Overcoming SimpleDateFormat Issues in JavaFX Applications
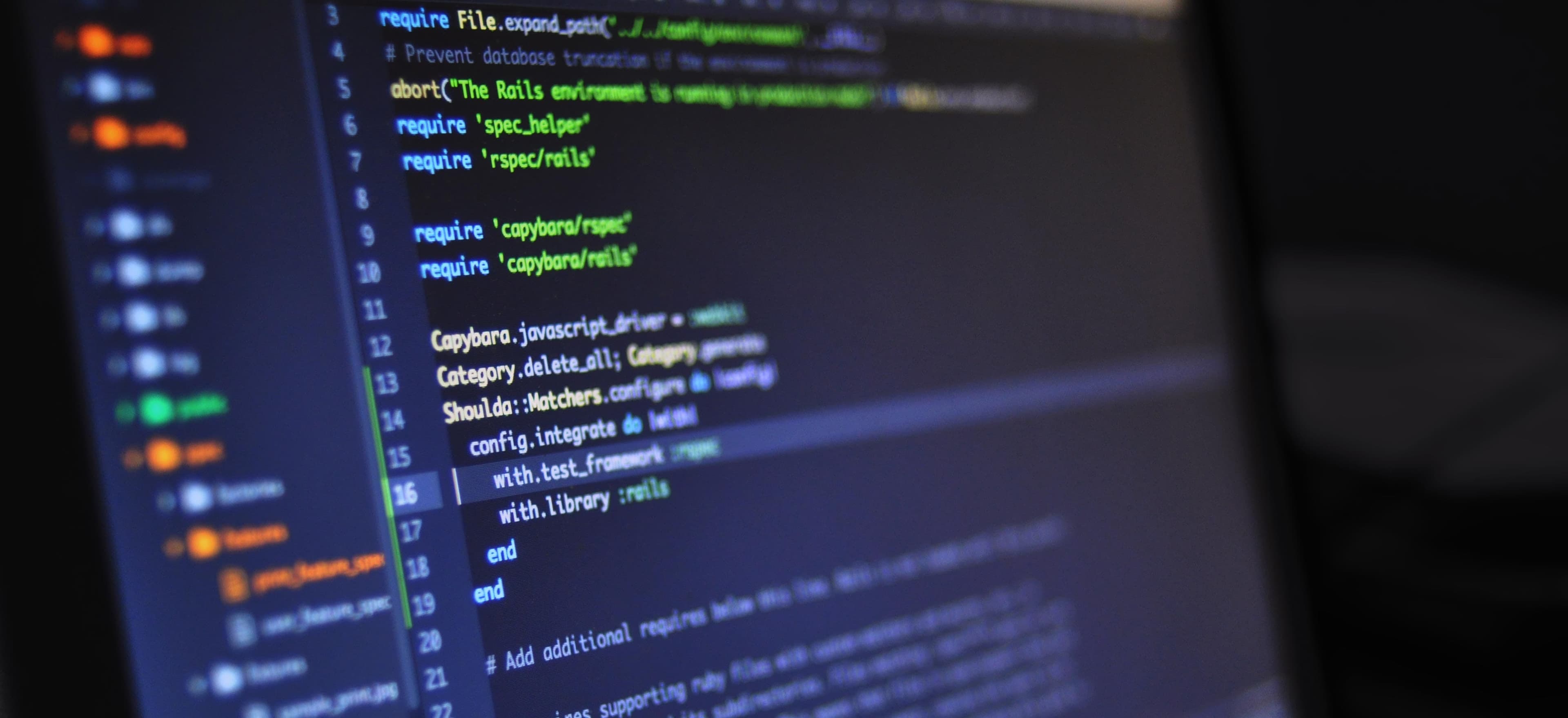
- Published on
Overcoming SimpleDateFormat Issues in JavaFX Applications
When developing JavaFX applications, handling date and time can often lead to complications, especially when using the SimpleDateFormat class. While this class has its merits, shortcomings in thread safety and performance can severely hinder the smooth functioning of your application. In this blog post, we will explore the common issues associated with SimpleDateFormat, discuss better alternatives, and provide code snippets with explanations to help optimize your date and time handling.
The Problem with SimpleDateFormat
Not Thread-Safe
One of the primary issues with SimpleDateFormat is its lack of thread safety. If multiple threads try to use the same instance of SimpleDateFormat concurrently, it could lead to inconsistent results or application crashes.
Performance Drawbacks
Creating a new instance of SimpleDateFormat every time you need to format or parse a date can become inefficient in applications with high-performance needs. This not only adds unnecessary overhead but can also lead to increased memory consumption.
Ambiguous Patterns
SimpleDateFormat often leads to confusion when working with different date formats. It is not always clear what pattern will provide the desired output, especially when dealing with locales.
Example:
SimpleDateFormat formatter = new SimpleDateFormat("dd/MM/yyyy");
String dateString = "31/12/2023";
try {
Date date = formatter.parse(dateString);
System.out.println("Parsed Date: " + date);
} catch (ParseException e) {
e.printStackTrace();
}
In this snippet, we create a SimpleDateFormat instance to parse a date string. However, if multiple threads reach this point simultaneously, it could result in unexpected outcomes.
Better Alternatives
To overcome the issues related to SimpleDateFormat, Java 8 introduced the java.time
package, which is a significant improvement over its predecessor. Specifically, we can leverage DateTimeFormatter
and the LocalDate
, LocalTime
, and LocalDateTime
classes to handle date and time in a more efficient and thread-safe manner.
Advantages of java.time Package
- Thread Safety: The classes in the java.time package are immutable and thread-safe, ensuring that shared usage does not lead to errors.
- Better Performance: They are designed to be more efficient than their predecessors.
- Clearer API: The API is more intuitive, making it easier to work with dates and times.
Transitioning to DateTimeFormatter
Using DateTimeFormatter
, we can simplify our date formatting and parsing process. Let's see an example:
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeParseException;
public class DateParser {
public static void main(String[] args) {
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
String dateString = "31/12/2023";
try {
LocalDate date = LocalDate.parse(dateString, formatter);
System.out.println("Parsed Date: " + date);
} catch (DateTimeParseException e) {
e.printStackTrace();
}
}
}
Commentary on the Code
In this example:
- We define a
DateTimeFormatter
with the desired pattern. - The
LocalDate
class is used to parse the date string, ensuring thread safety. - We handle potential parsing exceptions gracefully.
This example highlights the improved clarity and safety offered by java.time
classes.
Formatting Dates for UI in JavaFX
When building user interfaces in JavaFX, the formatting and display of dates can greatly enhance user experience. By using DateTimeFormatter
, we can easily format dates for UI components.
Example: Formatting for a JavaFX Label
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateDisplayApp extends Application {
@Override
public void start(Stage primaryStage) {
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd MMMM yyyy, hh:mm a");
LocalDateTime now = LocalDateTime.now();
Label dateLabel = new Label("Current Date and Time: " + now.format(formatter));
VBox vbox = new VBox(dateLabel);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setTitle("Date Display Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary on the Code
In this JavaFX application:
- We create a
Label
to display the current date and time. - The
DateTimeFormatter
formats the date to be user-friendly. - Thus, by leveraging the strengths of
java.time
, we maintain clarity while ensuring that our application runs smoothly.
Best Practices for Date Handling in JavaFX
-
Always Use
java.time
: Avoid SimpleDateFormat altogether and stick with the java.time package for all new developments. -
Define Formatters Once: Create a singleton instance of DateTimeFormatter to reuse across your application to reduce instantiation overhead.
-
Handle Time Zones Explicitly: If your application involves users from different time zones, make sure to account for it using ZonedDateTime.
-
Use
Property
Classes: For better integration with JavaFX, consider using JavaFX properties to bind date values to UI components.
Example of Time Zone Handling
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
public class TimeZoneExample {
public static void main(String[] args) {
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy HH:mm Z");
ZonedDateTime zonedDateTime = ZonedDateTime.now(); // Gets current date and time with timezone
System.out.println("Current Date and Time in Time Zone: " + zonedDateTime.format(formatter));
}
}
This code gets the current date and time, including the time zone, and formats it for display.
Additional Resources
To dive deeper into Java's time and date handling, you might find these links helpful:
Lessons Learned
Date and time handling can seem daunting, especially when working in a concurrent environment, but understanding the underlying API can significantly ease the process. By transitioning from SimpleDateFormat to the java.time package, you can create robust, thread-safe, and high-performance JavaFX applications. With the practical examples highlighted, you should now be equipped to handle dates efficiently in your JavaFX projects, paving the way for enhanced user experiences.
Embrace the java.time API, apply the best practices covered, and watch your applications flourish!