Common Mistakes in Spring MVC Select Option Handling
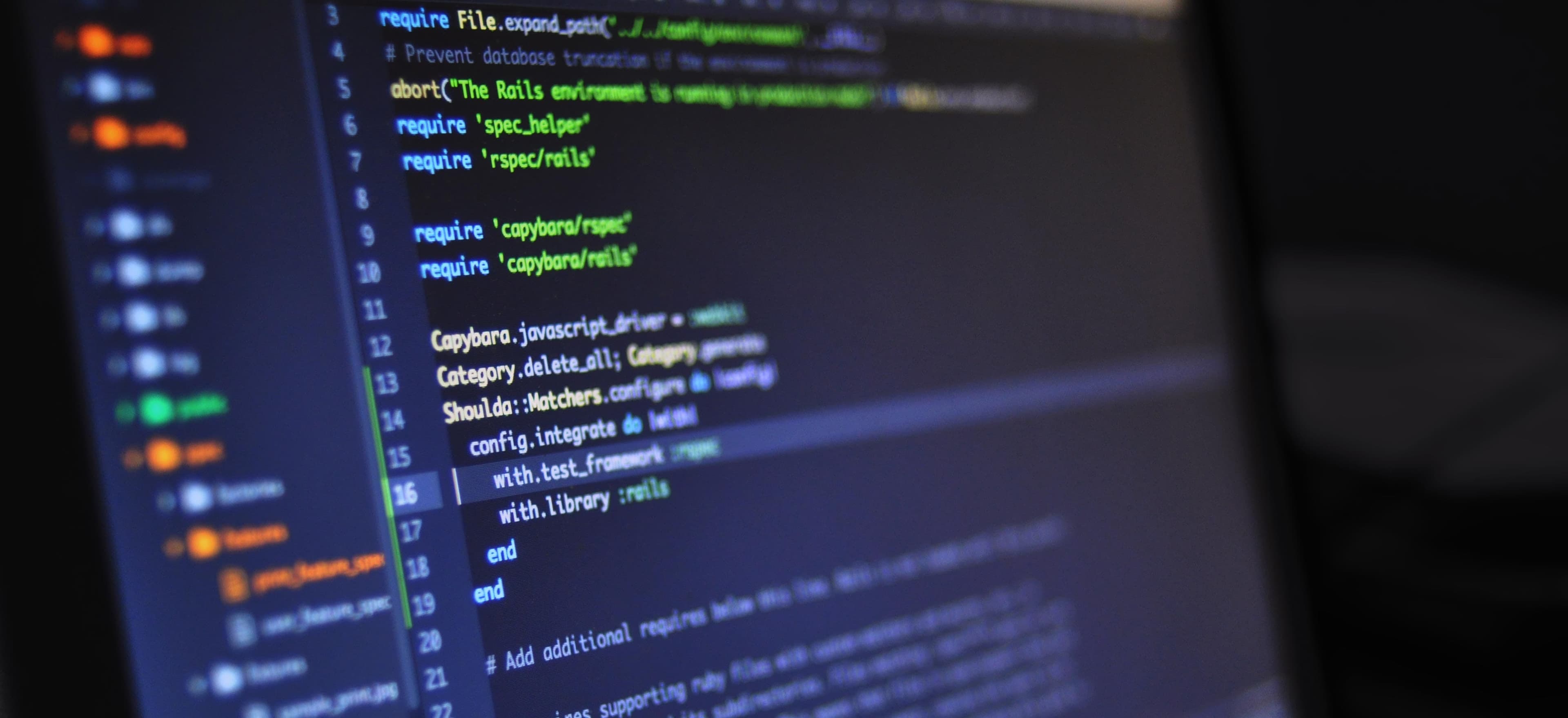
- Published on
Common Mistakes in Spring MVC Select Option Handling
When it comes to building web applications in Java, Spring MVC is one of the most popular frameworks. One task that often trips up developers is handling select options in forms. It's easy to overlook seemingly small details that can lead to significant bugs or undesirable user experiences. Understanding these common mistakes and learning how to avoid them will greatly enhance your Spring MVC skills.
Understanding the Basics
Select options are integral to user input forms, allowing users to choose from predefined values. In Spring MVC, populating these options and properly binding them to your model can be straightforward or quite complex, depending on the use case.
Before we dive into specific mistakes, let’s clarify a typical scenario:
@Controller
public class UserController {
@RequestMapping("/userForm")
public String showForm(Model model) {
model.addAttribute("user", new User());
model.addAttribute("roles", getRoles());
return "userForm";
}
private List<String> getRoles() {
return Arrays.asList("Admin", "Editor", "User");
}
}
In this example, we initialize a new user and pass a list of roles to the model. This list can be rendered as a select option in the view.
Common Mistakes to Avoid
1. Not Binding Selected Value Properly
One of the most common mistakes is failing to bind the selected value back to the model after form submission. Without this binding, the form data won't persist properly.
Here's a common scenario where developers forget to bind the chosen option:
<form action="/submitUser" method="post">
<select name="role" required>
<c:forEach items="${roles}" var="role">
<option value="${role}">${role}</option>
</c:forEach>
</select>
<input type="submit" value="Submit"/>
</form>
In your controller, ensure you handle the incoming role:
@PostMapping("/submitUser")
public String submitUser(@ModelAttribute User user) {
// user.getRole() should contain the selected value if bound correctly
// Save user details
return "redirect:/userSuccess";
}
Failure to properly bind can lead to a situation where user.getRole()
is null or holds an unexpected value.
2. Not Validating User Input
Another frequent misstep is neglecting validation. When input comes from a select option, ensure it's valid before processing.
@PostMapping("/submitUser")
public String submitUser(@ModelAttribute User user, BindingResult result) {
if (result.hasErrors()) {
return "userForm"; // Re-display form with error messages
}
// Continue processing user
}
Spring MVC provides built-in validation annotations that you can utilize, like @NotNull
on your User model fields. For further exploration of validation in Spring MVC, refer to Spring's official documentation.
3. Forgetting to Populate Select Options
Suppose you've set up the form view correctly, but the select options are empty. Often, developers forget or fail to implement the logic to populate these options.
Ensure you’re populating the options correctly within your controller:
model.addAttribute("roles", getRoles());
This mistake may seem minor but can frustrate users as they are unable to select any role.
4. Hardcoding Values Instead of Using Dynamic Lists
Hardcoding roles or any options, while tempting for quick testing, isn’t sustainable.
Instead of:
private List<String> getRoles() {
return Arrays.asList("Admin", "Editor", "User");
}
You should consider fetching these from a database or an external service. This flexibility allows for easier updates and better scalability.
Here's an example using a service to retrieve roles:
@Autowired
private RoleService roleService;
private List<String> getRoles() {
return roleService.findAllRoles();
}
This change ensures the application can evolve over time while keeping the logic centralized.
5. Ignoring User Experience
Another common oversight is not considering how select options impact user experience (UX). Too many options can overwhelm users.
Use a placeholder:
<option value="" disabled selected>Select a role</option>
This small addition enhances UX by guiding the user on selecting an option.
Further, consider grouping related options:
<select name="role">
<optgroup label="Administration">
<option value="Admin">Admin</option>
</optgroup>
<optgroup label="Content Management">
<option value="Editor">Editor</option>
</optgroup>
<optgroup label="Users">
<option value="User">User</option>
</optgroup>
</select>
Properly grouping options makes forms look cleaner and easier to navigate.
Lessons Learned
Handling select options in Spring MVC may seem trivial, yet common pitfalls can lead to significant issues. By binding the selected value correctly, validating user input, properly populating option lists, avoiding hardcoding, and focusing on user experience, you can ensure a smooth user interaction in your applications.
Resources
To deepen your understanding of Spring MVC:
Avoiding these common mistakes can elevate your Spring MVC applications and provide a seamless experience for your users. Happy coding!