Mastering EJB Interceptors: Common Pitfalls to Avoid
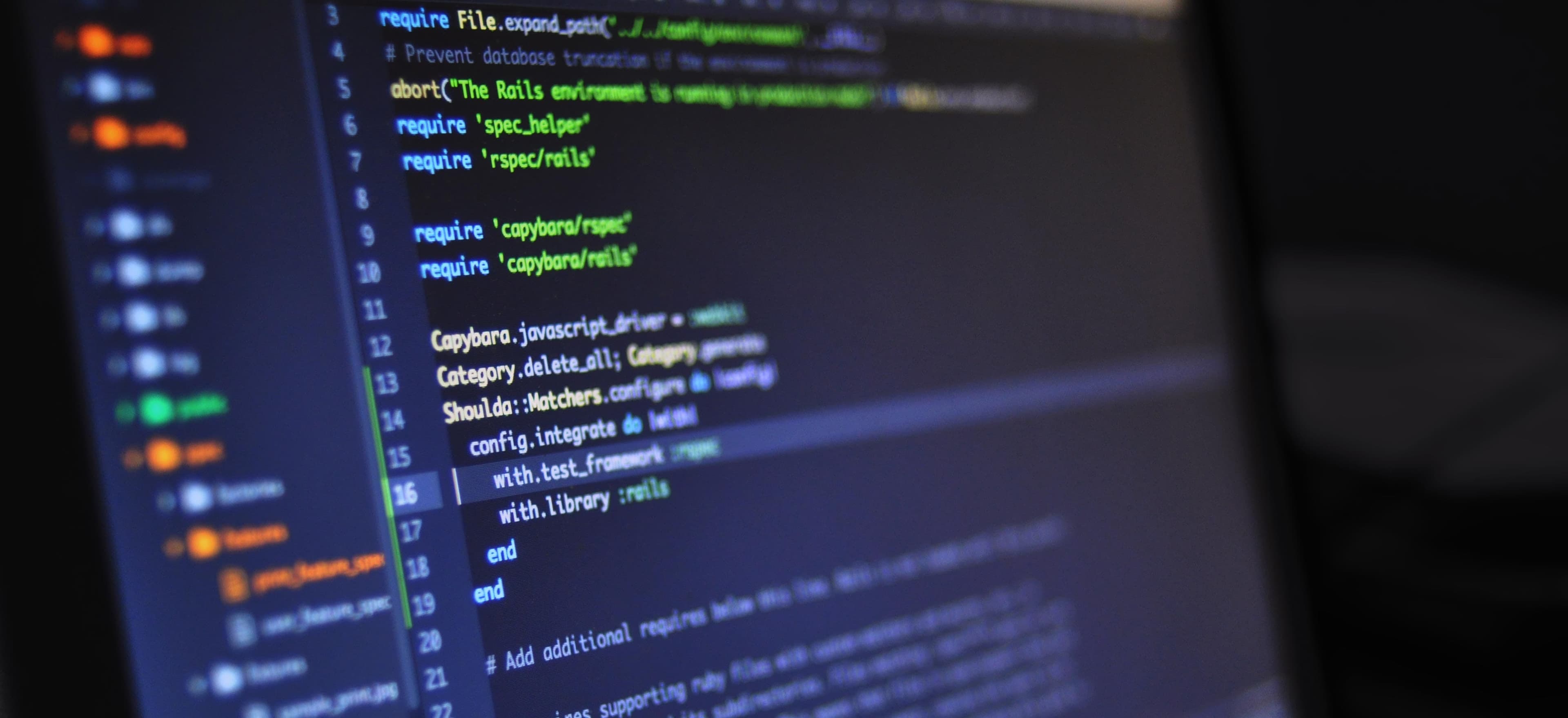
- Published on
Mastering EJB Interceptors: Common Pitfalls to Avoid
Enterprise JavaBeans (EJB) is a crucial part of the Java EE (Jakarta EE) platform, providing a robust backend architecture for building scalable and distributed applications. One important feature within EJB is the use of interceptors. Interceptors provide a powerful mechanism for decoupling cross-cutting concerns from business logic, but they also introduce potential pitfalls that developers should be aware of.
In this post, we will explore EJB interceptors, their benefits, and some common pitfalls that developers often encounter. We aim to equip you with the knowledge you need to leverage EJB interceptors effectively while avoiding common mistakes.
What Are EJB Interceptors?
EJB interceptors are a way to apply additional logic before or after the execution of business methods in an EJB component. They act as a layer that can wrap around business method calls, allowing you to handle concerns such as logging, transaction management, security, and performance monitoring without cluttering your business logic.
Benefits of EJB Interceptors
- Separation of Concerns: By using interceptors, you can keep your business logic clean and focused while handling cross-cutting concerns separately.
- Reusability: Interceptor methods can be reused across different beans, promoting a DRY (Don't Repeat Yourself) principle.
- Configurability: EJB interceptors can be easily configured at runtime, allowing flexible application logic.
Example: Basic EJB Interceptor
Here’s a simple example of creating and using an EJB interceptor:
import javax.interceptor.AroundInvoke;
import javax.interceptor.Interceptor;
import javax.interceptor.InvocationContext;
@Interceptor
public class LoggingInterceptor {
@AroundInvoke
public Object logMethodAccess(InvocationContext ctx) throws Exception {
System.out.println("Entering method: " + ctx.getMethod().getName());
try {
return ctx.proceed();
} finally {
System.out.println("Exiting method: " + ctx.getMethod().getName());
}
}
}
Explanation of the Code
- The
@Interceptor
annotation marks the class as an interceptor. - The
@AroundInvoke
annotation tells the EJB container thatlogMethodAccess()
should be executed before and after the target method. - The
InvocationContext
object provides access to the method being called and allows you to proceed with the original method call viactx.proceed()
.
Common Pitfalls with EJB Interceptors
While EJB interceptors offer many advantages, developers must navigate several common pitfalls to avoid issues. Below are some of those pitfalls, along with tips on how to prevent them.
1. Order of Invocation
One common mistake developers make is not understanding the order in which multiple interceptors are invoked. Interceptors are called in the order they are declared, which can lead to unexpected behaviors if one interceptor depends on another.
How to Avoid This Pitfall
To control the order of interceptor execution, make sure to explicitly define the ordering using @Interceptors
annotation.
@Interceptors({LoggingInterceptor.class, SecurityInterceptor.class})
public class MyEJB {
public void myBusinessMethod() {
// business logic here
}
}
2. Insufficient Context Handling
Interceptors can easily lead to context-related errors if they do not correctly propagate context information. Forgetting to pass the invocation context or not managing the state properly can result in unexpected behavior.
How to Avoid This Pitfall
Always ensure that ctx.proceed()
is called within a try-catch
block and handle exceptions correctly.
@AroundInvoke
public Object logMethodAccess(InvocationContext ctx) {
try {
return ctx.proceed(); // Proceed with the method
} catch (Exception e) {
// Handle exception gracefully
System.out.println("Error in method: " + ctx.getMethod().getName());
throw e; // Rethrow if needed
}
}
3. Interceptor Instantiation Issues
Another common issue is the misunderstanding of how interceptors are instantiated. Interceptors are managed by the EJB container, and it is essential not to create them manually, as doing so can lead to lifecycle management issues.
How to Avoid This Pitfall
Always let the EJB container handle the instantiation of interceptors. This ensures that the interceptor lifecycle is managed along with your EJBs.
4. Excessive Implementation of Cross-Cutting Concerns
While interceptors are great for separating cross-cutting concerns, it is crucial not to abuse them. Overloading interceptors with too many responsibilities can make debugging complex and reduce performance.
How to Avoid This Pitfall
Keep your interceptors focused on a single responsibility. If you find that an interceptor is handling too many different concerns, consider splitting it up into multiple, smaller interceptors.
5. Ignoring Transaction Management
When using interceptors, it's vital to understand the transaction boundaries. Failing to consider transactions can lead to data inconsistencies or even deadlocks.
How to Avoid This Pitfall
When managing transactions in interceptors, make sure you are aware of the context in which your business method operates. You may want to check if the current method should initiate or propagate a transaction.
import javax.annotation.Resource;
import javax.transaction.UserTransaction;
// Example of Transaction Management
@AroundInvoke
public Object manageTransaction(InvocationContext ctx) throws Exception {
UserTransaction transaction = ... // Obtain UserTransaction
try {
transaction.begin();
Object result = ctx.proceed();
transaction.commit();
return result;
} catch (Exception e) {
transaction.rollback();
throw e;
}
}
Closing Remarks
EJB interceptors are a powerful tool that can greatly enhance the design and implementation of enterprise applications. However, as with any feature, they come with potential pitfalls that can impact the functionality and maintainability of your code.
By understanding these common pitfalls and employing best practices, you can maximize the benefits of EJB interceptors while avoiding common mistakes. Remember, success in software development often comes down to making informed decisions and understanding the underlying behavior of the tools at your disposal.
By embracing the principles outlined in this article, you will be on your way to mastering EJB interceptors, leading to cleaner, more maintainable code that effectively addresses cross-cutting concerns in your applications.
For further reading on Java EE and EJB, you might find these resources helpful:
- Oracle's EJB Documentation
- Jakarta EE Specifications
- Design Patterns in Java EE
Feel free to share your experiences with EJB interceptors or any challenges you've faced in the comments below!
Checkout our other articles