Mastering Images in Android ListView: Common Pitfalls
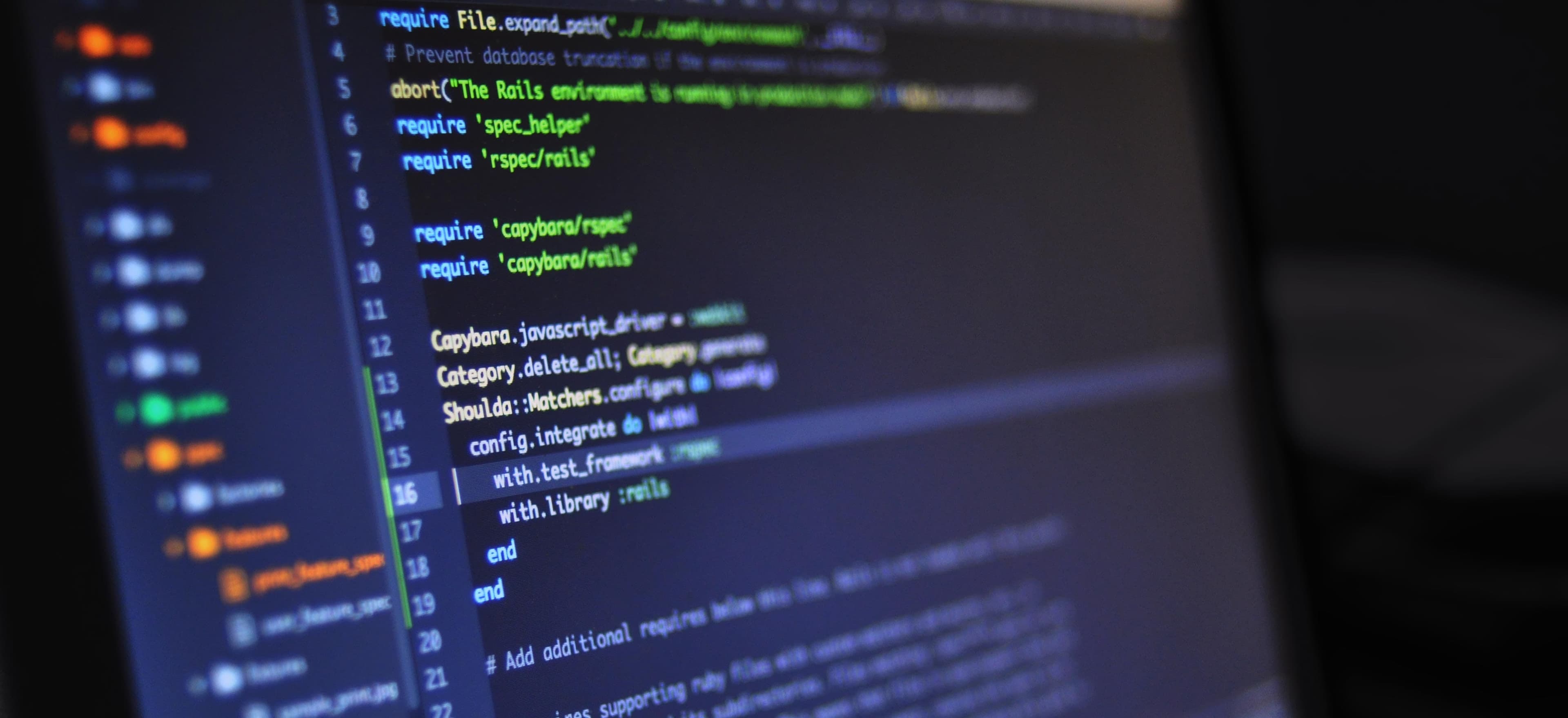
- Published on
Mastering Images in Android ListView: Common Pitfalls
Displaying images in a ListView on Android can significantly enhance the user experience. However, it can also lead to performance issues and memory pitfalls if not handled correctly. In this blog post, we'll dive into the common pitfalls of managing images in Android ListView and provide guidance on avoiding these issues.
Understanding ListView and Image Management
A ListView
is a view group that displays a list of scrollable items. Each item in this list can contain images, text, or any combination of UI components. When dealing with images, developers often face challenges like performance bottlenecks and memory leaks, especially when loading high-resolution images or a large number of images.
The Pitfalls of Images in ListView
Before we start looking at how to effectively work with images in a ListView, let's identify the common pitfalls developers encounter.
- Inadequate Image Loading: Loading images directly from a URL or file into views without optimization can lead to sluggish UI performance.
- Memory Leaks: Holding onto references to views or contexts can lead to memory leaks.
- Not Recycling Views: Failing to reuse view resources can increase the load on your app, resulting in poor performance.
- Lack of Caching: Not caching images can slow down scrolling and increase network usage unnecessarily.
Optimizing Image Loading in ListView
1. Use Efficient Image Libraries
Avoid handling image loading manually. Instead, leverage powerful libraries like Glide or Picasso which take care of various common issues internally.
Example using Glide
First, ensure to include the Glide dependency in your build.gradle file:
dependencies {
implementation 'com.github.bumptech.glide:glide:4.12.0'
annotationProcessor 'com.github.bumptech.glide:compiler:4.12.0'
}
Next, load images in your adapter class:
import com.bumptech.glide.Glide;
// Other imports...
public class MyAdapter extends ArrayAdapter<MyItem> {
public MyAdapter(Context context, List<MyItem> items) {
super(context, 0, items);
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
MyItem item = getItem(position);
if (convertView == null) {
convertView = LayoutInflater.from(getContext()).inflate(R.layout.item_layout, parent, false);
}
ImageView imageView = convertView.findViewById(R.id.image_view);
// Using Glide to load the image
Glide.with(getContext())
.load(item.getImageUrl())
.placeholder(R.drawable.placeholder) // Set a placeholder image
.error(R.drawable.error) // Set an error image
.into(imageView);
return convertView;
}
}
Why Use Glide?
- Placeholder and Error Handling: Ensures a smoother user experience by displaying a placeholder while the image is being loaded.
- Automatic Caching: Glide manages in-memory and disk caching automatically, which greatly reduces the need for repetitive image loading.
2. Image View Recycling
Since ListView recycles its views, ensure that you are properly managing view states. Use the ViewHolder
pattern to improve performance and readability.
ViewHolder Pattern Example
public class MyAdapter extends ArrayAdapter<MyItem> {
static class ViewHolder {
ImageView imageView;
TextView textView;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder viewHolder;
if (convertView == null) {
convertView = LayoutInflater.from(getContext()).inflate(R.layout.item_layout, parent, false);
viewHolder = new ViewHolder();
viewHolder.imageView = convertView.findViewById(R.id.image_view);
viewHolder.textView = convertView.findViewById(R.id.text_view);
convertView.setTag(viewHolder);
} else {
viewHolder = (ViewHolder) convertView.getTag();
}
MyItem item = getItem(position);
Glide.with(getContext())
.load(item.getImageUrl())
.placeholder(R.drawable.placeholder)
.error(R.drawable.error)
.into(viewHolder.imageView);
viewHolder.textView.setText(item.getName());
return convertView;
}
}
Why Use the ViewHolder Pattern?
- Reduced FindViewById Calls: Minimizes the number of times you call
findViewById()
, which is performance-intensive. - Better Performance: Significantly improves scrolling performance through efficient view recycling.
3. Implement Image Caching Strategy
If you're loading images from a remote server, ensure you have a caching strategy in place to avoid unnecessary network calls:
- In-Memory Cache: Efficient for recent images used within the same session.
- Disk Cache: Helpful for persisting images over sessions. Both Glide and Picasso automatically handle this for you.
4. Avoid Handling Bitmaps Directly
Loading high-resolution images directly into ImageView
s can lead to OutOfMemory errors. Always resize images before display.
Example
public static Bitmap decodeSampledBitmapFromResource(Resources res, int resId, int reqWidth, int reqHeight) {
final BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
BitmapFactory.decodeResource(res, resId, options);
options.inSampleSize = calculateInSampleSize(options, reqWidth, reqHeight);
options.inJustDecodeBounds = false;
return BitmapFactory.decodeResource(res, resId, options);
}
// Calculate sample size
public static int calculateInSampleSize(BitmapFactory.Options options, int reqWidth, int reqHeight) {
final int height = options.outHeight;
final int width = options.outWidth;
int inSampleSize = 1;
if (height > reqHeight || width > reqWidth) {
final int halfHeight = height / 2;
final int halfWidth = width / 2;
while ((halfHeight / inSampleSize) >= reqHeight && (halfWidth / inSampleSize) >= reqWidth) {
inSampleSize *= 2;
}
}
return inSampleSize;
}
Why Resize Images?
- Prevent OutOfMemory Errors: Resizing avoids allocating too much memory to the image.
- Improved Loading Times: Smaller image files load faster, leading to a smoother user experience.
Final Thoughts
Managing images in Android ListView can be tricky, but by understanding and implementing best practices, you can prevent performance issues and ensure a seamless experience for your users. By utilizing libraries like Glide or Picasso, adhering to the ViewHolder pattern, caching images effectively, and resizing images, you can expertly navigate the common pitfalls in image management.
For further reading, check out:
- Developing Android Apps
- Best Practices for Loading Images on Android
By integrating these strategies into your application, you can create a more efficient and visually appealing user interface that effectively showcases images in your ListView components. Happy coding!