Common Pitfalls in Building RESTful Web Services
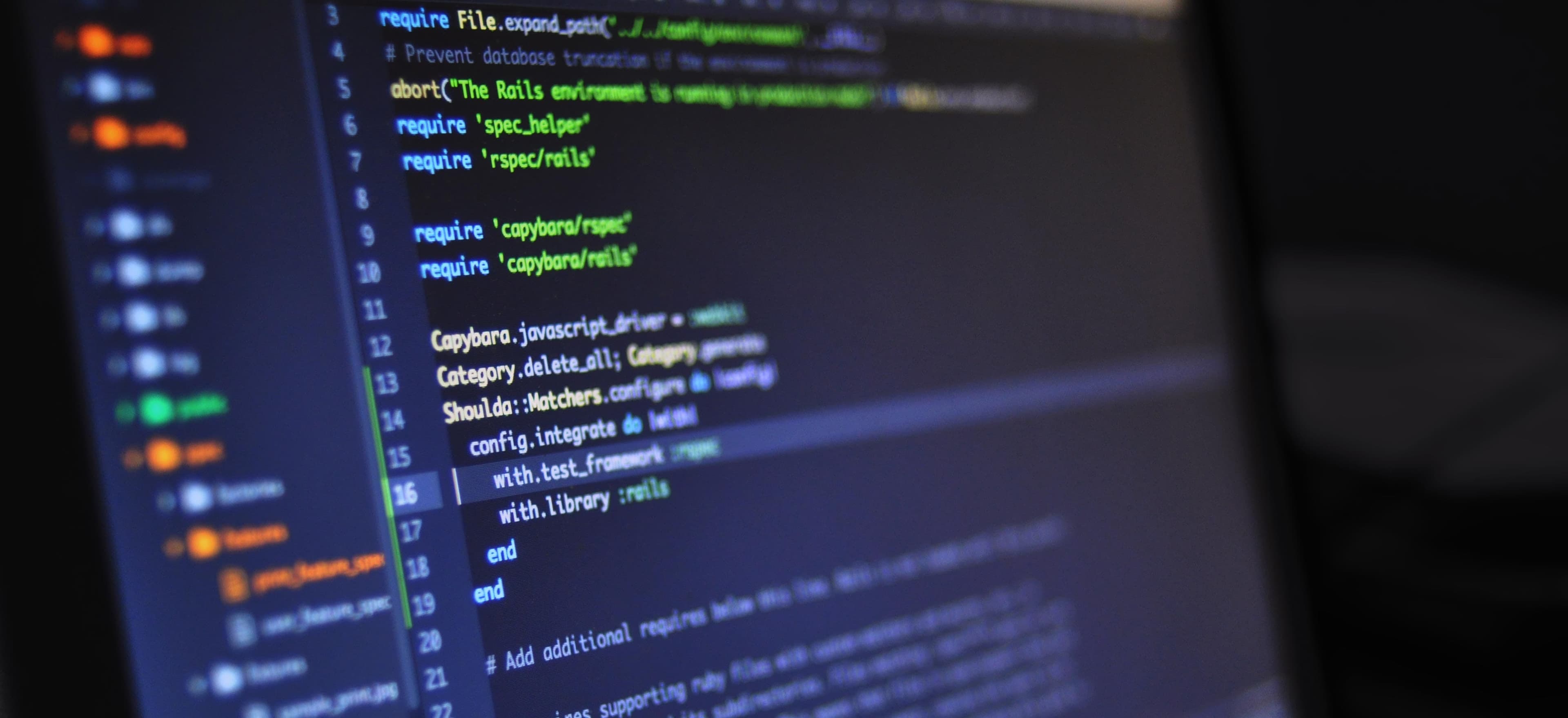
- Published on
Common Pitfalls in Building RESTful Web Services
Building RESTful web services is a well-established practice in software development, allowing applications to communicate over the internet using standard HTTP protocols. However, despite the simplicity of the concept, developers often stumble upon various challenges and pitfalls while implementing them. This blog aims to explore these common pitfalls and provide insights on how to avoid them.
Understanding REST Architecture
Before diving into the pitfalls, let's clarify what REST (Representational State Transfer) is. It is an architectural style that relies on stateless, client-server interactions using standard HTTP methods like GET, POST, PUT, DELETE, etc. By adhering to the principles of REST, we ensure scalability, performance, and usability in API design.
1. Overusing HTTP Methods
Problem:
One of the common pitfalls is misusing HTTP methods. Developers often tend to utilize the wrong method, which can confuse the intended action of a given request.
Solution:
- GET: Use for retrieval of data without side effects.
- POST: Use for creation of resources or operations that result in a change on the server.
- PUT: Use for updating an existing resource or creating a resource if it does not exist.
- DELETE: Use for deleting resources.
Example:
// Retrieve an existing resource using GET
@GetMapping("/users/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userService.findById(id);
if (user == null) {
return ResponseEntity.notFound().build();
}
return ResponseEntity.ok(user);
}
In this code snippet, we correctly use the GET method to retrieve a user by their ID. Misusing HTTP methods could lead to unexpected behaviors and poor API usability.
2. Ignoring Versioning
Problem:
APIs evolve over time, and failing to version them means that changes could break the client applications that heavily rely on specific features or outputs.
Solution:
Always version your REST APIs from the beginning. A common approach is to include the version in the URL.
Example:
@RestController
@RequestMapping("/api/v1/users")
public class UserController {
// Controller methods...
}
Adding "v1" to the URL allows you to introduce future versions, e.g., "v2", without breaking existing clients.
3. Not Handling Errors Properly
Problem:
Another common oversight is improper error handling. Developers sometimes assume that returning HTTP status codes is enough, thus neglecting detailed error messages.
Solution:
Always provide meaningful error messages along with the corresponding HTTP status codes.
Example:
@PostMapping("/users")
public ResponseEntity<?> createUser(@RequestBody User user) {
try {
User createdUser = userService.create(user);
return ResponseEntity.status(HttpStatus.CREATED).body(createdUser);
} catch (UserAlreadyExistsException e) {
return ResponseEntity.status(HttpStatus.CONFLICT)
.body("User already exists: " + e.getMessage());
}
}
In this example, we not only send back a status code but also a body containing an error message, making it easier for clients to understand the issue.
4. Lack of Appropriate Documentation
Problem:
Good documentation is critical for your RESTful API. Without it, your API may go unused or become a burden to clients trying to figure out how to interact with it.
Solution:
Use tools such as Swagger or OpenAPI for automatic documentation generation.
Example:
By annotating your code with OpenAPI annotations, you can generate a dynamic webpage that documents your API.
/**
* @swagger
* /users:
* get:
* summary: Get all users
* responses:
* 200:
* description: A list of users
*/
@GetMapping("/users")
public List<User> getAllUsers() {
return userService.getAllUsers();
}
This way, clients can easily refer to the documentation to understand how to interact with your service.
5. Forgetting to Implement Security
Problem:
Implementing security measures like authentication and authorization are often overlooked, leaving APIs vulnerable to attacks.
Solution:
Always use HTTPS and implement authentication mechanisms such as OAuth 2.0, JWT (JSON Web Tokens), or Basic Authentication.
Example:
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping("/secure")
@PreAuthorize("hasRole('USER')")
public ResponseEntity<String> secureEndpoint() {
return ResponseEntity.ok("This is a secure endpoint");
}
}
In this code snippet, we utilized Spring Security's @PreAuthorize
to secure access to the endpoint, allowing only users with 'USER' roles. For more in-depth information on securing REST APIs, check out this Spring Security Guide.
6. Not Using HATEOAS
Problem:
Failing to implement HATEOAS (Hypermedia As The Engine Of Application State) means that your API can't guide clients about available actions dynamically.
Solution:
Make your API self-descriptive. This allows clients to discover the possibilities of your API without prior knowledge of its structure.
Example:
@GetMapping("/users/{id}")
public EntityModel<User> getUserById(@PathVariable Long id) {
User user = userService.findById(id);
EntityModel<User> resource = EntityModel.of(user);
resource.add(linkTo(methodOn(UserController.class).getUserById(id)).withSelfRel());
return resource;
}
This EntityModel
wraps the user, and we add a self-link demonstrating how clients can understand the relationships and available operations.
To Wrap Things Up
Building and maintaining a RESTful web service poses various challenges. However, by acknowledging these common pitfalls—like misusing HTTP methods, neglecting versioning, improperly handling errors, lacking documentation, ignoring security, and skipping HATEOAS—we can build robust, secure, and user-friendly APIs.
By following best practices and understanding the importance of each decision made during the design and implementation process, we can create a RESTful API that stands the test of time and serves its intended purpose efficiently. As RESTful services continue to play a vital role in web development, avoiding these pitfalls will undoubtedly lead to more effective and enjoyable projects.
Further Reading
For those who want to delve deeper into RESTful API design principles, consider reading the RESTful Web Services book by Leonard Richardson and Sam Ruby.
If you're interested in learning more about security practices for RESTful APIs, check out the comprehensive article on securing REST APIs on OWASP.
By adopting these principles and understanding potential pitfalls, developers will significantly improve the quality and usability of their RESTful web services.
Happy coding!
Checkout our other articles