficient search capabilities but requires careful attention to indexing, searching, document representation, analyzers, and query parsing.
For more details, you can explore Apache Lucene's official documentation. Embrace the power of this library, and optimize your search applications effectively!
Further Reading
- Elasticsearch: The Definitive Guide
- Apache Solr Reference Guide
- Lucene in Action by Doug Cutting & Michael McCandless
Feel free to reach out or leave a comment if you have questions or additional insights on using Lucene in your projects!