Understanding JVM Class Loader Issues: Common Pitfalls
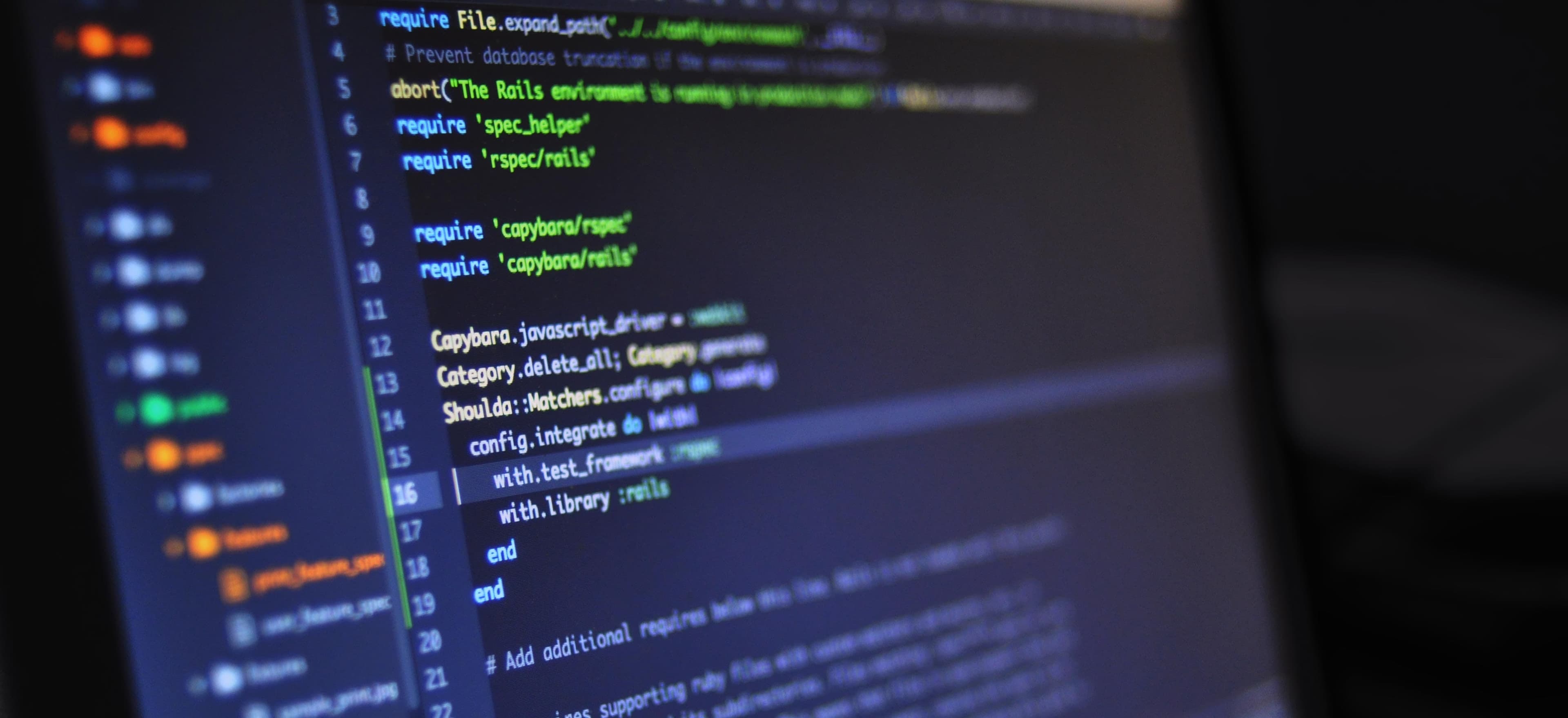
- Published on
Understanding JVM Class Loader Issues: Common Pitfalls
When it comes to Java Virtual Machine (JVM), class loading is one of the most fundamental mechanics that powers Java's dynamic capabilities. However, understanding class loaders can be quite complex, often leading to various pitfalls. In this blog post, we’ll break down class loader issues, clarify common misconceptions, and provide actionable insights to keep your Java applications running smoothly.
What is a Class Loader?
In Java, a class loader is a part of the Java Runtime Environment (JRE) that is responsible for loading class files into memory. This process involves locating the class file, reading its bytecode, and defining it within the JVM. There are three main types of class loaders in Java:
- Bootstrap Class Loader: Loads core Java classes from the JDK.
- Extension Class Loader: Loads classes from the Java extensions directory.
- System Class Loader: Loads classes from the application classpath.
Each class loader operates in a hierarchy and delegates the loading to its parent class loader. This delegation model is crucial for avoiding duplicate loading of classes.
Common Class Loader Issues
1. ClassNotFoundException
One of the most frequent issues developers encounter is the ClassNotFoundException
. This exception occurs when the JVM cannot find the class file. Reasons for this include:
- Class is not in the classpath.
- Incorrect package declaration in the Java file.
- The class file is located in a jar that is not included in the classpath.
To prevent this issue, always double-check your classpath settings.
Example Code Snippet
public class ClassLoaderExample {
public static void main(String[] args) {
try {
Class.forName("com.example.MyClass");
} catch (ClassNotFoundException e) {
System.err.println("Class not found: " + e.getMessage());
}
}
}
In the example above, Class.forName
attempts to load MyClass
. If it’s missing from the classpath, you’ll receive a ClassNotFoundException
which clearly indicates that the class loader could not locate the desired class.
2. NoClassDefFoundError
While ClassNotFoundException
indicates the class was never found, NoClassDefFoundError
occurs when a class was available at compile time but is missing during runtime. This usually happens due to:
- Class files being deleted or moved.
- Dependency issues when using build tools like Maven or Gradle.
- Conflicting versions of libraries.
3. Class Loader Conflicts
With the rise of microservices architecture and modular applications, class loader conflicts have increased. If multiple class loaders load the same class, you might face issues like:
- Incompatibility of classes due to different loaders.
- Outdated dependencies causing
ClassCastException
.
To mitigate this problem, you can use tools such as Maven to manage your dependencies ensuring all modules reference the same versions.
4. Class Initialization Issues
Sometimes classes fail to initialize due to static initialization blocks throwing exceptions, leading to a NoClassDefFoundError
even though the class was found initially. This often occurs because of:
- Faulty logic or errors in static blocks.
- Circular dependencies between classes.
Example Code Snippet
public class InitializationExample {
static {
if (true) { // Simulate condition that causes failure
throw new RuntimeException("Failed to initialize class");
}
}
public static void main(String[] args) {
try {
Class.forName("com.example.InitializationExample");
} catch (ClassNotFoundException | RuntimeException e) {
System.err.println("Class initialization failed: " + e.getMessage());
}
}
}
Here, the static block throws a RuntimeException
, preventing the class from being initialized. Always ensure that static initializers are robust and handle errors gracefully.
5. Visibility Issues with Different Class Loaders
Class loaders maintain separate namespaces for loaded classes. This means that two classes with the same name loaded by different class loaders are treated as different classes, leading to potential issues in type casting. You may encounter unexpected ClassCastException
if you attempt to cast between classes supposedly of the same type.
To ensure visibility and collaboration between different class loaders, ensure they share a common parent.
6. Classpath Issues in IDEs
Integrated Development Environments (IDEs) like IntelliJ IDEA and Eclipse can sometimes lead to class loader issues due to misconfigured classpaths. Always make sure:
- Your project dependencies are correctly set up.
- The output directories are configured properly.
- Clean and refresh the project when making significant changes to dependencies.
Best Practices for Managing Class Loaders
-
Consistent Packaging: Always maintain a standard directory structure for your classes, which aligns with package declarations.
-
Proper Dependency Management: Use dependency management tools like Maven or Gradle to manage library versions efficiently.
-
Monitoring Class Loading: Use JVM options like
-verbose:class
to monitor class loading activities during application runtime. -
Testing and Debugging: Employ unit testing to catch class-related issues early. Mock or stub dependencies to isolate and test your code effectively.
-
Documentation and Standards: Maintain clear documentation about class loading behavior, particularly in complex projects involving multiple class loaders.
-
Avoiding Static Initializers for Resource Management: It’s recommended to avoid complex logic in static initializers. Use static methods for resource management that handle exceptions properly.
Final Thoughts
Understanding JVM class loader issues is critical for any Java developer aiming to build robust applications. By familiarizing yourself with common pitfalls such as ClassNotFoundException
, NoClassDefFoundError
, and class loader conflicts, you can significantly reduce debugging time and enhance application stability.
For further reading, check out Java ClassLoaders - A Visual Explanation and JVM Class Loading Mechanism to deepen your understanding of these intricacies.
By applying best practices and understanding how class loaders work, you can leverage Java’s dynamic capabilities while minimizing issues in your development process. Happy coding!