Common pitfalls when starting with JavaFX 20: A guide
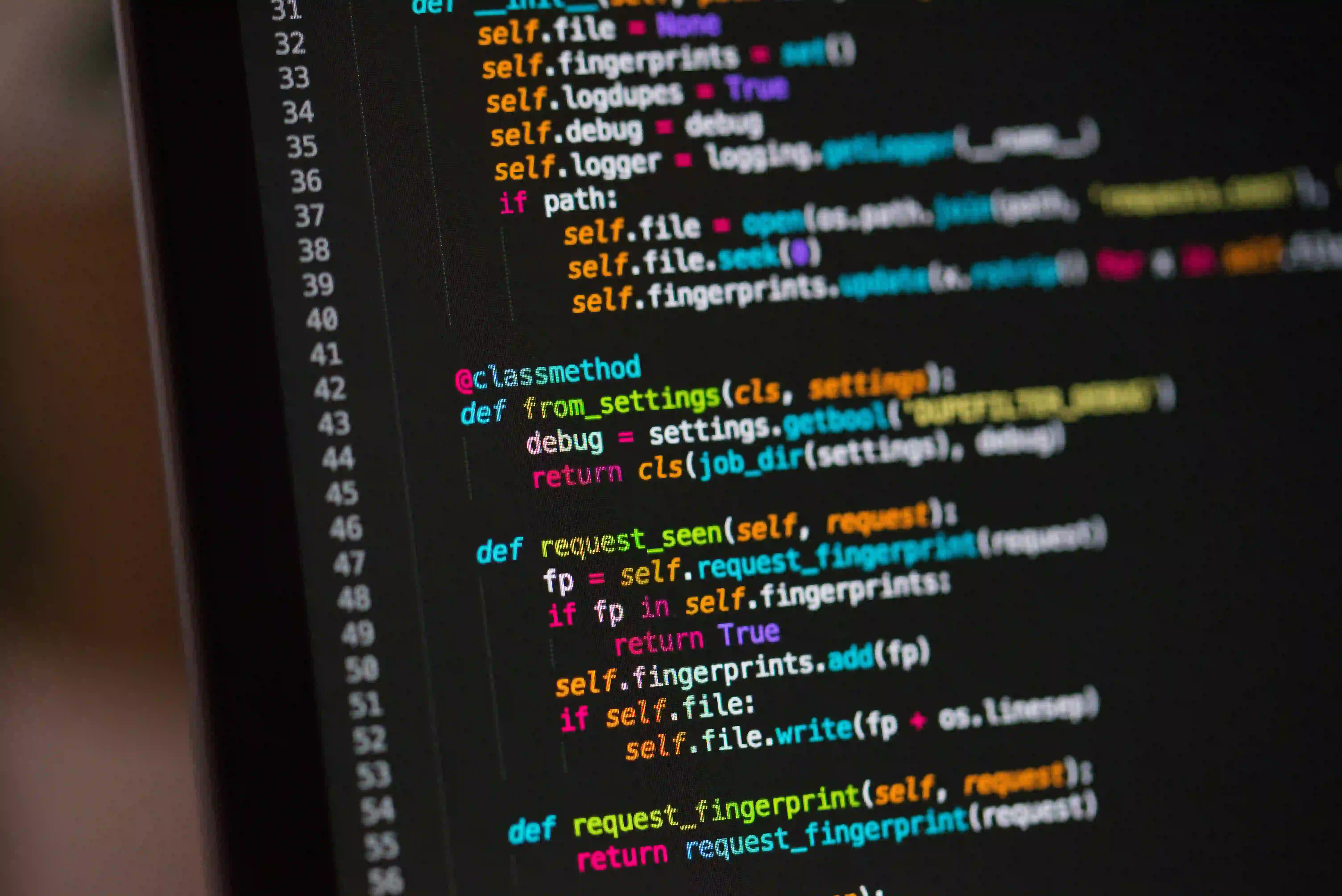
Common Pitfalls When Starting with JavaFX 20: A Guide
JavaFX is a robust platform designed for building rich client applications in Java. With JavaFX 20, developers can create visually appealing and modern desktop applications efficiently. However, new developers often encounter several common pitfalls that can lead to frustration and wasted time. In this guide, we’ll explore these pitfalls and provide practical solutions, along with code snippets for clarification.
Table of Contents
- Understanding JavaFX
- Pitfall #1: Failing to Set Up the Environment Properly
- Pitfall #2: Ignoring the MVC Architecture
- Pitfall #3: Overcomplicating the Scene Graph
- Pitfall #4: Misusing CSS Styling
- Pitfall #5: Neglecting Event Handling
- Pitfall #6: Poor Resource Management
- Pitfall #7: Underestimating the Value of Community
- Conclusion
Understanding JavaFX
JavaFX is the successor to Swing and is aimed at creating rich internet applications. It's built on Java, allowing developers to use their existing Java skills while offering features like hardware-accelerated graphics, audio, and a modern UI toolkit. To get started with JavaFX, ensure that you have the latest version of the JDK, appropriate libraries, and tools set up on your development environment.
Setting Up Your JavaFX Environment
Before diving into the potential pitfalls, make sure you have installed the latest version of JavaFX 20. You can download it from the official website. Use an IDE that supports JavaFX, such as IntelliJ IDEA or Eclipse, for better integration and ease of use.
Pitfall #1: Failing to Set Up the Environment Properly
One of the first mistakes many new developers make is not properly setting up their development environment. This includes following the right setup procedures for JavaFX libraries.
Solution
Make sure to:
- Install Java JDK 20.
- Download JavaFX 20 SDK.
- Add JavaFX to your project by including the libraries in your IDE settings.
Here’s an example of setting the VM arguments in IntelliJ IDEA:
--module-path "path_to_javafx_lib" --add-modules javafx.controls,javafx.fxml
This ensures that your application can access JavaFX’s controls and FXML modules.
Pitfall #2: Ignoring the MVC Architecture
JavaFX strongly supports the MVC (Model-View-Controller) design pattern, yet many beginners overlook this structured approach. Without MVC, your application can quickly become unmanageable.
Solution
Separate your application into three core components:
- Model: Represents the data structure.
- View: The FXML or scene that users interact with.
- Controller: Handles the logic and user input.
Here’s a basic implementation:
public class Model {
private SimpleStringProperty data = new SimpleStringProperty();
public String getData() {
return data.get();
}
public void setData(String value) {
data.set(value);
}
}
// In the Controller
public class Controller {
@FXML
private Label label;
private Model model;
public void setModel(Model model) {
this.model = model;
}
public void updateLabel() {
label.setText(model.getData());
}
}
This strategy makes your application easier to maintain and test.
Pitfall #3: Overcomplicating the Scene Graph
Another common mistake is overcomplicating the scene graph. A scene graph in JavaFX is a hierarchy of nodes (UI components). Beginners might try to place too many nodes in a single scene.
Solution
Keep the scene graph simple. Use containers effectively, such as VBox
, HBox
, or GridPane
, to structure your UI.
Here’s a simple example of using a VBox
:
VBox vbox = new VBox();
Label label = new Label("Welcome to JavaFX!");
Button button = new Button("Click Me!");
vbox.getChildren().addAll(label, button);
This structure keeps your UI organized and reduces performance overhead.
Pitfall #4: Misusing CSS Styling
JavaFX supports CSS for styling components, but it can be easy to misuse or overly rely on it.
Solution
Use CSS selectively. Start with small styles, then gradually build on them. Make sure that your stylesheets are linked correctly:
<AnchorPane stylesheets="@style.css">
<!-- UI Elements -->
</AnchorPane>
A sample CSS snippet could be:
.button {
-fx-background-color: blue;
-fx-text-fill: white;
}
This will ensure consistent styling across your application.
Pitfall #5: Neglecting Event Handling
JavaFX event handling can be a common source of confusion. Beginners often forget to properly attach event listeners, leading to unresponsive components.
Solution
Always set up event handlers for UI components. Here’s an example of an event handler for a button click:
button.setOnAction(event -> {
System.out.println("Button was clicked!");
});
By doing this, you ensure that interactions trigger the expected responses, improving user experience.
Pitfall #6: Poor Resource Management
Not managing resources effectively can lead to memory leaks or performance issues. JavaFX applications may load images or other resources improperly if not managed correctly.
Solution
Use the Image
class wisely. Prefer to use resource streams rather than direct file paths to reduce clutter.
Image image = new Image(getClass().getResourceAsStream("/images/sample.png"));
ImageView imageView = new ImageView(image);
This manages resources more efficiently, loading them from the JAR or classpath.
Pitfall #7: Underestimating the Value of Community
Lastly, many new JavaFX developers underestimate the importance of the community and available resources.
Solution
Engage with the community. Utilize forums, attend meetups, and contribute to JavaFX-related projects on platforms like GitHub. Websites like Stack Overflow and the JavaFX community are great places to seek support.
Closing the Chapter
Starting with JavaFX 20 can be daunting due to various pitfalls. However, by understanding common mistakes and how to avoid them, you can streamline your development process. Focus on proper environment setup, adhere to the MVC architecture, manage your scene graph efficiently, use CSS judiciously, implement event handling effectively, manage resources properly, and leverage the community’s knowledge.
With these guidelines, you are well on your way to creating beautiful and functional JavaFX applications. Happy coding!
If you have any questions or need further clarification, feel free to leave comments below or reach out to the community.