Securing XML with Digital Signatures: Common Pitfalls to Avoid
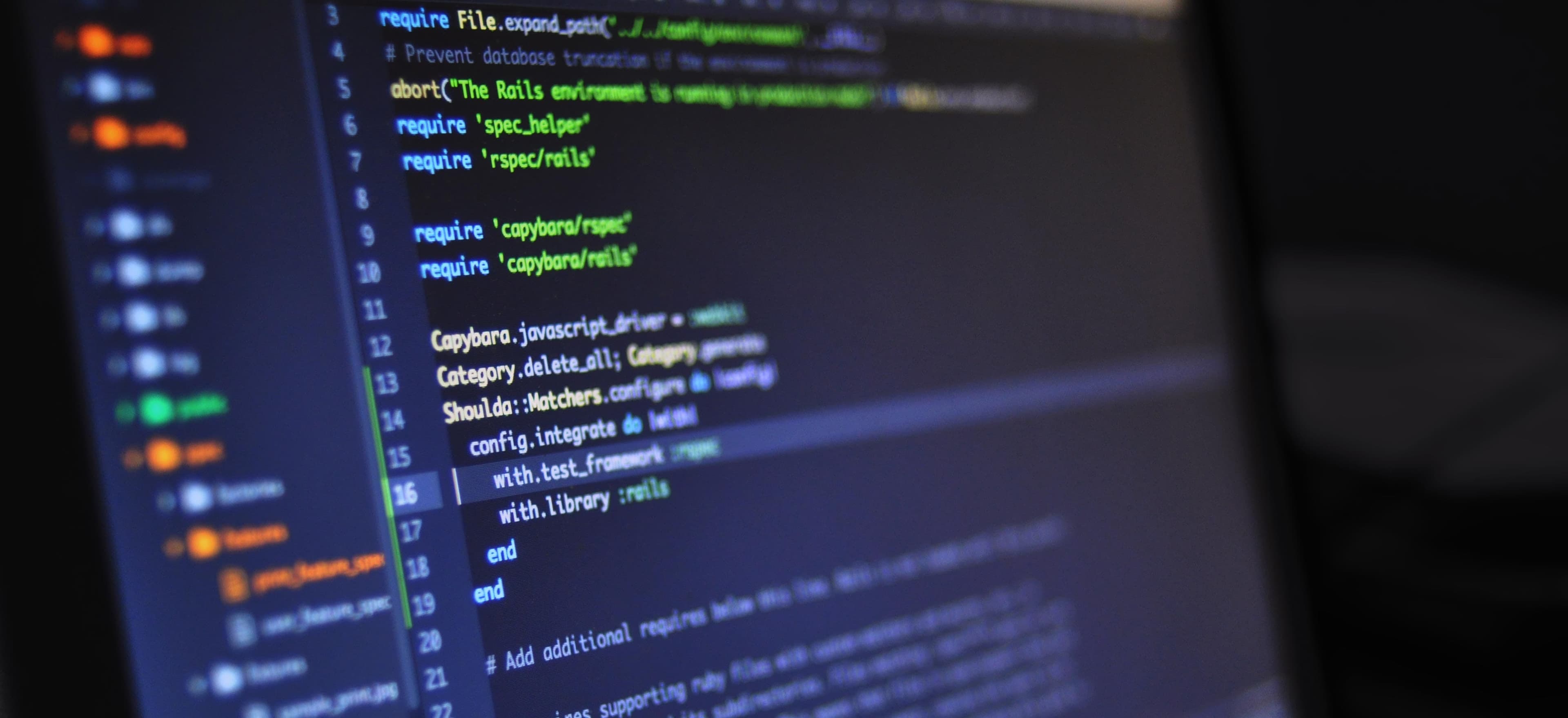
- Published on
Securing XML with Digital Signatures: Common Pitfalls to Avoid
In today's digital landscape, securing data is paramount. Extensible Markup Language (XML) is widely used for storing and transmitting structured data. However, XML can be vulnerable to various attacks if not handled properly. One of the best ways to secure XML documents is by using digital signatures. This blog post explores how to implement digital signatures in XML and highlights common pitfalls to avoid, ensuring your XML security is rock solid.
Understanding XML Digital Signatures
Digital signatures provide a means of verifying the authenticity and integrity of digital messages or documents. In the context of XML, a digital signature can ensure that the content has not been altered during transit and confirm the identity of the sender.
XML Signature Syntax
The XML digital signature is represented in the <Signature>
element adhering to the XML Signature Syntax and Processing standard, as specified by W3C. The basic structure consists of:
- SignedInfo: Contains the data being signed.
- SignatureValue: The generated digital signature.
- KeyInfo: Information about the key used to create the signature, enabling verification.
Why Use XML Digital Signatures?
Using XML digital signatures adds a layer of trustworthiness to your XML documents. The primary benefits include:
- Integrity: Any alteration of the signed XML will invalidate the signature.
- Authentication: Validating the signature confirms the identity of the sender.
- Non-repudiation: The sender cannot deny the creation of the signed document.
Implementing XML Digital Signatures in Java
The best way to implement XML digital signatures in Java is by utilizing the Java API for XML Processing (JAXP) along with the Java XML Digital Signature API (JSR 105). Below, we will walk through a typical implementation.
Example: Signing XML in Java
import javax.xml.crypto.*;
import javax.xml.crypto.dsig.*;
import javax.xml.crypto.dsig.dom.DOMSignContext;
import javax.xml.crypto.dsig.spec.SignatureMethodParameterSpec;
import javax.xml.crypto.dsig.spec.C14NMethodParameterSpec;
import javax.xml.crypto.key.*;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.transform.*;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import java.security.*;
import java.util.*;
public class XMLSignatureExample {
public static void main(String[] args) throws Exception {
// Create the Document
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
Document doc = db.newDocument();
Element root = doc.createElement("example");
doc.appendChild(root);
Element child = doc.createElement("data");
child.setTextContent("This is a test");
root.appendChild(child);
// Generate KeyPair for signing
KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("RSA");
keyPairGen.initialize(2048);
KeyPair keyPair = keyPairGen.generateKeyPair();
// Create a XMLSignatureFactory
XMLSignatureFactory xmlSigFactory = XMLSignatureFactory.getInstance("DOM");
// Create the SignedInfo object
Reference reference = xmlSigFactory.newReference(
"", // No URI, we are signing the entire document
xmlSigFactory.newDigestMethod(DigestMethod.SHA256, null));
SignedInfo signedInfo = xmlSigFactory.newSignedInfo(
xmlSigFactory.newCanonicalizationMethod(CanonicalizationMethod.INCLUSIVE, (C14NMethodParameterSpec) null),
xmlSigFactory.newSignatureMethod(SignatureMethod.RSA_SHA256, null),
Collections.singletonList(reference)
);
// Create the XMLSignature object
XMLSignature xmlSignature = xmlSigFactory.newXMLSignature(signedInfo, null);
// Sign the XML
DOMSignContext dsc = new DOMSignContext(keyPair.getPrivate(), doc.getDocumentElement());
xmlSignature.sign(dsc);
// Transform to OutputStream
TransformerFactory tf = TransformerFactory.newInstance();
Transformer trans = tf.newTransformer();
DOMSource src = new DOMSource(doc);
StreamResult res = new StreamResult(System.out);
trans.transform(src, res);
}
}
Code Commentary
-
Document Creation: The code begins by creating a new XML document with a root element. This is where you can define your XML's structure and content.
-
Key Generation: A secure RSA key pair is created for signing purposes. Using a strong key ensures the integrity and security of your signed XML.
-
Creating the Signature: The
SignedInfo
object encapsulates the reference and methods for signing. We use SHA-256 for hashing and RSA for signature encryption. -
Signing the Document: The document is signed, and the signature element is appended. The transformation at the end outputs the signed XML to the console.
Common Pitfalls in XML Digital Signatures
While the implementation of XML digital signatures is straightforward, various pitfalls can jeopardize security. Below are common mistakes developers make.
1. Not Canonicalizing the XML Document
Canonicalization is the process of transforming an XML document into a standard format before signing it. Omitting this step can lead to discrepancies during signature validation. Changes in whitespace or element ordering can invalidate a signature even if the content is semantically identical.
To Avoid This: Always use canonicalization before signing:
xmlSigFactory.newCanonicalizationMethod(CanonicalizationMethod.INCLUSIVE, null);
2. Using Weak Cryptography
Using outdated algorithms (like SHA-1) or weak key sizes (e.g., RSA < 2048 bits) can compromise security. Attackers could exploit this weakness to forge signatures or modify documents undetected.
To Avoid This: Always opt for stronger algorithms and key lengths. Update libraries to implement modern security standards.
3. Ignoring Key Management
Improper handling of cryptographic keys can expose your signed XML documents. This includes using hard-coded keys or failing to rotate keys.
To Avoid This: Implement a secure key management system that handles key generation, storage, and rotation policies.
4. Signing Unnecessary Data
It's common to sign additional non-critical information that can lead to bloated signatures. This could reduce performance and increase the risk of unauthorized changes.
To Avoid This: Sign only the data necessary for your application, maintaining a clear focus on what must be protected.
5. Failing to Verify Signatures
Ensuring the integrity of signed XML documents is essential. Developers sometimes forget the critical step of verifying signatures on receipt.
To Avoid This: Implement signature verification processes whenever XML documents are consumed. Use the public key of the signer to validate the signature.
Bringing It All Together
XML digital signatures provide a robust mechanism for ensuring the integrity and authenticity of XML documents. However, avoiding common pitfalls is crucial to maximize security. By following best practices in key management, canonicalization, and signature verification, you can confidently secure your XML data.
For additional insights, you might want to check out the official Java Cryptography Architecture documentation.
By being cognizant of these common mistakes and implementing effective solutions, you can protect your XML documents against breaches, ensuring that your data remains secure and trustworthy. Happy coding!
Checkout our other articles