Why Static Analysis Can't Fully Replace Code Reviews
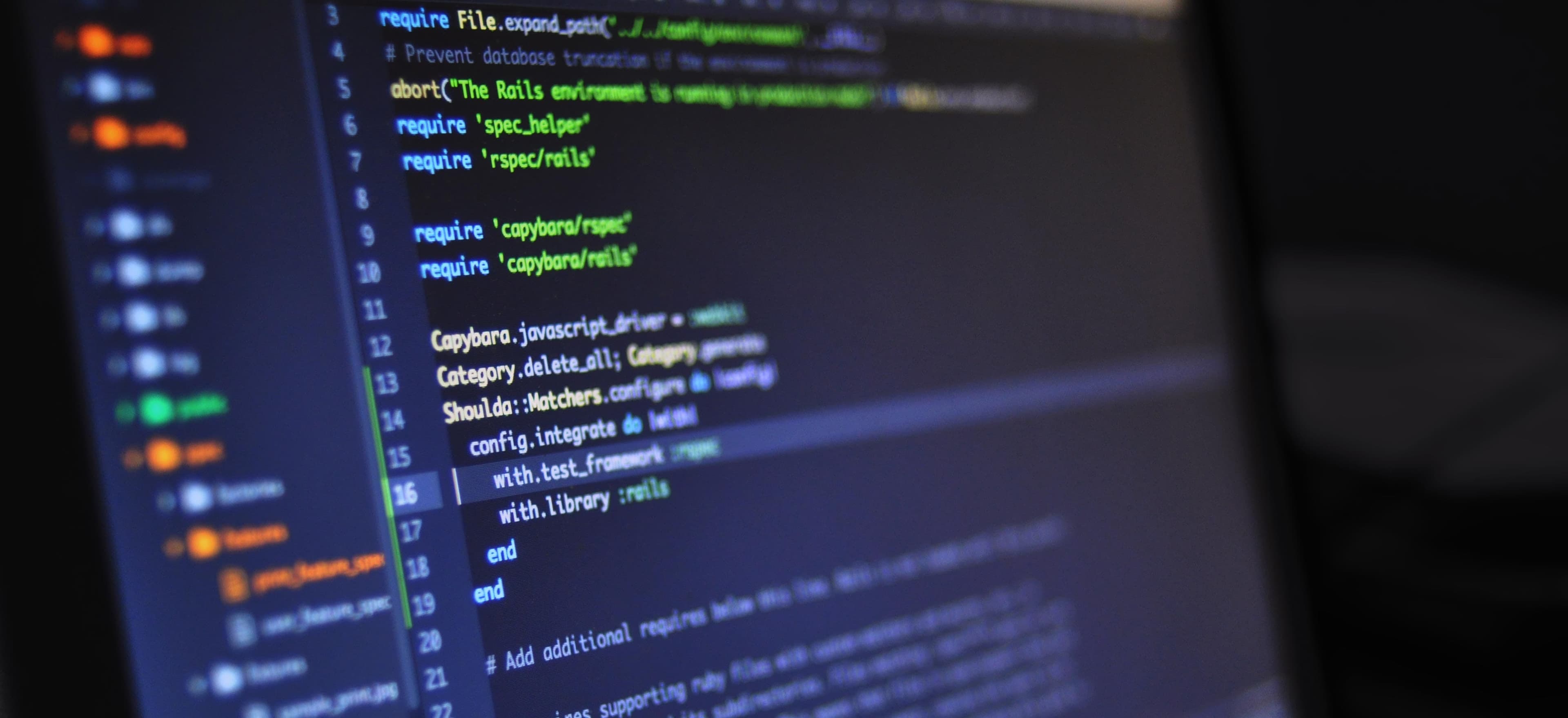
- Published on
Why Static Analysis Can't Fully Replace Code Reviews
In the world of software engineering, ensuring code quality is paramount. While static analysis tools have become essential instruments in our toolkit, we must critically evaluate the limitations of these tools. They can detect certain issues in the code, but they fall short in several areas that human code reviews can address more effectively.
What is Static Analysis?
Static analysis refers to the examination of source code without executing it. By using various tools, developers can identify potential errors, noncompliance with coding standards, and other improvements before the code even runs. This technique is extensively employed in modern development workflows to enhance code quality.
Example of Static Analysis
Consider a simple Java method that contains a possible null pointer exception:
public String getUserName(User user) {
return user.getName(); // Potential NullPointerException
}
A static analysis tool could flag this line for review because if user
is null
, it will lead to a runtime exception. While this is valuable information, it is crucial to complement such findings with deeper insights from a code review.
The Limitations of Static Analysis
Despite their capabilities, static analysis tools are not a panacea. Below are several limitations that warrant proper consideration.
1. Lack of Context
Static analysis tools operate based solely on the code present at a particular moment. They lack the context, such as design decisions, project architecture, or future requirements, that a human reviewer can discern.
For example, a tool might recommend removing a logging statement because it appears unnecessary. However, a developer may know that logging is crucial for debugging in production.
2. Misleading False Positives
Tools often flag issues that do not pose real threats to the code's functionality. Such false positives can result in "alert fatigue," where developers begin ignoring warnings, diluting the value of static analysis.
if (someVariable == null) {
// Some logic here
}
A static analysis tool might flag the condition as problematic even if the context clearly defines someVariable
is only null by design and during specific scenarios that are perfectly handled downstream.
3. Complexity of Business Logic
Business logic introduces complexities that automated tools may not easily interpret. A human reviewer can provide nuanced feedback on complex algorithms, whereas static analysis tools may merely assess the code's correctness without understanding its business implications.
4. Best Practices and Style Guideline
Static analysis tools can analyze code against predefined rules and ensure adherence to coding standards. However, human reviewers often provide insights beyond strict adherence, improving the overall readability and maintainability of the code.
// Following best practices, a more readable version would be:
public String getUserName(User user) {
if (user == null) {
return "Unknown User"; // Graceful handling of null values
}
return user.getName();
}
In this version, clarity is improved, making it easier to understand the logic at a glance, which a static analysis tool might overlook.
Code Reviews Enhance Team Collaboration
Human reviewers enrich the process by facilitating discussions among team members and fostering collaboration. Such interactions lead to shared knowledge, spreading best practices, and ultimately boosting the team's technical acumen.
1. Encouraging Learning and Development
Code reviews are excellent opportunities for junior developers to learn from more experienced developers. Jr. developers can ask questions and receive feedback that tools simply cannot offer, contributing to their professional growth.
2. Promoting Team Cohesion
Involving team members in the review process encourages constructive criticism, where teammates engage in meaningful discussions about each other's contributions. This fosters a sense of community and trust within teams.
Finding the Right Balance: Combining Both Approaches
A hybrid approach, combining static analysis with human code reviews, is essential for optimizing code quality. Here are some practical tips for implementing this combine method effectively:
1. Use Static Analysis Tools to Supplement Reviews
Static analysis tools should be the first line of defense. Use them to catch straightforward issues, allowing code reviewers to focus on higher-level questions such as design choices and architecture.
For instance, after running static analysis, you can focus on critical code segments by emphasizing the areas flagged by the tools as needing review.
2. Establish a Review Checklist
Creating a checklist can aid developers in what to look for during a code review. Include aspects like code clarity, adherence to coding standards, and alignment with project goals. This kind of guidance can help maintain quality and focus during peer reviews.
3. Give Constructive Feedback
When providing feedback during code reviews, practitioners should focus on constructive criticism. Encourage developers to learn from the code review process rather than simply pointing out mistakes.
4. Regularly Update Static Analysis Rules
As the codebase evolves, so should your static analysis rules. Revisiting and updating these rules will help align them with the current project needs, ensuring they stay relevant and effective.
A Final Look
While static analysis tools are valuable in identifying certain types of issues in code, they cannot fully replace the nuanced understanding, feedback, and collaboration brought by comprehensive code reviews. Expect to use both approaches in tandem, creating a culture of quality and continuous learning.
For further insight into static analysis, consider checking SonarQube or Checkstyle, which offer powerful features to enhance your static analysis efforts.
Establishing a healthy relationship between static analysis and code reviews will undoubtedly set your team up for success, improving both code quality and team dynamics.