Creating Engaging Callouts with JavaFX Animated Effects
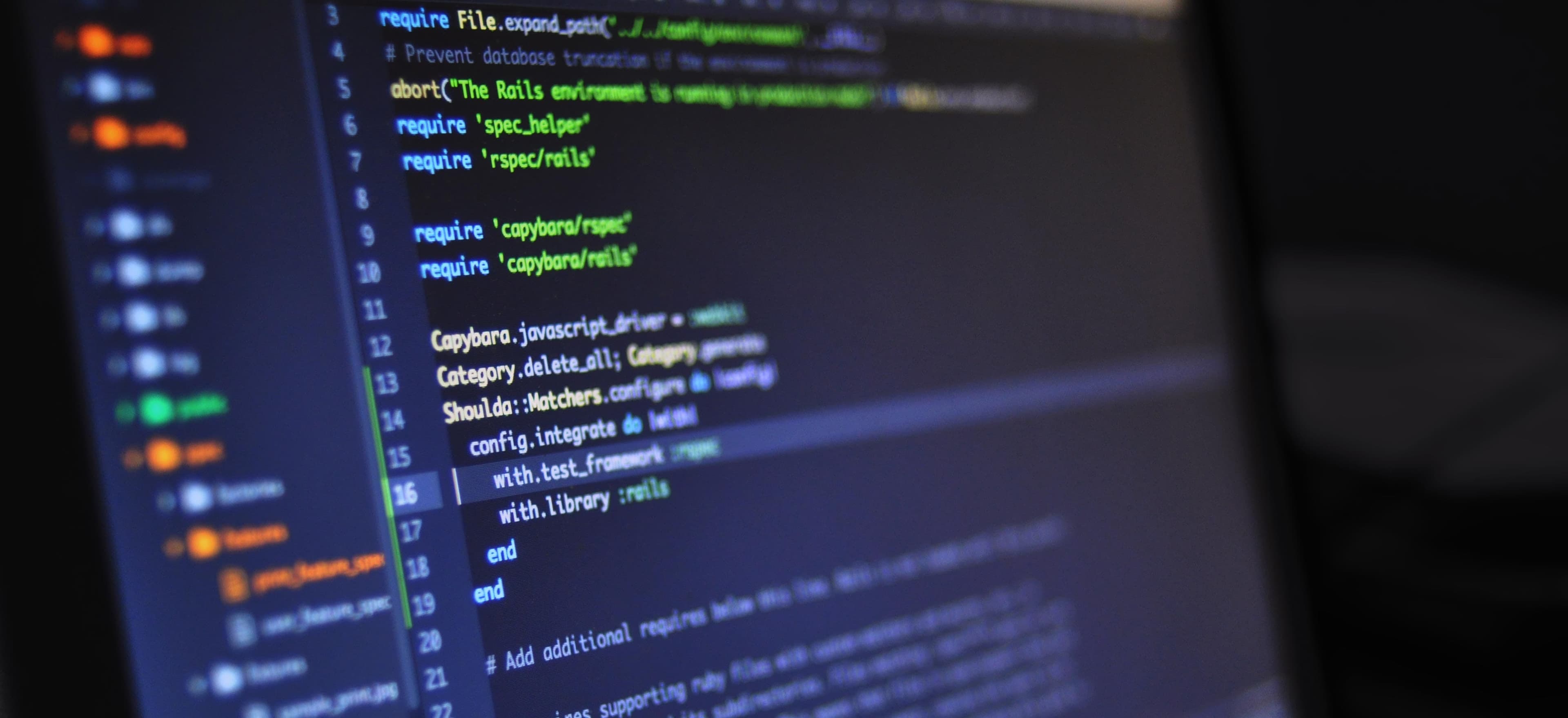
- Published on
Creating Engaging Callouts with JavaFX Animated Effects
In today's fast-paced digital world, user engagement is paramount. One effective way to capture and retain user attention in Java applications is through the use of callouts—informative pop-ups that convey information in a visually appealing manner. By incorporating animated effects, we can elevate the user experience even further.
In this blog post, we will explore how to create engaging callouts in JavaFX with animated effects. We will cover:
- Understanding Callouts: What are callouts and why use them?
- Setting Up Your JavaFX Environment: A quick guide.
- Creating a Basic Callout: Laying the foundation.
- Adding Animations: Making your callouts lively.
- Enhancing User Experience: Best practices for effective callouts.
- Conclusion: Bringing it all together.
Understanding Callouts
Callouts are graphical user interface elements used to attract users' attention to specific points of interest in an application. They can provide supplementary information, highlight important data, or guide the user through a process. By employing animations, we can make these callouts not only informative but also appealing, enhancing user retention and satisfaction.
Why Use Callouts?
- Focus Attention: Visually distinct elements draw user focus.
- Informative: They can consolidate important information in a concise manner.
- Interactive: Animated transitions encourage interactivity, leading to better user engagement.
Setting Up Your JavaFX Environment
Before delving into creating callouts, ensure that you have JavaFX set up in your development environment.
Installation Steps
- Download JavaFX: Get the latest version from Gluon.
- Configure Your IDE: Follow the setup instructions specific to your IDE. If you're using IntelliJ IDEA, for example, you can add JavaFX as a library in your project settings.
- Check Configuration: Ensure your project can locate JavaFX libraries.
Now that your environment is ready, let’s move on to building the callout.
Creating a Basic Callout
Let's create a simple callout that can display messages. We'll build a JavaFX application and add a button to trigger the callout.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
public class CalloutExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Show Callout");
Label callout = createCallout("This is a callout!");
button.setOnAction(e -> {
callout.setVisible(true);
});
StackPane layout = new StackPane();
layout.getChildren().addAll(button, callout);
Scene scene = new Scene(layout, 300, 250);
primaryStage.setTitle("Callout Example");
primaryStage.setScene(scene);
primaryStage.show();
}
private Label createCallout(String message) {
Label callout = new Label(message);
callout.setStyle("-fx-background-color: lightblue; -fx-padding: 10; -fx-visible: false; -fx-font-size: 14px;");
return callout;
}
public static void main(String[] args) {
launch(args);
}
}
Code Breakdown
- Creating the Scene: A
StackPane
is used to layer components, allowing for easy positioning of the callout and button. - Visibility Control: The callout is initially invisible and becomes visible when the button is clicked.
This basic callout does the trick, but to truly engage users, it's essential to add animations.
Adding Animations
Animations add a dynamic aspect to our callouts. For this, we will use the TranslateTransition
class from JavaFX.
Adding Animation to the Callout
We'll modify the previous code to include an animated entrance effect for the callout.
import javafx.animation.TranslateTransition;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.util.Duration;
public class AnimatedCalloutExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Show Animated Callout");
Label callout = createCallout("This is an animated callout!");
button.setOnAction(e -> {
animateCallout(callout);
});
StackPane layout = new StackPane();
layout.getChildren().addAll(button, callout);
Scene scene = new Scene(layout, 300, 250);
primaryStage.setTitle("Animated Callout Example");
primaryStage.setScene(scene);
primaryStage.show();
}
private Label createCallout(String message) {
Label callout = new Label(message);
callout.setStyle("-fx-background-color: lightblue; -fx-padding: 10; -fx-visible: false; -fx-font-size: 14px;");
return callout;
}
private void animateCallout(Label callout) {
callout.setVisible(true);
callout.setTranslateY(-20); // Start position
TranslateTransition transition = new TranslateTransition(Duration.seconds(0.5), callout);
transition.setFromY(-20);
transition.setToY(0);
transition.setAutoReverse(false);
transition.setCycleCount(1);
transition.play();
}
public static void main(String[] args) {
launch(args);
}
}
Animation Breakdown
- TranslateTransition: This class enables the callout to slide in from above, enhancing visibility.
- Duration: The duration of the transition is set to half a second for a quick, yet noticeable effect.
- Visibility Handling: The callout is made visible before the animation plays.
This callout will now smoothly slide down into the user's view, making it more engaging.
Enhancing User Experience
While the above implementations show how to create effective callouts, there are best practices to ensure they are user-friendly:
Best Practices for Callout Design
- Keep It Simple: Limit the amount of text and stick to essential information. Users appreciate clarity.
- Be Contextual: Ensure that the callout appears at relevant times, providing timely information.
- Avoid Overuse: Too many callouts can overwhelm users and diminish their effectiveness.
- Test Visibility: Use contrasting colors for your callouts to ensure they stand out against the background.
- Consider Accessibility: Ensure text is legible, with suitable font sizes and color contrasts, so it’s inclusive for all users.
Wrapping Up
By following the steps outlined in this article, you can enhance your JavaFX applications with engaging callouts complemented by animated effects. Not only do these elements grab users' attention, but they also offer essential information in a memorable way.
To further explore the capabilities of JavaFX, consider checking out the JavaFX Documentation for more advanced techniques and tools that can enhance your user interfaces. Engaging users through well-crafted design can significantly impact overall satisfaction and usability, making your application stand out in a crowded marketplace.
Feel free to experiment with variations of the callout we discussed, and happy coding!