Common Kafka Configuration Mistakes to Avoid in Spring
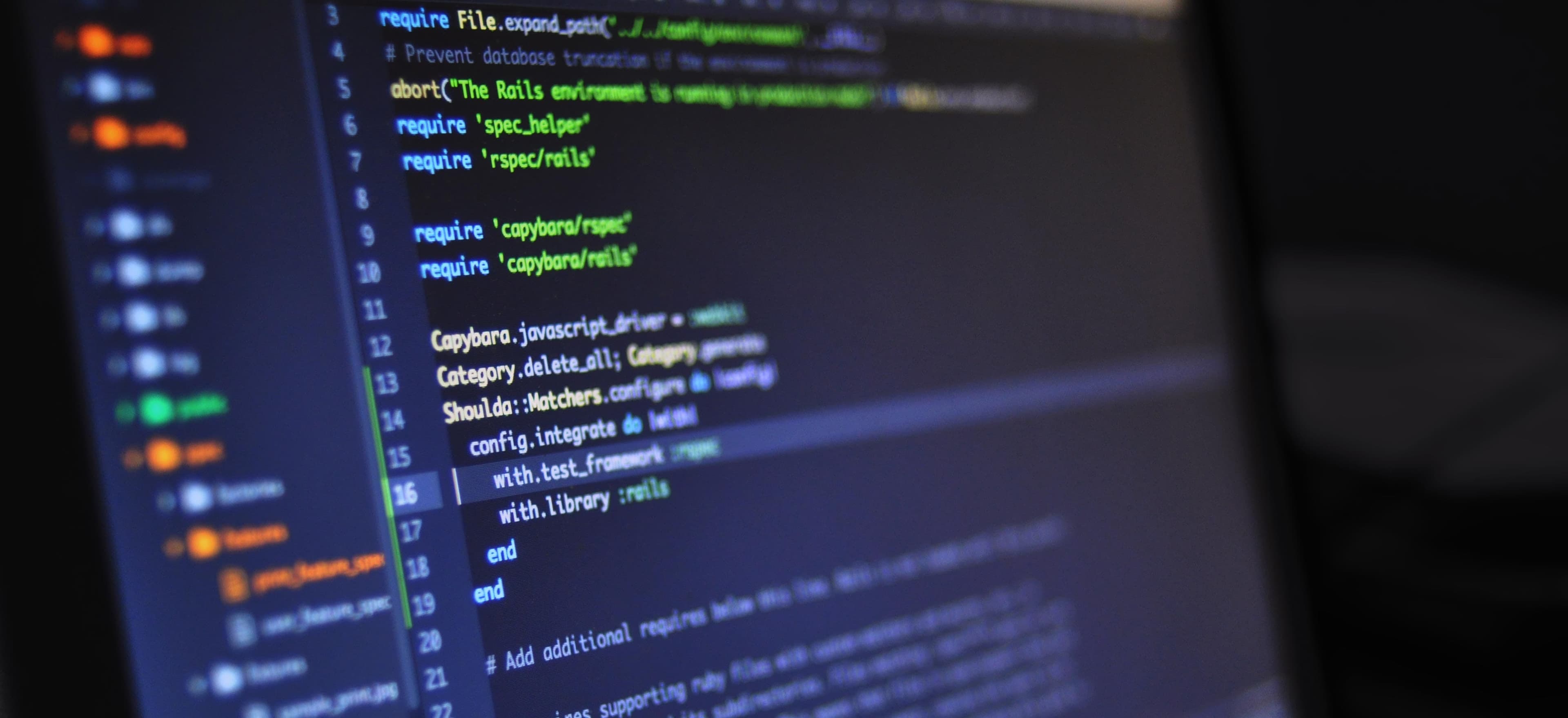
- Published on
Common Kafka Configuration Mistakes to Avoid in Spring
Kafka is becoming a staple for event-driven architectures and real-time data pipelines. When integrating Kafka into a Spring application, proper configuration is crucial for achieving reliability and performance. However, many developers encounter common pitfalls that can lead to frustrating bugs or performance bottlenecks. In this blog post, we will explore these common Kafka configuration mistakes and how to avoid them, ensuring your application functions seamlessly and efficiently.
1. Misconfiguring Producer Acknowledgments
One of the most essential settings for Kafka producers is the acks
property. This setting controls how many acknowledgments the producer expects from the broker before considering a request complete.
The Mistake
A common mistake is setting acks=0
, which may seem appealing for speed, as producers do not wait for any acknowledgment. However, this increases the risk of data loss.
The Solution
Set the acks
property to 1
or all
for increased reliability.
props.put(ProducerConfig.ACKS_CONFIG, "all");
By configuring acks to all
, you ensure that the leader broker waits for acknowledgment from all in-sync replicas before confirming receipt, thus reducing chances of data loss during broker failures.
2. Ignoring Consumer Group Configuration
Kafka operates on the concept of consumer groups, which allows multiple instances of consumers to share the load of message consumption. Many developers overlook the importance of configuring these groups properly.
The Mistake
Not configuring a group.id
for consumers can lead to unexpected behaviors when you have multiple consumer instances, as messages may be processed independently instead of being distributed evenly.
The Solution
Always set the group.id
property.
props.put(ConsumerConfig.GROUP_ID_CONFIG, "my-consumer-group");
This ensures that the consumers share the workload effectively, leading to better scalability and resource utilization.
3. Overlooking the auto.offset.reset
Property
The auto.offset.reset
property controls what happens when there is no initial offset or if the current offset does not exist.
The Mistake
If developers do not configure this property, the default value is latest
. This means that consumers will skip over any existing messages, which might not be the desired behavior when starting a new consumer group.
The Solution
Set the property wisely depending on your use case:
props.put(ConsumerConfig.AUTO_OFFSET_RESET_CONFIG, "earliest");
By setting it to earliest
, consumers will read all available messages from the beginning, ensuring that no data is lost during the initialization phase.
4. Not Setting Up Retry Policy for Producers
Kafka producers can fail due to various reasons, including network issues or broker unavailability. A common mistake is to leave out retry configurations.
The Mistake
Failing to configure retry settings can lead to lost messages in case of transient errors.
The Solution
Set up a retry policy for producers:
props.put(ProducerConfig.RETRIES_CONFIG, 3);
This configuration allows the producer to attempt to send messages a specified number of times before failing, thus increasing your application's resilience.
5. Not Considering the Serialization Mechanism
Serialization is crucial as it transforms the data into a byte array that Kafka can transport. A common mistake is not paying attention to the serialization format.
The Mistake
Using the default serializer without specifying a serialization mechanism for your data types can lead to compatibility issues and inefficient data processing.
The Solution
Specify the appropriate serializers for your producers and consumers.
props.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
props.put(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName());
By configuring custom serializers, you ensure that your data is processed correctly and can enhance performance based on your data types.
6. Skipping Topic Configuration
When producing messages, default configurations for topics can lead to significant issues like data retention and partition strategies.
The Mistake
Not defining key parameters like partitions
and replication.factor
, which can compromise data availability and performance.
The Solution
When creating topics, define these parameters explicitly.
kafka-topics --create --topic my-topic --bootstrap-server localhost:9092 --partitions 3 --replication-factor 2
Having multiple partitions helps with parallel processing, while an appropriate replication factor ensures high availability.
7. Inadequate Monitoring and Logging
Even well-configured Kafka setups can face runtime issues. Ignoring logging and monitoring makes it challenging to troubleshoot problems.
The Mistake
Relying solely on Kafka’s built-in logging without implementing application-level logs.
The Solution
Integrate logging frameworks like SLF4J and configure appropriate log levels.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class KafkaProducerExample {
private static final Logger logger = LoggerFactory.getLogger(KafkaProducerExample.class);
public void sendMessage(String message) {
logger.info("Sending message: {}", message);
// send message logic
}
}
Incorporating application logging provides deeper insight and helps trace issues more effectively.
8. Neglecting Consumer Polling Loop
Kafka consumers require a structured polling loop to efficiently retrieve messages.
The Mistake
A frequent oversight is to not maintain a proper polling loop, resulting in idle consumers that can’t keep up with incoming messages.
The Solution
Implement a solid polling mechanism:
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
records.forEach(record -> {
logger.info("Consumed message: {}", record.value());
// process record
});
}
This approach ensures that your consumer keeps fetching messages, ensuring that no data is left unprocessed.
The Bottom Line
Kafka is a powerful tool for building scalable and resilient event-driven systems. However, failing to configure Kafka properly can lead to serious issues, impacting data integrity and system performance. By avoiding these common mistakes and implementing the recommended solutions, you can make the most of your Kafka integration within Spring applications.
For further insights into Kafka, consider checking the official Apache Kafka documentation and explore community solutions on GitHub or through online forums.
Make your Kafka configurations count, enabling efficient real-time data processing and messaging in your systems!
Checkout our other articles