Why Passing Streams Beats Using Lists for Data Processing
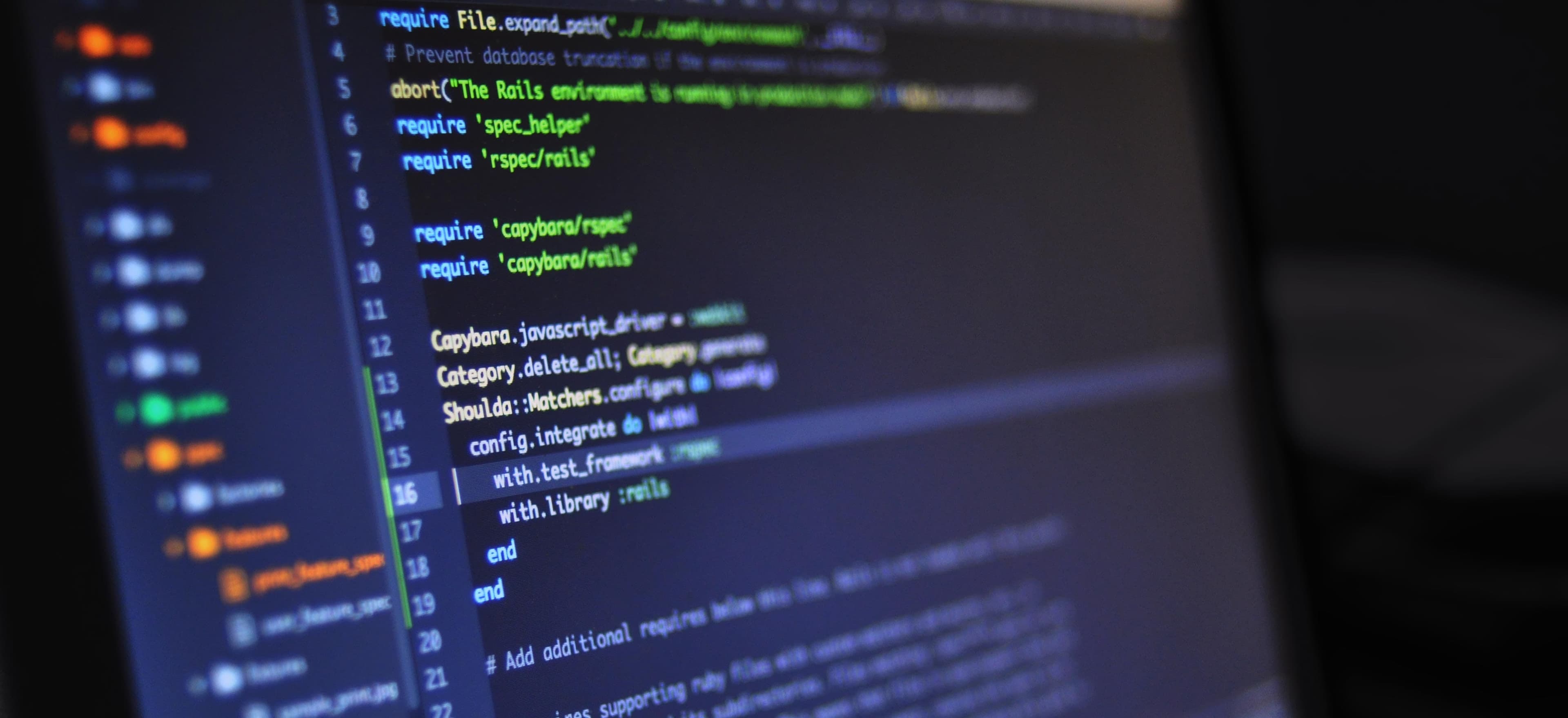
- Published on
Why Passing Streams Beats Using Lists for Data Processing
In the world of Java programming, data processing is a common task where developers often manipulate collections of data. Traditionally, developers have relied on lists to store and manage these collections. However, the introduction of Streams in Java 8 revolutionized the way we approach data processing. In this blog post, we'll explore the advantages of using Streams over Lists, examining their efficiency, readability, and capabilities.
The Basics of Streams
Before diving into their advantages, let’s clarify what Streams are. A Stream is a sequence of elements supporting sequential and parallel aggregate operations. Streams are not data structures. Instead, they are a view of a sequence of data as it is being processed.
Here's a simple example demonstrating creation of a Stream from a List:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Stream;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Creating a stream from a list
Stream<String> nameStream = names.stream();
// Printing the names using the stream
nameStream.forEach(System.out::println);
}
}
In this snippet, we converted a List of names into a Stream and used forEach
to print each name. Streams allow operations to be chained together, leading to cleaner and more expressive code.
Efficiency: Streams vs. Lists
One of the most significant benefits of Streams is efficiency. Let’s compare the performance implications of using Lists versus Streams:
Lazy Evaluation
Streams employ lazy evaluation, meaning that computation is not performed until results are needed. This can lead to significant performance improvements.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Example of lazy evaluation
names.stream()
.filter(name -> {
System.out.println("Filtering " + name);
return name.startsWith("A");
})
.collect(Collectors.toList());
In the above code, filtering occurs only when the terminal operation collect
is invoked. Until that point, no filtering happens.
Parallel Processing
Streams can process data in parallel effortlessly, harnessing the power of multi-core processors. Here’s how we can transform our previous example to run in parallel:
names.parallelStream()
.filter(name -> name.startsWith("A"))
.forEach(System.out::println);
This parallelStream
allows for concurrent processing, potentially leading to faster execution compared to iterating through a List sequentially.
Readability and Maintainability
Another compelling reason to use Streams over Lists is improved readability. Streams provide a fluent API that is often more intuitive.
Chaining Operations
With Lists, you often create multiple interim variables and write multiple lines of code to get the desired result. Streams provide a way to chain operations together, reducing boilerplate code.
Here’s an example that filters and collects names starting with 'A' in two different approaches:
Using List:
List<String> filteredNames = new ArrayList<>();
for (String name : names) {
if (name.startsWith("A")) {
filteredNames.add(name);
}
}
Using Streams:
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
The Stream version is more concise and easier to understand at a glance, contributing to better maintainability of your code.
Advanced Functional Operations
Streams support a range of functional operations, including map, reduce, and flatMap, enabling developers to perform complex data transformations easily.
Mapping Data
Consider an example where we have a list of names, and we want to retrieve their lengths:
List<String> lengths = names.stream()
.map(String::length)
.collect(Collectors.toList());
In this case, we transformed a List of names into a List of their lengths with a single, expressive line of code.
Reducing Data
The reduce
operation allows you to combine elements in a Stream into a single result. For instance, summing lengths of all names:
int totalLength = names.stream()
.map(String::length)
.reduce(0, Integer::sum);
This demonstrates how Streams can facilitate complex functional operations while keeping the code in a clean and readable format.
Java Streams vs. Other Languages
It's worth mentioning that the concept of Streams is not unique to Java. Many modern programming languages, such as Python (with generators) and JavaScript (with iterables), have similar abstractions. However, Java's implementation is particularly robust, integrating well within its object-oriented framework.
In Conclusion, Here is What Matters
Streams introduce a new paradigm for processing data in Java that surpasses the conventional List operations in multiple domains. By embracing Streams, we enhance the efficiency of our applications, improve code readability, and gain access to sophisticated functional operations.
While using Lists is still valid for many simple cases, the power of Streams cannot be ignored in a world where clean, efficient, and maintainable code is necessary. Start leveraging Streams in your projects today!
For further reading on Java Streams, you may want to explore the official Java documentation on Streams.
Happy Coding!
Checkout our other articles