Mastering @RequestParam: Common Mistakes to Avoid
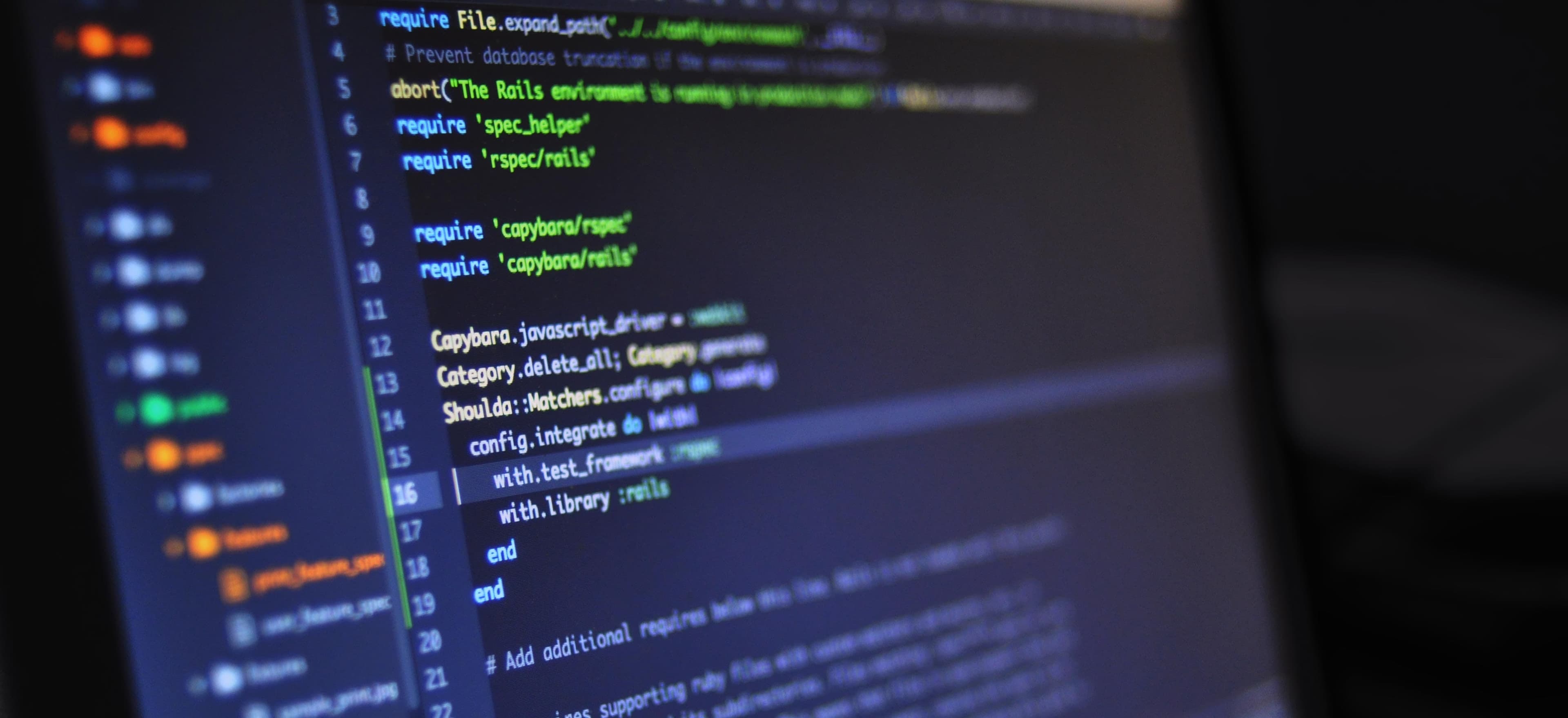
- Published on
Mastering @RequestParam: Common Mistakes to Avoid
When developing web applications in Spring, one of the most versatile annotations you'll encounter is @RequestParam
. This annotation is pivotal for handling HTTP request parameters in your controller methods. While using @RequestParam
seems straightforward, there are common pitfalls that many developers encounter. This blog post will delve into these common mistakes, provide guidance on how to avoid them, and offer best practices to enhance your Spring applications.
Understanding @RequestParam
The @RequestParam
annotation is part of the Spring Framework and is used to bind HTTP request parameters to Java method parameters in your controller. This allows a seamless interaction between your web forms and the backend processes.
Basic Example
Before diving into mistakes, let's start with a basic example. Here’s a simple request mapping where @RequestParam
is used:
@RestController
@RequestMapping("/api")
public class HelloController {
@GetMapping("/hello")
public String hello(@RequestParam String name) {
return "Hello, " + name + "!";
}
}
In this example, when the user accesses /api/hello?name=John
, the output would be Hello, John!
.
Common Mistakes to Avoid
1. Forgetting to Specify Required Parameters
By default, the @RequestParam
annotation treats parameters as required. If a required parameter is missing, Spring throws a MissingServletRequestParameterException
. It is essential to ensure that your frontend sends the necessary parameters or to gracefully handle their absence.
Solution
To prevent this exception, you can either add a default value or make the parameter optional.
Example:
@GetMapping("/greet")
public String greet(@RequestParam(required = false, defaultValue = "Guest") String name) {
return "Hello, " + name + "!";
}
In the above example, if name
is not provided, it defaults to "Guest" instead of causing an error.
2. Incorrect Data Type Handling
Another frequent error occurs when the data type of the parameter does not match the expected type in your method signature.
Solution
Spring automatically converts the incoming request parameters to the specified types when possible. However, you must ensure that the input adheres to expectations. If you expect an integer, the query string must always be a valid integer.
Example:
@GetMapping("/square")
public int square(@RequestParam int number) {
return number * number;
}
If you call /api/square?number=5
, it works fine. However, /api/square?number=abc
leads to a 400 Bad Request
error. To elegantly handle such scenarios, provide error handling in your application to alert users about invalid input types.
3. Ambiguity with Multiple Parameters
Spring allows you to pass multiple request parameters directly. However, ambiguity can arise if you possess parameters with the same name. If you do not address this carefully, your application may not behave as intended.
Solution
To handle multiple parameters effectively, ensure that you distinguish them clearly. Use lists when you expect multiple values under the same parameter name.
Example:
@GetMapping("/items")
public String getItems(@RequestParam List<String> item) {
return "Items requested: " + String.join(", ", item);
}
When users access /api/items?item=book&item=pen
, the output would be Items requested: book, pen
.
4. Lack of Validation
One of the common mistakes developers make is not validating the incoming parameters. This can lead to security vulnerabilities and application crashes.
Solution
Implement validation on your request parameters using the @Validated
or @Valid
annotations provided by Spring. Combine this with custom validation annotations to enforce more complex requirements.
Example:
@GetMapping("/register")
public String register(@RequestParam @Email String email) {
return "Email registered: " + email;
}
Here, the registration method expects a valid email format. If the input does not meet that format, Spring will automatically respond with a 400 Bad Request
.
5. Overlooking Query Parameter Defaults
When using optional parameters, it is crucial to define default values. Ignoring this can lead to null pointers or unintended behaviors.
Solution
Always set sensible default values for your optional parameters.
Example:
@GetMapping("/users")
public List<User> getUsers(@RequestParam(defaultValue = "10") int limit) {
return userService.getUsers(limit);
}
In this case, if the limit is not provided, it defaults to 10 users.
6. Ignoring Encoding Issues
When parameters contain special characters, they can become problematic if not properly encoded. Issues can arise with spaces, commas, etc.
Solution
Always ensure that your parameters are URL encoded, particularly if they include characters that may disrupt URI formatting.
For instance, the following URL should be encoded properly:
/api/search?query=spring framework
It should appear as:
/api/search?query=spring%20framework
Best Practices
Following best practices can significantly enhance the robustness and maintainability of your applications:
-
Use Descriptive Parameter Names: Even though query parameters are often short, descriptive names help maintain readability.
-
Utilize Strong Typing: Leverage enum types whenever possible to allow better validation of the expected values.
-
Document Your API: Using tools like Swagger could simplify the documentation of your endpoints and their parameters. Tools such as Springdoc OpenAPI can automatically generate OpenAPI documentation.
-
Make Use of @RestControllerAdvice: Create a global handler for exceptions that arise from
@RequestParam
usage to provide better user feedback. -
Keep Security in Mind: Validate all input parameters to avoid injection attacks and other security vulnerabilities.
The Last Word
The @RequestParam
annotation is a powerful feature within the Spring Framework, empowering developers to handle HTTP request parameters with ease. However, it is essential to be mindful of common pitfalls surrounding this annotation. By understanding these mistakes and adhering to best practices, you can develop resilient Spring applications, providing a seamless user experience without unexpected errors.
For further reading, explore the official Spring documentation on @RequestParam
. Happy coding!