Top Strategies for Managing JPA POJO Extensions
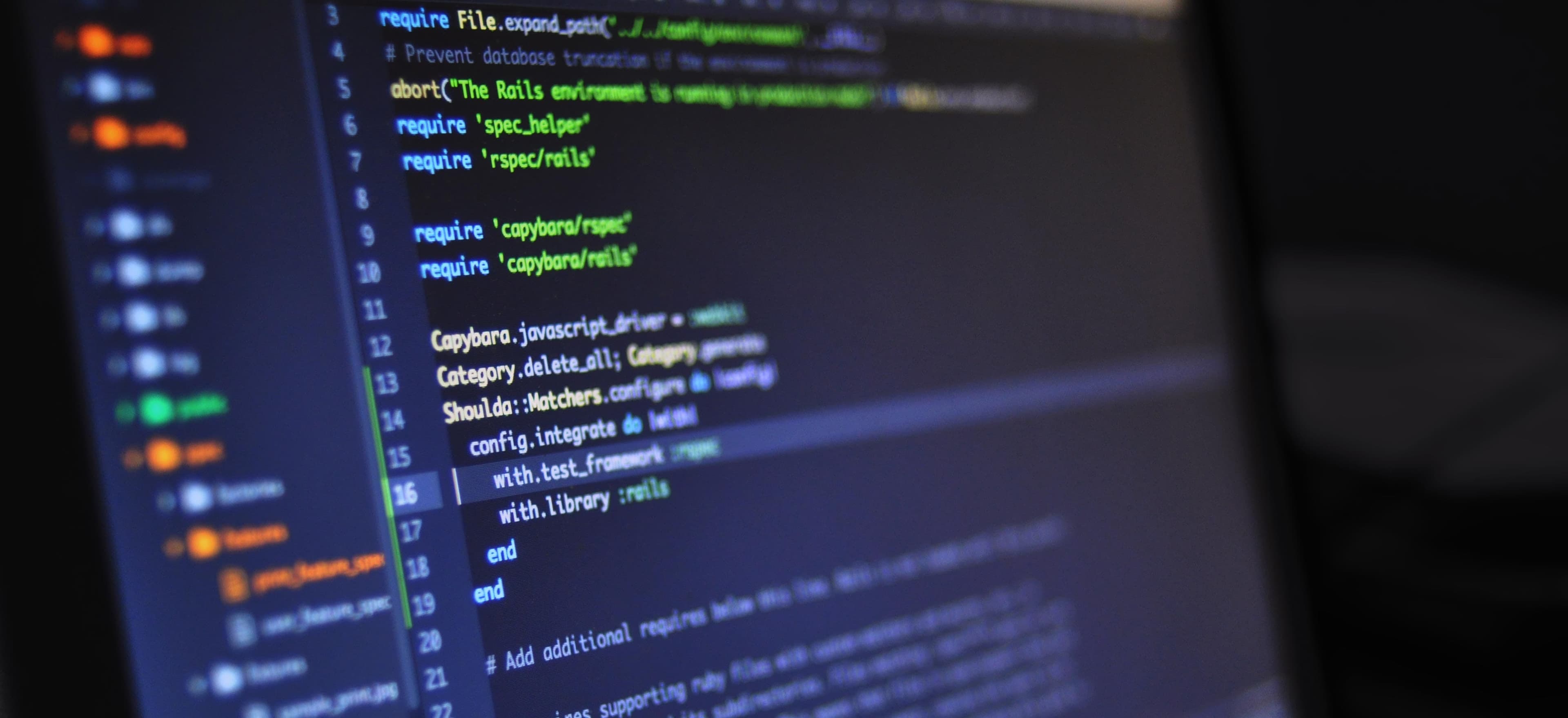
- Published on
Top Strategies for Managing JPA POJO Extensions
When working with Java Persistence API (JPA), you often encounter Plain Old Java Objects (POJOs) that represent your database entities. While handling these can be straightforward, things can get a bit tricky when you want to extend the functionality of these POJOs. In this blog post, we will delve into the top strategies for managing JPA POJO extensions effectively, ensuring clarity and ease of management.
What is JPA?
Java Persistence API (JPA) is a powerful framework that allows for object-relational mapping (ORM) in Java applications. It simplifies database interactions by allowing developers to work with data using Java objects rather than SQL. JPA is particularly beneficial for managing persistent data and handling complex data relationships.
Understanding POJOs in JPA
A POJO is a simple object that doesn't have to adhere to any particular structure or framework. POJOs in JPA are used to represent the entity model and are typically marked with annotations such as @Entity
, @Table
, and @Column
. This makes them convenient for database operations while keeping them simple.
Basic Example of a JPA POJO
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Constructors, Getters, and Setters
public User() {}
public User(String name, String email) {
this.name = name;
this.email = email;
}
public Long getId() {
return id;
}
public String getName() {
return name;
}
public String getEmail() {
return email;
}
}
In the example above, the User
class is marked as an entity. It represents a user table in the database, including essential attributes like name
and email
.
Strategies for Managing JPA POJO Extensions
1. Inheritance Strategy
One of the key strategies for extending JPA POJOs is through inheritance. JPA supports several inheritance mapping strategies, which allows you to define a base class and derive subclasses from it.
Types of Inheritance Strategies:
- Single Table Inheritance: All classes in the hierarchy are stored in a single table.
- Joined Table Inheritance: Each class in the hierarchy has its own table.
- Table Per Class Inheritance: Each class has its own table, and there is no additional table for the superclass.
Example Using Joined Table Inheritance
import javax.persistence.*;
@Entity
@Inheritance(strategy = InheritanceType.JOINED)
public abstract class Person {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// Getters and Setters
}
@Entity
public class Employee extends Person {
private double salary;
public Employee(String name, double salary) {
super(name);
this.salary = salary;
}
// Getters and Setters
}
@Entity
public class Customer extends Person {
private String email;
public Customer(String name, String email) {
super(name);
this.email = email;
}
// Getters and Setters
}
Why Use Inheritance?
- Code Reusability: Common attributes and methods are defined in the base class, reducing duplication.
- Type Safety: Subclasses can have specific behaviors while still being treated polymorphically as the base type.
2. Composition Over Inheritance
While inheritance can be useful, it often leads to complex hierarchies. Instead, consider using composition, where instead of extending classes, you include them as fields in your POJO.
Example of Composition
import javax.persistence.*;
@Entity
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private double totalAmount;
@ManyToOne
private User user;
// Constructors, Getters, and Setters
}
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// Now similar attributes can be added without strict inheritance rules
}
Why Use Composition?
- Flexibility: Easily extend POJO functionality by adding new fields without changing existing relationships.
- Decoupling: Allows POJOs to remain independent from one another, promoting better organization.
3. Mixins with Interfaces
Sometimes, it’s beneficial to have shared behavior across multiple entities without rooting them in a common superclass. Creating interfaces can help achieve this.
Example of Using Interfaces in JPA
public interface Auditable {
void setCreatedDate(LocalDateTime date);
LocalDateTime getCreatedDate();
}
@Entity
public class Product implements Auditable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private LocalDateTime createdDate;
@Override
public void setCreatedDate(LocalDateTime date) {
this.createdDate = date;
}
@Override
public LocalDateTime getCreatedDate() {
return createdDate;
}
}
Why Use Interfaces?
- Shared Behavior: Entities can implement the same interface, allowing for consistent behavior.
- No Strict Hierarchy: Allows for more flexibility in your design.
4. Use MapStruct for DTOs
Data Transfer Objects (DTOs) can help separate your internal POJO structures from what’s exposed externally—for instance, through APIs. Tools like MapStruct provide an efficient way to handle this mapping with annotations.
Example of MapStruct DTO
import org.mapstruct.Mapper;
import org.mapstruct.Mapping;
import org.mapstruct.factory.Mappers;
@Mapper
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper(UserMapper.class);
@Mapping(target = "emailAddress", source = "email")
UserDTO userToUserDTO(User user);
}
Why Use DTOs?
- Security: Prevent exposing sensitive fields.
- Performance: Allows selective loading of data.
5. Keep Your JPA POJOs Clean
Lastly, it's essential to keep your JPA POJOs focused. Ideally, these classes should only concern themselves with persistence logic.
- Avoid complex business logic in POJOs.
- Use service layers to handle such logic.
The Bottom Line
Managing JPA POJO extensions can be achieved through various strategies, including inheritance, composition, interfaces, and DTOs. Each approach has its unique advantages, and the right choice often depends on the specifics of your project. The goal is to maintain clarity, flexibility, and reusability in your code while adhering to object-oriented principles.
By embracing these strategies, you ensure that your JPA entities remain adaptable to changes, maintainable over time, and aligned with the overall architecture of your Java application.
For further reading, you may explore:
If you have further questions or insight on managing JPA POJO extensions, feel free to share your thoughts in the comments section!