Conquering Java's Virtual Threads: A Performance Pitfall
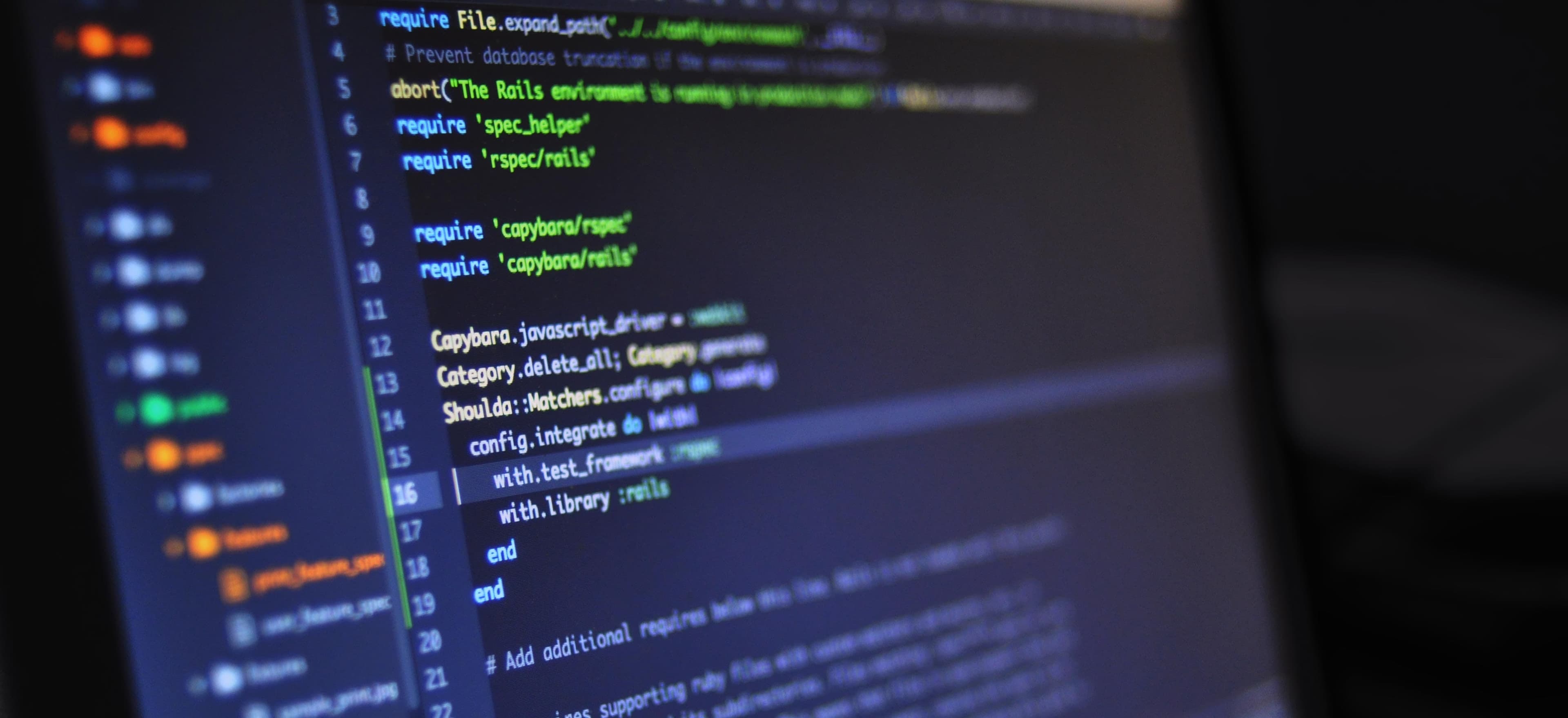
- Published on
Conquering Java's Virtual Threads: A Performance Pitfall
Java has undergone significant transformations since its inception, and one of the most exciting features introduced in recent years is virtual threads. This innovative feature is part of Project Loom, designed to simplify the development of concurrent applications in Java, making it easier than ever to manage simultaneous tasks. However, with new features come new challenges, and understanding the performance implications of virtual threads is essential.
In this blog post, we’ll delve deep into the implications of using virtual threads in Java applications. We will explore why and how virtual threads could lead to performance pitfalls, backed by practical examples and clear explanations.
What Are Virtual Threads?
Virtual threads are lightweight threads that allow developers to write asynchronous code in a way that resembles synchronous programming. Unlike traditional threads, which are mapped to the operating system’s native threads, virtual threads are managed by the Java Virtual Machine (JVM).
This means:
- Scalability: You can create thousands or even millions of virtual threads without the overhead associated with native threads.
- Simplicity: Code becomes easier to read and write, resembling standard blocking code while taking advantage of running tasks concurrently.
Example: Creating a Virtual Thread
Here's how you can create a virtual thread in Java:
Runnable task = () -> {
System.out.println("Running in a virtual thread!");
};
Thread.ofVirtual().start(task);
In the above snippet:
- We're using
Thread.ofVirtual()
to create a new virtual thread. - The task we define runs concurrently, enabling a non-blocking I/O operation.
This straightforward syntax masks a complex mechanism that, while beneficial in many scenarios, can lead to performance issues under certain conditions.
Potential Performance Pitfalls
While virtual threads offer many advantages, several potential pitfalls could harm performance:
1. Context Switching Overhead
In traditional multi-threading architectures, the JVM maps Java threads to native threads, making context switching relatively expensive. Virtual threads theoretically eliminate some of this overhead due to their lightweight nature. However, under certain conditions, the cost of context-switching can increase if not managed correctly.
Example: Context Switching Code
class ContextSwitchDemo {
public static void main(String[] args) {
Runnable task1 = () -> {
for (int i = 0; i < 10000; i++) {
// Simulated work
}
};
Runnable task2 = () -> {
for (int i = 0; i < 10000; i++) {
// Simulated work
}
};
for (int i = 0; i < 1000; i++) {
Thread.ofVirtual().start(task1);
Thread.ofVirtual().start(task2);
}
}
}
In this example, spawning too many virtual threads leads to increased context switching. While virtual threads can drastically improve throughput, overusing them can slow down performance due to higher context-switching overhead. Thus, understanding the optimal number of virtual threads for your application is critical.
2. Blocking Operations
While virtual threads are designed to handle blocking operations more efficiently than traditional threads, excessive blocking can still lead to performance degradation. When a virtual thread blocks, the underlying resources remain idle, causing a bottleneck.
Example: Blocking Operation
import java.net.HttpURLConnection;
import java.net.URL;
public class UrlFetcher {
public static void fetchUrl(String url) {
try {
HttpURLConnection connection = (HttpURLConnection) new URL(url).openConnection();
connection.setRequestMethod("GET");
connection.getResponseCode(); // this might block
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
for (int i = 0; i < 100; i++) {
Thread.ofVirtual().start(() -> fetchUrl("http://example.com"));
}
}
}
In the above code, while we are creating a virtual thread for each URL fetch, blocking on network I/O can lead to sub-optimal resource utilization. When any one of these threads blocks, the JVM may not manage resources effectively, potentially slowing down the application as a whole.
3. Error Handling and Tracing
Debugging concurrent applications can be challenging as it complicates stack traces. While virtual threads improve stack trace readability, seeing the origin of errors can still be complex especially when many virtual threads are in play.
4. Resource Management
With large numbers of virtual threads, such as those used to handle I/O-bound tasks, memory consumption can increase unexpectedly. Each virtual thread carries some overhead, and an unbounded number could lead to resource exhaustion.
Best Practices for Using Virtual Threads
To avoid performance pitfalls associated with virtual threads, here are several best practices to consider:
-
Limit the Number of Virtual Threads: Balance the number of virtual threads based on the available resources and workload characteristics.
-
Use Non-blocking I/O: Whenever possible, opt for non-blocking I/O operations to fully leverage the virtual threads’ capabilities.
-
Profile and Monitor Performance: Use JVM profiling tools to understand thread utilization and optimize your application accordingly.
-
Error Handling: Ensure robust error handling and logging in virtual threads to facilitate better debugging and maintenance.
-
Careful Thread Management: Create a thread pool if your application requires a predictable and controlled number of threads.
Closing Remarks
Java’s virtual threads provide powerful mechanisms to enhance the performance and simplicity of concurrent programming. However, understanding the potential performance pitfalls is equally crucial. By effectively managing context switches, avoiding blocking operations, and applying best practices, developers can enjoy the benefits of virtual threads without falling into performance traps.
Keep educating yourself about new features in Java, like the impressive changes coming with Project Loom. For more information on Java's virtual threads, check out the Project Loom Official Page and the Java 17 Documentation.
Java continues to evolve, and being aware of both its strengths and limitations will allow developers to build efficient, performance-oriented applications that harness the power of concurrency effectively. Happy coding!
Checkout our other articles