Struggling with JPA Reference Identity Issues? Here's How!
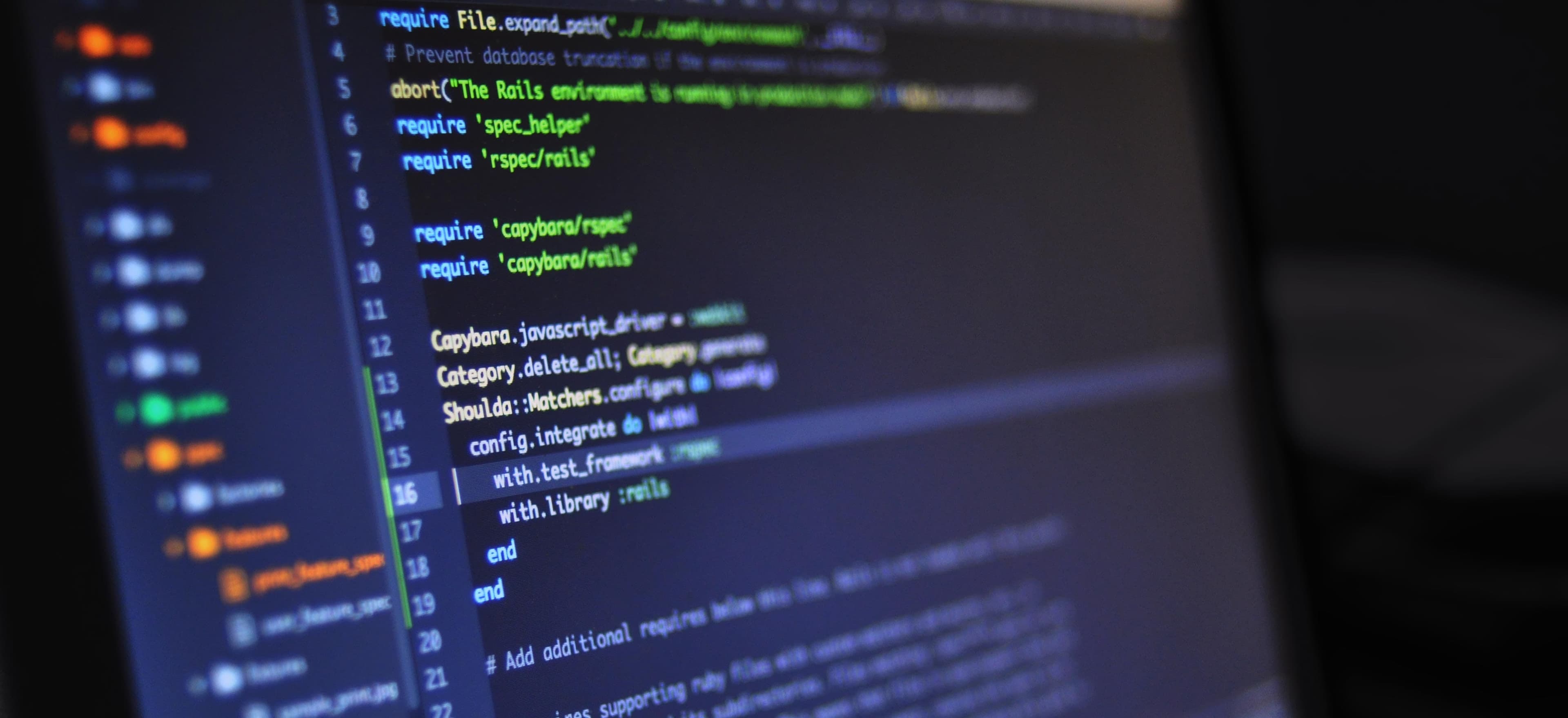
- Published on
Struggling with JPA Reference Identity Issues? Here's How!
Java Persistence API (JPA) is a powerful framework that can simplify database interactions in Java applications. However, when working with JPA, many developers encounter reference identity issues that can lead to unexpected behaviors. In this article, we'll explore common problems related to reference identities in JPA, alongside solutions to help you navigate these challenges.
What is JPA?
JPA is a specification that provides a way to manage relational data in Java applications. It allows developers to map Java objects to database tables, simplifying database operations and improving development speed. JPA serves as the backbone for various frameworks, such as Hibernate, EclipseLink, and OpenJPA.
Understanding Reference Identity Issues
Reference identity issues arise mainly from the complex relationships between entities. In a typical JPA setup, an entity may reference another entity through a relationship like @OneToOne
, @OneToMany
, or @ManyToMany
. When these relationships are not managed correctly, it can lead to inconsistencies and unexpected behaviors, such as:
- Duplicated entities
- Lazy loading issues
- Problems with cascading operations
Understanding the fundamental settings and how references are managed can help mitigate these challenges.
Entity Relationships: A Quick Overview
To comprehend reference identity issues better, let’s briefly discuss JPA entity relationships:
@OneToOne
: This relationship indicates that one entity is associated with one instance of another entity.@OneToMany
: One entity can be associated with multiple instances of another entity.@ManyToOne
: Many instances of one entity can be associated with one instance of another entity.@ManyToMany
: Multiple instances of one entity can relate to multiple instances of another entity.
Example: One-to-Many Relationship
Let's consider a simple example featuring a one-to-many relationship between Author
and Book
entities.
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private List<Book> books = new ArrayList<>();
// Constructors, getters, and setters omitted for brevity
}
In this example, the Author
class is the owner of the relationship, indicated by the mappedBy
attribute in @OneToMany
. This attribute references the field in the Book
entity that maps the relationship.
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
@JoinColumn(name = "author_id")
private Author author;
// Constructors, getters, and setters omitted for brevity
}
In the Book
class, the @ManyToOne
annotation denotes that many Books
can belong to one Author
, establishing a link through the foreign key author_id
.
Common Reference Identity Issues and Solutions
Despite the clear relationships defined in our entities, developers can still encounter several reference identity issues. Let's delve into some of the most common problems and their respective solutions.
1. Duplicate Entity Entries
It often occurs that when an entity is persisted, JPA treats it as a new instance instead of recognizing it as an existing one. This behavior is commonly caused by the lack of proper entity equality checks.
Solution: Override equals
and hashCode
methods in your entity classes.
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Author)) return false;
Author author = (Author) o;
return id != null && id.equals(author.id);
}
@Override
public int hashCode() {
return 31;
}
By implementing these methods, JPA can effectively manage and compare entities, preventing the creation of duplicates.
2. Lazy Loading Issues
Lazy loading is a common strategy in JPA to optimize data retrieval. However, when working with lazy-loaded entities, accessing them outside the context of a transaction can lead to LazyInitializationException
.
Solution: Always ensure that your entities are accessed within an active transaction and consider using fetch types wisely.
Author author = entityManager.find(Author.class, 1L); //Transaction context active
List<Book> books = author.getBooks();
Alternatively, you can use FetchType.EAGER
, but be cautious: it loads all associated entities regardless of necessity, potentially leading to performance issues.
3. Handling Cascading Operations
Cascading options in JPA determine how operations like persist
, merge
, remove
, and refresh
are propagated to associated entities. Misconfigured cascading can lead to operations not performing as expected.
Solution: Robust Configuration of Cascade Types
@OneToMany(mappedBy = "author", cascade = {CascadeType.PERSIST, CascadeType.MERGE}, fetch = FetchType.LAZY)
Using the sufficient cascade type helps maintain referential integrity without unnecessarily impacting performance or introducing bugs. For more information on cascade options, you can refer to the JPA documentation.
4. Detaching Entities
By default, JPA manages the lifecycle of entities. When you detach an entity, it loses its persistence context. Attempting to operate on it afterward can result in errors.
Solution: Reattach the entity using the merge()
method.
Author detachedAuthor = entityManager.find(Author.class, 1L);
entityManager.merge(detachedAuthor); // Reattach to persistence context
Key Takeaways
Navigating reference identity issues in JPA requires a solid understanding of entity relationships, as well as diligent application of best practices. By implementing proper equality checks, managing transaction contexts effectively, configuring cascading correctly, and handling entity states, you can enhance your application's performance and reliability.
Understanding these elements will lead to writing cleaner, more manageable code devoid of unexpected behaviors. As you develop with JPA, remember to continually refine your approach to entity management.
For more insights on entity management, visit the Hibernate User Guide.
By applying these strategies, you'll find that reference identity issues will not only become manageable, but your experience with JPA will also greatly improve. Happy coding!