Troubleshooting Common Spring Data REST Issues in CRUD Apps
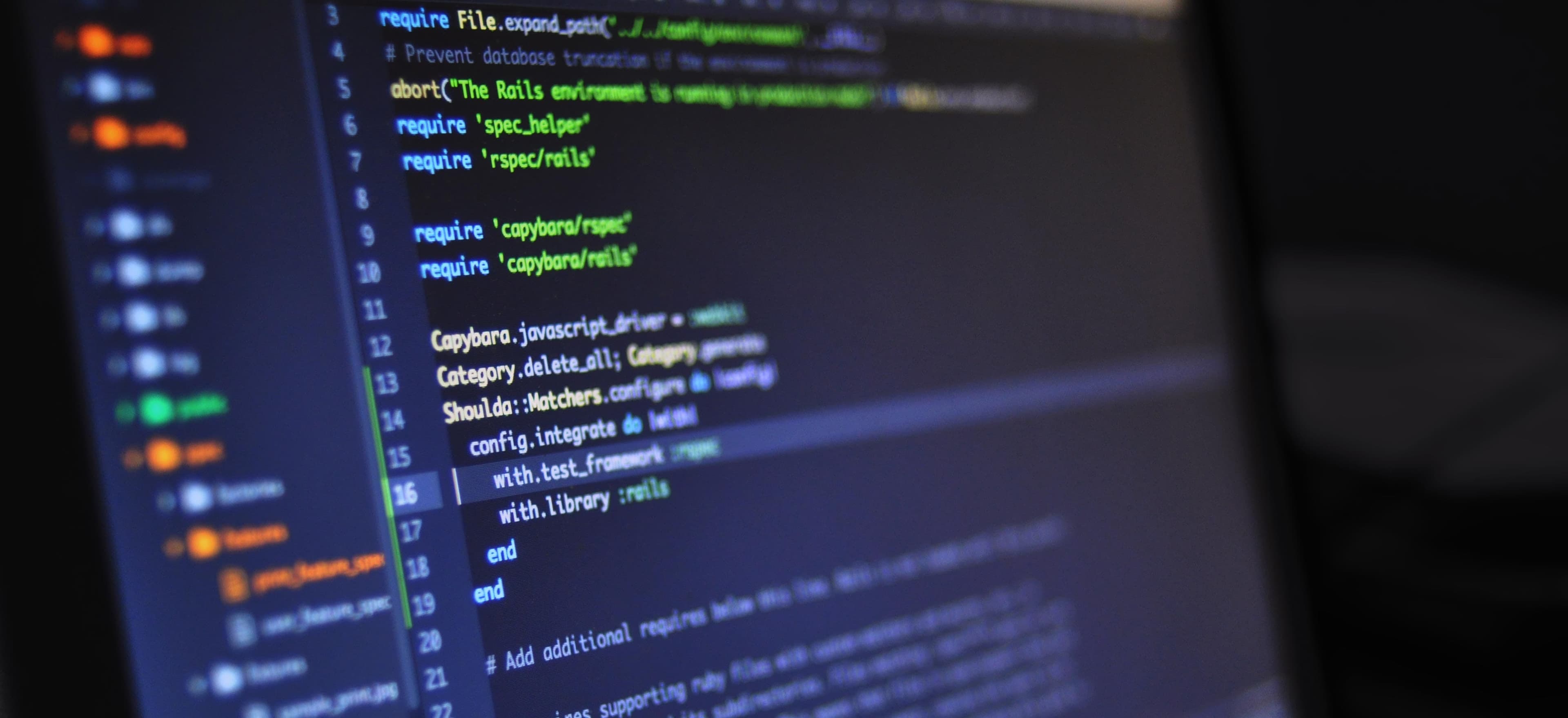
- Published on
Troubleshooting Common Spring Data REST Issues in CRUD Apps
Spring Data REST is an indispensable framework for developers looking to quickly expose their Spring Data repositories as RESTful APIs. However, as with any powerful tool, problems can arise. This blog post will guide you through the common issues you may encounter when working with Spring Data REST in your CRUD applications. We will explore the challenges, provide example code, and offer solutions to make your development process smoother.
Table of Contents
What is Spring Data REST?
Spring Data REST is part of the Spring ecosystem that simplifies the creation of hypermedia-driven RESTful APIs by automatically exposing Spring Data repositories. It handles CRUD operations for you, generating endpoints based on repository methods.
For instance, if you have a repository interface for an entity called Book
, Spring Data REST will automatically generate endpoints to create, read, update, and delete the Book
resources without requiring any additional code from you.
Example repository definition:
import org.springframework.data.repository.CrudRepository;
import com.example.demo.entity.Book;
public interface BookRepository extends CrudRepository<Book, Long> {
}
When you run your Spring Boot application, the following endpoints will be available by default:
- GET
/books
to retrieve all books - POST
/books
to create a new book - GET
/books/{id}
to retrieve a specific book - PUT
/books/{id}
to update a specific book - DELETE
/books/{id}
to delete a specific book
This functionality is great; however, issues can occur. Let’s explore some common pitfalls.
Common Issues
1. Missing or Broken Endpoints
Symptoms:
- You notice that the expected endpoints are not available or return 404 errors.
Solution:
- First, check if your application is correctly scanning for repositories. Ensure that your main application class is located in a higher package than your repository interfaces. Also, use the
@EnableSpringDataWebSupport
annotation if you need pagination features.
Example:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
Why This Matters: The class needs to be in a suitable package to enable component scanning. If it's not, your repository definitions won't be recognized, leading to missing endpoints.
2. Security Concerns
Symptoms:
- Unauthorized access attempts are blocked but lead to confusing error messages.
Solution:
- Spring Security often comes into play with Spring Data REST. You can customize security configurations by overriding a few methods.
Here’s a basic example using Java Config:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/books/**").permitAll() // Allow all access to books
.anyRequest().authenticated(); // Require authentication for other requests
}
}
Why This Matters: Security is a core component in any application. You might want endpoints to be publicly accessible for CRUD operations while securing others. Understanding how to configure Spring Security in conjunction with REST is crucial.
3. Handling Relationships
Symptoms:
- CRUD operations for related entities result in unexpected behavior or data.
Solution:
- Spring Data REST supports relationships between entities. Ensure your entity relationships are correctly set up with annotations like
@ManyToOne
or@OneToMany
. Use the@RestResource
annotation to fine-tune visibility.
Example:
import javax.persistence.*;
import java.util.Set;
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "author")
private Set<Book> books;
// Getters and setters
}
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
@JoinColumn(name = "author_id", nullable = false)
private Author author;
// Getters and setters
}
Why This Matters: If relationships are not correctly defined, it can create a cascade of issues in CRUD data integrity. Maintaining consistent relationships is vital for the overall functionality of your application.
4. Customizing Responses
Symptoms:
- You want to send additional information or format your response in a specific way but do not know how to customize it.
Solution:
- Spring Data REST provides several ways to customize responses. Use projection or the
@RepositoryRestResource
annotation to extend the capabilities.
Example:
import org.springframework.data.rest.core.annotation.RestResource;
import org.springframework.data.rest.core.annotation.RepositoryRestResource;
@RepositoryRestResource
public interface BookRepository extends CrudRepository<Book, Long> {
@RestResource(path = "by-title", rel = "by-title")
List<Book> findByTitle(@Param("title") String title);
}
Why This Matters: Customizing the API's response format is essential for client applications that may expect data in a specific structure or for performance optimization.
The Closing Argument
Spring Data REST is a powerful framework that allows for rapid development of RESTful services. By being aware of common pitfalls such as missing endpoints, security concerns, handling relationships, and customizing responses, you can ensure a streamlined development process.
For more resources on Spring Data REST, check out the Spring Data REST Reference Documentation and Spring Security Fundamentals.
Eliminating these common issues will not only save you development time but also enhance the quality and robustness of your applications. Happy coding!