Troubleshooting Manual Validation in Spring MVC Forms
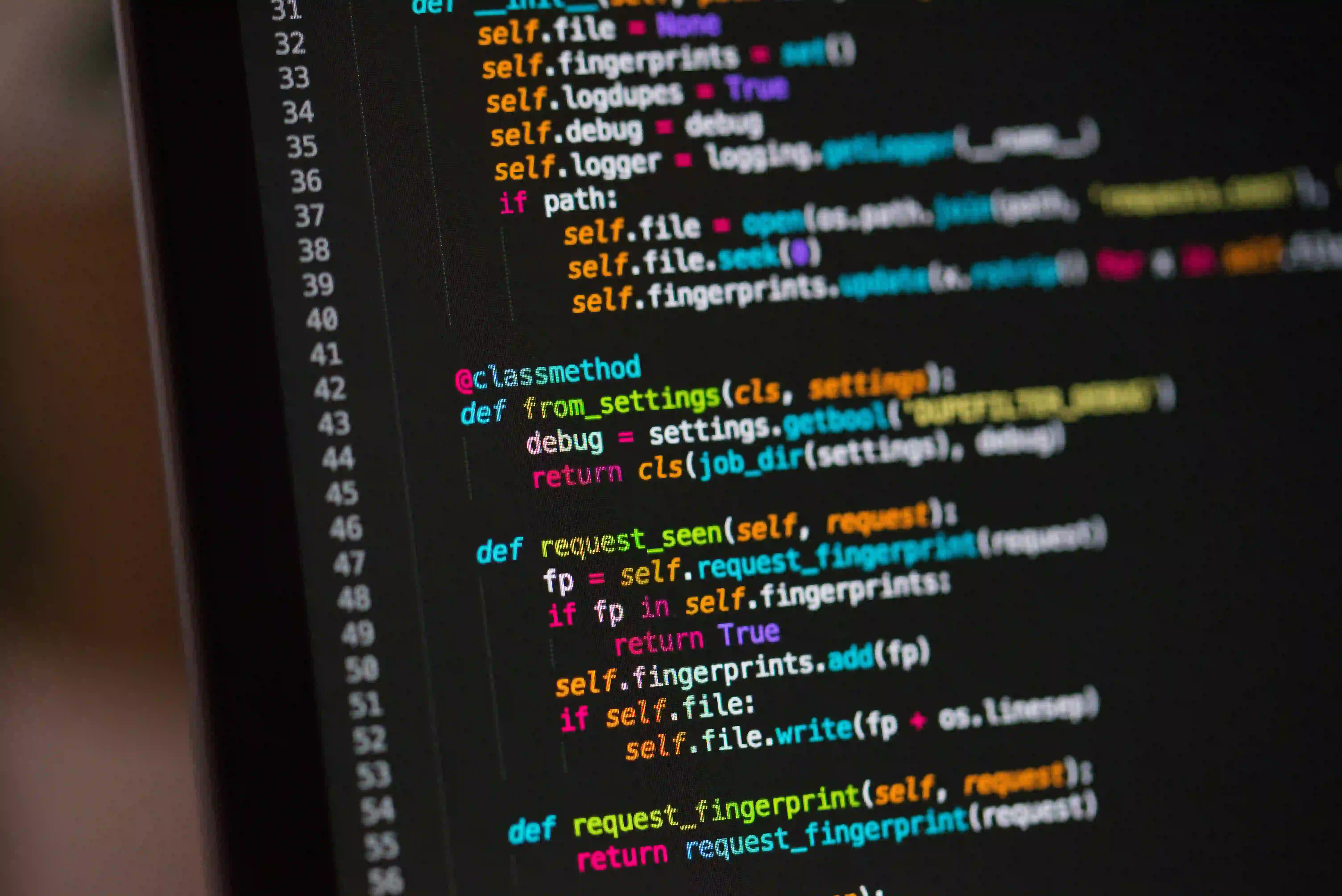
Troubleshooting Manual Validation in Spring MVC Forms
When building web applications with Spring MVC, form validation is a critical aspect to ensure data integrity and enhance user experience. Unfortunately, you might encounter issues during the validation process, particularly when dealing with manual validation. In this blog post, we will explore common pitfalls and their resolutions to help you troubleshoot manual validation in Spring MVC forms effectively.
Table of Contents
- Understanding Spring MVC Form Validation
- Setting Up Your Spring MVC Project
- Common Manual Validation Techniques
- Troubleshooting Validation Issues
- Example Code Snippets
- Conclusion
Understanding Spring MVC Form Validation
Spring MVC offers a robust mechanism for validating data submitted through forms. It allows you to define your validation rules using annotations, Java classes, or XML configurations. Manual validation refers to implementing custom validation logic instead of relying solely on the built-in validators provided by Spring.
Manual validation can be particularly useful in scenarios where complex business logic needs to be factored in. However, it also introduces potential challenges. Understanding these challenges is key to implementing effective manual validation.
Setting Up Your Spring MVC Project
Before diving into validation techniques, it’s essential to have a Spring MVC project set up. This project will serve as our testing ground for validation mechanisms.
Maven Dependencies
To get started, you will need the following Maven dependencies in your pom.xml
:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.4.0</version>
</dependency>
<dependency>
<groupId>javax.validation</groupId>
<artifactId>validation-api</artifactId>
<version>2.0.1.Final</version>
</dependency>
<dependency>
<groupId>org.hibernate.validator</groupId>
<artifactId>hibernate-validator</artifactId>
<version>6.1.0.Final</version>
</dependency>
Configure Spring MVC
You must configure the DispatcherServlet
and enable annotation-based configuration. Here’s how you can do that in your web.xml
:
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
You would also need the configuration class to enable MVC:
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
}
Common Manual Validation Techniques
When implementing manual validation, several techniques stand out:
-
Custom Validator Class: Create a class that implements the
Validator
interface. -
Using @InitBinder: Customize the binding and validation of data directly within the controller.
-
Validation Logic in Controller: Implement validation directly in your controller methods.
Example of a Custom Validator
Creating a custom validator allows you to reuse validation logic across different controllers. Here's an example of how to create and use a custom validator:
public class UserValidator implements Validator {
@Override
public boolean supports(Class<?> clazz) {
return User.class.equals(clazz);
}
@Override
public void validate(Object target, Errors errors) {
User user = (User) target;
if (user.getUsername() == null || user.getUsername().isEmpty()) {
errors.rejectValue("username", "Username cannot be empty");
}
if (user.getAge() < 0) {
errors.rejectValue("age", "Age cannot be negative");
}
}
}
In your controller, you can register this validator like so:
@InitBinder
protected void initBinder(WebDataBinder binder) {
binder.setValidator(new UserValidator());
}
Troubleshooting Validation Issues
Despite best efforts, you may still encounter validation issues. Here are some common problems and how to resolve them:
Problem 1: Validation Not Triggering
If validation is not being triggered at all, recheck the following:
-
Is the Validator Registered? Ensure the custom validator is registered via
@InitBinder
.☕snippet.java@InitBinder protected void initBinder(WebDataBinder binder) { binder.setValidator(new UserValidator()); }
-
Correct Binding of Form: Make sure that the form fields bind correctly to the properties of the
User
object.
Problem 2: Error Messages Not Displaying
If error messages are not displaying in your views, check:
-
Model Attribute Handling: Ensure you're passing the model attribute to the view.
☕snippet.java@GetMapping("/userForm") public String showForm(Model model) { model.addAttribute("user", new User()); return "userForm"; }
-
Thymeleaf Template: Ensure the error message is rendered properly in the view.
Problem 3: Business Logic Issues
If your validation logic is flawed due to incorrect business rules, you should:
-
Review Logic: Go through your validate method step by step.
-
Logging: Use logging to confirm the flow and see where it diverges from expected behavior. Implement logging in your validate method like this:
@Override
public void validate(Object target, Errors errors) {
User user = (User) target;
if (user.getUsername().length() < 3) {
logger.warn("Username too short: {}", user.getUsername());
errors.rejectValue("username", "Username must be at least 3 characters long");
}
}
Example Code Snippets
Now let’s combine everything into a simple example of a Spring MVC application that showcases manual validation:
- User Model:
public class User {
private String username;
private int age;
// Getters and setters
}
- Controller:
@Controller
public class UserController {
@InitBinder
protected void initBinder(WebDataBinder binder) {
binder.setValidator(new UserValidator());
}
@GetMapping("/userForm")
public String showForm(Model model) {
model.addAttribute("user", new User());
return "userForm";
}
@PostMapping("/submitUser")
public String submitUser(@ModelAttribute("user") User user, BindingResult result) {
if (result.hasErrors()) {
return "userForm";
}
// Save user or perform business logic
return "redirect:/success";
}
}
- Thymeleaf Template (userForm.html):
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>User Form</title>
</head>
<body>
<form th:action="@{/submitUser}" th:object="${user}" method="post">
<input type="text" th:field="*{username}" placeholder="Enter your username"/>
<p th:if="${#fields.hasErrors('username')}" th:errors="*{username}">Username Error</p>
<input type="number" th:field="*{age}" placeholder="Enter your age"/>
<p th:if="${#fields.hasErrors('age')}" th:errors="*{age}">Age Error</p>
<button type="submit">Submit</button>
</form>
</body>
</html>
Key Takeaways
Manual validation in Spring MVC forms is a powerful tool that can enhance your application's data integrity. By understanding how to troubleshoot common validation issues, such as failing triggers and error display problems, you can implement an effective validation strategy. With proper configuration, custom validators, and a systematic troubleshooting approach, you enhance both the reliability of your application and the experience of your users.
Remember, validation is not just about catching errors but also about guiding users towards providing the correct input. Happy coding!