Mastering API Gateway Patterns in Microservice Architecture
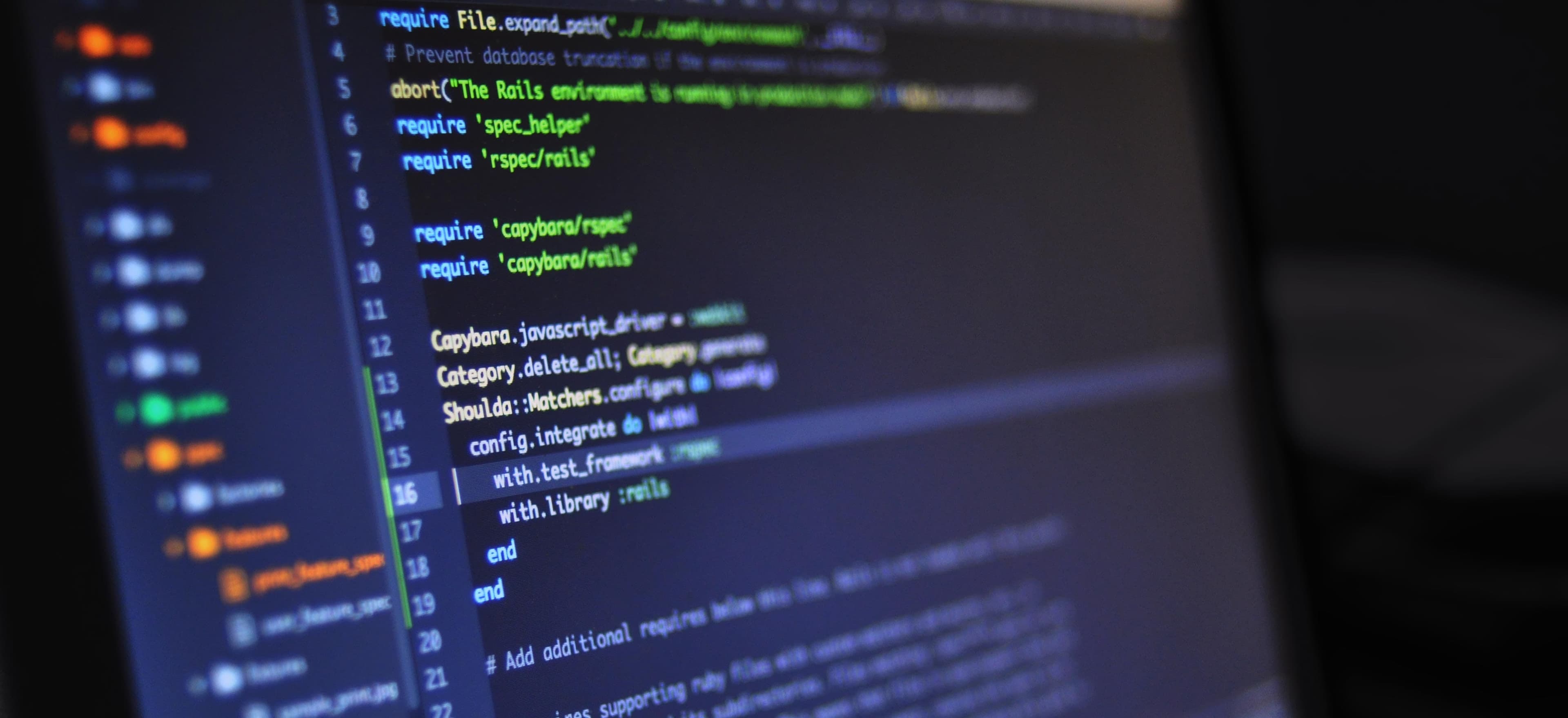
- Published on
Mastering API Gateway Patterns in Microservice Architecture
Microservice architecture has revolutionized how we build and deploy applications. One key component of this architecture is the API Gateway, which serves as the entry point for clients accessing backend services. This blog post aims to provide you with a comprehensive understanding of API Gateway patterns in microservices, breaking down their purpose, advantages, and implementation strategies.
What is an API Gateway?
An API Gateway is a server that acts as an intermediary between clients and microservices. It facilitates communication, enforces security, and manages traffic. By consolidating multiple backend services under a single entry point, it simplifies client interactions while enabling developers to maintain their microservices independently.
Key Functions of API Gateways
- Request Routing: Distributes client requests to the appropriate microservices based on URL paths and methods.
- Load Balancing: Allocates incoming requests across multiple instances of microservices, enhancing performance and availability.
- Security: Implements authentication, authorization, and encryption to protect sensitive data.
- Caching: Stores frequently accessed data to reduce load and improve response times.
- Rate Limiting: Controls the number of requests a client can make in a specified timeframe, preventing abuse.
Why Use an API Gateway?
API Gateways provide several benefits, making them a popular choice in microservice architectures:
- Simplicity: Clients only need to interact with one endpoint, reducing complexity in client-side code.
- Centralized Management: Enables consistent policies to handle authentication, logging, and monitoring across all services.
- Enhanced Security: Offers a single point to implement security measures, reducing vulnerabilities across multiple services.
- Performance: Features like caching and load balancing improve the user experience by ensuring faster response times.
Common API Gateway Patterns
API Gateway patterns vary by function and use case. Below are some of the most commonly employed patterns, each with distinct advantages.
1. Simple Forwarding
In this pattern, the API Gateway acts merely as a reverse proxy, forwarding requests from clients to the appropriate microservices.
Example:
@RestController
public class ApiGatewayController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/api/{service}/{endpoint}")
public ResponseEntity<String> forwardRequest(@PathVariable String service, @PathVariable String endpoint) {
String serviceUrl = "http://" + service + "/" + endpoint;
return restTemplate.getForEntity(serviceUrl, String.class);
}
}
Why this code? Using RestTemplate
, the API Gateway takes the incoming request and forwards it to the designated microservice. This keeps the gateway lightweight but can lead to complex routing logic as the architecture grows.
2. Aggregation
This pattern collects data from multiple services and combines them into a single response. It is particularly useful for scenarios where a client needs data from different microservices.
Example:
@RestController
public class AggregationController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/api/aggregate")
public ResponseEntity<AggregateResponse> aggregateData() {
User user = restTemplate.getForObject("http://user-service/api/user/1", User.class);
Order order = restTemplate.getForObject("http://order-service/api/order/1", Order.class);
return ResponseEntity.ok(new AggregateResponse(user, order));
}
}
Why this code? The API Gateway hits multiple endpoints, gathers user and order-related information, and combines them into a single response. This reduces the number of client requests while improving efficiency.
3. Security Gateway
This pattern offloads security responsibilities from microservices to the API Gateway. It handles authentication and authorization before forwarding requests.
Example:
@RestController
@RequestMapping("/secure")
public class SecureController {
@GetMapping("/api/resource")
public ResponseEntity<String> getSecureResource(HttpServletRequest request) {
String token = request.getHeader("Authorization");
if (!isTokenValid(token)) {
return ResponseEntity.status(HttpStatus.UNAUTHORIZED).build();
}
// Forward to microservices after validation
return ResponseEntity.ok("Secure Data");
}
private boolean isTokenValid(String token) {
// Token validation logic
return "valid-token".equals(token);
}
}
Why this code? The API Gateway verifies the authorization token before making any calls to the service. This centralizes security management and simplifies service implementation since they do not need to handle these concerns.
4. Rate Limiting
Implementing rate limiting through the API Gateway helps control traffic and prevents excessive load on backend microservices.
Example:
@Autowired
private RedisTemplate<String, Integer> redisTemplate;
@GetMapping("/api/resource")
public ResponseEntity<String> getResource(HttpServletRequest request) {
String clientId = request.getHeader("Client-ID");
Integer requestCount = redisTemplate.opsForValue().increment(clientId);
if (requestCount > MAX_REQUESTS_PER_MINUTE) {
return ResponseEntity.status(HttpStatus.TOO_MANY_REQUESTS).build();
}
return ResponseEntity.ok("Resource Data");
}
Why this code? Here, the API Gateway uses Redis to track and limit requests from individual clients to prevent overuse of resources. This helps maintain the stability of services.
Implementing an API Gateway
Now that we have covered various API Gateway patterns, let’s discuss how to implement an API Gateway within a microservice architecture.
Choosing the Right Tool
Several frameworks help in implementing API Gateways, including:
- Spring Cloud Gateway: A popular choice for Java developers leveraging Spring Boot for microservices.
- Kong: An open-source API Gateway that can easily be extended with plugins.
- AWS API Gateway: A managed service that simplifies API development while providing excellent scalability.
Setting Up Spring Cloud Gateway
Here’s a quick primer on how to set up Spring Cloud Gateway.
-
Add Dependencies: Include the necessary dependencies in your
pom.xml
file.<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-gateway</artifactId> </dependency>
-
Configure Routes: Use application properties to define routes.
spring: cloud: gateway: routes: - id: user-service uri: http://localhost:8081 predicates: - Path=/api/user/** - id: order-service uri: http://localhost:8082 predicates: - Path=/api/order/**
-
Run the Application: Start the Spring Boot application, and it will begin routing traffic to defined microservices.
Testing the API Gateway
Testing your API Gateway can be automated using tools like Postman or JUnit:
@Test
public void testForwardRequest() throws Exception {
mockMvc.perform(get("/api/user-service/api/user/1"))
.andExpect(status().isOk())
.andExpect(content().string(containsString("User Details")));
}
Why this code? Automated tests validate functionality and reliability, ensuring the API Gateway works as intended.
Lessons Learned
The API Gateway is an essential component of microservice architecture. It streamlines communication between clients and services while centralizing security, load balancing, and more. Understanding API Gateway patterns is crucial for building scalable and maintainable systems.
For more insights into microservices and API management, check out Spring Cloud Documentation.
By mastering the implementation of various API Gateway patterns, you set a solid foundation for designing robust microservice solutions that can adapt as your organization grows. Start experimenting with these patterns today and see how they can transform your architecture!
Checkout our other articles